“If Java had true garbage collection, most programs would delete themselves upon execution” -Robert Sewell
“如果Java拥有真正的垃圾回收,那么大多数程序将在执行时删除自己” -Robert Sewell
The process that Java programs perform when managing automatic memory management is called “garbage collection in Java”. When a Java program runs on the JVM, the heap keeps the objects that had been created. When the programs run, some objects will no longer be needed to run the program. The garbage collector finds these unreachable, unused objects and removes them in order to free up memory. The biggest benefit of the Java garbage collection is that it automatically handles the deletion of the unused, unreachable objects from the root node. Garbage collection is a daemon thread in Java. When the heap runs out of space we get an exception called a “java.lang.OutOfMemoryError”.
Java程序在管理自动内存管理时执行的过程称为“ Java垃圾收集”。 当Java程序在JVM上运行时,堆将保留已创建的对象。 程序运行时,不再需要某些对象来运行程序。 垃圾收集器会找到这些无法访问的未使用对象,并删除它们以释放内存。 Java垃圾回收的最大好处是,它可以自动处理从根节点删除未使用的,无法访问的对象。 垃圾回收是Java中的守护程序线程。 当堆空间不足时,我们会收到一个称为“ java.lang.OutOfMemoryError”的异常。
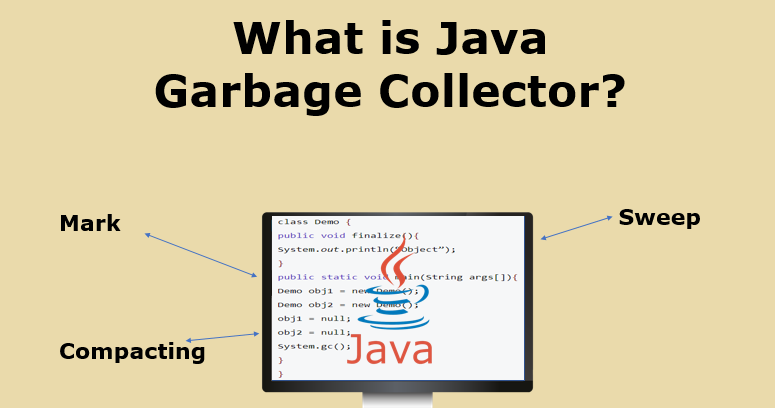
There are three steps in the Garbage Collector Process:
垃圾收集器过程包含三个步骤:
Mark: In this step, the garbage collector goes through the root node of the application and marks the unreachable, unused objects in the Heap.
标记:在此步骤中,垃圾收集器遍历应用程序的根节点,并在堆中标记不可达的未使用对象。
Delete/Sweep: This step deletes the unreachable objects from the heap
删除/清除:此步骤将从堆中删除无法访问的对象
Compacting: After deleting the unreachable objects, the remaining objects are everywhere in the heap. So they need to be arranged to correct order and free up space for the heap.
压缩:删除无法访问的对象后,其余对象在堆中无处不在。 因此,需要安排它们以更正顺序并释放堆空间。
Please note that when we need more space in the hard disk of the computer we can perform disk cleanup action. This process will arrange the files into the correct order, thereby gaining more space.) This compacting process is a time-consuming process.
