In the previous blog of this series we saw some basic concepts related to notifications and also learned how to make a basic notification using channels. In this blog we will take a step further and learn how to add actions to our notifications. We will also see some properties that we can set to have better control of our notification.
在本系列的上一篇博客中 ,我们了解了一些与通知有关的基本概念,还学习了如何使用渠道进行基本通知。 在此博客中,我们将更进一步,并学习如何向通知中添加操作。 我们还将看到一些属性,我们可以设置这些属性以更好地控制通知。
通知动作: (Notification actions:)
Apart from the default tap action of a notification which opens the app we can also set other actions that can be completed directly from the notifications.
除了打开应用程序的通知的默认点击动作外,我们还可以设置其他可以直接从通知中完成的动作。
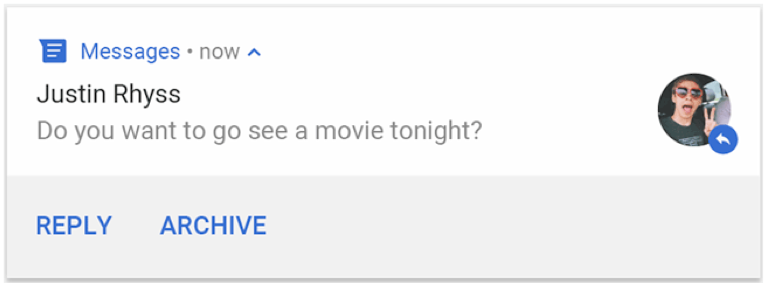
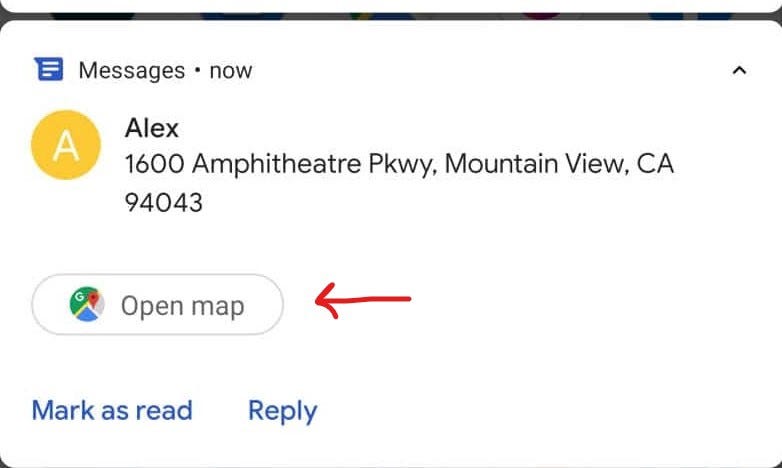
Starting in Android 7.0 (API level 24), you can also add an action to reply to messages or enter other text directly from the notification.
从Android 7.0(API级别24)开始,您还可以添加操作以回复消息或直接从通知中输入其他文本。
Starting in Android 10 (API level 29), the platform can automatically generate action buttons with suggested intent-based actions.
从Android 10(API级别29)开始,该平台可以自动生成带有建议的基于意图的操作的操作按钮。
添加一个动作按钮: (Adding an action button:)
A notification can offer up to three action buttons that allow the user to respond quickly, but these action buttons should not duplicate the action performed when the user taps the notification.
