jest vue fn
Crucial pieces of functionality in cloud native applications is implemented in Functions. In the case of Oracle Cloud Infrastructure specifically, the Functions framework is typically Project Fn and the implementation language of choice is … up to the DevOps team. Popular languages for implementing Functions include Go, Python, Java and JavaScript.
在“功能”中实现了云本机应用程序中的关键功能。 具体来说,对于Oracle Cloud Infrastructure,Functions框架通常是Project Fn,而选择的实现语言则取决于……由DevOps团队决定。 用于实现功能的流行语言包括Go,Python,Java和JavaScript。
Automated code level tests are essential to describe and prove the behavior of the software and to allow rapid and safe refactoring of the code. These tests should be executable in a fully automated way, run against the code as it is without special provisions in the code for running the test, without requiring deployment of the code to a central environment and focus on the core: not the external dependencies used by the code at runtime and not the frameworks that wrap the code at runtime. In the case of Fn Functions, automated code level unit tests should not include the Fn libraries — but only deal with the code the developers in the DevOps team have written to implement the required functionality in the code.
自动化代码级测试对于描述和证明软件的行为以及允许快速安全地重构代码至关重要。 这些测试应以完全自动化的方式执行,可以针对代码运行,而无需在代码中进行特殊规定以运行测试,而无需将代码部署到中央环境并专注于核心:而不是使用外部依赖项取决于运行时的代码,而不是运行时包装代码的框架。 对于Fn函数,自动代码级单元测试不应包括Fn库-而是仅处理DevOps团队中开发人员编写的代码,以在代码中实现所需的功能。
This article describes what we can do with regard to these tests for Javascript based functions. It shows how we use Jest for writing the test and for running the test and for mocking the FDK (Fn framework). This last step means that we can test the code without executing any part of the Fn libraries.
本文介绍了针对基于Javascript函数的测试,我们可以做些什么。 它显示了我们如何使用Jest编写测试,运行测试以及模拟FDK(Fn框架)。 最后一步意味着我们可以在不执行Fn库任何部分的情况下测试代码。
The steps — that I will demonstrate in detail below are:
这些步骤-我将在下面详细说明:
- Prepare environment for Fn (install Fn Server and Fn Client) 为Fn准备环境(安装Fn Server和Fn Client)
- Install Jest (using NPM) 安装Jest(使用NPM)
- Create Function with JavaScript runtime 使用JavaScript运行时创建函数
- Configure Jest in function’s package.json 在函数的package.json中配置Jest
- Create a Mock FDK 创建一个模拟FDK
- Create a Test module func_test.js (require the function to test, require mock fdk, optionally: define setup steps to prepare for tests, define tests : description, call and expectation) 创建一个测试模块func_test.js(需要测试功能,需要模拟fdk,可选:定义准备测试的设置步骤,定义测试:描述,调用和期望)
- Run tests through npm to get a report fo the passed and failed tests(no interaction with the FDK libraries takes place when the tests are executed) 通过npm运行测试以获取已通过和失败测试的报告(执行测试时不会与FDK库进行交互)
在最简单的情况下进行演示:Hello World (Demonstration in simplest possible case: Hello World)
In an environment set up for Fn and Node development — with Fn Server and Client and with Node and NPM installed — I will demonstrate the installation and configuration of Jest and the creation of the hello function and its corresponding test.
在为Fn和Node开发设置的环境中(安装了Fn Server和Client并安装了Node和NPM),我将演示Jest的安装和配置以及hello函数的创建及其相应的测试。
First, create a function hello using the Node runtime:
首先,使用Node运行时创建一个函数hello :
fn init — runtime node hello
Change to the newly created directory hello and inspect the contents: package.json, func.yaml and func.js — the actual function. For our purpose of testing the function implementation (func.js) we do not really care about func.yaml and how to deploy and invoke the function. So let’s not bother with that.
转到新创建的目录hello并检查以下内容:package.json,func.yaml和func.js —实际功能。 为了测试功能实现(func.js),我们并不真正在乎func.yaml以及如何部署和调用功能。 因此,让我们不必理会。
Install the npm testing module jest (see [jest documentation] for details on how to get started). Jest has rapidly become the industry’s choice for testing JavaScript & Node applications.
安装npm测试模块jest (有关如何入门的详细信息,请参见[ jest文档 ])。 Jest已Swift成为测试JavaScript和Node应用程序的行业首选。
Execute this command to install jest as a development time dependency:
执行以下命令以将jest安装为开发时间依赖项:
npm install — save-dev jest
Add this snippet to `package.json` to have jest invoked whenever npm test is executed — creating a new property at the same level as main and dependencies:
将此代码段添加到package.json中,以便在执行npm test时调用笑话-在与main和dependencies相同的级别上创建一个新属性:
,"scripts": {"test": "jest"}
At this point, we can run Jest through npm test. However, there are no tests defined at this point, so this would not be very meaningful.
