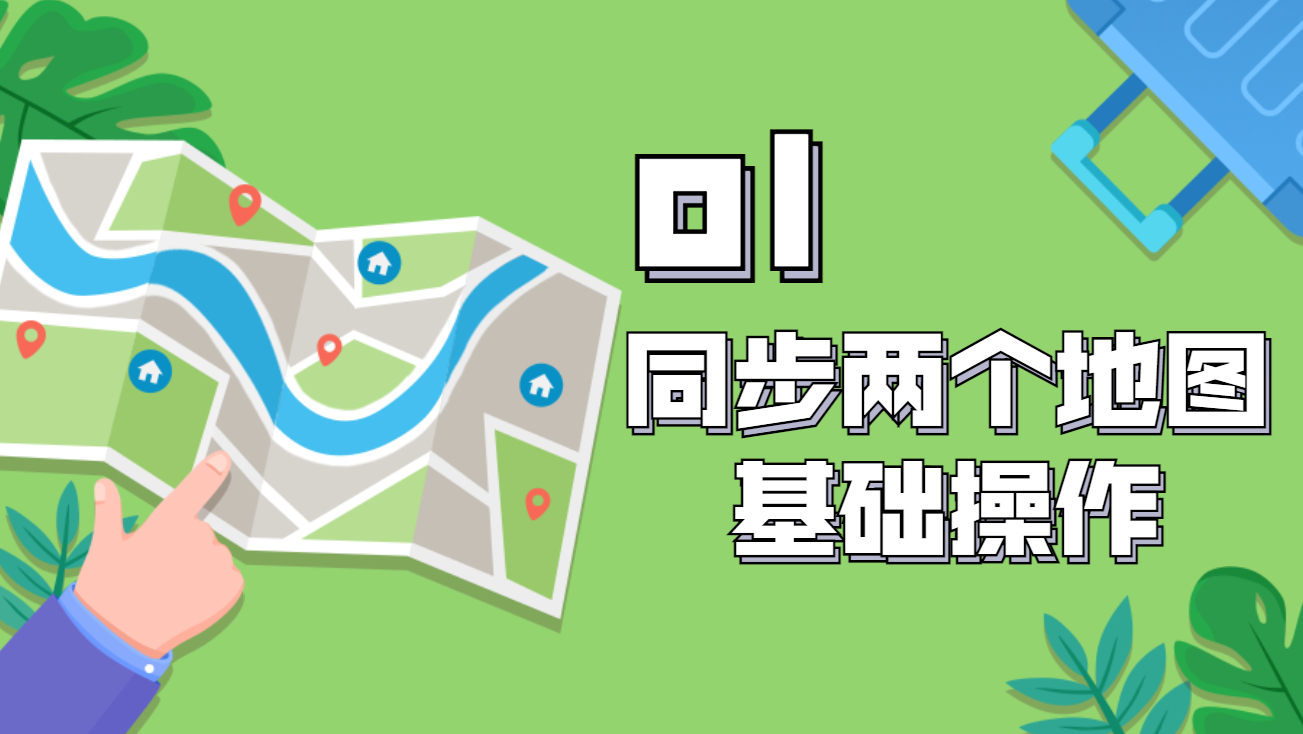
Vite + Vue3 + OpenLayers 同步两个地图基础操作
一、本文简介
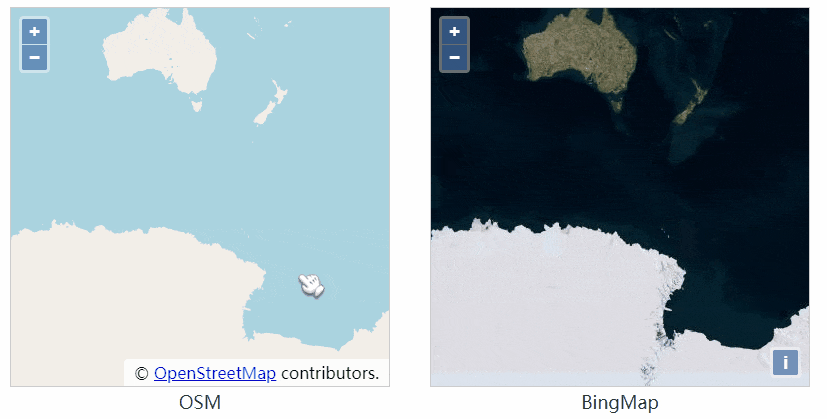
Vite + Vue3 + OpenLayers 同步两个地图基础操作
两个独立的容器,使用了不同的图源。但不管操作哪个容器的地图,另一个也会跟着变化。
二、开发环境
Vite + Vue3 + ol6
# 1、使用 Vite 创建项目;取个好听的项目名;拉取 vue 的代码模板
npm init vite@latest
# 2、初始化项目
cd you-project
npm install
# 3、安装 ol
npm i ol -S
# 4、启动项目
npm run dev
使用 Vite
初始化项目并安装 ol
,更详细做法可以查看 『Vite + Vue3 + OpenLayers 起步』
思路
- 两个地图容器,分别使用不同的图源
- 绑定同一个视图层
两个地图使用同一个view,所以在移动、缩放、旋转等操作都是同步的。
编码
<!-- ol - 同步两个地图 -->
<template>
<div class="map__container">
<!-- OSM地图容器 -->
<div id="OSM" class="map__x"></div>
<!-- bing地图容器 -->
<div id="BingMaps" class="map__x"></div>
</div>
</template>
<script setup>
import { ref, onMounted } from 'vue'
import { Map, View } from 'ol'
import Tile from 'ol/layer/Tile'
import OSM from 'ol/source/OSM'
import BingMaps from 'ol/source/BingMaps'
import 'ol/ol.css'
const mapO = ref(null) // 绑定OSM地图实例的变量
const mapB = ref(null) // 绑定Bing地图实例的变量
// 公共的视图
const mapView = new View({
center: [0, 0],
zoom: 2
})
// OSM图层
const layerO = new Tile({
source: new OSM()
})
// Bing图层
const layerB = new Tile({
source: new BingMaps({
key: 'AiZrfxUNMRpOOlCpcMkBPxMUSKOEzqGeJTcVKUrXBsUdQDXutUBFN3-GnMNSlso-',
imagerySet: 'Aerial'
})
})
// 初始化2个地图
function initMap () {
mapO.value = new Map({
target: 'OSM',
layers: [layerO], // 使用OSM图层
view: mapView // 使用了同一个视图层
})
mapB.value = new Map({
target: 'BingMaps',
layers: [layerB], // 使用Bing图层
view: mapView // 使用了同一个视图层
})
}
onMounted(() => {
// 在元素加载完之后再执行地图初始化
initMap()
})
</script>
<style lang="scss" scoped>
.map__container {
width: 800px;
height: 380px;
margin-bottom: 60px;
display: flex;
justify-content: space-between;
.map__x {
width: 380px;
height: 380px;
box-sizing: border-box;
border: 1px solid #ccc;
position: relative;
}
#OSM::after,
#BingMaps::after {
position: absolute;
display: block;
font-size: 18px;
left: 50%;
bottom: -28px;
transform: translateX(-50%);
}
#OSM::after {
content: 'OSM'
}
#BingMaps::after {
content: 'BingMap'
}
}
</style>
更多推荐
如果不清楚 OpenLayers
是什么,可以阅读: 『Vite + Vue3 + OpenLayers 起步』