至于 GraphQL 的简介,大家可以自行搜索, 这里不再赘述。
我们直接开始!
本文使用的开发工具:
IntelliJ IDEA Ultimate
- 新建 SpringBoot 项目: 点击 New -> Project -> Spring Initializer
- 选择依赖 Spring Web Starter
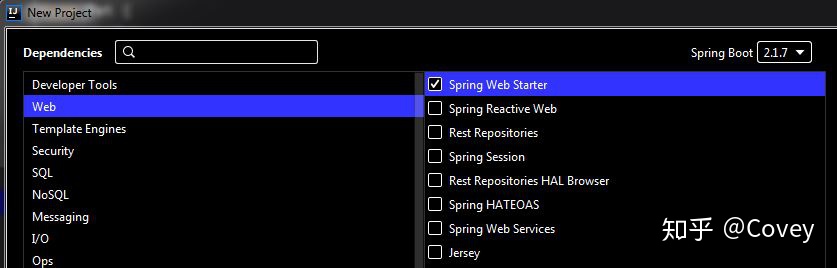
3. 打开pom.xml 文件 添加如下 Maven 依赖
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.graphql-java</groupId>
<artifactId>graphql-java-tools</artifactId>
<version>5.2.4</version>
</dependency>
<dependency>
<groupId>com.graphql-java</groupId>
<artifactId>graphql-spring-boot-starter</artifactId>
<version>5.0.2</version>
</dependency>
<dependency>
<groupId>com.graphql-java</groupId>
<artifactId>graphiql-spring-boot-starter</artifactId>
<version>5.0.2</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
4. 直接启动项目, 在浏览器输入 http://localhost:8080/graphiql
如出现以下画面,则说明启动成功,此网页工具可用来测试 GraphQL 语句
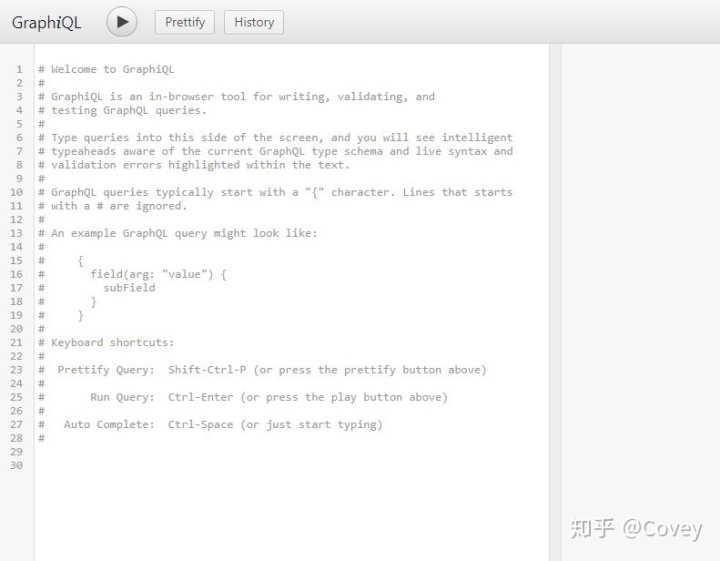
但现在我们还不能做任何事,因为项目还是空的
5. 接下来,在 resources 文件夹下新建一个 petshop.graphqls 文件

6. 在 petshop.graphqls 文件中添加如下内容,用于声明 API 可接受与返回的参数
type Query {
pets: [Pet]
animals: [Animal]
}
type Pet {
id: Int
type: Animal
name: String
age: Int
}
enum Animal {
DOG
CAT
BADGER
MAMMOTH
OOOOOO
}
6. 创建一个 entities 包,在包中新建一个 Pet 类
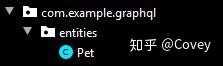
Pet 类内容如下:
package com.example.graphql.entities;
import com.example.graphql.enums.Animal;
/**
* @author Covey Liu, covey@liukedun.com
* Date: 8/27/2019 2:15 PM
*/
public class Pet {
private long id;
private String name;
private Animal type;
private int age;
public Pet(long id, String name, Animal type, int age) {
this.id = id;
this.name = name;
this.type = type;
this.age = age;
}
public Pet() {
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Animal getType() {
return type;