此时,我们可以通过npm test运行Jest。 但是,目前还没有定义测试,因此这没有什么意义。
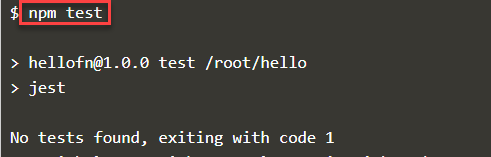
To test *func.js* as well — before the function is deployed to a container- we will use Jest — and in particular the mocking capabilities of Jest. The function — func.js — uses the Fn FDK framework — to handle HTTP requests that are handed to the function for procesing. However, in the test situation, we want to test outside the scope and context of the Fn framework and without any HTTP requests being made.
为了测试* func.js *(在将该功能部署到容器之前),我们将使用Jest-尤其是Jest的模拟功能。 函数func.js使用Fn FDK框架来处理传递给该函数进行处理的HTTP请求。 但是,在测试情况下,我们希望在Fn框架的范围和上下文之外进行测试,而不进行任何HTTP请求。
This can be achieved by using a mock for the fdk module. Jest allows us to define a mock in the following way :
这可以通过对fdk模块使用模拟来实现。 Jest使我们可以通过以下方式定义模拟:
- create file fdk.js in the folder __mocks__/@fnproject under the hello function 在hello函数下的__mocks __ / @ fnproject文件夹中创建文件fdk.js
implement the mock version of module @fnproject/fdk with a mock version of function handle
使用功能句柄的模拟版本实现模块@ fnproject / fdk的模拟版本
create file func.test.js that uses module @fnproject/fdk and runs tests against func.js
创建使用模块@ fnproject / fdk并针对func.js运行测试的文件func.test.js
The result is shown in the figure. Function hello consists of package.json, func.yaml and func.js and is extended with fdk.js in a mock directory. The code level unit test is defined in func.test.js — named after the the module under scrutiny — requires both fdk.js (and gets the mock implementation) and func.js. It defines the tests against func.js.
结果如图所示。 函数hello由package.json,func.yaml和func.js组成,并通过模拟目录中的fdk.js扩展。 代码级单元测试是在func.test.js中定义的-以受审查的模块命名-需要fdk.js(并获得模拟实现)和func.js。 它定义了针对func.js的测试。
When npm test is executed, Jest is ran and it recognizes by name that func.test.js is a unit test it should run, and so it will. This executes the test cases against func.js — without FDK involvement and without deploying the Function.
当执行npm test时 ,Jest被运行,并且通过名称识别到func.test.js是它应该运行的单元测试,因此也会运行。 这将针对func.js执行测试用例-无需FDK参与,也无需部署功能。
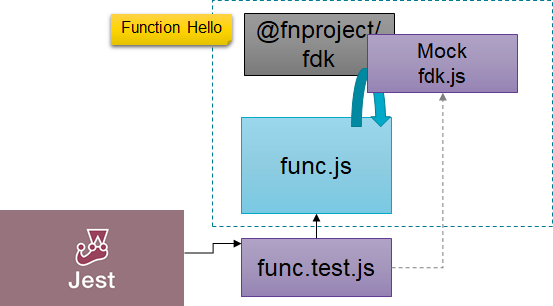
Create the new file for implementing the mock fdk.js:
创建用于实现模拟fdk.js的新文件:
mkdir /root/hello/__mocks__mkdir /root/hello/__mocks__/@fnprojecttouch /root/hello/__mocks__/@fnproject/fdk.js
Copy this code snippet to the file fdk.js:
将此代码段复制到文件fdk.js中:
const handle = function (f) {theFunction = freturn f}let theFunctionconst functionCache = function getFunction() {return theFunction}exports.handle = handleexports.functionCache = functionCache
When the func.js is required, it invokes function handle on the fdk module and passes a function as input parameter. This function is the actual Fn function implementation that handles the input and context objects to the Fn function. In the case of the mock fdk module, the handle function will simply retain the function reference in local variable theFunction.
当需要func.js,它调用FDK模块功能句柄并传递函数作为输入参数。 此函数是实际的Fn函数实现,用于处理Fn函数的输入和上下文对象。 如果是模拟fdk模块,则handle函数将仅将函数引用保留在局部变量theFunction中 。
The test function invokes functionCache to retrieve the handle to this function and subsequently invoke it — just as it would be in case of the normal invocation of the Fn function.