请注意,当我们在计算机硬盘上需要更多空间时,可以执行磁盘清理操作。 此过程将按正确的顺序排列文件,从而获得更多空间。)此压缩过程非常耗时。
垃圾收集器的过程 (Process of the Garbage Collector)
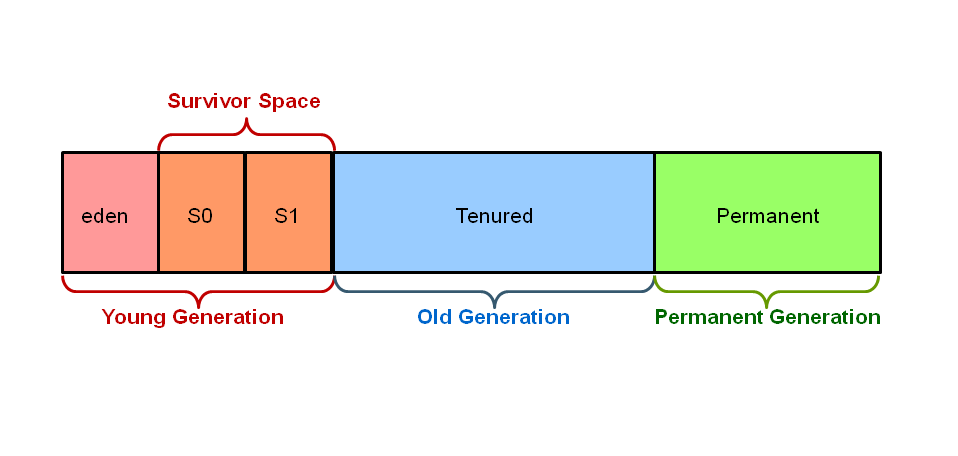
There are two main garbage collectors called the Minor Garbage Collector and the Major Garbage Collector. There are three main parts in the Heap:
有两个主要的垃圾收集器,分别称为次要垃圾收集器和主要垃圾收集器。 堆中包含三个主要部分:
- The Permanent Generation 永久的一代
- The Old (Tenured) Generation老一代
- The Young Generation年轻一代
The Young Generation consists of Eden Space, Survival Space 0, and Survival Space 1. New objects stay in the Young Generation and the old objects stay in the Old Generation. When programs create a new object, they put it into the Eden Space. When the Eden Space gets filled with objects, then the minor garbage collector comes and performs the three steps (mark, sweep, and compacting). After this, the surviving objects are put into the Survival Space 0. When the program next runs, the Eden space gets filled with the objects. Once again, the minor garbage collector comes and performs three steps across the Eden space and survival space 0. Then, surviving objects are put into the Survival Space 1. This process will continue to cycle as needed and every time the objects are marked with the number of times that they have survived the garbage collector.
年轻一代由伊甸园空间,生存空间0和生存空间1组成。新对象保留在年轻一代中,旧对象保留在老一代中。 程序创建新对象时,会将其放入Eden Space。 当Eden Space中充满对象时,次要垃圾收集器就会出现并执行三个步骤(标记,清除和压缩)。 此后,将幸存的对象放到生存空间0中。当程序下次运行时,伊甸园空间将被这些对象填充。 再次,次要垃圾收集器进入并在Eden空间和生存空间0上执行三个步骤。然后,将生存对象放入生存空间1。此过程将根据需要继续循环,并且每次用他们在垃圾收集器中存活下来的次数。
There is a value called the “threshold value”. When objects survive numbers of rounds that match the threshold value, they are promoted into the Old Generation. The minor garbage collector runs until the Old Generation is filled or ready to be filled up. Then the major garbage collector comes and performs the mark, sweep, and compacting steps all across the Heap; a time-consuming process called the “Stops the World ”. This is because when the major garbage collector runs, the whole application is stopped. This process can be seen visually from the VisualVM.
有一个值称为“阈值”。 当对象在与阈值匹配的轮数中幸存时,它们将被提升为旧一代。 次要垃圾收集器将一直运行到老一代已经装满或准备装满为止。 然后,主要的垃圾收集器来到并在整个Heap上执行标记,清除和压缩步骤; 一个耗时的过程称为“世界停止”。 这是因为当主要垃圾收集器运行时,整个应用程序将停止。 可以从VisualVM直观地看到此过程。
Occasionally, memory leaks can happen in the programs. When that happens, designers try to perform garbage collection manually to save the application from these leaks but this isn’t a good practice as when memory leaks happen, Heap dumps should be observed and analyzed in order to find the root of the problem.
