firebase登录验证
This article provides a short introduction to setting up and using the Firebase Authentication API in a React environment.
本文简要介绍了在React环境中设置和使用Firebase身份验证API。
The first step is to create a new firebase project, and under Project Overview, create a new web app. It will open a new page that allows you to register your app. You’ll end up with a config object that might look like this.
第一步是创建一个新的firebase项目 ,然后在“项目概述”下创建一个新的Web应用程序。 它将打开一个新页面,可让您注册您的应用。 您将得到一个看起来像这样的配置对象。
const firebaseConfig = {
apiKey: 'AIzaXXXXXXXXXXXXXXXXXXXXXXX',
authDomain: 'burger-builder-XXXX.firebaseapp.com',
databaseURL: 'https://burger-builder-XXXXXX.firebaseio.com',
projectId: 'burger-builder-XXXX',
storageBucket: 'burger-builder-XXXX.appspot.com',
messagingSenderId: 'XXXXXXX',
appId: "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"
};
As an alternative, you can also use environment variables in React applications, you only need to prefix them with REACT_APP_
for them to be available from within your code asprocess.env.REACT_APP_<variable name>
. All environment variables should be in a .env
, .env.local
or .env.production
in the root folder of your application.
或者,您也可以在React应用程序中使用环境变量,只需为它们加上REACT_APP_
前缀,它们就可以在您的代码中以process.env.REACT_APP_<variable name>
。 所有环境变量都应位于应用程序根文件夹中的.env
, .env.local
或.env.production
中。
在React中配置Firebase (To Configure Firebase in React)
Next, set up firebase in React by creating a firebase.js
file:
接下来,通过创建一个firebase.js
文件在React中设置firebase.js
:
Your .env
file may look like this:
您的.env
文件可能如下所示:
REACT_APP_API_KEY=XXXXxxxx
REACT_APP_AUTH_DOMAIN=xxxxXXXX.firebaseapp.com
REACT_APP_DATABASE_URL=https://xxxXXXX.firebaseio.com
REACT_APP_PROJECT_ID=xxxxXXXX
REACT_APP_STORAGE_BUCKET=xxxxXXXX.appspot.com
REACT_APP_MESSAGING_SENDER_ID=xxxxXXXX
We’ve set Firebase up in React, but we’re not using it anywhere. An easy way to do so is by creating a Firebase instance with the Firebase class, and then importing that instance in every React component where it’s needed. This is not the ideal way for 2 reasons:
我们已经在React中设置了Firebase,但没有在任何地方使用它。 一种简单的方法是通过使用Firebase类创建一个Firebase实例,然后将该实例导入需要的每个React组件中。 这不是理想的方法,原因有两个:
- It would make your application more error-prone. Firebase should be initialized as a singleton (once) in React applications, and by exposing it in every component that needs it, you might end up with multiple instances 这会使您的应用程序更容易出错。 Firebase应该在React应用程序中初始化为单例(一次),并且通过将它公开在需要它的每个组件中,您可能会遇到多个实例
- It makes it harder to test your components 它使测试组件变得更加困难
The more ideal way to create one Firebase instance in your application is with the help of React’s Context API:
在应用程序中创建一个Firebase实例的更理想的方法是借助React的Context API:
import React, { createContext } from "react";const FirebaseContext = createContext(null);export default FirebaseContext;
createContext()
provides two new components; FirebaseContext.Provider
provides a single Firebase instance at the top-level of your application component tree — index.js
. FirebaseContext.Consumer
is available to get the Firebase instance in any component when needed.
createContext()
提供了两个新组件; FirebaseContext.Provider
在应用程序组件树的顶层index.js
提供一个Firebase实例。 FirebaseContext.Consumer
可用于在需要时获取任何组件中的Firebase实例。
使用Firebase身份验证API进行身份验证 (Authentication with Firebase Authentication API)
On to the main point of this article; communicating with Firebase through the Firebase Authentication API and our Firebase instance. We’ll be looking at how to authenticate users with email/password, Google, Facebook, and GitHub.
