I always had a myth in my mind where I used to think that if I am using classes in my code then I am using Object Oriented approach but at some point I found out that I was wrong.
我一直有个神话,我曾经想过,如果我在代码中使用类,那么我将使用面向对象的方法,但是在某个时候,我发现自己错了。
Recently, while I was reading book “Clean Code” by Robert C. Martin, I have gone through some examples which took me by surprise. There were some examples used for demonstrating the procedural coding style and OO coding style and this took me by surprise because both the examples were using classes and methods. So I read it and learned some very good insight. In this article, I would explain what I learned after all that time.
最近,当我阅读罗伯特·C·马丁(Robert C. Martin)的书《清洁代码》时 ,我经历了一些例子,这些使我感到惊讶。 有一些示例用于说明过程编码风格和OO编码风格,这让我感到惊讶,因为这两个示例都使用类和方法。 因此,我阅读了此书并学到了一些很好的见解。 在本文中,我将解释我在这段时间里学到的东西。
过程编程 (Procedural Programming-)
Wikipedia defines procedural programming as:
维基百科将过程编程定义为:
From this definition, it is asserted that procedural programming is really just the act of specifying a set of ordered steps needed to implement the requested functionality. It also implies about how those steps are implemented is a detail that’s not related to the paradigm. The important thing is that it’s imperative in how it works.
根据这个定义,可以断言过程编程实际上只是指定实现所需功能所需的一组有序步骤的动作。 这也暗示着这些步骤是如何实现的,是与范式无关的细节。 重要的是,它的工作方式至关重要。
Let’s look at a few examples:
让我们看几个例子:
The below code is procedural-
以下代码是程序性的-
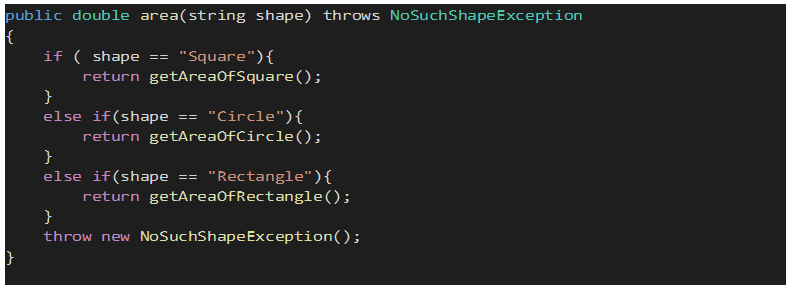
In a procedural programming language, a program basically consists of a sequence of instructions each of which tells the computer to do something such as we are doing it, and functions are written for the accomplishment of a specific tasks, here in this example we have created different methods for calculating areas of different geometries.
在过程编程语言中,程序基本上由一系列指令组成,每条指令告诉计算机执行我们正在执行的操作,并且编写函数来完成特定任务,在此示例中,我们创建了此程序用于计算不同几何形状的面积的不同方法。
Now the approach will remain procedural, even if it uses an object:
现在,即使使用对象,该方法也将保持过程性:
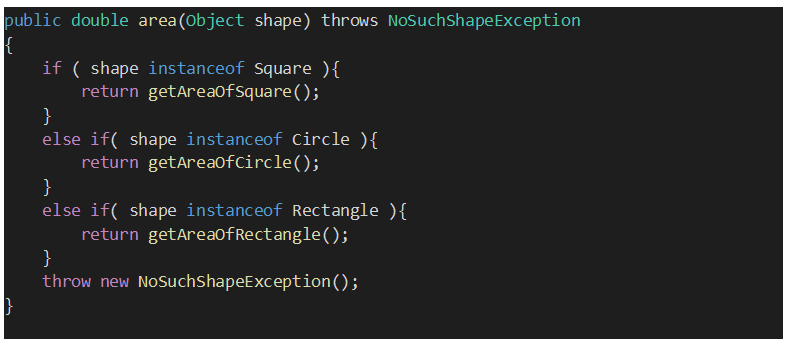
Now, also even though we are using object the paradigm we are following is same. We still have sequence of instructions, and functions for accomplishing different tasks.
