本文用来学习python中一些高级特性,参考文章tutorial, 官网。
Class/Objects
下面是Python中创建class和object的代码示例。
类定义中第一行字符串用来解释类的功能,可使用ClassName.__doc__输出。
类变量empCount可以使用Employee.empCount访问。类似于Java中的static变量,可被类的所有object共享。
__init__为构造函数或初始化函数,用来创建类对象时使用。其他类的方法都存在参数self,表示类对象本身,调用时可不提供。
创建类对象不需要使用new。
#!/usr/bin/python3
class Employee:
'Common base class for all employees'
empCount = 0
def __init__(self, name, salary):
self.name = name
self.salary = salary
Employee.empCount += 1
def displayCount(self):
print("Total Employee %d" % Employee.empCount);
def displayEmployee(self):
print("Name : ", self.name, ", Salary: ", self.salary);
emp = Employee("Zara", 2000);
emp.displayEmployee();
emp.displayCount();
emp2 = Employee("Manni", 5000);
emp2.displayEmployee();
emp2.displayCount();
我们可以在任何时候添加、删除、修改类属性。
emp1.age = 7 # Add an 'age' attribute.
emp1.age = 8 # Modify 'age' attribute.
del emp1.age # Delete 'age' attribute.
hasattr(emp1, 'age') # Returns true if 'age' attribute exists
getattr(emp1, 'age') # Returns value of 'age' attribute
setattr(emp1, 'age', 8) # Set attribute 'age' at 8
delattr(empl, 'age') # Delete attribute 'age'
每个Python class都存在内置的类属性。
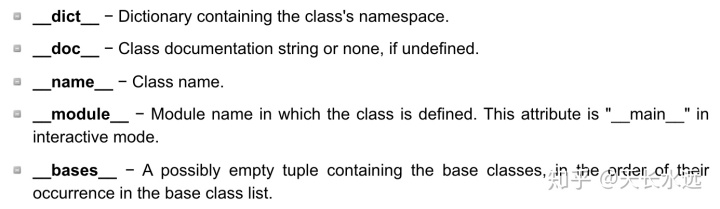
Python会自动清理不被需要的object,通过object reference count来决定。
a = 40 # Create object <40>
b = a # Increase ref. count of <40>
c = [b] # Increase ref. count of <40>
del a # Decrease ref. count of <40>
b = 100 # Decrease ref. count of <40>
c[0] = -1 # Decrease ref. count of <40>
也可以显示定义destructor __del__(), 该函数在object将被删除时被调用,用来清理object使用的非内存资源。
#!/usr/bin/python
class Point:
def __init__( self, x=0, y=0):
self.x = x
self.y = y
def __del__(self):
class_name = self.__class__.__name__
print class_name, "destroyed"
pt1 = Point()
pt2 = pt1
pt3 = pt1
print id(pt1), id(pt2), id(pt3) # prints the ids of the obejcts
del pt1
del pt2
del pt3
Python中支持类继承,下面是代码示例。通过将父类放在子类的括号内来表达继承关系,若继承多个类,只需添加多个类。子类中可以重写父类中的方法。
#!/usr/bin/python
class Parent: # define parent class
parentAttr = 100
def __init__(self):
print "Calling parent constructor"
def parentMethod(self):
print 'Calling parent method'
def setAttr(self, attr):
Parent.parentAttr = attr
def getAttr(self):
print "Parent attribute :", Parent.parentAttr
class Child(Parent): # define child class
def __init__(self):
print "Calling child constructor"
def childMethod(self):
print 'Calling child method'
使用issubclass(sub, sup)可以判断是否为父类子类关系,使用isinstance(obj, Class)可以判断是否为类和实例关系。
下面是一些基础的可以被重写的类函数。
__init__ ( self [,args...] )
# Constructor (with any optional arguments)
# Sample Call : obj = className(args)
__del__( self )
# Destructor, deletes an object
# Sample Call : del obj
__repr__( self )
# Evaluable string representation
# Sample Call : repr(obj)
__str__( self )
# Printable string representation
# Sample Call : str(obj)
__cmp__ ( self, x )
# Object comparison
# Sample Call : cmp(obj, x)