If you are a new developer you must have heard about this dragon called testing that needs slaying. If you have some experience you probably know why it’s so important to add tests to your project as soon as possible. So without getting into why it’s important, let's get straight into the how. In this article, you will be introduced to the basics of writing tests for an Android app that we will develop.
如果您是新开发人员,那么您一定听说过这条需要杀死的巨龙。 如果您有一定的经验,您可能会知道为什么尽快将测试添加到项目中如此重要。 因此,在不弄清楚它为什么重要的情况下,让我们直接看一下如何做。 在本文中,将向您介绍为我们将开发的Android应用编写测试的基础。
We will start an empty Android project. If you wish to follow along you can check out the code for empty Android project here.
我们将启动一个空的Android项目。 如果您希望继续学习,可以在此处签出空白Android项目的代码。
First we will write an Instrument test for our app. Instrumented unit tests are tests that run on physical devices and emulators. To read more about Instrumented tests you can checkout official documentation here.
首先,我们将为我们的应用程序编写一个Instrument测试。 仪器化的单元测试是在物理设备和仿真器上运行的测试。 要了解有关仪器测试的更多信息,可以在此处查看官方文档。
Let’s get started…
让我们开始吧…
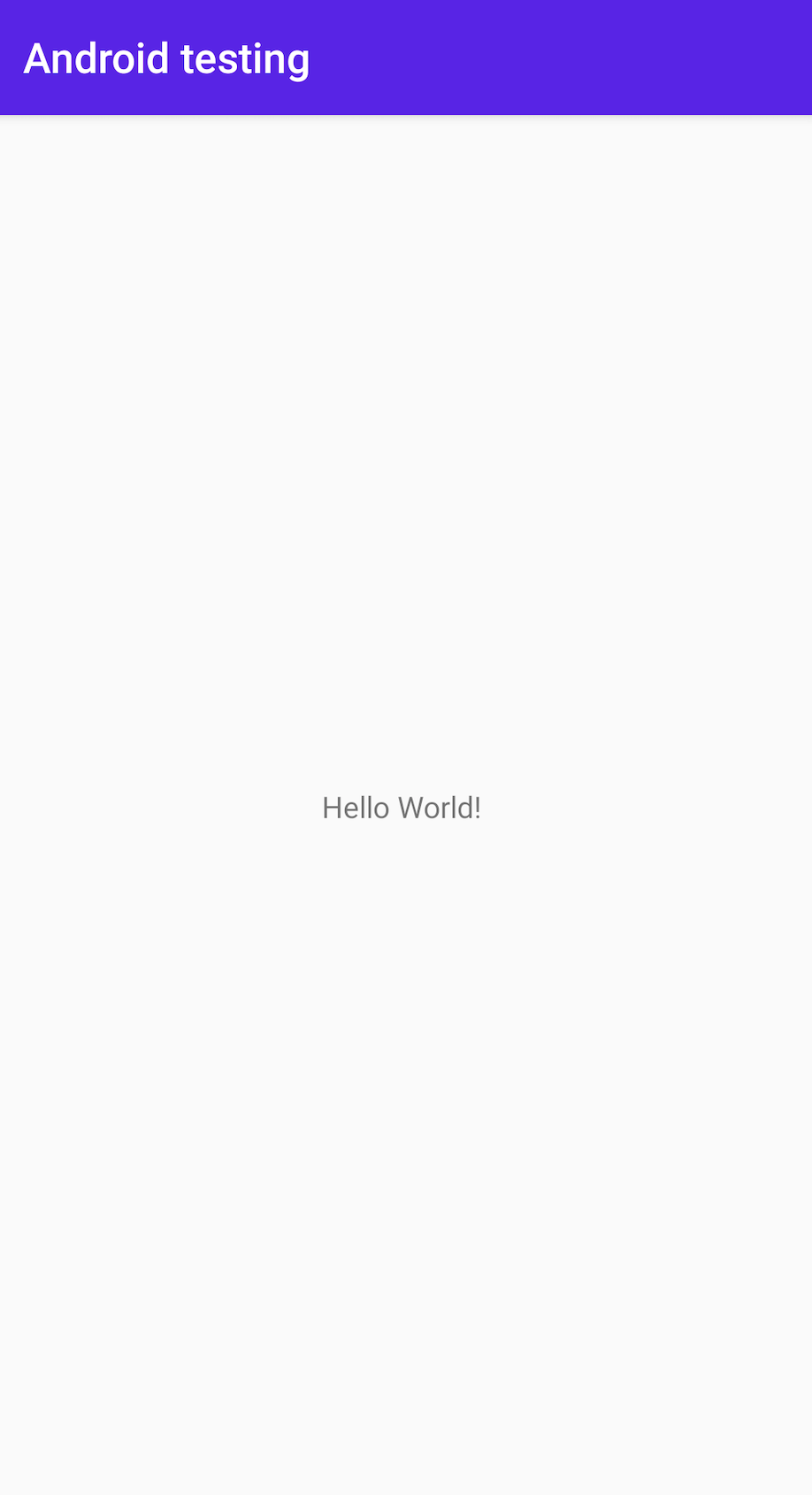
In this app we have a TextView which says “Hello World”.