现在,即使我们正在使用对象,我们遵循的范例也是相同的。 我们仍然有指令序列,以及完成不同任务的功能。
This is still procedural, even though it uses a class:
即使使用了一个类,它仍然是程序性的:
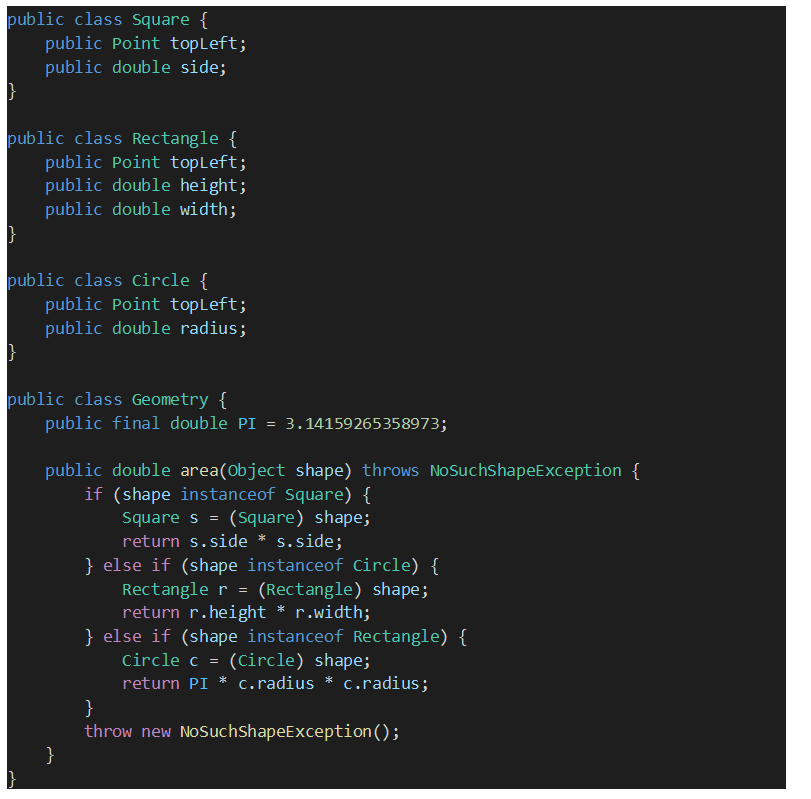
Now, though we have created the class for different geometry but still it is procedural. Procedural programming doesn’t have to do anything with whether we use classes or not, it’s a programming approach.
现在,尽管我们已经为不同的几何创建了该类,但是它仍然是过程性的。 无论我们是否使用类,过程编程都不需要做任何事情,它是一种编程方法。
The three codes mentioned above use the same programming approach. The only difference between them is the way the routines are resolved but each code is procedural. Each one has discrete steps that must be taken into consideration.
上面提到的三个代码使用相同的编程方法。 它们之间的唯一区别是例程的解析方式,但是每个代码都是过程性的。 每个步骤都有必须考虑的离散步骤。
Let’s take a look at how the OOP approach for this program will look like –
让我们看一下该程序的OOP方法如何-
面向对象编程 (Object Oriented Programming-)
According to Wikipedia, Object Oriented Programming is —
根据Wikipedia的说法,面向对象编程是-
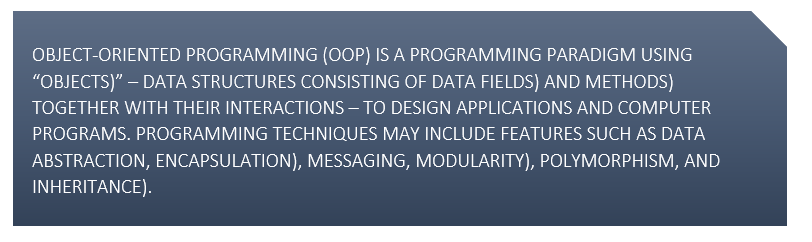
The concepts that we can take it out from here are –
我们可以从此处了解的概念是–
- It must abstract the data concepts into modular units. 它必须将数据概念抽象为模块化单元。
- It must have some polymorphic way to execute code. 它必须具有某种执行代码的多态方法。
- It must at least partially encapsulate that code and functionality. 它必须至少部分封装该代码和功能。
Now let’s implement these concepts on above example –
现在,让我们在上面的示例中实现这些概念–
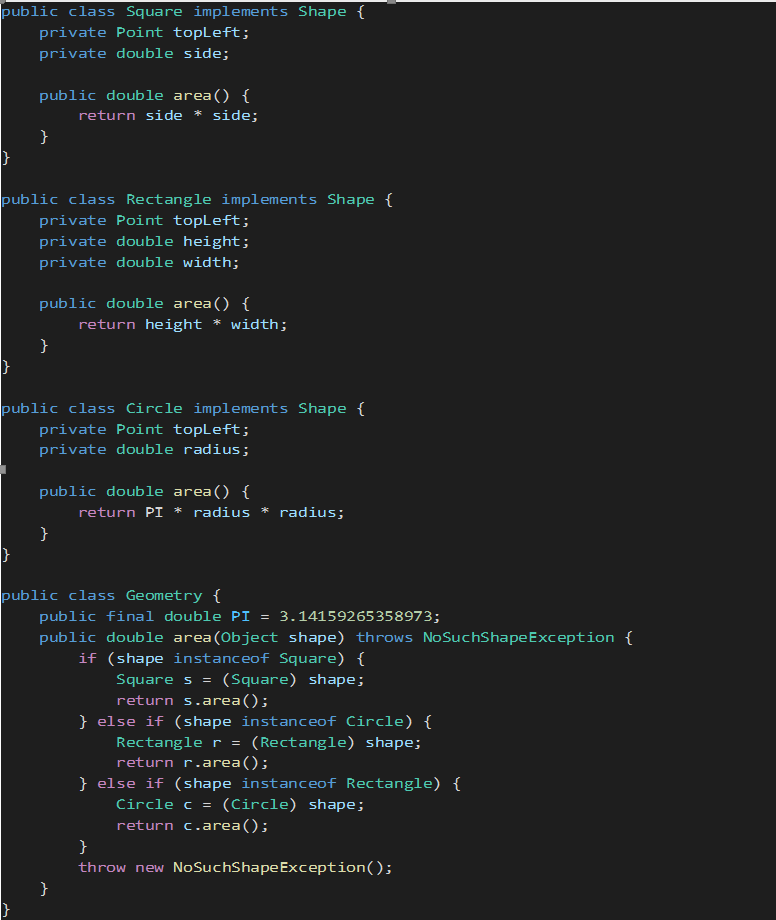
有什么不同? (What‘s the difference?)
Objects used in OOP hide the data behind abstractions and expose functions that operate on them.
