研究长字符串快速全文检索技术,实现某电力公司新闻中心新闻稿件全文检索统计系统。
1、 设计实现适合新闻稿件的基础类库
2、 新闻稿件全文检索功能实现
3、 新闻稿件按照关键字统计查询
代码如下
Paper.h
1 #pragma once 2 #ifndef PAPER_H 3 // we're here only if PAPER_H has not yet been defined 4 #define PAPER_H 5 6 // Definition of PAPER_H class and related functions goes here 7 #include <iostream> 8 #include <string> 9 #include <vector> 10 #define For(o,n) for (int i = 0; i < n; i++) 11 using namespace std; 12 class Paper { 13 protected: 14 int yy, mm, dd; 15 string unite; 16 string title; 17 //there declarations for kinds of news 18 public: 19 friend istream& operator >> (istream&, Paper&); 20 friend ostream& operator <<(ostream&, const Paper &); 21 Paper(); 22 Paper(const Paper &asp); 23 virtual ~Paper() {} 24 void SetYMDear(const int year, const int month, const int day); 25 int GetYear()const; 26 int GetMonth()const; 27 int GetDay()const; 28 string GetTitle()const; 29 string GetUnite()const; 30 void SetUnite(const string Unite); 31 void SetTitle(const string Title); 32 virtual int CountWords(const string text) = 0; 33 }; 34 class Word :virtual public Paper { 35 protected: 36 string contain; 37 public: 38 friend istream& operator >> (istream&, Word&); 39 friend ostream& operator <<(ostream&, const Word &); 40 Word(); 41 Word(const string con); 42 ~Word() { 43 44 } 45 int CountWords(const string text); 46 }; 47 class vedio :virtual public Paper { 48 protected: 49 string act; 50 string Vname; 51 public: 52 friend istream& operator >> (istream&, vedio&); 53 friend ostream& operator <<(ostream&, const vedio&); 54 vedio(); 55 ~vedio() {} 56 vedio(const string ac, const string vn); 57 string GetAct()const; 58 string GetVName()const; 59 int CountWords(const string text); 60 }; 61 class Record :virtual public vedio { 62 protected: 63 string ReName; 64 public: 65 friend istream& operator >> (istream&, Record&); 66 friend ostream& operator <<(ostream&, const Record &); 67 Record(); 68 ~Record() {} 69 Record(const string re); 70 int CountWords(const string text); 71 }; 72 class Poem :virtual public Word { 73 public: 74 Poem() {} 75 ~Poem() {} 76 int CountWords(const string text); 77 }; 78 class Other :virtual public Word { 79 public: 80 Other() {} 81 ~Other() {} 82 int CountWords(const string text); 83 }; 84 #endif
Realize.h
1 #include"Paper.h" 2 istream& operator >> (istream&in, Paper&rhp) { 3 in >> rhp.yy >> rhp.mm >> rhp.dd >> rhp.title >> rhp.unite; 4 in.ignore(); 5 //getline(in, rhp.contain); 6 return in; 7 } 8 ostream& operator <<(ostream&out, const Paper &rhp) { 9 out << rhp.yy << endl 10 << rhp.mm << endl 11 << rhp.dd << endl 12 << rhp.title << endl 13 << rhp.unite << endl; 14 // << rhp.contain << endl; 15 return out; 16 } 17 Paper::Paper() :yy(0), mm(0), dd(0), unite("xxx"), title("!!!") {} 18 Paper::Paper(const Paper &asp) { yy = asp.yy; mm = asp.mm; dd = asp.dd; unite = asp.unite; title = asp.title; } 19 void Paper::SetYMDear(const int year, const int month, const int day) { yy = year, mm = month, dd = day; } 20 void Paper::SetTitle(const string Title) { title = Title; } 21 void Paper::SetUnite(const string Unite) { unite = Unite; } 22 int Paper::GetDay()const { return dd; } 23 int Paper::GetYear()const { return yy; } 24 int Paper::GetMonth()const { return mm; } 25 string Paper::GetTitle()const { return title; } 26 string Paper::GetUnite()const { return unite; } 27 28 istream& operator >> (istream&in, Word&rhp) { 29 in >> rhp.yy >> rhp.mm >> rhp.dd >> rhp.title >> rhp.unite; 30 in.ignore(); 31 getline(in, rhp.contain); 