继续本文的要点; 通过Firebase身份验证API和我们的Firebase实例与Firebase通信。 我们将研究如何使用电子邮件/密码,Google,Facebook和GitHub对用户进行身份验证。
使用电子邮件/密码进行身份验证 (Authenticating with Email/Password)
First, we need to activate the email/password authentication provider on the Firebase console. On your project’s Firebase dashboard, you can find a menu item that says “Authentication”. Select it and click the “Sign-In Method” menu item afterward. There you can enable the authentication with Email/Password:
首先,我们需要在Firebase控制台上激活电子邮件/密码身份验证提供程序。 在项目的Firebase仪表板上,您可以找到菜单项“身份验证”。 选择它,然后单击“登录方法”菜单项。 您可以在此处使用电子邮件/密码启用身份验证:
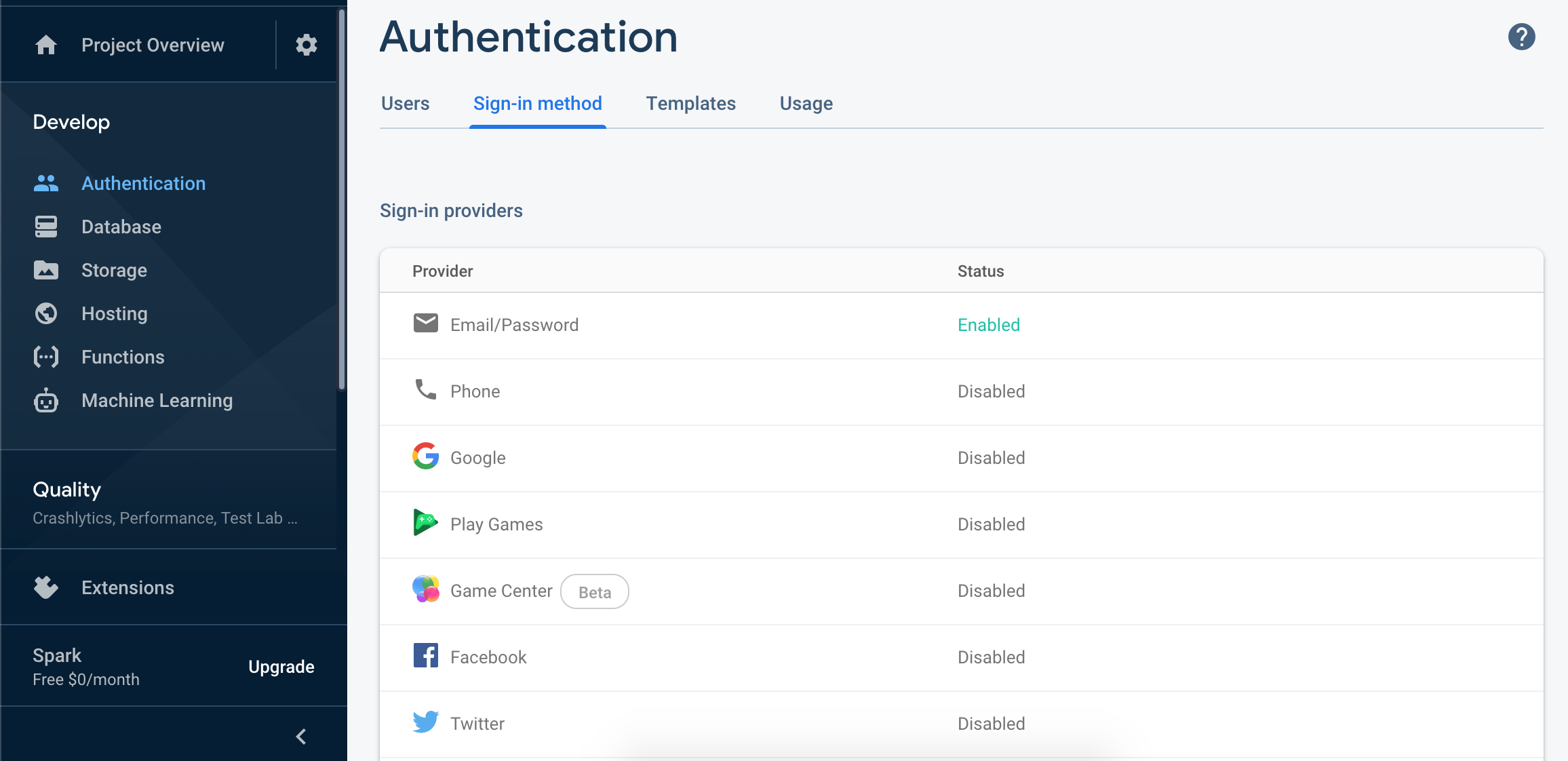
To implement the email/password auth provider in the application, we need to import and instantiate the package responsible for all authentication from Firebase in firebase/firebase.js
. Then call the functions to create, sign in and out users, and update and reset their password:
要在应用程序中实现email / password身份验证提供程序,我们需要在firebase/firebase.js
从Firebase导入并实例化负责所有身份验证的包。 然后调用这些函数来创建,登录和注销用户,以及更新和重置其密码:
...
