grpc协议
gRPC is a burgeoning piece of technology that allows you to build web services where you can write code in your codebase’s native language; without writing your 50th custom HTTP Client to conform to some Swagger doc that you read on your company’s ill-maintained documentation hub. In this article, I will outline some of the basic concepts of gRPC and Protocol Buffers, some use cases for these technologies and finish with a simple demo of some cross-language gRPC services in action. I will also outline some common concerns at the end to consider. For official documentation, check out grpc.io.
gRPC是一项新兴的技术,它使您可以构建Web服务,从而可以使用代码库的本地语言编写代码。 而无需编写第50个自定义HTTP客户端以符合您在公司维护不良的文档中心上阅读的某些Swagger文档。 在本文中,我将概述gRPC和协议缓冲区的一些基本概念,这些技术的一些用例,并以运行中的一些跨语言gRPC服务的简单演示结尾。 最后,我还将概述一些常见问题,以供考虑。 有关官方文档,请查看grpc.io。
什么是协议缓冲区? (What are Protocol Buffers?)
Protocol buffers are a mechanism for serializing structured data. It is language-independent and uses an Interface Definition Language (IDL) for defining the shape of a given message and the properties within that message. Protobuf message definitions have values, but no methods; they are data-holders. Protobuf messages are defined in .proto
files.
协议缓冲区是一种用于序列化结构化数据的机制。 它与语言无关,并且使用接口定义语言(IDL)来定义给定消息的形状和该消息中的属性。 Protobuf消息定义有值,但没有方法; 他们是数据持有者。 Protobuf消息在.proto
文件中定义。
Protocol buffers has a compilation tool called protoc
that can compile your code in many supported languages. The current list of officially-supported as of the writing of this article includes:
协议缓冲区具有一个称为protoc
的编译工具,可以用许多受支持的语言编译您的代码。 截至本文撰写时,当前官方支持的列表包括:
- C / C++ C / C ++
- Objective C 目标C
- C# C#
- Dart 镖
- Go 走
- Java Java
- Kotlin (JVM) Kotlin(JVM)
- Javascript (Node) Javascript(节点)
- PHP PHP
- Python Python
- Ruby Ruby
- Javascript (Web) Javascript(网络)
There are other languages that have implementations but are not part of the “official” list yet, such as Swift and Haskell. You can find some of them on the grpc GitHub account.
还有其他具有实现功能但还不属于“官方”列表的语言,例如Swift和Haskell。 您可以在grpc GitHub帐户上找到其中的一些。
Protobufs use default values for all properties, so if you do not define an int
, for example, it will default to 0
. Strings will default to empty strings, booleans to false, etc.
Protobuf使用所有属性的默认值,因此,例如,如果您未定义int
,则它将默认为0
。 字符串默认为空字符串,布尔默认为false,等等。
You can define a protobuf message in a .proto
file like so:
您可以在.proto
文件中定义protobuf消息,如下所示:
message MovieItem {
//1 is the index used when serializing; do not reuse if the type of data changes!
string name = 1; double price = 2;
bool inStock = 3;
}
那么,什么是gRPC? (What is gRPC, then?)
gRPC is a group of technologies that allow you to communicate with other applications using Remote procedure calls (RPCs). Remote procedure calls are function calls that you make in your own codebase, but they execute on another machine, then return in your app. This abstracts the network from you, letting you call methods as if they were local code.
gRPC是一组技术,可让您使用远程过程调用(RPC)与其他应用程序进行通信。 远程过程调用是您在自己的代码库中进行的函数调用,但它们在另一台计算机上执行,然后返回您的应用程序。 这样可以从您那里抽象网络,让您像调用本地代码一样调用方法。
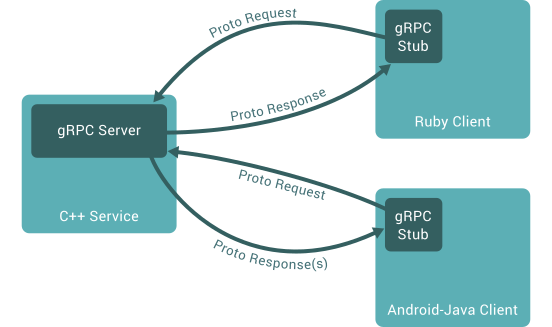
gRPC uses Protocol Buffers to serialize data between clients and servers, and has extra .proto
syntax for defining services used in your applications. With the help of a gRPC plugin for protoc
(or a plugin as part of your build script for your given platform), you can generate code that will give you the methods needed to call a given service, all with native typing in your language of choice (if applicable)!
gRPC使用协议缓冲区在客户端和服务器之间序列化数据,并具有额外的.proto
语法来定义应用程序中使用的服务。 借助用于protoc
的gRPC插件(或作为给定平台的构建脚本的一部分的插件),您可以生成代码,这些代码将为您提供调用给定服务所需的方法,所有代码均以本地语言输入选择(如果适用)!
You can define a gRPC service in your .proto
file like this (we will dissect this .proto
file later to explain how it works):
您可以像下面这样在.proto
文件中定义gRPC服务(我们将在稍后剖析.proto
文件以解释其工作原理):
syntax = "proto3";package moviecatalog;service MovieCatalog { rpc SaveNewMovie (MovieItem) returns (AddMovieResponse) {}
rpc FetchExistingMovie (FetchMovieRequest) returns (MovieItem) {}
}message MovieItem {
string name = 1;
double price = 2;
bool inStock = 3;
}message AddMovieResponse {
bool wasSaved = 1;
int32 itemId = 2;
}message FetchMovieRequest {
string name = 1;
}
gRPC supports multiple data transfer methods: Request + Response (like your typical REST service), Server-side streaming (out of scope for this article), Client-side streaming (out of scope for this article), Bidirectional streaming (out of scope for this article).
gRPC支持多种数据传输方法:请求+响应(如典型的REST服务),服务器端流传输(本文范围之外),客户端流传输(本文范围之外),双向流传输(范围之外)对于本文)。
Examples of these other transfer methods can be explored in a future post.
这些其他传输方法的示例可以在以后的文章中探讨。
为什么我应该使用gRPC而不是REST(HTTP + JSON)? (Why should I use gRPC instead of REST (HTTP+JSON)?)
When using Protocol Buffers for sending messages over the network, your payloads are serialized in binary, so they are much smaller than XML or JSON on the wire. This can save you bandwidth ($$$) and improve network performance.
当使用协议缓冲区通过网络发送消息时,您的有效负载将以二进制序列化,因此它们比在线上的XML或JSON小得多。 这样可以节省带宽($$$)并提高网络性能。
NOTE: There is a slight overhead for serializing and deserializing binary packets (and a couple of bytes in the headers), so if you have thousands of super tiny messages from multiple clients, you may not save as much in terms of milliseconds compared to REST. However, in most cases, especially long-lasting connections, gRPC will beat out REST most of the time. For benchmarks, check out this article.
注意:序列化和反序列化二进制数据包(标头中有几个字节)会产生一些开销,因此,如果您有成千上万来自多个客户端的超细微消息,那么与REST相比,您节省的时间可能不会太多。 但是,在大多数情况下,尤其是持久的连接,gRPC在大多数情况下会击败REST。 有关基准,请查看本文 。
Since gRPC uses code generators that give you native code for your given language, this saves a lot of time in overhead when writing custom serializers for JS