android读取文件内容
学习Android开发 (Learning Android Development)
If you search on how can Android read a text file, you can find several solutions on Stackoverflow. However, they all need context
to read the file either from res
or asset
folder.
如果您搜索Android如何读取文本文件,则可以在Stackoverflow上找到几种解决方案。 但是,它们都需要context
来从res
或asset
文件夹中读取文件。
// file store in /assets
val inputStream = context.assets.open("test.txt")// or file store in /res/raw
val inputStream = context.resources.openRawResource(R.raw.test)
In unit testing, we will like to avoid using context
. With that, how can we read from a file?
在单元测试中,我们将避免使用context
。 这样,我们如何读取文件?
E.g. will be useful for doing Mock Web Service test as below
例如,对于进行如下所示的Mock Web Service测试将非常有用
Java classLoader来解救 (Java classLoader came to rescue)
Apparently we can read a file using classLoader
显然我们可以使用classLoader
读取文件
val inputStream = javaClass.classLoader?.getResourceAsStream(fileName)
With this, we can read the file from the resources
folder, without needing any Context.
这样,我们可以从resources
文件夹中读取文件,而无需任何上下文。
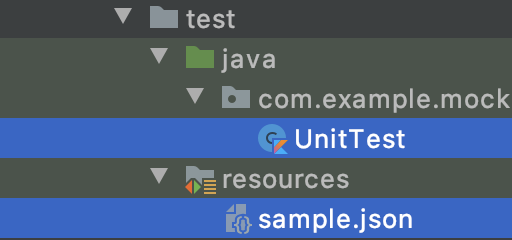
Below is the detail of how to read the file just to provide complete reference
下面是如何阅读文件的详细信息,仅提供完整的参考
读取JSON样式文件 (Reading JSON style file)
Assuming we just need to read JSON file, so we don’t care about the next line, then we can just use readLine()
假设我们只需要读取JSON文件,所以我们不在乎下一行,那么我们可以使用readLine()
@Throws(IOException::class)
fun readFileWithoutNewLineFromResources(fileName: String): String {
var inputStream: InputStream? = null
try {
inputStream =
javaClass.classLoader?.getResourceAsStream(fileName)
val builder = StringBuilder()
val reader = BufferedReader(InputStreamReader(inputStream))
var str: String? = reader.readLine()
while (str != null) {
builder.append(str)
str = reader.readLine()
}
return builder.toString()
} finally {
inputStream?.close()
}
}
This will exclude all \n
, but it is good enough for some cases (e.g. JSON formated content).
这将排除所有\n
,但是在某些情况下(例如JSON格式的内容)就足够了。
读取所有字符串内容 (Reading All String Content)
If one would like to include all characters including \n
, then we’ll need to use read()
instead
如果要包含所有字符,包括\n
,那么我们需要使用read()
代替
@Throws(IOException::class)
fun readFileWithNewLineFromResources(fileName: String): String {
var inputStream: InputStream? = null
try {
inputStream =
javaClass.classLoader?.getResourceAsStream(fileName)
val builder = StringBuilder()
val reader = BufferedReader(InputStreamReader(inputStream))
var theCharNum = reader.read()
while (theCharNum != -1) {
builder.append(theCharNum.toChar())
theCharNum = reader.read()
}
return builder.toString()
} finally {
inputStream?.close()
}
}
Updated: A shorter version
更新:较短的版本
Refer here for the explanation
请参考这里的说明
@Throws(IOException::class)
fun readFileWithNewLineFromResources(fileName: String): String {
return getInputStreamFromResource(fileName)?.bufferedReader()
.use { bufferReader -> bufferReader?.readText() } ?: ""}
读取二进制文件 (Reading Binary File)
In case the file is binary, you can do as below
如果文件是二进制文件,则可以执行以下操作
@Throws(IOException::class)
fun readBinaryFileFromResources(fileName: String): ByteArray {
var inputStream: InputStream? = null
val byteStream = ByteArrayOutputStream()
try {
inputStream =
javaClass.classLoader?.getResourceAsStream(fileName)
var nextValue = inputStream?.read() ?: -1
while ( nextValue != -1 ) {
byteStream.write(nextValue)
nextValue = inputStream?.read() ?: -1
}
return byteStream.toByteArray()
} finally {
inputStream?.close()
byteStream.close()
}
}
To convert ByteArray to String, just use
String(byteArray)
will do.要将ByteArray转换为String,只需使用
String(byteArray)
。
Updated: A shorter version
更新:较短的版本
Refer here for the explanation
请参考这里的说明
fun readBinaryFileFromResources(fileName: String): ByteArray {
ByteArrayOutputStream().use { byteStream ->
getInputStreamFromResource(fileName)?.copyTo(byteStream)
return byteStream.toByteArray()
}}
android读取文件内容