通知最多可以提供三个操作按钮,使用户可以快速响应,但是这些操作按钮不应与用户点击通知时执行的操作重复。
Let’s add an action button in our notification from the previous blog. It is similar to setting the tap action by creating a pending intent only change is that we set it using addAction() to attach it to the notification. We can create intents for both activities or broadcast receivers as shown below:
让我们在上一个博客的通知中添加一个操作按钮。 这与通过创建一个挂起的意图来设置点击动作类似,唯一的变化是我们使用addAction()将其设置为将其附加到通知中。 我们可以为活动或广播接收者创建意图,如下所示:
// adding action for activity
val activityActionIntent = Intent(application, TargetActivity::class.java).apply {
flags = Intent.FLAG_ACTIVITY_NEW_TASK or Intent.FLAG_ACTIVITY_CLEAR_TASK
}
val activityActionPendingIntent: PendingIntent =
PendingIntent.getActivity(application, 0, activityActionIntent, 0)
// adding action for broadcast
val broadcastIntent = Intent(application, MyBroadcastReceiver::class.java).apply {
putExtra("action_msg", "some message for toast")
}
val broadcastPendingIntent: PendingIntent =
PendingIntent.getBroadcast(application, 0, broadcastIntent, 0)
You can create your own BroadcastReciever. Here is a sample that i am using in the above example:
您可以创建自己的BroadcastReciever。 这是我在以上示例中使用的示例:
class MyBroadcastRecieverReceiver : BroadcastReceiver() {
override fun onReceive(context: Context?, intent: Intent?) {
Toast.makeText(context, intent?.getStringExtra("action_msg"), Toast.LENGTH_SHORT).show()
}
}
Then add these actions to the builder like this:
然后将这些操作添加到构建器中,如下所示:
val builder = NotificationCompat.Builder(application, CHANNEL_CODE)
.setSmallIcon(R.mipmap.ic_launcher_round)
.setContentTitle("title")
.setContentText("notification content")
.setStyle(
NotificationCompat.BigTextStyle()
.bigText("long notification content")
)
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
// Set the intent that will fire when the user taps the notification
.setContentIntent(tapPendingIntent)
.setAutoCancel(true)
//for adding action
.addAction(R.drawable.google_plus_icon, "Activity Action", activityActionPendingIntent)
.addAction(R.drawable.google_plus_icon, "Broadcast Action", broadcastPendingIntent)
And that’s all, this will give two action buttons and your defined actions.
就是这样,这将提供两个动作按钮和您定义的动作。
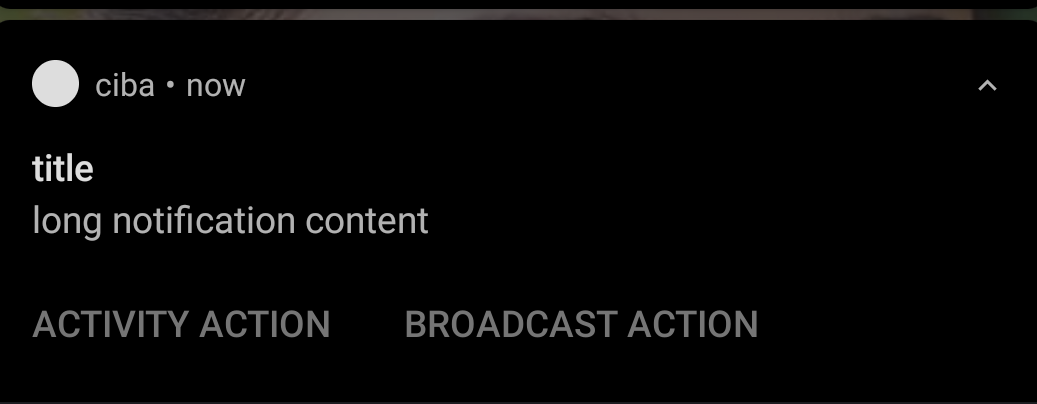
Note: In Android 10 (API level 29) and higher, the platform automatically generates notification action buttons if an app does not provide its own. If you don’t want your app’s notifications to display any suggested replies or actions, you can opt-out of system-generated replies and actions by using
setAllowGeneratedReplies()
andsetAllowSystemGeneratedContextualActions()
.注意:在Android 10(API级别29)及更高版本中,如果应用程序不提供自己的通知,则平台会自动生成通知操作按钮。 如果您不希望应用程序的通知显示任何建议的答复或操作,则可以使用
setAllowGeneratedReplies()
和setAllowSystemGeneratedContextualActions()
退出系统生成的答复和操作。
在通知中添加进度栏: (Add a Progress Bar in Notifications:)
Sometimes in our notifications we need to show the status of an ongoing operation using progress bars. Let’s see how to add one in our notification.
有时,在通知中,我们需要使用进度条显示正在进行的操作的状态。 让我们看看如何在通知中添加一个。
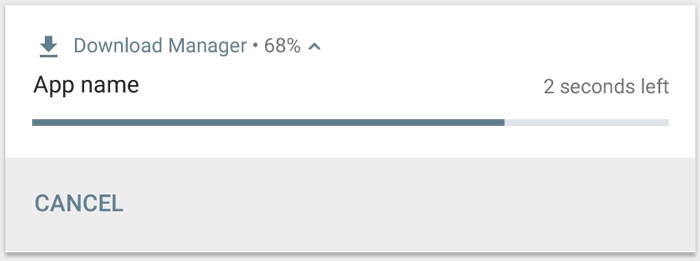
If you can estimate how much of the operation is complete at any time, use the “determinate” form of the indicator (as shown above) by calling setProgress(max, progress, false)
. The first parameter is what the "complete" value is (such as 100); the second is how much is currently complete, and the last indicates this is a determinate progress bar.
