In today’s blog post, I will build an algorithm that validates given inputs as anagrams. Here is one of the most classic interview challenge named Valid Anagram which I picked from LeetCode’s Top Interview Questions List:
在今天的博客文章中,我将构建一种算法来验证给定输入的字谜。 这是我从LeetCode的“热门面试问题列表”中选出的最经典的面试挑战之一,称为“有效Anagram” :
Given two strings s and t, write a function to determine if t is an anagram of s (You may assume the string contains only lowercase alphabets).
给定两个字符串s和t ,编写一个函数以确定t是否是s的字谜(您可以假设该字符串仅包含小写字母)。
Words that are anagrams are formed from another by rearranging its letters, so they will have the same letters with the same frequency in a different order. “listen” and “silent” can be given as an example to a common anagram. For this challenge, we need to determine if given strings are valid anagrams of each other. Let’s see a couple of examples to better understand the question:
anagram s的单词是通过重新排列字母而由另一个单词形成的,因此它们将以相同的频率以不同的顺序具有相同的字母。 “听”和“沉默”可以作为常见字谜的一个例子。 对于这个挑战,我们需要确定给定的字符串是否彼此有效。 让我们看几个例子来更好地理解这个问题:
Example 1:Input: s = "anagram", t = "nagaram"
Output: trueExample 2:Input: s = "rat", t = "car"
Output: false
To solve a problem, there are always multiple approaches and solutions with varying runtimes. Sorting the characters of both strings and then comparing them one by one can be the first thing that comes to our mind to solve this given problem, but remember that time takes to sort is larger than O(n). My approach will be to use a hashmap as our data structure and we will not need to use inbuilt methods of JavaScript in this way:
为了解决问题,总有多种方法和解决方案具有不同的运行时间。 对两个字符串的字符进行排序,然后一一比较它们可能是解决这个给定问题的第一件事,但是请记住,排序所需的时间大于O(n)。 我的方法是将哈希图用作我们的数据结构,并且我们不需要以这种方式使用JavaScript的内置方法:
Compare the lengths of the two given strings and return
false
if they are not equal.比较两个给定字符串的长度,如果它们不相等,则返回
false
。- Create an empty object to store all the characters as keys and their number of occurrences as values. 创建一个空对象以将所有字符存储为键,并将其出现次数存储为值。
- Iterate through the characters of the first string and add each character into the hash as a key by setting their value to 1 and then increment the value at the key by 1 on each occurrence. 遍历第一个字符串的字符,并将每个字符的值设置为1,将每个字符作为键添加到哈希中,然后在每次出现时将键的值增加1。