在这个应用程序中,我们有一个TextView,上面写着“ Hello World”。
We will now change the text in the app and then we will see if we can test whether the code we have written can be tested.
现在,我们将在应用程序中更改文本,然后查看是否可以测试编写的代码是否可以测试。
Go to the strings.xml file in app resources and add a new string resource. Let’s call it string_to_be_tested.
转到应用程序资源中的strings.xml文件,然后添加一个新的字符串资源。 我们称它为string_to_be_tested。
<string name="string_to_be_tested">We are going to test whether this string is shown in our App.</string>
Now go to your MainAcitivity’s layout file activity_main.xml and set the value of TextView to this string resource and we will also add an id attribute to our TextView.
现在转到您的MainAcitivity的布局文件activity_main.xml并将TextView的值设置为此字符串资源,我们还将向我们的TextView添加一个id属性。
<TextView
android:id="@+id/text_container"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/string_to_be_tested" />
An id attribute allows us to uniquely identify our TextView on the screen.
id属性使我们可以在屏幕上唯一标识TextView。
Now run the app and you will see the text in the app in place of Hello world.
现在运行该应用程序,您将在应用程序中看到文本代替Hello world。
Before we begin writing our test we need to understand what we are testing. In our app we show a text in a TextView and the text shown is present as a string resource.
在开始编写测试之前,我们需要了解我们正在测试的内容。 在我们的应用程序中,我们在TextView中显示文本,并且显示的文本作为字符串资源显示。
Our test will verify that the string present as resource is shown on the screen when the app is opened.
我们的测试将验证打开应用程序时屏幕上是否显示了作为资源显示的字符串。
To begin our testing process, first we need to add some dependencies. In our app’s top-level build.gradle
file, we need to specify these libraries as dependencies:
要开始测试过程,首先我们需要添加一些依赖项。 在我们应用程序的顶级build.gradle
文件中,我们需要将这些库指定为依赖项:
dependencies {
androidTestImplementation 'androidx.test:runner:1.1.0'
androidTestImplementation 'androidx.test:rules:1.1.0'
// Optional -- Hamcrest library
androidTestImplementation 'org.hamcrest:hamcrest-library:1.3'
// Optional -- UI testing with Espresso
androidTestImplementation 'androidx.test.espresso:espresso-core:3.1.0'
// Optional -- UI testing with UI Automator
androidTestImplementation 'androidx.test.uiautomator:uiautomator:2.2.0'
}
To use JUnit 4 test classes, make sure to specify AndroidJUnitRunner
as the default test instrumentation runner in your project by including the following setting in your app's module-level build.gradle
file:
要使用JUnit 4测试类,请通过在应用程序的模块级build.gradle
文件中包含以下设置,确保将AndroidJUnitRunner
指定为项目中的默认测试工具build.gradle
器:
android {
defaultConfig {
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
}
The above dependency part is taken as is from official documentation. You can read the official documentation here. https://developer.android.com/training/testing/unit-testing/instrumented-unit-tests.
以上依赖项部分取自官方文档。 您可以在此处阅读官方文档。 https://developer.android.com/training/testing/unit-testing/instrumented-unit-tests 。
Now go to project tile on left side in Android studio and from the dropdown on the top, select “Android” and then open app->Java and look for the app’s package name with (androidtest) on the right side.
现在转到Android Studio左侧的项目图块,然后从顶部的下拉列表中选择“ Android”,然后打开app-> Java,并在右侧使用(androidtest)查找该应用的包名称。
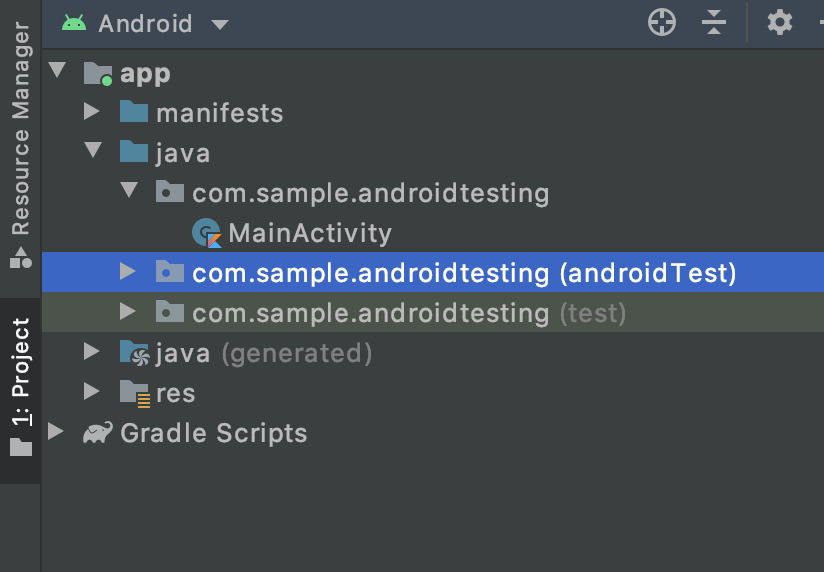
Now create a new class in the package selected in Blue above.
