Best Time to Buy and Sell Stock Ⅰ
Say you have an array for which the ith element is the price of a given stock on day i.
If you were only permitted to complete at most one transaction (i.e., buy one and sell one share of the stock), design an algorithm to find the maximum profit.
Note that you cannot sell a stock before you buy one.
Example 1:
Input: [7,1,5,3,6,4] Output: 5 Explanation: Buy on day 2 (price = 1) and sell on day 5 (price = 6), profit = 6-1 = 5. Not 7-1 = 6, as selling price needs to be larger than buying price.
Example 2:
Input: [7,6,4,3,1] Output: 0 Explanation: In this case, no transaction is done, i.e. max profit = 0.
1 public int maxProfit(int[] prices) { 2 if (prices == null || prices.length == 0 ) return 0; 3 int[] dp = new int[prices.length]; 4 int minprice = prices[0]; 5 for ( int i = 1 ; i < prices.length ; i ++ ){ 6 dp[i] = Math.max(dp[i-1],prices[i]-minprice); 7 minprice = Math.min(minprice,prices[i]); 8 } 9 return dp[dp.length-1]; 10 }
运行时间1ms。
Best Time to Buy and Sell Stock II
Say you have an array for which the ith element is the price of a given stock on day i.
Design an algorithm to find the maximum profit. You may complete as many transactions as you like (i.e., buy one and sell one share of the stock multiple times).
Note: You may not engage in multiple transactions at the same time (i.e., you must sell the stock before you buy again).
Example 1:
Input: [7,1,5,3,6,4] Output: 7 Explanation: Buy on day 2 (price = 1) and sell on day 3 (price = 5), profit = 5-1 = 4. Then buy on day 4 (price = 3) and sell on day 5 (price = 6), profit = 6-3 = 3.
Example 2:
Input: [1,2,3,4,5] Output: 4 Explanation: Buy on day 1 (price = 1) and sell on day 5 (price = 5), profit = 5-1 = 4. Note that you cannot buy on day 1, buy on day 2 and sell them later, as you are engaging multiple transactions at the same time. You must sell before buying again.
Example 3:
Input: [7,6,4,3,1] Output: 0 Explanation: In this case, no transaction is done, i.e. max profit = 0.
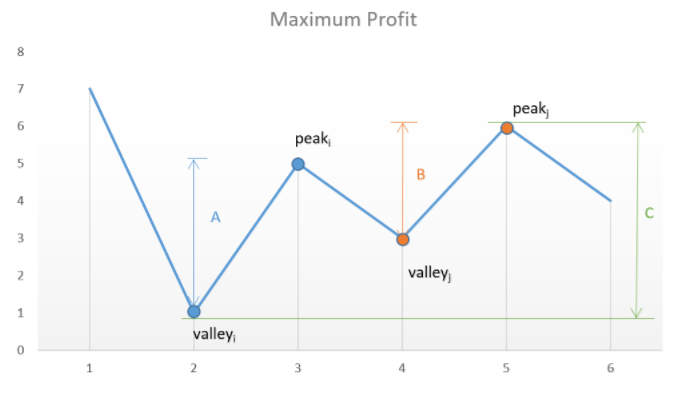
可以看到我们要求的最大收益,其实就是这个折线上升的那几段的差。在上图就是A,B。因此,我们的思路如下:
对数组进行一次遍历,如果price[i]>price[i-1],就对应着一个上升线段,所以我们令former=price[i-1],end=price[i];然后进入一段循环,找end的最大值。因为price[i+1]...可能会一直上升,也可能会下降,我们就需要找最高点。这个思路应该是比较容易理解,且很容易实现的。代码如下:
1 class Solution { 2 public int maxProfit(int[] prices) { 3 int maxprofit = 0; 4 for ( int i = 1 ; i < prices.length ; i ++ ){ 5 int former = prices[i-1]; 6 int end = prices[i]; 7 if ( end > former ){ 8 for ( int j = i ; j < prices.length ; j ++ ){ 9 if ( prices[j] >= end ) { 10 end = prices[j]; 11 } 12 if ( prices[j] < end || j == prices.length - 1 ){ 13 i = j; 14 break; 15 } 16 } 17 maxprofit += (end-former); 18 } 19 } 20 return maxprofit; 21 } 22 }
注意第12——15行,当发现最高点之后,需要将i的位置移动到新的点。
运行时间1ms,击败99.98%的人。我也没闹明白为啥效率这么高,感觉算法的时间复杂度是O(n^2)呀。。