canvas api
Any code that uses the word context can be simplified. This means, the canvas, as an open source way to make games, art, apps, visualizations and more in HTML with JavaScript, is off to a rough start with its first few lines:
任何使用单词context的代码都可以简化。 这意味着,画布是使用JavaScript制作HTML,用HTML制作游戏,艺术品,应用程序,可视化及更多内容的一种开源方式,它从头几行开始是一个粗略的开始:
var c = document.getElementById("myCanvas");
var ctx = c.getContext("2d");
ctx.beginPath();
ctx.arc(95, 50, 40, 0, 2 * Math.PI);
ctx.stroke();
A big NO THANK YOU to getContext(“2d”)
非常感谢您的getContext(“ 2d”)
Artists and designers who are likely to use the canvas will shy away from these words — I know I did! Also, nobody wants to use document.getElementById()
to access an object! That is the main reason jQuery became so popular with its $(selector)
.
可能使用画布的艺术家和设计师会回避这些话-我知道我做到了! 同样,没有人想要使用document.getElementById()
访问对象! 这是jQuery凭借$(selector)
变得如此流行的主要原因。
画布框架 (Canvas Frameworks)
Enter canvas frameworks to make your life easier. Also see ◎ Your Guide to Selecting a JavaScript Canvas Library or Framework.
输入画布框架,使您的生活更轻松。 另请参见◎ 《选择JavaScript画布库或框架的指南》 。
p5js (p5js)

The p5js framework (a port of Processing originally in Java) provides a setup function which runs at the start and a draw function that loops at the frame rate. You could put the circle code in the setup in this case.
p5js框架 (Java最初是Processing的端口)提供了在开始时运行的设置功能和以帧速率循环的绘制功能。 在这种情况下,您可以将圆圈代码放入设置中。
function setup() {
createCanvas(500, 500);
}function draw() {
background(220);
fill(204, 101, 192, 127);
stroke(127, 63, 120);
circle(100, 100, 50);
}

This is simple but it avoids the traditional JavaScript event structure. I like the versatility of JavaScript events— being able to turn things on and off, go from scene to scene, etc. We need events for working with components and other interactivity. P5js is good for its “SKETCHES” but not optimal for the general Interactive Media described in◎ Your Guide to When to Use a JavaScript Canvas Library or Framework.
这很简单, 但是 避免了传统JavaScript事件结构。 我喜欢JavaScript事件的多功能性-能够打开和关闭事物,从一个场景到另一个场景等等。我们需要事件来处理组件和其他交互性。 P5js的“草图”很好,但对于◎ 《何时使用JavaScript画布库或框架的指南》中 所述的常规交互式媒体却不是最佳选择 。
ZIM (ZIM)

With the ZIM JavaScript Canvas Framework, the traditional JavaScript event structure is maintained with the adjustment that:
使用ZIM JavaScript Canvas Framework ,可以通过以下调整来维护传统JavaScript事件结构:
addEventListener() has been replaced with on()
addEventListener()已替换为on()
const frame = new Frame("fit", 500, 500, light);
frame.on("ready", app);function app() {
const stage = frame.stage;
new Circle(50, pink, purple).loc(100,100);
stage.update();
}
The “fit” will scale the canvas to fit in the Browser window. The light, pink and purple sample colors in ZIM or traditional HTML color strings and hex colors can be used .We could use a function literal or arrow function for the event function. The stage is where we put things to be seen. The loc() method will add to the stage automatically, but any container could be chosen as the next parameter. The manual addition of stage.update() helps conserve battery on mobile and should be called whenever there is a change that needs to be drawn. If we need a draw loop we use a Ticker with uses the JavaScript requestAnimationFrame()
.
“适合”将缩放画布以适合浏览器窗口。 可以使用ZIM中的浅色,粉红色和紫色样本颜色,也可以使用传统HTML颜色字符串和十六进制颜色。事件函数可以使用函数文字或箭头函数。 在此阶段,我们可以看到一些东西。 loc()方法将自动添加到舞台,但是可以选择任何容器作为下一个参数。 手动添加stage.update()有助于节省移动设备上的电池电量,每当需要绘制更改时都应调用此方法。 如果需要绘制循环,可以使用带有JavaScript requestAnimationFrame()
代码的Ticker 。