}
public void setType(Animal type) {
this.type = type;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
7. 创建eums包,在包中新建Animal类
Animal类内容如下
package com.example.graphql.enums;
/**
* @author Covey Liu, covey@liukedun.com
* Date: 8/27/2019 2:09 PM
*/
public enum Animal {
/**
* Animal
*/
DOG,
CAT,
BADGER,
MAMMOTH,
OOOOOO
}
8. 创建 resolvers包,在包中新建 Query类, 用于返回请求数据
Query类内容如下:
package com.example.graphql.resolvers;
import com.coxautodev.graphql.tools.GraphQLQueryResolver;
import com.example.graphql.entities.Pet;
import com.example.graphql.enums.Animal;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.List;
/**
* @author Covey Liu, covey@liukedun.com
* Date: 8/27/2019 2:18 PM
*/
@Component
public class Query implements GraphQLQueryResolver {
public List<Pet> pets() {
List<Pet> pets = new ArrayList<>();
Pet aPet = new Pet();
aPet.setId(1L);
aPet.setName("Covey's cat");
aPet.setAge(3);
aPet.setType(Animal.CAT);
pets.add(aPet);
return pets;
}
public List<Animal> animals() {
Animal animal = Animal.MAMMOTH;
Animal animal1 = Animal.BADGER;
Animal animal2 = Animal.CAT;
Animal animal3 = Animal.OOOOOO;
Animal animal4 = Animal.DOG;
List<Animal> animalList = new ArrayList<>(4);
animalList.add(animal);
animalList.add(animal1);
animalList.add(animal2);
animalList.add(animal3);
animalList.add(animal4);
return animalList;
}
}
9. 确认结构如下图所示,重启应用
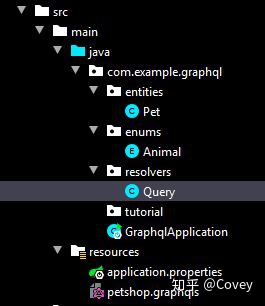
10. 成功运行后打开浏览器 http://localhost:8080/graphiql
在左侧输入以下请求:(可自行删改),点击运行按钮
{
pets {
name
type
age
}
animals
}
11. 根据请求格式,成功响应数据
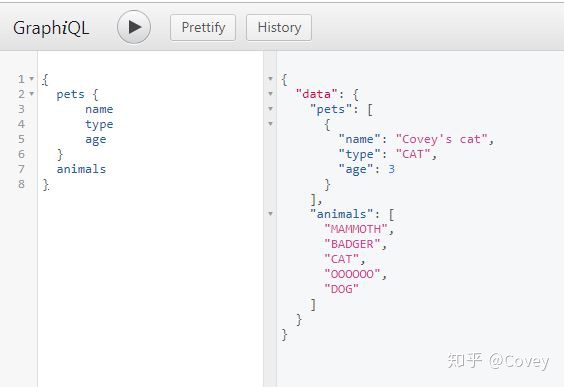
12. 尝试改变请求格式,获得不同数据,比如我只想获得宠物的名字
{
pets {
name
}
}
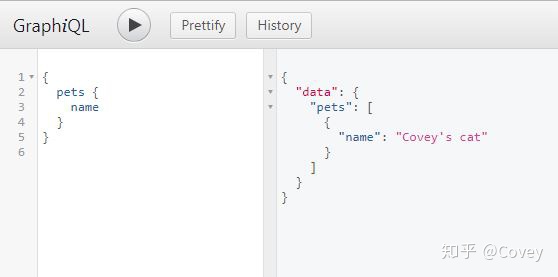