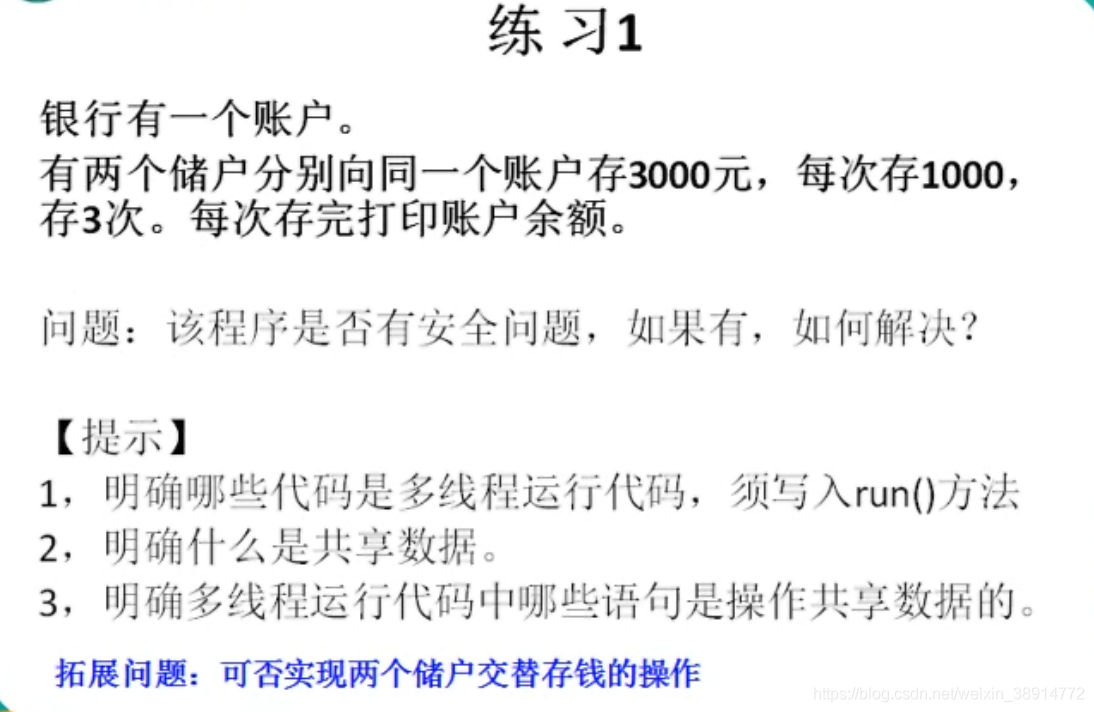
public class Account {
private double balance;
public Account(double balance) {
this.balance = balance;
}
public double getBalance() {
return this.balance;
}
public void setBalance(double balance) {
balance = balance;
}
public void cun(double money) {
synchronized(this) {
balance += money;
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + "存了:" + balance + "元");
}
}
}
public class Customer {
private Account acct;
public Account getAcct() {
return acct;
}
public void setAcct(Account acct) {
this.acct = acct;
}
public Customer(Account acct){
this.acct = acct;
}
}
public class MyThread1 extends Thread{
private Customer cus;
public MyThread1(Customer cus) {
this.cus = cus;
}
public void run() {
for(int i = 0;i < 3;i++) {
cus.getAcct().cun(1000);
}
}
}
public class Test1 {
public static void main(String[] args) {
Account acct = new Account(0);
Customer cu1 = new Customer(acct);
Customer cu2 = new Customer(acct);
MyThread1 mt1 = new MyThread1(cu1);
MyThread1 mt2 = new MyThread1(cu2);
mt1.setName("甲");
mt2.setName("乙");
mt1.start();
mt2.start();
}
}