便利性 (Conveniences)
ZIM has many conveniences to make coding easier for everyone from kids to professionals. For more details, see ◎ Your Guide to Conveniences when Coding on the Canvas.
ZIM具有许多便利,使从孩子到专业人士的每个人都更容易进行编码。 有关更多详细信息,请参见《◎ 在画布上进行编码时的便利性指南》 。
From a “plain English” standpoint let’s talk about ZIM DUO which was launched in ZIM version 2 and is a way that we can use parameters in the traditional format or as a single parameter that is an object literal { } with properties that match the parameter names.
从“普通英语”的角度出发,我们来讨论一下ZIM版本2中启动的ZIM DUO,它是一种我们可以使用传统格式的参数或者将其用作对象常量{}且具有与参数匹配的属性的单个参数的方式。名称。
This is called a configuration object.
这称为配置对象。
Here is the code example from above using configuration objects:
这是上面使用配置对象的代码示例:
const frame = new Frame({
scaling:"fit",
width:500,
height:500,
color:light
});
frame.on("ready", app);function app() {
const stage = frame.stage; new Circle({radius:50, color:pink, borderColor:purple})
.loc(100,100);
stage.update();
}
Now, it is plain to see what the parameters are. It is up to you which way to provide the parameters. Sometimes it is good to see the parameters but at times this will mean more code. At other times, it is less code with the configuration object. For example:
现在,很容易看到参数是什么。 由您决定提供参数的方式。 有时看参数是件好事,但有时这意味着需要更多代码。 在其他时候,配置对象的代码更少。 例如:
new Rectangle(null,null,red,null,null,20).center();
// a corner of 20
new Rectangle({color:red, corner:20}).center();
We need to put null
(or undefined
) for parameter placeholders. With traditional parameters, we would have to keep track of how many nulls and in what order to place the parameters whereas with the configuration object, the order does not matter. Components and other methods often have many parameters so ZIM DUO is very handy.
我们需要为参数占位符放置null
(或undefined
)。 对于传统参数,我们将必须跟踪多少个空值以及以什么顺序放置参数,而对于配置对象,顺序并不重要。 组件和其他方法通常具有许多参数,因此ZIM DUO非常方便。


让我们编码 (Let’s code)
Let’s do a little coding together. A more complete introduction can be found in ◎ Your Guide to Coding Creativity on the Canvas.
让我们一起做一些编码。 可以在◎ 您的画布上的编码创意指南中找到更完整的介绍。

第1步 (Step 1)
We have an Online Editor for ZIM Kids and it is not such a bad thing to think like a kid again as we try something new. Please explore with us. You will get so much more out of this article if you go to the Online Editor, type or paste this code and press TEST:
我们拥有ZIM Kids的在线编辑器 ,在尝试新事物时再次像小孩子一样思考并不是一件坏事。 请与我们一起探索。 如果您转到在线编辑器 ,输入或粘贴此代码,然后按TEST ,则将从本文中获得更多帮助:
new Rectangle(200,300,white)
.center()
.drag();

The stripes in the background are just a backing for the kids site. The editor also has the Frame code in it and a stage.update() by default. Note that we use chaining which allows us to string along methods as each method returns the object. This is a convenience feature of ZIM especially with the added short chainable methods we will see below.
背景中的条纹仅是儿童网站的后盾。 默认情况下,编辑器还具有Frame代码和stage.update()。 请注意,我们使用链接,它允许我们在每个方法返回对象时沿方法串接。 这是ZIM的一项便利功能,尤其是在我们将在下面看到的添加的短可链接方法中。
第2步 (Step 2)
Let’s imagine that the rectangle is a piece of paper that we want to be able to throw in the trash. Adjust the code to the following. Note the short chainable methods sca()
for scale, rot()
for rotation and pos()
for position. These can be set as properties too such as scaleX
, scaleY
, rotation
, x
and y
but the short chainable methods are very convenient! For instance, if we were not going to need references to these objects next, we would not even have to assign them to variables.
假设矩形是一张我们希望能够扔进垃圾桶的纸。 将代码调整为以下内容。 注意,可缩放的短链方法sca()
用于缩放, rot()
用于旋转,而pos()
用于位置。 这些也可以设置为属性,例如scaleX
, scaleY
, rotation
, x
和y
但短链方法非常方便! 例如,如果我们接下来将不需要引用这些对象,则甚至不必将它们分配给变量。
const paper = new Rectangle(200,300,white)
.center()
.drag();
const trash = new Flare({thickness:100})
.sca(.7)
.rot(-90)
.pos(50,50,RIGHT,BOTTOM);

A Flare is an unusual shape unique to ZIM that lets you make shapes that flare out such as this trash can. It is no big deal but the shapes in the picture below are made with Flares.
喇叭形是ZIM特有的不寻常形状,可让您制作出喇叭形的形状,例如垃圾桶。 没什么大不了,但是下图中的形状是用Flares制成的。

