Github上的http://SQLite.net应该算是C#使用SQLite数据库最简单的方法了。
https://github.com/praeclarum/sqlite-net
1、环境配置
1》安装http://SQLite.net
1】Nuget安装:sqlite-net-pcl or sqlite-net-sqlcipher
2】源代码安装:SQLite.cs
2》安装SQLite3:复制sqlite3.dll到工作目录或者系统PATH目录
2、创建数据表(Table)
1》支持的数据类型
1】Int32=int32
2】Byte|UInt16|SByte|Int16=int32
3】bool=0|1
4】Single|Double|Decimal=double
5】TimeSpan=long
6】DateTime=long|string
//if StoreDateTimeAsTicks==true,stored as long
7】DateTimeOffset=long
8】byte[]=byte[](blob)
9】Guid=string
10】Uri=string
11】UriBuilder=string
12】enum=int|string
//if apply StoreAsTextAttribute,stored as string
13】Nullable<T>
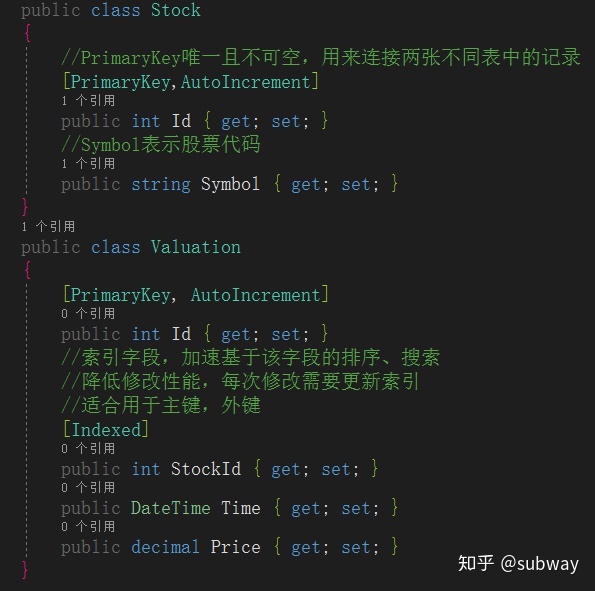
3、连接数据库
var db = new SQLiteConnection("foofoo");
4、创建表
1》返回值:CreateTableResult
1】Created:新建表
2】Migrated:存在旧表,将新的字段加入旧表
db.CreateTable<Stock>();
db.CreateTable<Valuation>();
2》创建多个表:CreateTables
1】泛型版本可以最多指定5个类型,非泛型版本不限数量
CreateTablesResult CreateTables<T, T2, T3, T4, T5> (CreateFlags createFlags = CreateFlags.None)
public CreateTablesResult CreateTables (CreateFlags createFlags = CreateFlags.None, params Type[] types)
2】返回值:通过CreateTablesResult.Result[type]确认是否创建成功
public class CreateTablesResult
{
public Dictionary<Type, CreateTableResult> Results { get; private set; }
public CreateTablesResult ()
{
Results = new Dictionary<Type, CreateTableResult> ();
}
}
5、插入记录
1》Insert:[PrimaryKey,AutoIncrement]修饰的属性无需赋值,赋值无效
var rowAdded=db.Insert(new Stock() { Symbol = "ChongHong" });
Console.WriteLine("记录加入数量:"+rowAdded);
2》尝试插入,如果违反唯一的约束则替代//使用UniqueAttribute修饰的属性
public int InsertOrReplace (object obj)
3》插入多数据,默认启动事务
public int InsertAll (System.Collections.IEnumerable objects, bool runInTransaction = true)
//extra=“OR REPLACE”则启用替代插入
public int InsertAll (System.Collections.IEnumerable objects, string extra, bool runInTransaction = true)
4》插入blob数据
public byte[] Data { get; set; }
6、查询数据
1》完整查询,无变量
List<Valuation> query=db.Query<Valuation>("select * from Valuation where Id=1")
2》完整查询,有变量:?作为占位符,被后续参数替代
public List<T> Query<T> (string query, params object[] args) where T : new()
var vals = db.Query<Valuation>("select * from Valuation where ?>?","Id",3);
3》查询返回不同的数据类型
public class ValuationInfo
{
public DateTime Date { get; set; }
public decimal Money { get; set; }
}
List<ValuationInfo> query=db.Query<ValuationInfo>(@"select Time as Date,Price as Money from Valuation where Id =1");
4》查询单个字段:ExecuteScalar<T>
var price=db.ExecuteScalar<decimal>("select Price from Valuation where Id=?", 6);
5》查询单条记录Find,如果没有记录不会报错,参数为主键值
var v = db.Find<Valuation>(6);
6》查询单条记录Get,如果没有记录会报错,参数为主键值
