基本介绍
1) 客户端不应该依赖它不需要的接
口,即一个类对另一个类的依赖
应该建立在最小的接口上
2) 先看一张图:
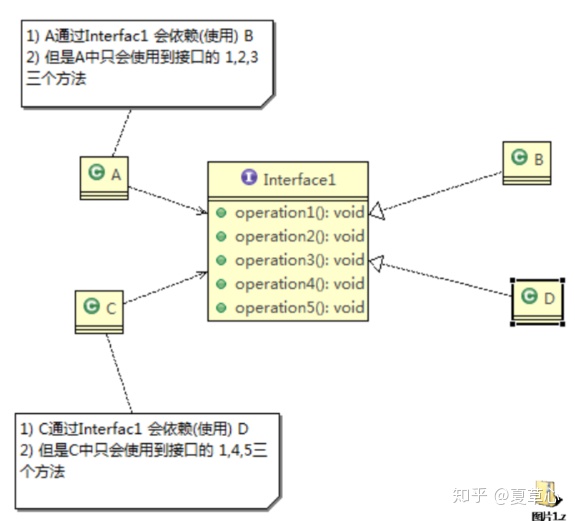
3)
类A通过接口Interface1依赖类B,类C通过
接口Interface1依赖类D,如果接口
Interface1对于类A和类C来说不是最小接口,
那么类B和类D必须去实现他们不需要的方
法。
4)
按隔离原则应当这样处理:
将接口Interface1拆分为独立的几个接口,
类A和类C分别与他们需要的接口建立依赖
关系。也就是采用接口隔离原则
应用实例
1) 类A通过接口Interface1依赖类B,
类C通过接口Interface1依赖类D,
请编写代码完成此应用实例。
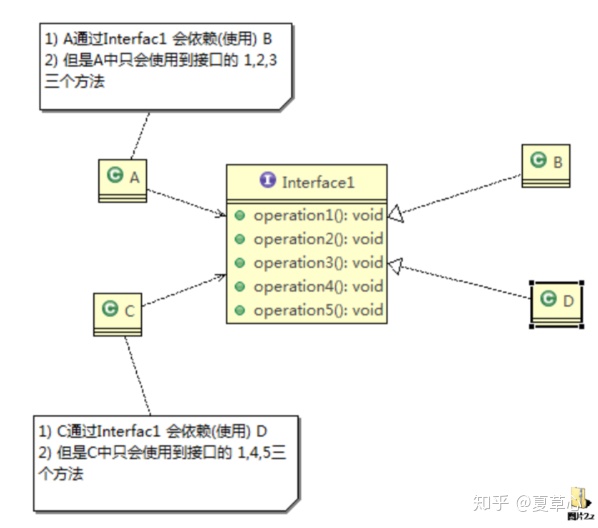
代码
namespace Segregation1 {
/** 1.类A通过接口Interface1依赖类B,类C通过
接口Interface1依赖类D,如果接口
Interface1对于类A和类C来说不是最小接口,
那么类B和类D必须去实现他们不需要的方
法。
2.按隔离原则应当这样处理:
将接口Interface1拆分为独立的几个接口,
类A和类C分别与他们需要的接口建立依赖
关系。也就是采用接口隔离原则
*/
interface Interface1 {
opreation1(): void;
opreation2(): void;
opreation3(): void;
opreation4(): void;
opreation5(): void;
}
class B implements Interface1 {
opreation1(): void {
console.log('B 实现了 operation1');
}
opreation2(): void {
console.log('B 实现了 operation2');
}
opreation3(): void {
console.log('B 实现了 operation3');
}
opreation4(): void {
console.log('B 实现了 operation4');
}
opreation5(): void {
console.log('B 实现了 operation5');
}
}
class D implements Interface1 {
opreation1(): void {
console.log('D 实现了 operation1');
}
opreation2(): void {
console.log('D 实现了 operation2');
}
opreation3(): void {
console.log('D 实现了 operation3');
}
opreation4(): void {
console.log('D 实现了 operation4');
}
opreation5(): void {
console.log('D 实现了 operation5');
}
}
//A类通过接口Interface1依赖(使用)B类,但是只会用到1,2,3方法
class A {
depend1(i: Interface1): void {
i.opreation1();
}
depend2(i: Interface1): void {
i.opreation2();
}
depend3(i: Interface1): void {
i.opreation3();
}
}
//C类通过接口Interface1依赖(使用)B类,但是只会用到1,4,5方法
class C {
depend1(i: Interface1): void {
i.opreation1();
}
depend4(i: Interface1): void {
i.opreation4();
}
depend5(i: Interface1): void {
i.opreation5();
}
}
}
应传统方法的问题和使用接口隔离原则改进
1) 类A通过接口Interface1依赖类B,类C通过接口Interface1依赖类D,如果接口
Interface1对于类A和类C来说不是最小接口,那么类B和类D必须去实现他们不
需要的方法
2) 将接口Interface1拆分为独立的几个接口,类A和类C分别与他们需要的接口建立
依赖关系。也就是采用接口隔离原则
3) 接口Interface1中出现的方法,根据实际情况拆分为三个接口
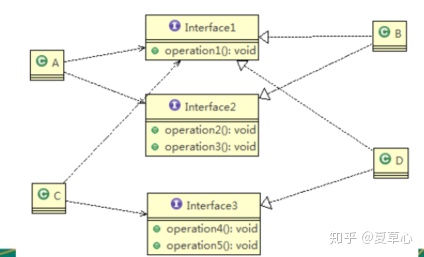
4) 代码实现
namespace Segregation1 {
/** 1.类A通过接口Interface1依赖类B,类C通过
接口Interface1依赖类D,如果接口
Interface1对于类A和类C来说不是最小接口,
那么类B和类D必须去实现他们不需要的方
法。
2.按隔离原则应当这样处理:
将接口Interface1拆分为独立的几个接口,
类A和类C分别与他们需要的接口建立依赖
关系。也就是采用接口隔离原则
*/
//接口1
interface Interface1 {
opreation1(): void;
}
//接口2
interface Interface2 {
opreation2(): void;
opreation3(): void;
}
//接口3
interface Interface3 {
opreation4(): void;
opreation5(): void;
}
class B implements Interface1, Interface2 {
opreation1(): void {
console.log('B 实现了 operation1');
}
opreation2(): void {
console.log('D 实现了 operation2');
}
opreation3(): void {
console.log('D 实现了 operation3');
}
}
class D implements Interface1, Interface3 {
opreation1(): void {
console.log('D 实现了 operation1');
}
opreation4(): void {
console.log('D 实现了 operation4');
}
opreation5(): void {
console.log('D 实现了 operation5');
}
}
//A类通过接口Interface1,Interface2依赖(使用)B类,但是只会用到1,2,3方法
class A {
depend1(i: Interface1): void {
i.opreation1();
}
depend2(i: Interface2): void {
i.opreation2();
}
depend3(i: Interface2): void {
i.opreation3();
}
}
//C类通过接口Interface1,Interface3依赖(使用)B类,但是只会用到1,4,5方法
class C {
depend1(i: Interface1): void {
i.opreation1();
}
depend4(i: Interface3): void {
i.opreation4();
}
depend5(i: Interface3): void {
i.opreation5();
}
}
let a = new A();
a.depend1(new B());//A类通过接口去依赖B类
a.depend2(new B());
a.depend3(new B());
let c = new C();//C类通过接口D去依赖D类
c.depend1(new D());
c.depend4(new D());
c.depend5(new D());
}