OOP中使用的对象将数据隐藏在抽象背后,并公开对其进行操作的函数。
In the first procedural programming approach, classes Square, Rectangle and Circle are exposing their data attributes, “side”, “height and width”, and “center and radius” respectively. The class Geometry can directly work and access their data attributes. Indeed it would expose implementation even if the variables were private and we were using variable getters and setters.
在第一种过程编程方法中,Square,Rectangle和Circle类分别公开其数据属性“侧面”,“高度和宽度”以及“中心和半径”。 几何类可以直接工作并访问其数据属性。 的确,即使变量是私有的并且我们使用变量的getter和setter方法,它也会公开实现。
Hiding implementation is not just putting a layer of functions between the variables. Hiding implementations is about abstracting behavior. A class does not push its variables out through getters and setters. Instead, it exposes abstract interfaces that allows its users to manipulate the essence of data, without even knowing its implementation. As it is implemented in OOP approach example, each class has its own area functions. Now, no class other than the respective shape class knows about the implementation of calculating area. The classes are exposing “area ()” function that operate on their respective data attributes and provides the resulted area.
隐藏实现不仅是在变量之间添加功能层。 隐藏实现是关于抽象行为。 类不会通过getter和setter推送其变量。 相反,它公开了抽象接口,使用户可以操纵数据的本质,甚至不知道其实现。 在OOP方法示例中实现时,每个类都有其自己的区域功能。 现在,除了各个形状类别外,没有其他类别知道计算区域的实现。 这些类将公开对它们各自的数据属性进行操作并提供结果区域的“ area()”函数。
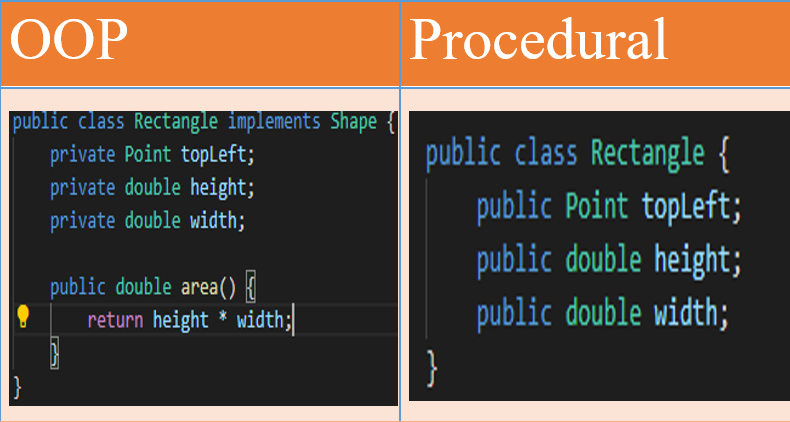
Now let’s go back to OOP example and revise the concepts that we have taken out from that definition of Wikipedia for OOP.