Valuation v = db.Get<Valuation>(2);
7、更新记录
1》Execute
1】无变量,字符串参数必须使用引号
db.Execute(@"update Stock set Symbol='Gree' where Id=1");
2】有变量
public int Execute (string query, params object[] args)
db.Execute(@"update Stock set Symbol=? where Id=?","Ali",1);
2》Update
1】传入的对象必须指定PK属性的值,该值作为条件
2】对象未赋值的属性被更改为默认值
3】返回值为更改的记录数量
var r=db.Update(new Valuation() { Price=8.8M,Time=DateTime.Now,Id=1 });
//相当于执行以下SQL语句
//update Valuation set Price=8.8, set Time=当前时间, set StockId=0 where Id=1
3》UpdateAll
1】参数1:修改对象的集合。相当于对传入的每个对象执行Update操作
2】参数2:指定是否为原子性操作
3】返回值:修改记录的数量
List<Valuation> newVals = new List<Valuation>();
newVals.Add(new Valuation() { Price = 1M, StockId = 1, Id = 1 });
newVals.Add(new Valuation() { Price = 2M, StockId = 2, Id = 2 });
newVals.Add(new Valuation() { Price = 3M, StockId = 3, Id = 3 });
var r=db.UpdateAll(newVals,true);
9、删除记录
1》Delete (object objectToDelete):只需指定PK属性的值
var r=db.Delete(new Valuation() { Id = 1 });
2》Delete<T> (object primaryKey):只需传入PK值
db.Delete<Valuation>(4);
3》DeleteAll<T> ():删除指定表的所有数据!!!
10、删除表
1》public int DropTable<T> ():丢弃表,不可恢复
10、备份数据库
1》Backup (string destinationDatabasePath, string databaseName = "main")
//只需传入参数一,备份数据库的名称
//还没弄清备份原理,是把数据库文件作为整个文件复制的粗备份,还是记录级别的细备份,猜测是细备份,不然太low了……
db.Backup("foofoo.bak");
11、其他函数
1》public TableQuery<T> Table<T> () :返回一个可供查询的表
2》public TableMapping GetMapping (Type type, CreateFlags createFlags = CreateFlags.None):返回根据类型自动创建的映射表
3》public int CreateIndex (string indexName, string tableName, string[] columnNames, bool unique = false):为指定列创建索引
4》public void RunInTransaction (Action action):事务性操作
db.RunInTransactionAsync(tran => {
// database calls inside the transaction
tran.Insert(stock);
tran.Insert(valuation);
});
5》public string SaveTransactionPoint ():创建还原点
var save = db.SaveTransactionPoint();
db.RollbackTo(save);//还原记录
12、属性
1》public bool Trace { get; set; }:是否记录操作
2》public Action<string> Tracer { get; set; }:记录操作的自定义委托
db.Trace = true;
db.Tracer = str =>
{
using (var streamWriter = new StreamWriter("db.log", append: true, encoding:Encoding.UTF8))
{
streamWriter.WriteLine(DateTime.Now.ToString()+'t'+str);
}
};
//更改源码,不然CommandText的值很迷
if (Connection.Trace) {
var text = CommandText;
foreach (var data in source)
{
var index = text.IndexOf('?');
if (index>=0)
{
text=text.Remove(index, 1);
text = text.Insert(index, data.ToString());
}
else
{
break;
}
}
Connection.Tracer?.Invoke ("Executing: " + text);
}
3》public bool TimeExecution { get; set; }:是否记录错误时间
4》public bool StoreDateTimeAsTicks { get; private set; }:是否将时间存储为Ticks,默认true