场景:考虑一个文本编辑器,包含剪切菜单、粘帖按钮、文本编辑区域、剪贴板等对象,它们需要相互引用和交互。
结构
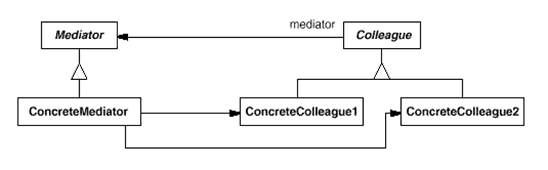
代码















































































































































































要点:
1、本模式将多个对象间复杂的关联关系解耦,对多个对象间的控制逻辑进行集中管理,变“多个对象互相关联”为“多个对象和一个中介者关联”,简化了系统维护,能更好地面对变化。
2、随着控制逻辑的复杂化,Mediator具体对象的实现可能相当复杂,这时候可对其进行分解处理。
3、Facade模式是解耦系统外到系统内(单向)的对象关联关系;本模式是解耦系统内各个对象之间(双向)的关联关系。