现在让我们回到OOP示例,并修改从Wikipedia for OOP定义中获得的概念。
The OOP approach example is abstracting the data concepts into modular units.
OOP方法示例将数据概念抽象为模块化单元。
- The OOP approach example is using the “area ()” method polymorphically. OOP方法示例多态使用“ area()”方法。
- And the data and the methods are encapsulated together. 并将数据和方法封装在一起。
So if someone is using object, it should not be assumed that he is writing OOP and because someone is using functions, they are using procedural programming, which is not true.
因此,如果某人正在使用对象,则不应假定他正在编写OOP,并且由于某人正在使用函数,因此他们正在使用过程编程,这是不正确的。
什么时候使用? (What to use when?)
ow suppose we want to calculate Parameters also, then the respective shape (Circle, Square, and Rectangle) will be unaffected and any other class that depends on them will also remain unaffected. But if I add a new class for shape like Triangle, then I must change all the functions in Geometry to deal with it. However, in case of OPP approach example none of the existing functions are effected. But if I add a new function all of the shape class will change.
假设我们也要计算参数,则相应的形状(圆形,正方形和矩形)将不受影响,并且依赖于它们的任何其他类别也将不受影响。 但是,如果我为Triangle这样的形状添加了一个新类,则必须更改Geometry中的所有函数以对其进行处理。 但是,在使用OPP方法示例的情况下,不会影响任何现有功能。 但是,如果添加新功能,则所有形状类都会更改。
There is a complimentary nature in these two approaches, as it is summarized in Clean Code book by Robert C. Martin -
罗伯特·C·马丁(Robert C. Martin)的“清洁代码”书中总结了这两种方法的互补性质-
Procedural code make it easy to add new functions without changing the existing data structures, OO Code, on the other hand, make it easy to add new classes without changing existing functions.
程序代码使添加新功能而不更改现有数据结构变得容易,而OO代码使添加新类而不更改现有功能变得容易。
So, we can conclude that when we want to add new data types rather than new functions, for these cases OO approach is appropriate. Whereas, when we want to add new functions as opposed to data types, procedural code is more appropriate.
因此,我们可以得出结论,当我们想添加新的数据类型而不是新的功能时,对于这些情况,OO方法是合适的。 而当我们想添加新功能而不是数据类型时,过程代码更合适。
Other aspects to consider include performance vs maintainability. If one intend on having a specialized program that won’t have change requirements and is designed for high performance, their efforts will be better served when building with PP concepts. A short script like programs that run against crontab, windows Powershell, or Launchctl shines here.
要考虑的其他方面包括性能与可维护性。 如果有人打算制定一个没有变更要求并且专为高性能而设计的专业程序,那么在使用PP概念进行构建时,他们的工作将得到更好的服务。 像在crontab,Windows Powershell或Launchctl上运行的程序这样的简短脚本在这里闪耀。
结论 (Conclusion)
Procedural vs OOP is an approach to writing code, not how one writes it. If someone is focusing on “Steps” and an ordered way of writing a program then he is more likely writing a procedural code. But if someone is focusing on state transformations and encapsulated abstractions, he is writing OOP.
程序与OOP是一种编写代码的方法,而不是一种编写方式。 如果某人专注于“步骤”和编写程序的有序方法,那么他更有可能编写程序代码。 但是,如果有人专注于状态转换和封装的抽象,那么他正在编写OOP。
The choice of usage from these approaches depends upon the application we want to write. It depends upon the aspects such as Procedural performance, maintainability, re-usability etc.
从这些方法中选择用法取决于我们要编写的应用程序。 它取决于诸如过程性能,可维护性,可重用性等方面。
Thanks for reading this article.
感谢您阅读本文。
If you have any additional explanations, or any comments please do not hesitate to add them below. I will be glad to know them.
如果您有其他说明或意见,请随时在下面添加。 我将很高兴认识他们。
翻译自: https://medium.com/@yashshavikashyap/procedural-vs-object-oriented-coding-style-a25b0a78f01b