Loop over the second string and check if the character is in the object created above. If not, return
false
. If the character exists in the hash, decrement the value for that character by 1.循环遍历第二个字符串,并检查字符是否在上面创建的对象中。 如果不是,则返回
false
。 如果哈希中存在该字符,则将该字符的值减1。After both loops are done, return
true
, because this means that both the lengths and characters of the strings are equal.在完成两个循环之后,返回
true
,因为这意味着字符串的长度和字符都相等。
Let’s see a simple implementation of the above logic in JavaScript:
让我们看一下上述逻辑在JavaScript中的简单实现:
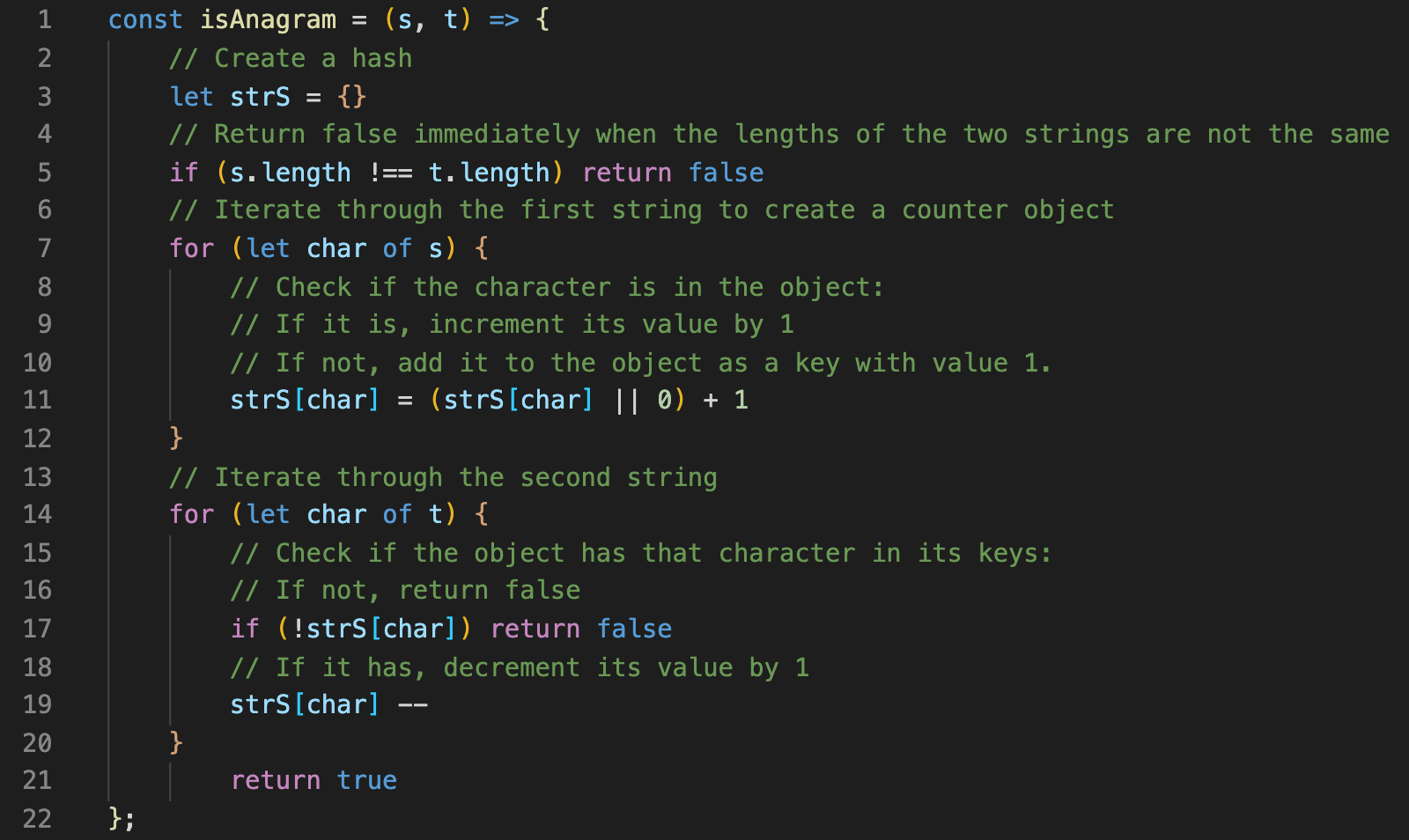
An anagram of a word can be created by rearranging the letters of the word using each letter only once. isAnagram
function above will compare two strings to see if they contain the same characters the same number of times. We first check if both strings are of the same length, if not; no comparison is needed. Then create a hashmap and iterate over one of the strings to determine the number of times each character appeared. This step stores the characters of the string as keys and their appearance as values. In the second iteration with for…of
loop, check if the characters of the second string already exist in the object. If it does, subtract one from its value. If it doesn’t, immediately return false
because the strings are not anagrams. Return true
if both loops complete, it means that the lengths and characters of the strings are analyzed and we can conclude that the strings are anagrams.
可以通过仅使用每个字母一次重新排列单词的字母来创建单词的字谜。 上面的isAnagram
函数将比较两个字符串,以查看它们是否包含相同字符相同的次数。 我们首先检查两个字符串的长度是否相同(如果不是); 无需比较。 然后创建一个哈希表并遍历字符串之一,以确定每个字符出现的次数。 此步骤将字符串的字符存储为键,并将其外观存储为值。 在for…of
循环的第二次迭代for…of
,检查第二个字符串的字符是否已存在于对象中。 如果是这样,请从其值中减去一个。 如果不是,则立即返回false
因为字符串不是字谜。 如果两个循环都完成,则返回true
,这意味着将分析字符串的长度和字符,我们可以得出结论,这些字符串是字谜。
Here’s what it looks like without comments:
这是没有注释的样子:
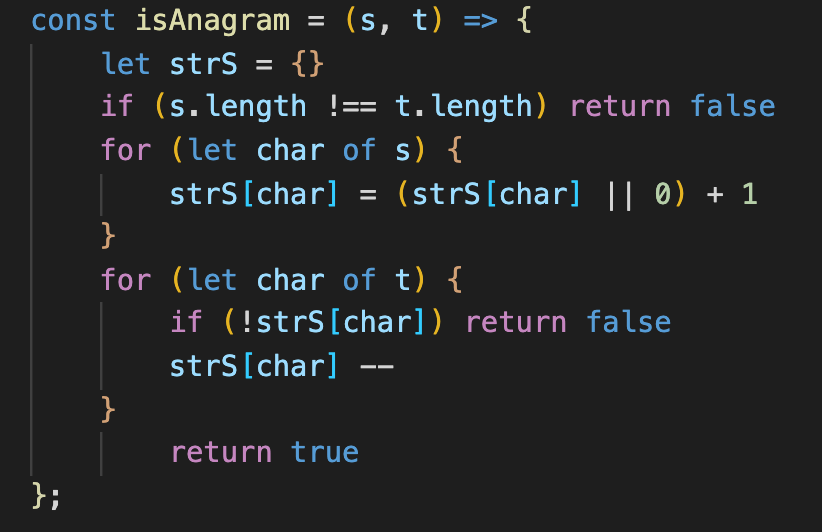
For our approach, we used two iterations (one per string) and provided a solution in linear time, so the time complexity is O(n), where n
is the length of the string. Note that we are looping over both strings only once.
对于我们的方法,我们使用了两次迭代(每个字符串一个),并提供了线性时间的解决方案,因此时间复杂度为O(n) ,其中n
是字符串的长度。 请注意,我们仅循环两个字符串一次。
翻译自: https://medium.com/javascript-in-plain-english/javascript-valid-anagram-challenge-70bc346fdf4c