有时,程序中可能发生内存泄漏。 发生这种情况时,设计人员将尝试手动执行垃圾回收以从这些泄漏中保存应用程序,但这不是一个好习惯,因为当发生内存泄漏时,应该观察并分析堆转储以找到问题的根源。
垃圾收集器 (Garbage Collectors)
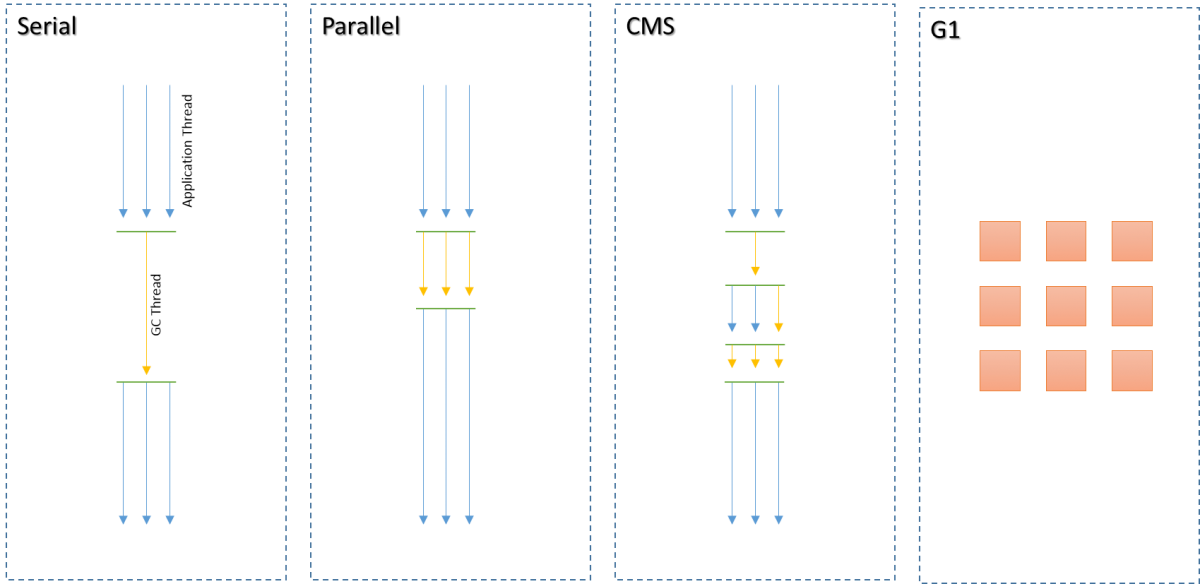
The following are types of garbage collectors:
以下是垃圾收集器的类型:
Serial Collector: A basic garbage collector that runs in a single thread and can be used for basic applications.
串行收集器:一个基本的垃圾收集器,它在单个线程中运行,并且可用于基本应用程序。
Concurrent Collector: A thread that performs garbage collection along with the application. “Stops the World” only happens during mark/re-mark (it does not wait for the Old Generation to be filled).
并发收集器:与应用程序一起执行垃圾收集的线程。 “停止世界”仅在标记/重新标记期间发生(它不等待老一代填充)。
Parallel Collector: Uses Multiple CPUs to perform, does not kick in until the Heap is near full. “Stops the world” when this runs.
并行收集器:使用多个CPU来执行,直到堆接近满时才启动。 运行时“停止世界”。
G1 Garbage Collector -This divides the Heap into different regions and during garbage collection it can collect a sub-set of regions. This has better Heap utilization.
G1垃圾收集器-将堆分为不同的区域,在垃圾收集过程中,它可以收集区域的子集。 这具有更好的堆利用率。
结论 (Conclusion)
Having a strong understanding of memory management is key to writing good programs with better memory utilization. This article has explored some advanced concepts of garbage collection in Java that I hope will assist you in completing your work more accurately.
对内存管理有深入的了解是编写具有更好内存利用率的良好程序的关键。 本文探讨了Java垃圾收集的一些高级概念,希望它们可以帮助您更准确地完成工作。
I’d like to thank you for reading my article, I hope to write more articles on these types of advanced concepts in the future to keep an eye on my account if you liked what you read today!
感谢您阅读我的文章,如果您喜欢今天阅读的内容,希望以后能写更多关于这些高级概念的文章,以关注我的情况!
References :
参考文献:
My previous articles on Java :
我以前关于Java的文章:
翻译自: https://medium.com/@PasinduUkwatta/advanced-concepts-of-java-garbage-collection-325c56c77829