现在,在上面蓝色中选择的包中创建一个新类。
Since we are testing MainActivity, Let’s call the class MainActivityTest. In this class create a function that will contain the testing code.
由于我们正在测试MainActivity,因此我们将其称为MainActivityTest类。 在此类中,创建一个包含测试代码的函数。
package com.sample.androidtesting
import org.junit.Test
class MainActivityTest {
@Test
fun checkIfStringIsCorrect() {
}
}
At this point you can run the empty test and you will see that your test passes.
此时,您可以运行空测试,您将看到测试通过。
Now let’s add some code to this class. First we need to specify which Activity we will be testing. So we will create a rule that tells the test runner which Activity to launch.
现在让我们向此类添加一些代码。 首先,我们需要指定要测试的活动。 因此,我们将创建一条规则,告诉测试运行者启动哪个活动。
@get:Rule
var activityRule: ActivityScenarioRule<MainActivity> =
ActivityScenarioRule(MainActivity::class.java)
Now we need to get the value of string from our resources so we can use the value to match with the text in our TextView. To get the string we need to create another rule to get an instance of resources.
现在,我们需要从资源中获取字符串的值,以便可以使用该值与TextView中的文本进行匹配。 要获取字符串,我们需要创建另一个规则以获取资源实例。
var resources: Resources = InstrumentationRegistry.getInstrumentation().targetContext.resourcesprivate lateinit var stringToBeChecked: String
@Before
fun getStringFromResources() {
stringToBeChecked = resources.getString(R.string.string_to_be_tested)
}
Before annotation allows getStringFromResources() method to be called before our test function checkIfStringIsCorrect() is executed. This way we can initialize any variables required for running our tests.
在批注允许执行测试功能checkIfStringIsCorrect()之前调用getStringFromResources()方法之前。 这样,我们可以初始化运行测试所需的任何变量。
Now to test the value of text in our TextView.
现在在我们的TextView中测试文本的值。
@Test
fun checkIfStringIsCorrect() {
onView(withId(R.id.text_container)).check(matches(withText(stringToBeChecked)))
}
Let’s analyse the code we added here
让我们分析一下我们在此处添加的代码
onView(withId(R.id.text_container))
In this part we are looking for a view with id attribute equal to text_container. onView returns a ViewInteraction for view which matches with condition specoified as it’s arguments.
在这一部分中,我们正在寻找一个id属性等于text_container的视图。 onView返回View的ViewInteraction,该View与指定为参数的条件匹配。
withId(R.id.text_container)
A matcher that matches Views based on its resource id.
根据资源ID匹配视图的匹配器。
check(matches(withText(stringToBeChecked)))
When we have the ViewInteraction, we call check with ViewAssertion. Check() method Checks the given ViewAssertion on the the view selected by the current view matcher.
当我们拥有ViewInteraction时,我们使用ViewAssertion调用check。 Check()方法在当前视图匹配器选择的视图上检查给定的ViewAssertion。
matches(withText(stringToBeChecked))
This view assertion checks whether the text in TextView matches with the value of stringToBeChecked. matches() method returns a matcher that matches TextView based on its text property value.
此视图断言检查TextView中的文本是否与stringToBeChecked的值匹配。 matchs()方法返回一个匹配器,该匹配器根据其text属性值匹配TextView。
The combined code for this class is here
此类的组合代码在这里
package com.sample.androidtesting
import android.content.res.Resources
import androidx.test.espresso.Espresso.onView
import androidx.test.espresso.assertion.ViewAssertions.matches
import androidx.test.espresso.matcher.ViewMatchers.withId
import androidx.test.espresso.matcher.ViewMatchers.withText
import androidx.test.ext.junit.rules.ActivityScenarioRule
import androidx.test.filters.LargeTest
import androidx.test.platform.app.InstrumentationRegistry
import org.junit.Before
import org.junit.Rule
import org.junit.Test
@LargeTest
class MainActivityTest {
@get:Rule
var activityRule: ActivityScenarioRule<MainActivity> =
ActivityScenarioRule(MainActivity::class.java)
var resources: Resources = InstrumentationRegistry.getInstrumentation().targetContext.resourcesprivate lateinit var stringToBeChecked: String
@Before
fun getStringFromResources() {
stringToBeChecked = resources.getString(R.string.string_to_be_tested)
}
@Test
fun checkIfStringIsCorrect() {
onView(withId(R.id.text_container)).check(matches(withText(stringToBeChecked)))
}
}
That’s it. We have written a test that can ensure the simple behavior of our app. You can checkout the final code here
而已。 我们编写了一个测试,可以确保我们应用程序的简单行为。 您可以在此处签出最终代码
You can read the second part of this article here
您可以在这里阅读本文的第二部分
翻译自: https://medium.com/swlh/getting-started-with-android-testing-63a95c3d3576