react 部署
How I used React and Firebase to create a low-dependency, light-weight configuration with user authentication and state
我如何使用React和Firebase创建具有用户身份验证和状态的低依赖性,轻量级配置
This is not intended to be a how-to but a small set of examples I think best exemplify how React and Firebase easily work together.
这并非旨在作为一个方法,而是一小部分示例,我认为最好地例证了React和Firebase如何轻松地协同工作。
React is the perfect tool for exploring new web software and cool web services like Firebase. React and Firebase, alone supply everything you need to build and deploy a lightweight application with state, a database, and user authentication. Here I will go into some examples of how to use Firebase for the backend and how to replace Redux with React Hooks.
React是探索新的Web软件和酷炫的Web服务(如Firebase)的理想工具。 React和Firebase单独提供了构建和部署具有状态,数据库和用户身份验证的轻量级应用程序所需的一切。 在这里,我将介绍一些如何在后端使用Firebase以及如何用React Hooks替换Redux的示例。
I recently put together a WhatsApp clone based on this video from Clever Programmer. This application is a great example of how to integrate React Hooks with Firebase to manage state and user authentication in a low-configuration and efficient design.
我最近根据来自Clever Programmer的视频整理了一个WhatsApp克隆 。 该应用程序是一个很好的示例,说明了如何在低配置和高效的设计中将React Hooks与Firebase集成在一起以管理状态和用户身份验证。
The configuration of this project is in stark contrast to past projects of mine in which I have built the backed myself. I do feel the need to remain in the practice of building REST APIs and backend logic and I do feel tremendous gratification after a successful compile and deploy of an app like that, however, if your intention is to explore the bleeding edge of front-end tech and get it online easily then Firebase is a great solution.
这个项目的配置与我过去建立自己支持的项目完全相反。 我确实感到有必要继续练习构建REST API和后端逻辑,并且在成功编译并部署了这样的应用程序后,我确实感到非常满足,但是,如果您的目的是探索前端的前沿技术并使其轻松上线,那么Firebase是一个很好的解决方案。
Let’s start with how I manage state without Redux. I start in “src/StateProver.js”
让我们从没有Redux的状态管理开始。 我从“ src / StateProver.js”开始
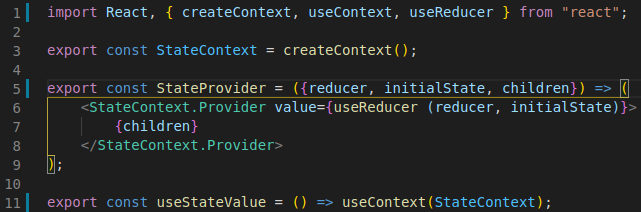
I use the React Context API. It creates a data layer to wrap the application and works very much like Redux without adding to your dependencies. Passing a variable for “children” (this will be our <App/> component), a reducer, and an initial state to the createContext() function, we create an “enhanced component” and assign it to a value of a function that returns a component. This is called a “higher-order component” and it is named <StateProvider/>, it is used similar to Redux <Provider/>. This is the data layer that we use throughout the application. On line 11 I create the container that allows me to pass actions to my reducer to update the state with useContext().
我使用React Context API。 它创建了一个数据层来包装应用程序,并且非常像Redux一样工作,而无需增加依赖项。 将变量“孩子”(这将是我们的<App />组件),化简器和初始状态传递给createContext()函数,我们创建“增强的组件”并将其分配给一个返回一个组件。 这称为“高阶组件”,名为<StateProvider />,其用法类似于Redux <Provider />。 这是我们在整个应用程序中使用的数据层。 在第11行上,我创建了一个容器,该容器允许我将操作传递给我的reducer以使用useContext()更新状态。
The reducer used for StateContext is just, as a reducer should be, a plain-old JavaScript function. You can see here that reducer.js below, exports an “initialState”, an “actionTypes” object, and the reducer function. This architecture functions the same as Redux but it just looks and feels cleaner if you ask me.
正如减少器应该那样,用于StateContext的减少器只是一个普通JavaScript函数。 您可以在此处看到以下reducer.js导出“ initialState”,“ actionTypes”对象和reducer函数。 这种架构的功能与Redux相同,但如果您问我,它的外观和感觉就更干净了。