import "firebase/auth";const firebaseConfig = {
...
};class Firebase {
constructor() {
...
this.auth = app.auth();
} createUserWithEmailAndPassword = (email, password) =>
this.auth.createUserWithEmailAndPassword(email, password); signInWithEmailAndPassword = (email, password) =>
this.auth.signInWithEmailAndPassword(email, password); signOut = () => this.auth.signOut();
passwordReset = (email) => this.auth.sendPasswordResetEmail(email); passwordUpdate = (password) => this.auth.currentUser.updatePassword(password);}export default Firebase;
When called, all functions are run asynchronously to be resolved later with either a success or a failure. For example, the Firebase API will return an error if you try signing in a user that does not already exist.
调用时,所有函数都异步运行,以以后解决成功或失败的情况。 例如,如果您尝试登录尚不存在的用户,则Firebase API将返回错误。
向Google进行身份验证 (Authenticate with Google)
In the same vein, in order to enable Google authentication in your Firebase React application, the Google sign-in method needs to be enabled in your Firebase console.
同样,为了在Firebase React应用程序中启用Google身份验证,需要在Firebase控制台中启用Google登录方法。
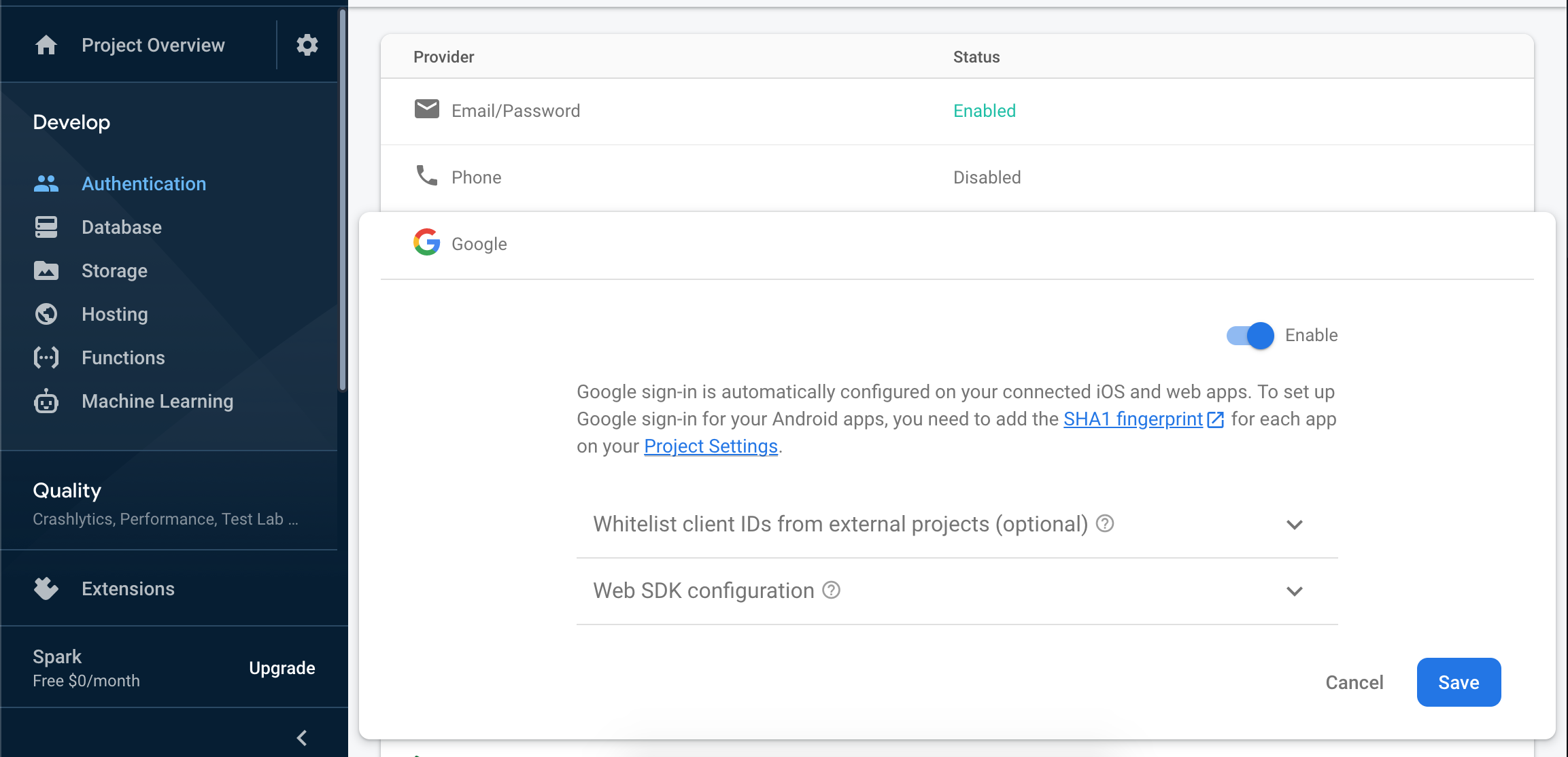
The Firebase Authentication API also provides functions that allow users to sign in or register with a provider. Depending on whether you’d like the form to appear as a popup or a new page, you can use signInWithPopup
or signInWithRedirect
. We first need to instantiate the provider — in our case, google — then call the function to authenticate with it. In the example below, I used the function to sign in with a popup. Note that it may make for a better user experience to sign in with a redirect in mobile devices.
