rxj热血江hsf湖私服
Let’s say you are developing an application where as part of your app you want to upload some files and you want to use existing component from UI framework. In this example I am using Angular and DevExtreme as UI framework.
假设您正在开发一个应用程序,在该应用程序中,您要上传一些文件,并且要使用UI框架中的现有组件。 在此示例中,我使用Angular和DevExtreme作为UI框架。
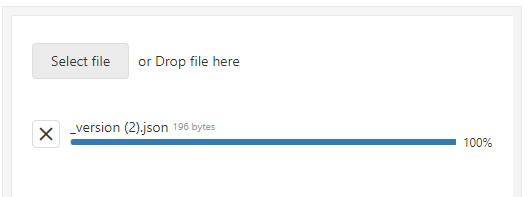
TL;DR: This guide serves as how-to since the official documentation is lacking details in this manner. This article was written retrospectively, so some info may be missing or incomplete.
TL; DR:由于官方文档缺少这种方式的详细信息,因此本指南可用作操作指南。 本文是回顾性的,因此某些信息可能会丢失或不完整。
先决条件 (Prerequisites)
To use FileUploader module from devextreme ui framework you have to setup project first. Here is how: https://js.devexpress.com/Documentation/Guide/Angular_Components/Getting_Started/Create_a_DevExtreme_Application/
要使用devextreme ui框架中的FileUploader模块 ,必须首先设置项目。 方法如下: https : //js.devexpress.com/Documentation/Guide/Angular_Components/Getting_Started/Create_a_DevExtreme_Application/
FileUploader的配置选项 (Configuration Options of FileUploader)
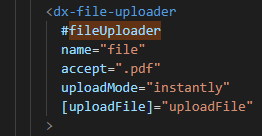
#templateSelector is needed so you can select Devextreme’s file uploader component using Angular’s @ViewChild
#templateSelector是必需的,因此您可以使用Angular的@ViewChild选择Devextreme的文件上传器组件
name self describing :)
名称自我描述:)
accept is set up if you want to filter file types in Explorer window
如果要在资源管理器窗口中过滤文件类型,则设置接受
uploadMode decides the behavior of component itself, so let’s set up instant (no need to press any button to upload)
uploadMode决定组件本身的行为,因此让我们进行即时设置(无需按任何按钮即可上传)
uploadUrl in bigger applications you want to use secure connection to API, right? So the default uploadUrl property wouldn’t be sufficient because it routes you to current domain, instead of some preferred API URL. Best way to do this, is to have custom service which will handle connection to API back-end and also handle security.
的uploadURL I N更大的应用程序,您要使用的API安全连接,对不对? 因此,默认的uploadUrl属性是不够的,因为它会将您路由到当前域,而不是一些首选的API URL。 最好的方法是拥有自定义服务 ,该服务将处理与API后端的连接并处理安全性。
[uploadFile] is a function that uploads a file. This is where our magic starts to happen. Here is how
[uploadFile]是上传文件的功能。 这就是我们的魔术开始发生的地方。 这是怎么
uploadFile(file, uploadInfoCallback) {
let formData = new FormData();
formData.append("file", file, file.name);
return this.dataService
.upload(this.URL_UPLOAD, formData, uploadInfoCallback)
.toPromise()
}
See? we just create new form and append the file to it. Then call our service upload observable and convert it toPromise(). We do this because frameworks core uses promises. Do not forget callback function, it is important to track you file upload progress.
看到? 我们只是创建新表单并将文件附加到其中。 然后将我们的服务上传称为可观察到的并将其转换为Promise()。 我们之所以这样做,是因为框架核心使用了承诺。 不要忘记回调函数,重要的是跟踪您的文件上传进度。
服务 (Service)
In service there is no need to setup special header parameters about form, I learned it the hard way. If you are using interceptor and want to use cookies, you have to setup withCredentials to true, here is the right place to do it.
在服务中,无需设置有关表单的特殊标头参数,我很难学到。 如果您正在使用拦截器并想使用Cookie,则必须将withCredentials设置为true,这是正确的位置。
/**
* Method for file uploader component
* @param url uri string
* @param data form data File - The file that is uploaded.
* @param uploadInfo progress callback - Function that you should call to notify the widget about the file upload progress.
*/
public upload(url: string, data: any, uploadInfoCallback: Function): Observable<any> {
return this.http
.post<any[]>(url, data, { withCredentials: true, reportProgress: true, observe: 'events' } // no need to setup any headers
)
.pipe(
map((event) => {
switch (event.type) {
case HttpEventType.UploadProgress:
uploadInfoCallback && uploadInfoCallback(Math.round(event.loaded));
return event;
default:
return event;
}
})
)
};
Here we are using POST method from common HttpClient to upload file, where we setup url, options. In options we can specify usage of credentials, additional headers, and most importantly progress reporting.
在这里,我们使用来自普通HttpClient的POST方法上传文件,并在其中设置url,options。 在选项中,我们可以指定凭据的使用,其他标题以及最重要的进度报告。
Since we are using observable, we can observe events emitted from there. This setup will pipe us events of progress type, so we can catch them and pass info into callback to show current progress.
由于我们正在使用observable ,因此可以观察从那里发出的事件。 此设置将通过管道传递进度类型的事件,因此我们可以捕获它们并将信息传递给回调以显示当前进度。
清除小部件 (Clear the widget)
Remember that #templateSelector we set up? So basically you want to reset the widget after uploading and closing window. When/where to do it? At beginning of course, which means on showing the widget. In this example widget is placed inside of popup with onShowing method.
还记得我们建立的是#templateSelector? 因此,基本上,您想在上载和关闭窗口后重置窗口小部件。 什么时候/在哪里做? 当然在开始时,这意味着要显示小部件。 在此示例中,小部件通过onShowing方法放置在弹出窗口的内部。
onShowing(e) {
if (this.fileUploader) {
// clear file uploader
this.fileUploader.instance.reset();
// optionally use this.fileUploader.instance.option("value", []);
}
}
沙盒 (Sandbox)
You can play around with this code example in sandbox here https://codesandbox.io/s/e71zj
您可以在此处的沙箱中试用此代码示例, 网址为https://codesandbox.io/s/e71zj
最后的话 (Final word)
This guide walks you thought implementing of DevExtreme’s FileUploader components using custom API service and callback function to show progress. Mix of observables and callbacks works well in this particular use case, but I guess it’s not best practice. Also keep in mind unsubscribing.
本指南使您想到使用自定义API服务和回调函数来实现DevExtreme的FileUploader组件的实现以显示进度。 在特定的用例中,可观察性和回调的混合效果很好,但是我认为这不是最佳实践。 还请记住不要订阅 。
下一个 (Next)
dxDataGrid custom storing
dxDataGrid自定义存储
rxj热血江hsf湖私服