测试函数调用functionCache来检索该函数的句柄,并随后对其进行调用-就像在正常调用Fn函数的情况下一样。
Create the file func.test.js for the Jest tests for module func andImplement func.test.js as follows:
为模块func和实现func.test.js的Jest测试创建文件func.test.js ,如下所示:
// simply require func.js registers the function (input, context) with mock fdkconst func = require( './func.js' );const fdk=require('@fnproject/fdk');const name ="Bob"const input = {"name":name}const context = {"_headers":{"Host":"localhost","Content-Type":"application/json"}}const theFunction = fdk.functionCache() // get the function that was registered in func.js with the (mock) fdk handlertest(`Test of func.js for ${name}`, () => {expect(theFunction(input,context).message).toBe(`Hello ${name}`);});test(`Test of func.js for Host header in context object`, () => {expect(theFunction(input,context).ctx._headers.Host).toBe(`localhost`);});
The story for this test: the test loads the object to test — func.js — and the mock for the Fn FDK. The require of func.js causes the call in func.js to fdk.handle() to take place; this loads the reference to function object defined in func.js in the functionCache. The test gets the reference to the function in the local variable theFunction. Two tests are defined:
该测试的故事:该测试将加载要测试的对象func.js和Fn FDK的模拟。 func.js的需求导致func.js中对fdk.handle()的调用发生; 这会将引用加载到functionCache中func.js中定义的函数对象。 测试在局部变量theFunction中获取对该函数的引用。 定义了两个测试:
when the function is invoked with an object that contains a name property, does the response object contain a message property that has the specified value?
当使用包含名称属性的对象调用该函数时,响应对象是否包含具有指定值的消息属性?
when the function is invoked with a second object — context — does the response contain a ctx object
当使用第二个对象( 上下文 )调用该函数时,响应是否包含ctx对象
Run the test using
使用运行测试
npm test
This should report on the tests for function func, the real function implementation — but still without the runtime interference of Fn. One test passes, and one fails:
这应该报告功能func的测试,这是实际的功能实现-但仍然不会受到Fn的运行时干扰。 一项测试通过,但一项失败:
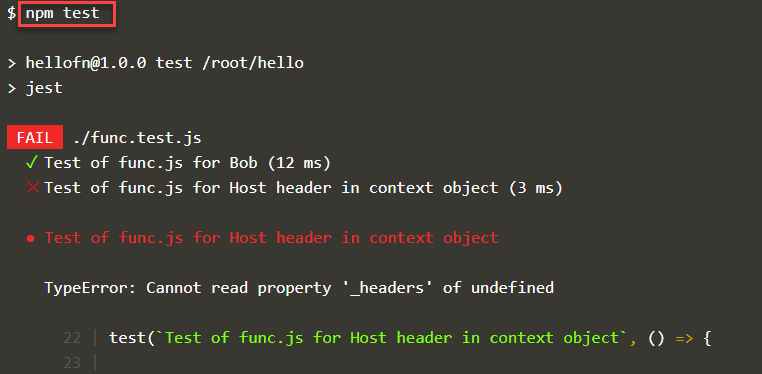
To make the failing test pass, a small change is required in the generated code in func.js:
为了使测试通过失败,在func.js中生成的代码中需要做一点改动:
const fdk=require('@fnproject/fdk');fdk.handle(function(input, context){ // adding context parameterlet name = 'World';if (input.name) {name = input.name;}console.log('\nInside Node Hello World function')return {'message': 'Hello ' + name, "ctx":context} // return the context object})
Re-Run the test using
使用以下命令重新运行测试
npm test
This should result in an even more positive report: both tests now pass.
这应该会产生更积极的报告:现在两项测试都通过了。
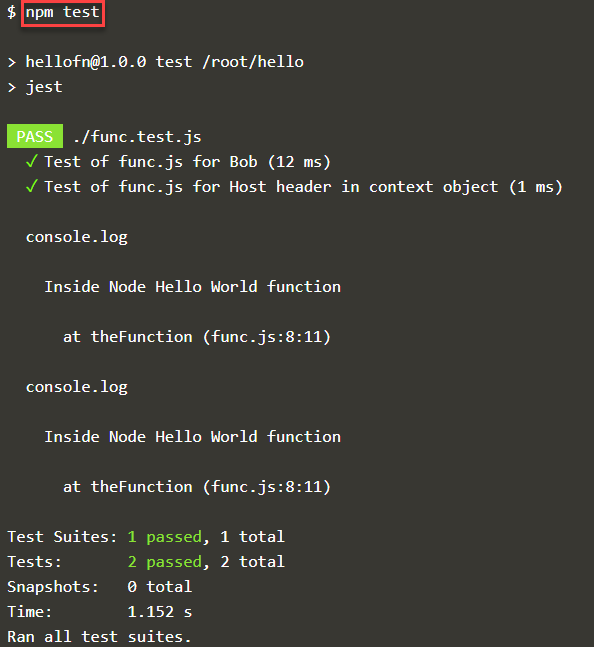
Handson教程 (Handson Tutorial)
If you want to work with Project Fn and specifically with Javascript functions and adding automated Jest based tests, you can try your hand at this Katacoda scenario in a cloud based tutorial environment: https://katacoda.com/redexpertalliance/courses/oci-course/introduction-fn
如果您想使用Project Fn,特别是使用Javascript函数并添加基于Jest的自动化测试,可以在基于云的教程环境中尝试以下Katacoda方案: https ://katacoda.com/redexpertalliance/courses/oci- 课程/介绍-fn
翻译自: https://medium.com/@lucasjellema/automated-unit-testing-of-node-fn-functions-using-jest-d34f3493cb62
jest vue fn