Firebase身份验证API还提供了允许用户登录或注册到提供程序的功能。 根据您希望表单显示为弹出窗口还是新页面,可以使用signInWithPopup
或signInWithRedirect
。 我们首先需要实例化提供程序(在我们的例子中为google),然后调用该函数以对其进行身份验证。 在下面的示例中,我使用了该功能并通过弹出窗口登录。 请注意,通过重定向在移动设备中登录可能会带来更好的用户体验。
In trying to prevent duplicating code, I created a function the runs a switch on the passed provider then finally signs in with the passed provider. This way, I only call this.auth.signInWithPopup
once.
为了防止重复代码,我创建了一个函数,在传递的提供程序上运行一个开关,然后最终使用传递的提供程序进行登录。 这样,我只调用一次this.auth.signInWithPopup
。
Then in the component, attach a handler to the “Google” button and call this function, passing in “google” as the argument.
然后在组件中,将处理程序附加到“ Google”按钮并调用此函数,并传入“ google”作为参数。
通过Facebook进行身份验证 (Authenticate with Facebook)
Authenticating with Facebook is very similar to authenticating with Google, at least in the code. The main difference is that you need to set up a Facebook developer account and create an app there. You’ll receive an application ID and secret which you then provide Firebase to enable the connection.
至少在代码中,使用Facebook进行身份验证与使用Google进行身份验证非常相似。 主要区别在于您需要设置一个Facebook开发人员帐户并在其中创建一个应用程序。 您将收到一个应用程序ID和密码,然后提供Firebase来启用连接。
To create a Facebook developer account, sign in to your Facebook account here. Go to “My Apps”, and create an app. After creating your new app, you’ll be taken to the app dashboard. From there, your app ID and secret should be under Basic settings
要创建Facebook开发者帐户,请在此处登录您的Facebook帐户。 转到“我的应用程序”,然后创建一个应用程序。 创建新应用后,您将被带到应用仪表板。 从那里开始,您的应用ID和密码应位于基本设置下

Copy and paste your app ID and secret into your Firebase console after enabling the Facebook sign-in method, then also make sure to add the provided OAuth redirect URI to your Facebook app configuration.