如果您可以随时估计要完成多少操作,请通过调用setProgress(max, progress, false)
来使用指标的“确定”形式(如上所示setProgress(max, progress, false)
。 第一个参数是“ complete”值(例如100); 第二个是当前完成的数量,最后一个表明这是一个确定的进度栏。
As your operation proceeds, continuously call setProgress(max, progress, false)
with an updated value for progress
and re-issue the notification as shown below:
随着操作的进行,用更新的progress
值连续调用setProgress(max, progress, false)
并重新发出通知,如下所示:
val PROGRESS_MAX = 100
val PROGRESS_CURRENT = 0
NotificationManagerCompat.from(context).apply {
// Issue the initial notification with zero progress
builder.setProgress(PROGRESS_MAX, PROGRESS_CURRENT, false)
notify(notificationId, builder.build())
// Do the job here that tracks the progress.
// Usually, this should be in a
// worker thread
// To show progress, update PROGRESS_CURRENT and update the notification with:
// builder.setProgress(PROGRESS_MAX, PROGRESS_CURRENT, false);
// notificationManager.notify(notificationId, builder.build());
// When done, update the notification one more time to remove the progress bar
builder.setContentText("Operation complete")
.setProgress(0, 0, false)
notify(notificationId, builder.build())
}
At the end of the operation, progress
should equal max
. You can either leave the progress bar showing when the operation is done or remove it. In either case, remember to update the notification text to show that the operation is complete. To remove the progress bar, call setProgress(0, 0, false)
.
在操作结束时, progress
应等于max
。 您可以让进度条显示操作完成的时间,也可以将其删除。 无论哪种情况,都请记住更新通知文本以表明操作已完成。 要删除进度条,请调用setProgress(0, 0, false)
。
Note: Because the progress bar requires that your app continuously update the notification, this code should usually run in a background service.
注意:由于进度条要求您的应用不断更新通知,因此该代码通常应在后台服务中运行。
To display an indeterminate progress bar (a bar that does not indicate percentage complete), call setProgress(0, 0, true)
. The result is an indicator that has the same style as the progress bar above, except the progress bar is a continuous animation that does not indicate completion. The progress animation runs until you call setProgress(0, 0, false)
and then update the notification to remove the activity indicator.
要显示不确定的进度条(不表示完成百分比的条),请调用setProgress(0, 0, true)
。 结果是指示器具有与上面的进度条相同的样式,除了进度条是不指示完成的连续动画。 进度动画将一直运行,直到您调用setProgress(0, 0, false)
,然后更新通知以删除活动指示器。
Remember to change the notification text to indicate that the operation is complete.
切记更改通知文本以指示操作已完成。
Note: If you actually need to download a file, you should consider using
DownloadManager
, which provides its own notification to track your download progress.注意:如果您实际上需要下载文件,则应考虑使用
DownloadManager
,它提供了自己的通知来跟踪您的下载进度。
设置系统范围的类别: (Set a system-wide Category:)
Android uses some pre-defined system-wide categories to determine whether to disturb the user with a given notification when the user has enabled Do Not Disturb mode.
当用户启用“ 请勿打扰”模式时,Android使用一些预定义的系统范围的类别来确定是否以给定的通知打扰用户。
If your notification falls into one of the pre-defined notification categories defined in NotificationCompat
—such as CATEGORY_ALARM
, CATEGORY_REMINDER
, CATEGORY_EVENT
, or CATEGORY_CALL
—you should declare it as such by passing the appropriate category to setCategory()
in your builder:
如果您的通知属于NotificationCompat
定义的预定义通知类别之一(例如CATEGORY_ALARM
, CATEGORY_REMINDER
, CATEGORY_EVENT
或CATEGORY_CALL
则应通过将适当的类别传递给构建器中的setCategory( )
来声明它:
.setCategory(NotificationCompat.CATEGORY_MESSAGE)
This information about your notification category is used by the system to make decisions about displaying your notification when the device is in Do Not Disturb mode.