第三步 (Step 3)
We need to find out if we have dropped the paper on the trash and if so remove the paper. We would test this on a pressup
event. Add the following code. This time we use an arrow function to specify the function to call when the event happens. Note the stage.update() after removing the paper.
我们需要确定是否将纸张丢到了垃圾箱中,如果有,请取出纸张。 我们将在一次pressup
事件中对此进行测试。 添加以下代码。 这次我们使用箭头函数指定事件发生时要调用的函数。 取出纸张后记下stage.update()。
paper.on("pressup", ()=>{
if (paper.hitTestBounds(trash)) {
paper.removeFrom();
stage.update();
}
});

第4步 (Step 4)
Let’s add an Emitter when we remove the paper. Emitters are great for rewards and effects. Add Emitter code that is paused to start and then we spurt the emitter when we throw out the paper (we no longer need the stage.update() as the emitter does that for us):
取出纸张时,我们添加一个发射器。 发射器非常适合奖励和效果。 添加暂停启动的Emitter代码,然后在抛出纸张时喷出发射器(我们不再需要stage.update(),因为发射器为我们完成了此工作):
const emitter = new Emitter({startPaused:true});paper.on("pressup", ()=>{
if (paper.hitTestBounds(trash)) {
paper.removeFrom();
emitter.loc(paper).spurt(15);
}
});

The Emitter gets located at the paper’s registration point.
发射器位于纸张的注册点。
A registration point is the place in an object where the object gets positioned. It is also the point about which the object will scale and rotate.
注册点是对象中对象所在的位置。 这也是对象缩放和旋转的点。
Let’s demonstrate the registration point with this code adjustment:
让我们通过此代码调整来演示注册点:
new Grid({percent:false});const paper = new Rectangle(200,300,white)
.loc(300,300)
.outline()
.drag();

There is a round red circle at the top left corner of the paper. This means the registration point is at the top left for rectangles and rectangular shaped objects such as buttons and pictures. (The registration point is in the center for circular objects like Circle and Dial.) The paper is located at 300,300 which means that the object is placed so its registration point is at 300,300.
纸张的左上角有一个红色的圆形圆圈。 这意味着注册点在矩形和矩形对象(如按钮和图片)的左上方。 (注册点位于圆形对象(如“圆形”和“刻度盘”)的中心。)纸张位于300,300,这表示放置该对象的位置使其注册点位于300,300。
Let’s get rid of the grid and see what happens when we rotate the rectangle:
让我们摆脱网格,看看旋转矩形时会发生什么:
const paper = new Rectangle(200,300,white)
.loc(300,300)
.outline()
.rot(45)
.drag();

The paper rotates around its registration point. (Scale works the same way.) The outline() is like a snapshot in time. It stays where it was when it was made. If you outline after the rotation then the outline would be rotated with the rectangle.
纸张绕其对位点旋转。 (Scale的工作方式相同。)outline()就像是时间上的快照。 它保留在制作时的位置。 如果在旋转之后绘制轮廓,则轮廓将随矩形一起旋转。
We can change the registration point with regX and regY properties or the reg(x, y) short chainable method but quite often we want to center the registration point so we can just use centerReg(). Try this:
我们可以使用regX和regY属性或reg(x,y)短链可更改方法更改注册点,但是通常我们希望将注册点居中,因此我们可以仅使用centerReg()。 试试这个:
const paper = new Rectangle(200,300,white)
.centerReg()
.loc(300,300)
.outline()
.rot(45)
.drag();

The red circle is in the middle of the rectangle now. The paper rotates around its center which is usually better for animation. The paper has moved up and to the left because the registration point has been adjusted inside the object. The registration point is still at 300, 300.
现在红色圆圈在矩形的中间。 纸张绕其中心旋转,通常更适合动画。 纸张已向上和向左移动,因为已在对象内部调整了对位点。 注册点仍然是300、300。
第5步 (Step 5)
If we want the emitter to show up at center of the paper then we can just centerReg()
the paper rather than center()
the paper. Adjust the code. Now when you throw out the paper the emitter is centered on the paper.
如果我们希望发射器出现在纸张的中心,那么我们可以只对纸张进行centerReg()
而不是对纸张进行center()
。 调整代码。 现在,当您扔掉纸张时,发射器会在纸张上居中。
const paper = new Rectangle(200,300,white)
.centerReg()
.drag();