32 return in; 33 } 34 ostream& operator <<(ostream&out, const Word &rhp) { 35 out << rhp.yy << endl 36 << rhp.mm << endl 37 << rhp.dd << endl 38 << rhp.title << endl 39 << rhp.unite << endl 40 << rhp.contain << endl; 41 return out; 42 } 43 Word::Word() :contain("xxx") { 44 45 } 46 Word::Word(const string con) { contain = con; } 47 int Word::CountWords(const string text) { 48 int ans = 0; 49 auto pos = contain.find(text); 50 while (pos != string::npos) { 51 ans++; 52 pos = contain.find(text, ++pos); 53 } 54 return ans; 55 } 56 istream& operator >> (istream&in, vedio&rhp) { 57 in >> rhp.yy >> rhp.mm >> rhp.dd >> rhp.title >> rhp.unite >> rhp.Vname; 58 in.ignore(); 59 getline(in, rhp.act); 60 return in; 61 } 62 ostream& operator <<(ostream&out, const vedio &rhp) { 63 out << rhp.yy << endl 64 << rhp.mm << endl 65 << rhp.dd << endl 66 << rhp.title << endl 67 << rhp.unite << endl 68 << rhp.act << endl 69 << rhp.Vname << endl; 70 return out; 71 } 72 vedio::vedio() :act("xxx"), Vname("XXXX") {} 73 vedio::vedio(const string ac, const string vn) { act = ac, Vname = vn; } 74 string vedio::GetAct()const { return act; } 75 string vedio::GetVName()const { return Vname; } 76 int vedio::CountWords(const string text) { 77 int ans = 0; 78 int pos = act.find(text); 79 while (pos != string::npos) { 80 ans++; 81 pos = act.find(text, ++pos); 82 } 83 return ans; 84 } 85 istream& operator >> (istream&in, Record&rhp) { 86 in >> rhp.yy >> rhp.mm >> rhp.dd >> rhp.title >> rhp.unite >> rhp.ReName; 87 in.ignore(); 88 getline(in, rhp.act); 89 return in; 90 } 91 ostream& operator <<(ostream&out, const Record &rhp) { 92 out << rhp.yy << endl 93 << rhp.mm << endl 94 << rhp.dd << endl 95 << rhp.title << endl 96 << rhp.unite << endl 97 << rhp.act << endl 98 << rhp.ReName << endl; 99 return out; 100 } 101 Record::Record() :ReName("XXXX") {} 102 Record::Record(const string re) { ReName = re; } 103 int Record::CountWords(const string text) { 104 return vedio::CountWords(text); 105 } 106 int Poem::CountWords(const string text) { 107 int ans = 0; 108 string flag = GetTitle(); 109 auto pos = flag.find(text); 110 while (pos != string::npos) { 111 ans++; 112 pos = flag.find(text, ++pos); 113 } 114 return ans; 115 } 116 int Other::CountWords(const string text) 117 { 118 int ans = 0; 119 string flag = GetTitle(); 120 auto pos = flag.find(text); 121 while (pos != string::npos) { 122 ans++; 123 pos = flag.find(text, ++pos); 124 } 125 return ans; 126 } 127 void ShowSTD() { 128 cout << "Please input kinds of News in follow fomat." << endl 129 << "Word or Poem or Other News:" << endl 130 << "Year Month Day Title Unite\nContain(Contain at the end of ENTER)" << endl 131 << "vedio or Record News:" << endl 132 << "Year Month Day Title Unite Filename\nAbstract(Abstract at the end of ENTER)" << endl; 133 }
test.cpp
1 #include"Realize.h" 2 int main() { 3 ShowSTD(); 4 Paper *tp[5]; 5 Word a1; 6 vedio a2; 7 Record a3; 8 Poem a4; 9 Other a5; 10 string flag; 11 int n = 5; 12 cout << "\nNow Inpt!!\nWord:"; 13 cin >> a1; 14 tp[0] = &a1; 15 cout << "vedio:"; 16 cin >> a2; 17 tp[1] = &a2; 18 cout << "Record:"; 19 cin >> a3; 20 tp[2] = &a3; 21 cout << "Poem:"; 22 cin >> a4; 23 tp[3] = &a4; 24 cout << "Other:"; 25 cin >> a5; 26 tp[4] = &a5; 27 cout << "Please enter the word you want to find:"; 28 cin >> flag; 29 For(o, n)cout << tp[i]->CountWords(flag) << endl; 30 cout << endl; 31 For(o, n)cout << *(tp[i]) << endl; 32 return 0; 33 }
测试截图如下:
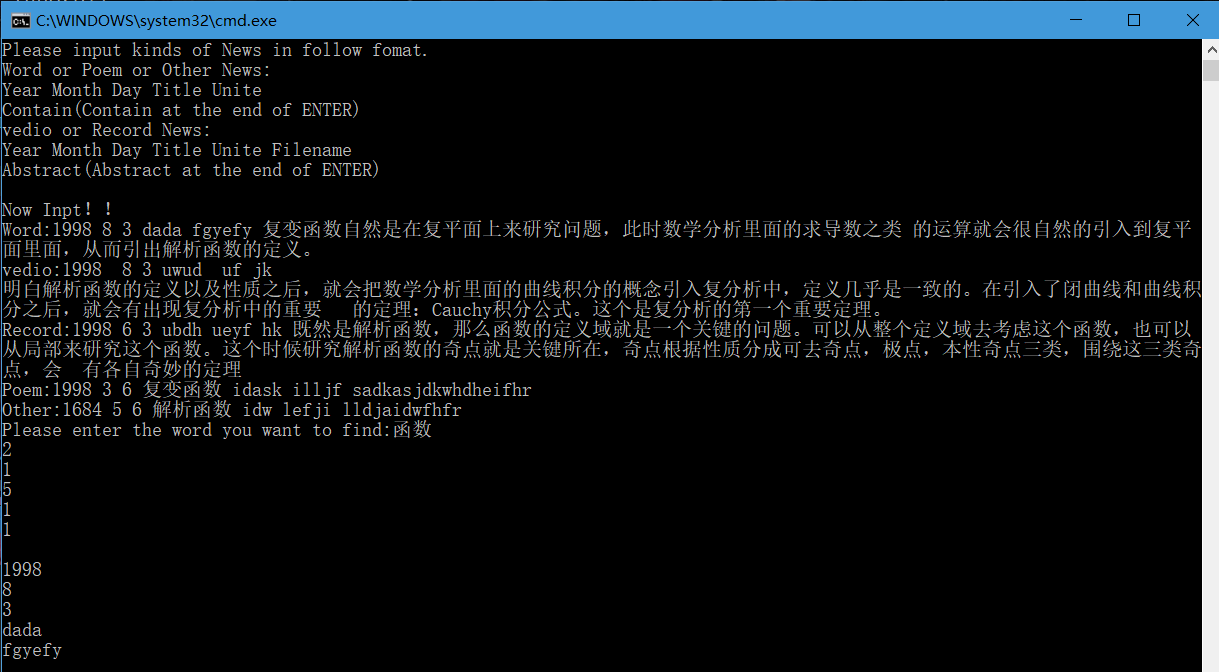