单元测试编写
As software developers we all know how important it is to unit test the code that we write. Some of us write tests that verify the happy paths only (bad) and others look for high code coverage numbers. But how many of you are actually writing thorough tests? Let’s take a look at what this means.
作为软件开发人员,我们都知道对所编写的代码进行单元测试的重要性。 我们中的一些人编写的测试仅验证(不正确的)快乐路径,而另一些人则寻找较高的代码覆盖率数字。 但是实际上你们当中有多少人在编写全面的测试? 让我们看看这意味着什么。
Thorough - Test normal & failure conditions, invalid inputs, and boundary conditions.
彻底-测试正常和故障条件,无效输入和边界条件。
Repeatable - Tests must always give you the same results every time so avoid depending on things that might change such as an external server.
可重复-测试每次都必须始终为您提供相同的结果,因此请避免依赖可能发生变化的事物(例如外部服务器)。
Focused - Tests should exercise one specific aspect of your code at a time. A failure in a unit test should lead you to an actual bug in your code.
重点突出-测试应该一次练习代码的一个特定方面。 单元测试失败会导致您在代码中遇到实际错误。
Verifies Behavior - Tests should test behavior without making assumptions on implementations. Avoid over mocking with Mockito.
验证行为-测试应在不对实现进行假设的情况下测试行为。 避免与Mockito过度嘲笑。
Fast - Tests should be fast since 70% of your tests should consist of unit tests.
快速-测试应该快速,因为70%的测试应该包含单元测试。
Concise - Tests should be easy to understand and be a clear blueprint of what the code under test is doing.
简洁-测试应该易于理解,并且是被测代码正在做什么的清晰蓝图。
测试1:不可重复 (Test 1: Not Repeatable)
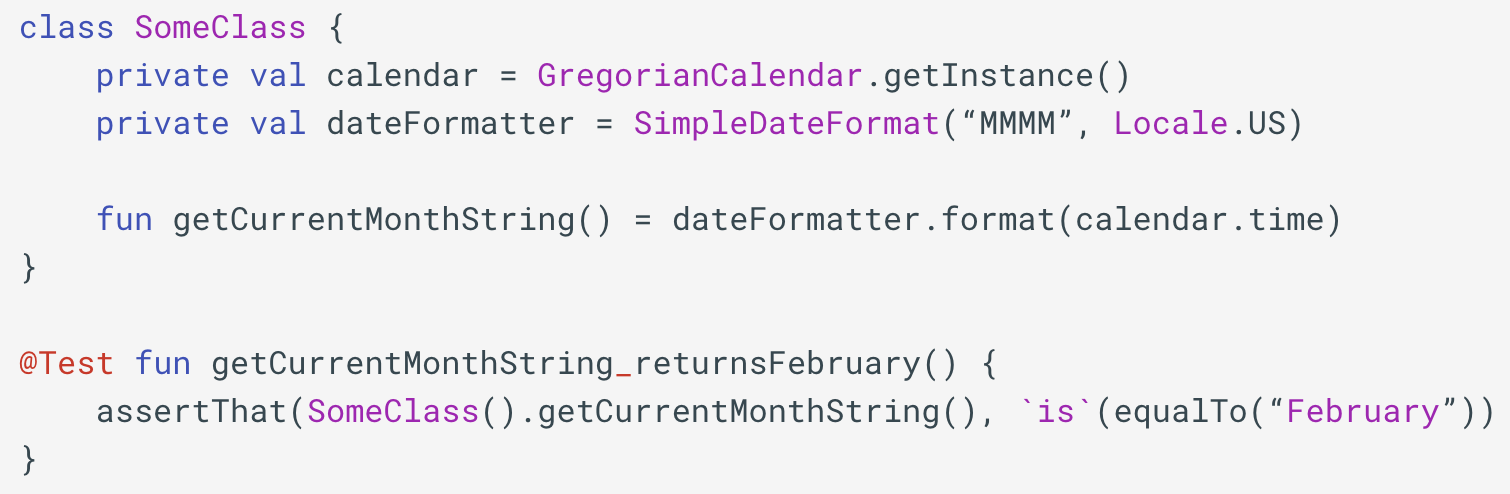
Above we see a test that is testing SomeClass#getCurrentMonthString. Way too often I see this mistake made during code reviews and it violates the repeatability characteristic. The reason is the default locale, timezone, and system time are being used. This means 1 month from now this test will return March instead of February which will instantly begin failing.
在上方,我们看到一个测试SomeClass#getCurrentMonthString的测试。 我经常看到此错误是在代码审查期间犯的,它违反了可重复性特征。 原因是使用了默认语言环境,时区和系统时间。 这意味着从现在开始的1个月内该测试将返回3月而不是2月,而2月将立即开始失败。
Let’s see how this same test looks with these issues fixed.
让我们看看修复了这些问题后的测试结果。