第6步 (Step 6)
As a final step, we will animate the paper back to the center if the paper is not thrown out. Adjust the code in the pressup event function. Here is the final code:
作为最后一步,如果纸张未抛出,我们将使纸张动画回到中心。 调整pressup事件功能中的代码。 这是最终代码:
const paper = new Rectangle(200,300,white)
.centerReg()
.drag();
const trash = new Flare({thickness:100})
.sca(.7)
.rot(-90)
.pos(50,50,RIGHT,BOTTOM);
const emitter = new Emitter({startPaused:true});paper.on("pressup", ()=>{
if (paper.hitTestBounds(trash)) {
paper.removeFrom();
emitter.loc(paper).spurt(15);
} else {
paper.animate({
props:{x:stageW/2, y:stageH/2, rotation:"360"},
time:.5
});
}
});

The rotation is in quotes because it is relative rotation. So 360 degrees from the current rotation. If we used 360 it would animate to 360 degrees the first time but the second time, it would already be at 360 degrees.
旋转用引号引起来,因为它是相对旋转。 因此从当前旋转角度为360度。 如果我们使用360,则它将在第一时间进行360度动画处理,但第二次将在360度进行动画处理。
动画 (Animation)
At the end of this intro video to ZIM we start showing ZIM compared to other frameworks. ZIM is consistently half the size or better. The reason why is that we try as hard as possible to use plain English.
在本ZIM简介视频的结尾,我们开始展示ZIM与其他框架的对比。 ZIM始终是大小的一半或更好。 原因是我们尽力使用简单的英语。
For instance, here is animation:
例如,这是动画:
new Circle(50,red).center().animate({
props:{x:100, y:100, scale:0},
time:1,
loop:true,
rewind:true
});
In HTML and CSS this is a mess. First of all, there are no circles so you would have to apply a border-radius to a div. Uggh. Then you would be applying a transform in a separate location for the keyframes and this does not include old browser fixes. Basically, ZIM is 25% the size and 38% the size of the animation code.
在HTML和CSS中,这是一团糟。 首先,没有圆,因此您必须将边界半径应用于div。 U 然后,您将在关键帧的单独位置应用转换,并且这不包括旧的浏览器修复程序。 基本上,ZIM的大小是动画代码的25%,是动画代码的38%。
<style>
#circle {
width:100px;
height:100px;
border-radius:50px;
position:absolute;
left:300px;
top:300px;
background-color:red;
animation-name: myCircle;
animation-duration: 1s;
animation-iteration-count: infinite;
animation-direction: alternate;
}
@keyframes myCircle {
to {left:100px; top: 100px; transform:scale(0, 0);}
}
</style>
<div id=circle></div>