火力基地 (Firebase)
Firebase is a platform developed by Google for creating mobile and web applications. It was originally an independent company founded in 2011. In 2014, Google acquired the platform and it is now their flagship offering for app development. Wikipedia
Firebase是Google开发的用于创建移动和Web应用程序的平台。 它最初是一家成立于2011年的独立公司。2014年,Google收购了该平台,现在它已成为其应用程序开发的旗舰产品。 维基百科
Getting started on Firebase is easy and the console is simple enough. Here I would like to focus on the code in my application that allows me to deploy and host the app. By importing the Firebase package you can initialize your application and database as well as create the tools you will need to accomplish the easiest user authentication method I have ever seen. Going back to my Login.js file and the signIn() function.
在Firebase上入门很容易,控制台也很简单。 在这里,我想重点介绍应用程序中的代码,该代码使我能够部署和托管该应用程序。 通过导入Firebase程序包,您可以初始化应用程序和数据库,以及创建完成我所见过的最简单的用户身份验证方法所需的工具。 回到我的Login.js文件和signIn()函数。

You can see that I have imported auth and provider from firebase.js. These variables are containers for functions of the Firebase package I initialized in firebase.js and imported. When the user clicks the “Sign in with Google” button, signIn() is called. I use another function of Firebase called signInWithPopup(). This takes a function “provider” as an argument. We create the provider as a container for GoogleAuthProvider() which gives the user a pop-up to select the account they want to use and returns a JSON object of that user's information.
您可以看到我已经从firebase.js导入了auth和provider。 这些变量是我在firebase.js中初始化并导入的Firebase软件包功能的容器。 当用户单击“使用Google登录”按钮时,将调用signIn()。 我使用Firebase的另一个函数signInWithPopup()。 这将函数“提供者”作为参数。 我们将提供程序创建为GoogleAuthProvider()的容器,该容器为用户提供一个弹出窗口以选择他们要使用的帐户,并返回该用户信息的JSON对象。
The really fun part of this application is the Firestore database. You can set up the database on firebase.com and then create a container for a function called firestore() that is the link between the app and the database.
该应用程序真正有趣的部分是Firestore数据库。 您可以在firebase.com上设置数据库,然后为一个名为firestore()的函数创建一个容器,该函数是应用程序与数据库之间的链接。
Here is an example of how easy it is to call your database and access collections within. Here you will also see that I have incorporated hooks to access specific entries in the database in the order I need to update the state of the component.
这是一个调用数据库并访问其中的集合有多么容易的示例。 在这里,您还将看到我已合并了一些挂钩,以按需要更新组件状态的顺序访问数据库中的特定条目。

I am getting roomId from a hook, useParams(). It allows me to access the params of the URL which contains the unique room id. As roomId is passed to the dependency array in useEffect(), it is run whenever roomId changes. What I wanted to point out was how I sort through the database. Remember that db is a container for our firestore() function. On line 31 I begin adding all the messages in a particular chat to the state of the component. I call db.collectio(“rooms”) which selects the “rooms” collection. I then select the room I want by passing in the roomId. I use the timestamp column of my database to organize the messages. The method onSnapShot() is a realtime listener that is run whenever the database updates. That’s right. This is a method that fires on your app every time the database updates. This allows for real-time two-way communication between your client and server.
我从一个挂钩中获取roomId,useParams()。 它允许我访问包含唯一房间ID的URL的参数。 由于将roomId传递给useEffect()中的依赖项数组,因此只要roomId发生更改,它就会运行。 我想指出的是如何对数据库进行排序。 请记住,db是我们的firestore()函数的容器。 在第31行,我开始将特定聊天中的所有消息添加到组件的状态。 我调用db.collectio(“ rooms”)来选择“ rooms”集合。 然后,我通过传入roomId选择想要的房间。 我使用数据库的时间戳列来组织消息。 onSnapShot()方法是一个实时侦听器,每当数据库更新时便运行该侦听器。 那就对了。 这种方法在每次数据库更新时都会在您的应用程序上触发。 这样可以在客户端和服务器之间进行实时双向通信。
In using React and Firebase you find many examples of code that looks like this. Simple to use and easy to integrate. These two tools are all I needed to build this great application.
在使用React和Firebase时,您会发现许多类似于以下代码的示例。 简单易用,易于集成。 这两个工具是构建此出色应用程序所需的全部。
react 部署