启用Facebook登录方法后,将您的应用ID和密码复制并粘贴到Firebase控制台中,然后还要确保将提供的OAuth重定向URI添加到您的Facebook应用配置中。
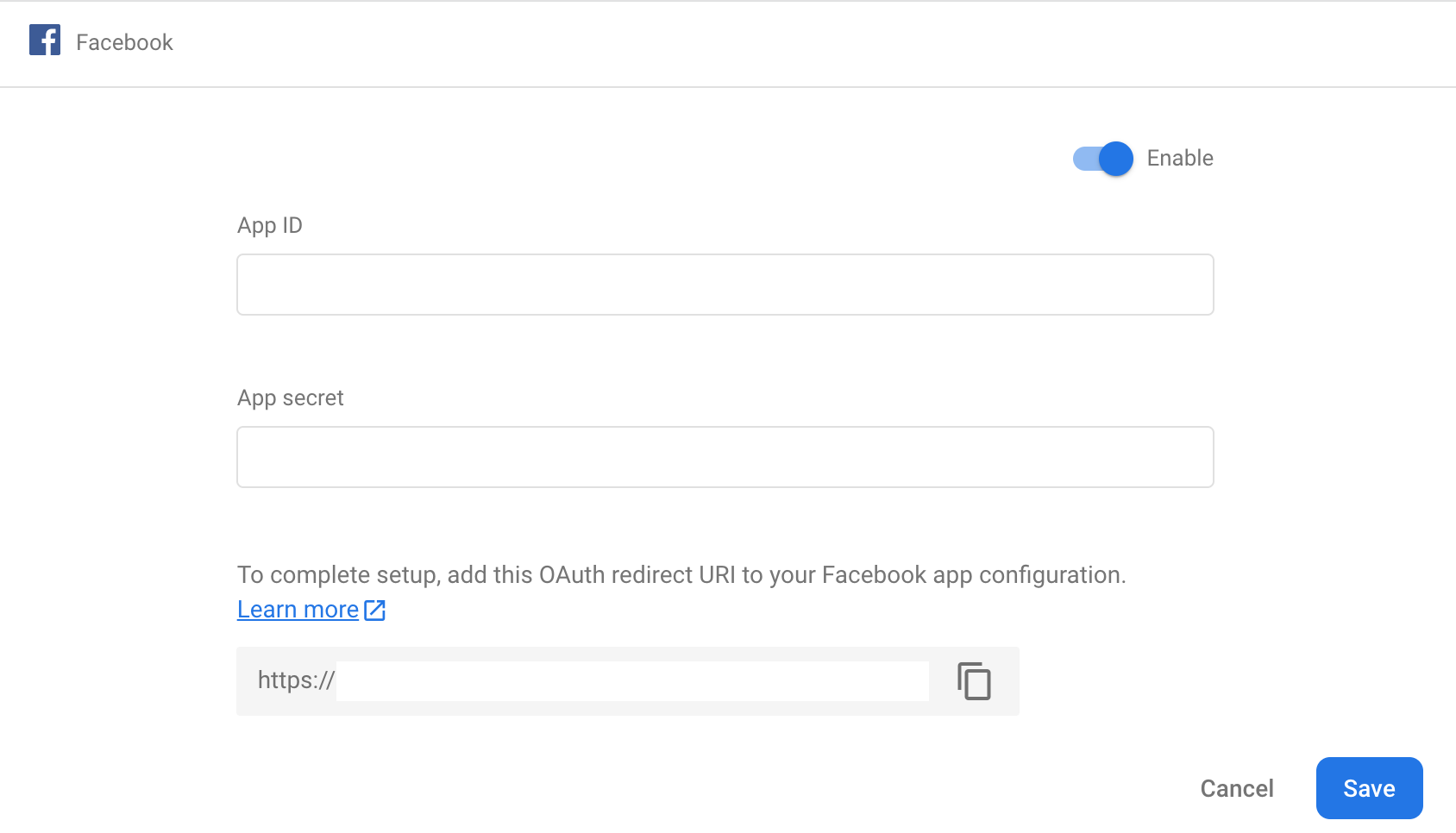
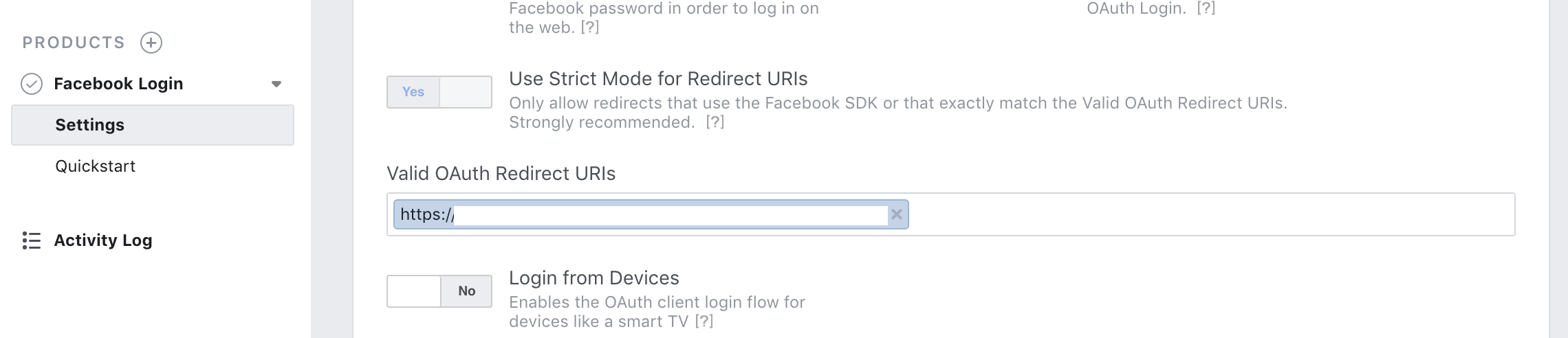
From here, it's the same as before; just instantiate your Facebook provider with new firebase.auth.FacebookAuthProvider()
and pass that into the sign-in function.
从这里开始,和以前一样。 只需使用new firebase.auth.FacebookAuthProvider()
实例化您的Facebook提供程序,然后将其传递到登录功能即可。
使用Github进行身份验证 (Authenticate with Github)
Similar to Facebook, we first need to register our application on Github as a developer application and get the app ID and secret, while providing the authorized callback URL provided by Firebase. Everything else is the same; instantiate the Github provider and pass that into the sign-in function:
与Facebook类似,我们首先需要在Github上将我们的应用程序注册为开发人员应用程序,并获取应用程序ID和密码,同时提供Firebase提供的授权回调URL。 其他一切都一样; 实例化Github提供程序,并将其传递给登录功能:
const githubProvider = new firebase.auth.GithubAuthProvider();return this.auth.signInWithPopup(githubProvider);
Don’t forget to attach the function to the Github sign-in button in your Login component.
不要忘记将该功能附加到您的Login组件中的Github登录按钮。
结论 (Conclusion)