当设备处于“请勿打扰”模式时,系统会使用有关通知类别的信息来决定显示通知的决策。
Note: You are not required to set a system-wide category and should only do so if your notifications match one of the categories defined by in
NotificationCompat
.注意:不需要设置系统范围的类别,仅当您的通知与
NotificationCompat
定义的类别之一匹配时,才应进行设置。
控制锁定屏幕可见性: (Control Lock Screen Visibility:)
To control the level of detail visible in the notification from the lock screen, call setVisibility()
and specify one of the following values:
要控制在锁定屏幕上的通知中可见的详细程度,请调用setVisibility()
并指定以下值之一:
VISIBILITY_PUBLIC
shows the notification's full content.VISIBILITY_PUBLIC
显示通知的全部内容。VISIBILITY_SECRET
doesn't show any part of this notification on the lock screen.VISIBILITY_SECRET
不在锁定屏幕上显示此通知的任何部分。VISIBILITY_PRIVATE
shows basic information, such as the notification's icon and the content title, but hides the notification's full content.VISIBILITY_PRIVATE
显示基本信息,例如通知的图标和内容标题,但隐藏通知的全部内容。
Note: These can be accessed from the NotificationMangerCompact class. For example NotificationMangerCompact.VISIBILITY_PUBLIC.
注意:可以从NotificationMangerCompact类访问它们。 例如,NotificationMangerCompact.VISIBILITY_PUBLIC。
When VISIBILITY_PRIVATE
is set, you can also provide an alternate version of the notification content which hides certain details. For example, an SMS app might display a notification that shows You have 3 new text messages but hides the message contents and senders. To provide this alternative notification, first create the alternative notification with NotificationCompat.Builder
as usual. Then attach the alternative notification to the normal notification with setPublicVersion()
.
设置VISIBILITY_PRIVATE
,您还可以提供隐藏某些详细信息的通知内容的备用版本。 例如,一个SMS应用程序可能会显示一条通知,该通知显示您有3条新短信,但隐藏了消息内容和发件人。 要提供此替代通知,请像往常一样先使用NotificationCompat.Builder
创建替代通知。 然后使用setPublicVersion()
将替代通知附加到常规通知。
However, the user always has final control over whether their notifications are visible on the lock screen and can even control that based on your app’s notification channels.
但是,用户始终可以最终控制他们的通知是否在锁定屏幕上可见,甚至可以根据您应用的通知渠道进行控制。
更新通知 (Update a notification)
To update this notification after you’ve issued it, call NotificationManagerCompat.notify()
again, passing it a notification with the same ID you used previously. If the previous notification has been dismissed, a new notification is created instead.
要在发出通知后对其进行更新,请再次调用NotificationManagerCompat.notify()
,并向其传递一个具有与先前使用的相同ID的通知。 如果先前的通知已被取消,则会创建一个新的通知。
You can optionally call setOnlyAlertOnce()
so your notification interrupts the user (with sound, vibration, or visual clues) only the first time the notification appears and not for later updates.
您可以选择调用setOnlyAlertOnce()
以便您的通知仅在第一次出现通知时才中断用户(具有声音,振动或视觉提示),而不会在以后进行更新。
Note: Android applies a rate limit when updating a notification. If you post updates to a notification too frequently (many in less than one second), the system might drop some updates.
注意: Android在更新通知时会应用速率限制。 如果您过于频繁地发布更新通知(许多时间少于一秒钟),则系统可能会丢弃某些更新。
删除通知 (Remove a notification)
Notifications can be canceled in the following ways:
可以通过以下方式取消通知:
- The user dismisses the notification. 用户关闭该通知。
The user clicks the notification, and you called
setAutoCancel()
when you created the notification.用户单击通知,然后在创建通知时调用
setAutoCancel()
。You call
cancel()
for a specific notification ID. This method also deletes ongoing notifications.您为特定的通知ID调用
cancel()
。 此方法还删除正在进行的通知。You call
cancelAll()
, which removes all of the notifications you previously issued.您调用
cancelAll()
,它将删除您先前发出的所有通知。If you set a timeout when creating a notification using
setTimeoutAfter()
, the system cancels the notification after the specified duration elapses. If required, you can cancel a notification before the specified timeout duration elapses.如果使用
setTimeoutAfter()
创建通知时设置了超时,则系统会在经过指定的持续时间后取消通知。 如果需要,您可以在指定的超时时间过去之前取消通知。
This is all for this blog, we will see how to create expandable and time-sensitive(urgent) notifications in the next blog. That will also cover the concept of notification groups.
这是本博客的全部内容,我们将在下一个博客中了解如何创建可扩展且时间敏感(紧急)的通知。 这还将涵盖通知组的概念。
Feel free to comment if there are doubts or if you feel anything needs to be corrected😀.
如果有任何疑问或您觉得需要纠正任何问题,请随时发表评论😀。
翻译自: https://medium.com/swlh/android-notifications-2-adding-actions-and-modifying-properties-33db057fcc58