compontent组件名称如何定义?
Vue.component('name', { /* ... */ })
第一个参数就是“name'”组件的名字。
如果我们想在HTML中写这样的的组件名称,<my-name></my-name>。
那么我们在对组件进行定义的名称的时候要这样写
Vue.component('yName', { /* ... */ })
即首字母大写。
组件分为全局注册和局部注册。
全局注册
Vue.component('MyName', { // ... 选项 ... })
全局注册简单的来说,在任何地方都可以使用这个组件
局部注册
var MyName={// ... 组件内容 ...}
局部注册后然后在components里面定义一下。
new Vue({
el:"#demo",
components:{
'my-name': MyName,
}
})
定义component组件
首先我们来定义简单的组件一个写法。
HTML
<div id="demo">
<btn-content></btn-content>
<btn-content></btn-content>
</div>
javascript
<script type="text/template" id="template">
<button>按钮点击</button>
</script>
<script>
Vue.component("btn-content", {
data: {},
template: '#template'
})
new Vue({
el: "#demo"
})
</script>
关于这个<script type="text/template" id="template">写法,可以参考前期写的这篇文章。soviya:vue-template三种写法介绍zhuanlan.zhihu.com
我们在输出页面上可以看到有两个点击按钮。接下来,我们想点击按钮执行点击弹出内容加一操作。
点击事件是用什么指令?用v-on,关于v-on操作可以参考这篇文章
soviya:VUE操作classstyle绑定属性绑定事件事件修饰符zhuanlan.zhihu.com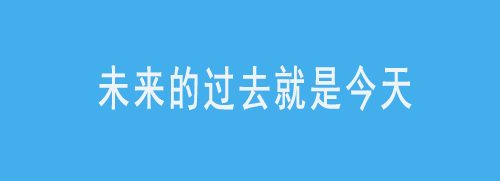
原HTML不变。
javascript
script type="text/template" id="template">
<button @click="num">按钮点击{{msg}}次</button>
</script>
<script>
Vue.component("btn-content", {
data: function() {
return {
msg: 0
}
},
methods: {
num: function() {
let msg = (this.msg) ++;
alert("您即将点击完成" + (msg + 1) + "次");
}
},
template: '#template'
})
new Vue({
el: "#demo"
})
</script>
这里需要注意的是,data必须是一个函数,那么data为什么是一个函数呢?假如组件中的data不是函数也可以执行的话,那么会和当前实例中的data起冲突。改变其一,另外一个也会随着改变。但是如果组件中data是一个函数的话,那么里面所定义的内容只在当前有效,不会污染到其它地方。
组件注册分为全局注册和局部注册,上面的实例给出的是全局注册。
通过 Prop 向子组件传递数据
HTML
<div id="demo">
<msg title="来自父组件的标题内容"></msg>
</div>
javarscript
<script type="text/template" id="template">
<h1>{{"子组件引用:"+title}}</h1>
</script>
<script>
Vue.component("msg", {
props: ['title'],
template: '#template'
})
new Vue({
el: "#demo"
})
</script>
通过v-for来循环相似的的模块
例
HTML
<div id="demo">
<msg v-for="post in posts" :title="post.title">
{{"字组件引用:"+post.title}}
</msg>
</div>
javascript
<script type="text/template" id="template">
<h1>{{title}}</h1>
</script>
<script>
Vue.component("msg", {
props: ['title'],
template: '#template'
})
new Vue({
el: "#demo",
data: {
posts: [{
id: 1,
title: '第一段内容'
},
{
id: 2,
title: '第二段内容'
},
{
id: 3,
title: '第三段内容'
}
]
}
})
</script>
现在我们想在msg这个组件里面再加h1内容。
<script type="text/template" id="template">
<h1>{{content}}</h1>
<h3>{{title}}</h3>
</script>
这是错误的写法,因为每个组件中都只能用一个根元素,所以要建立一个标签然后使期包裹起来。
按照上面得出,下面这个是正确的写法
<script type="text/template" id="template">
<div>
<h1>{{content}}</h1>
<h3>{{title}}</h3>
</div>
</script>
现在有一个需求,就是点击子组件里面的按钮,然后改变父级里面所有字体的大小。
HTML
<div id="demo">
<div :style="{fontSize:ftSize+'rem'}">
<msg @chage="ftSize+=0.1" v-for="post in posts" :title="post.id">
{{"字组件引用:"+post.title}}
</msg>
</div>
</div>
javascript
<script type="text/template" id="template">
<div>
<h3>{{content}}</h3>
<button @click="$emit('chage')">点击我改变父级全局文字大小</button>
</div>
</script>
<script>
Vue.component("msg", {
data: function() {
return {
content: "这是一段文字",
}
},
props: ['post'],
template: '#template'
})
new Vue({
el: "#demo",
data: {
ftSize: 1,
posts: [{
id: 1,
title: '第一段内容'
},
{
id: 2,
title: '第二段内容'
},
{
id: 3,
title: '第三段内容'
}
]
}
})
</script>
如果想让子改变父,那么首先在父定义一个想要改变的事件。然后在子使用$emit("父级定义的事件名称"。
当事件内容过多的时候,我们写在当前元素不好,不利于阅读。我们要写在methods方法里面。
HTML
<div id="demo">
<div :style="{fontSize:ftSize+'rem'}">
<msg @chage="onEnlargeText" v-for="post in posts" :title="post.id">
{{"字组件引用:"+post.title}}
</msg>
</div>
</div>
javascript
<script type="text/template" id="template">
<div>
<h3>{{content}}</h3>
<button @click="$emit('chage',0.1)">点击我改变父级全局文字大小</button>
</div>
</script>
<script>
Vue.component("msg", {
data: function() {
return {
content: "这是一段文字",
}
},
props: ['post'],
template: '#template'
})
new Vue({
el: "#demo",
data: {
ftSize: 1,
posts: [{
id: 1,
title: '第一段内容'
},
{
id: 2,
title: '第二段内容'
},
{
id: 3,
title: '第三段内容'
}
]
},
methods: {
onEnlargeText: function(num) {
this.ftSize += num
}
}
})
</script>
组件上使用v-model
HTML
<div id="demo">
<msg></msg>
</div>
javascript
<script type="text/template" id="template">
<div>
<input v-model="value" type="text" />
<h1>{{value}}</h1>
</div>
</script>
<script>
Vue.component("msg", {
data: function() {
return {
value: this.value
}
},
template: '#template',
methods: {
}
})
new Vue({
el: "#demo",
data: {},
methods: {
}
})
</script>