Firebase is a simple, yet powerful tool that allows developers to focus on building applications and worry less about handling the infrastructure. Other than handling authentication, Firebase can also handle asset storage, database management, and hosting, but that’s just the surface. Explore more of Firebase can do here.
Firebase是一个简单但功能强大的工具,使开发人员可以专注于构建应用程序,而不必担心处理基础结构。 除了处理身份验证之外,Firebase还可以处理资产存储,数据库管理和托管,但这仅仅是表面。 探索更多Firebase在这里可以做的事情 。
One thing that’s worth mentioning again when introducing Firebase to your React applications is to make sure that Firebase is initialized as a singleton. This boosts the integrity of your app and makes it a little less error-prone.
将Firebase引入React应用程序时,值得再次提及的一件事是确保将Firebase初始化为单例。 这可以提高应用程序的完整性,并减少出错的可能性。
下一步 (Next Steps)
Now that your application handles user authentication, a good next step might be setting up protected routes in the system to allow users to see the information that is specific to them. In our application, for example, a protected route could be a profile page where users might see their past orders, and update their profile info (like their password).
既然您的应用程序已经处理了用户身份验证,那么下一步可能是在系统中设置受保护的路由,以允许用户查看特定于他们的信息。 例如,在我们的应用程序中,受保护的路线可以是个人资料页面,用户可以在其中查看其过去的订单,并更新其个人资料信息(例如密码)。
翻译自: https://medium.com/weekly-webtips/authentication-in-react-with-firebase-3a23fa631e2e
firebase登录验证