Of course, you can use GSAP which is an excellent Tween engine. ZIM and GSAP have very similar animation features. GSAP is chained from a Tween object which is not optimal. For the most part, it is easier to chain to the object you are animating as we can flatten the animation commands to work well with the ZIM DUO technique. ZIM also animates along paths with dragging if desired and the paths are user editable. See the ZIM Intro for an example. And the ZIM NIO (version 9) site for advanced examples.
当然,您可以使用GSAP,它是出色的Tween引擎。 ZIM和GSAP具有非常相似的动画功能。 GSAP是从不是最佳的Tween对象链接而成的。 在大多数情况下,更容易将其链接到要设置动画的对象,因为我们可以展平动画命令以与ZIM DUO技术配合使用。 如果需要,ZIM还可沿路径设置动画,并且用户可以编辑路径。 有关示例,请参见ZIM简介 。 以及ZIM NIO (版本9)站点的高级示例。
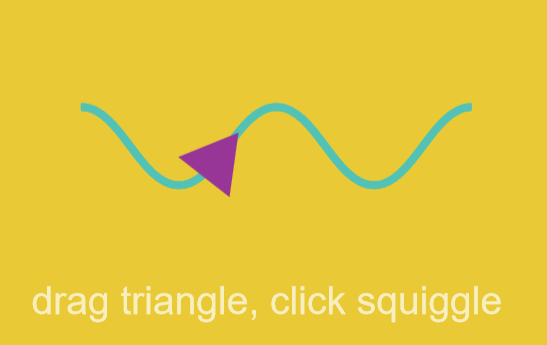
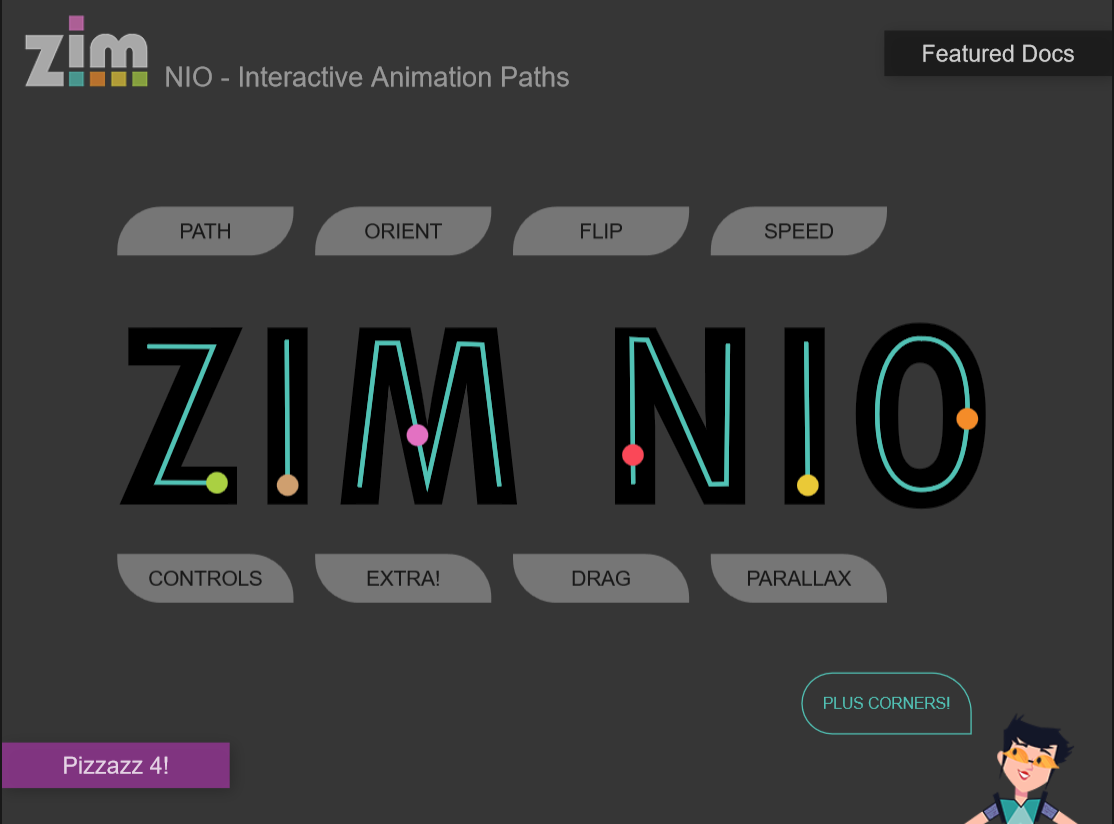
结论 (Conclusion)
We have demonstrated in this article that simple and clear words can be used in code. This makes the coding on the canvas a wonderful place to learn code as well. Here are some further resources: ZIM Skool, ZIM Kids and Code JavaScript with Creative Coding video series.
在本文中,我们证明了可以在代码中使用简单明了的单词。 这也使画布上的编码成为学习代码的绝佳场所。 以下是一些其他资源: ZIM Skool , ZIM Kids和带有创意编码的Code JavaScript视频系列。
Others agree that simple, clear code is the way to go:
其他人同意,简单,清晰的代码是可行的方法:
It has never been easier to create beautiful interactivity with so little code — Iestyn Jones, Founder of eChalk E-learning.
只需编写很少的代码,就可以轻松创建漂亮的交互性-eChalk电子学习的创始人Iestyn Jones。
ZIM makes creating very visual projects easy yet still keeps the traditional coding structures for transferable skills — Rex van der Spuy, Author of Foundation Game Design with HTML5 and JavaScript
ZIM使创建非常直观的项目变得容易,但仍保留了可移植技能的传统编码结构 -Rex van der Spuy,使用HTML5和JavaScript的基础游戏设计的作者
When I show developers ZIM they say they have never found such an easy-to-learn and use platform — Ami Hanya CloseAppGame with over 2 million plays on ZIM games.
当我向开发人员展示ZIM时,他们说他们从未找到过这样一个易于学习和使用的平台 -Ami Hanya CloseAppGame,在ZIM游戏中拥有超过200万的播放次数。
If you would like to explore more about coding on the canvas you are welcome to visit ◎ Your Guide to Coding Creativity on the Canvas.
如果您想了解有关在画布上编码的更多信息,欢迎访问◎ 您在画布上编码创意的指南 。
All the best! Dr Abstract.
祝一切顺利! 抽象博士。

Follow us on Twitter at ZIM Learn and here is ZIM Learn on YouTube!
翻译自: https://medium.com/javascript-in-plain-english/the-canvas-in-plain-english-da6c95bb2bb5
canvas api