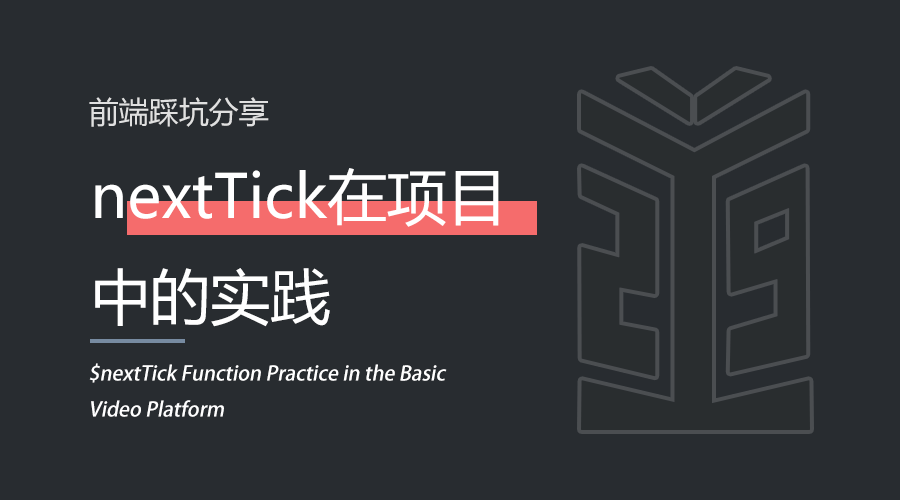
前言
在项目中经常需要在视图层立即显示数据,而有时候由于异步数据传递的原因,在页面上并不会立即显示页面,这时候就需要使用Vue提供的nextTick这个方法,其主要原因是Vue的数据视图是异步更新的,用官方的解释就是:
Vue 实现响应式并不是数据发生变化之后 DOM 立即变化,而是按一定的策略进行 DOM 的更新。
其中说到的事件循环也是前端面试中常问到的一个点,本文不做具体展开,有兴趣的同学可参考这篇文章 一次弄懂Event Loop(彻底解决此类面试问题)
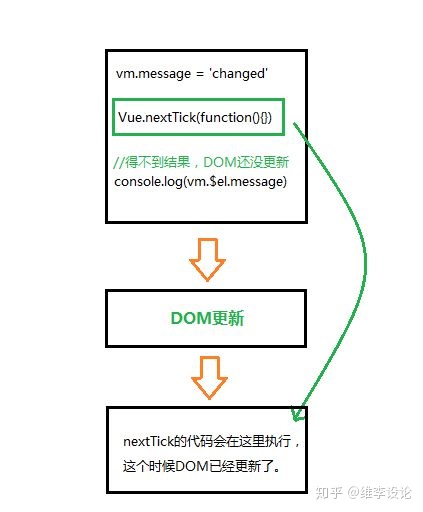
踩坑目录
- 模板案例数据在视图上显示
- 兄弟组件间异步数据传递
- $nextTick源码实现解析
踩坑案例
模板案例数据在视图上显示
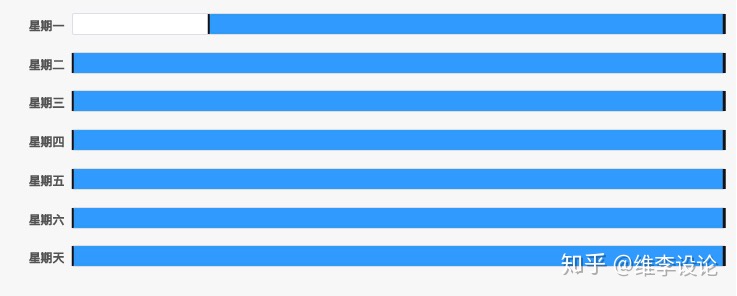
[bug描述] 页面上点击重置后将模板视图渲染会一个固定数据下的视图
[bug分析] 点击后需要立即显示在页面上,这是典型的nextTick需要应用的场景
[解决方案]
此处还有一个坑就是对于数组类型的监听是基于一个地址的,因而如果需要Vue的Watcher能够监视到就需要符合数组监听的那几种方法,这里直接新建,相当于每次的地址都会发生变化,因而可以监听到
async resetTemplate() { this.template = []; await this.$nextTick(function() { this.template = [ { week: '1', starttime: '00:00:00', endtime: '00:00:00' }, { week: '2', starttime: '00:00:00', endtime: '00:00:00' }, { week: '3', starttime: '00:00:00', endtime: '00:00:00' }, { week: '4', starttime: '00:00:00', endtime: '00:00:00' }, { week: '5', starttime: '00:00:00', endtime: '00:00:00' }, { week: '6', starttime: '00:00:00', endtime: '00:00:00' }, { week: '7', starttime: '00:00:00', endtime: '00:00:00' } ]; }); }
兄弟组件间异步数据传递
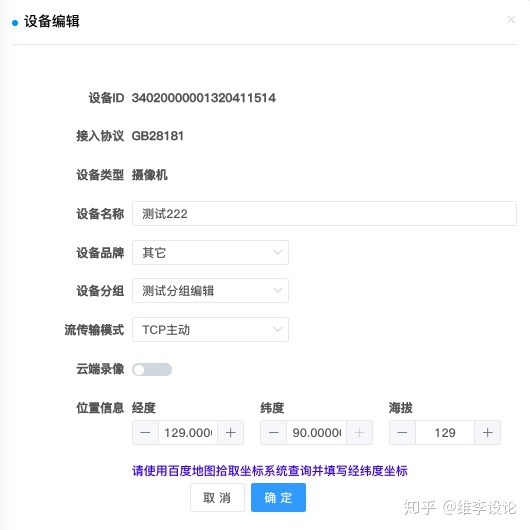
[bug描述] 页面修改弹窗中的输入框字段需要复写进对应字段,利用Props传递数据进去后并不会直接修改数据
[bug分析] 此场景下数据是通过子组件emit给父组件,父组件获取数据后通过props传递给弹窗,在v-model中获取数据是异步的
[解决方案]
这是比较不常见的一种使用$nextTick去处理v-model异步数据传递的方法(ps: 关于emit/on的发布订阅相关的介绍,有兴趣的同学可以看一下这篇文章 vue发布订阅者模式$emit、$on),利用的是父组件的数据延迟到下一个tick去给子组件传递,子组件在对应页面上及时渲染的方法,除了这种方法还有其他方法,具体可参考这篇文章 详解vue父组件传递props异步数据到子组件的问题
edit(data) { this.isManu = true; let [content,pos] = data; this.manuPos = pos; this.form = content; this.$nextTick(function(){ this.$refs.deviceEdit.form.deviceid = content.deviceId; this.$refs.deviceEdit.form.devicename = content.deviceName; this.$refs.deviceEdit.form.devicebrand = content.deviceBrand; this.$refs.deviceEdit.form.devicegroup = content.deviceGroup; this.$refs.deviceEdit.form.mediatrans = content.mediaTrans; this.$refs.deviceEdit.form.cloudstorage = content.cloudStorage; this.$refs.deviceEdit.form.longitude = content.longitude; this.$refs.deviceEdit.form.latitude = content.latitude; this.$refs.deviceEdit.form.altitude = content.altitude; }) },
$nextTick源码实现解析
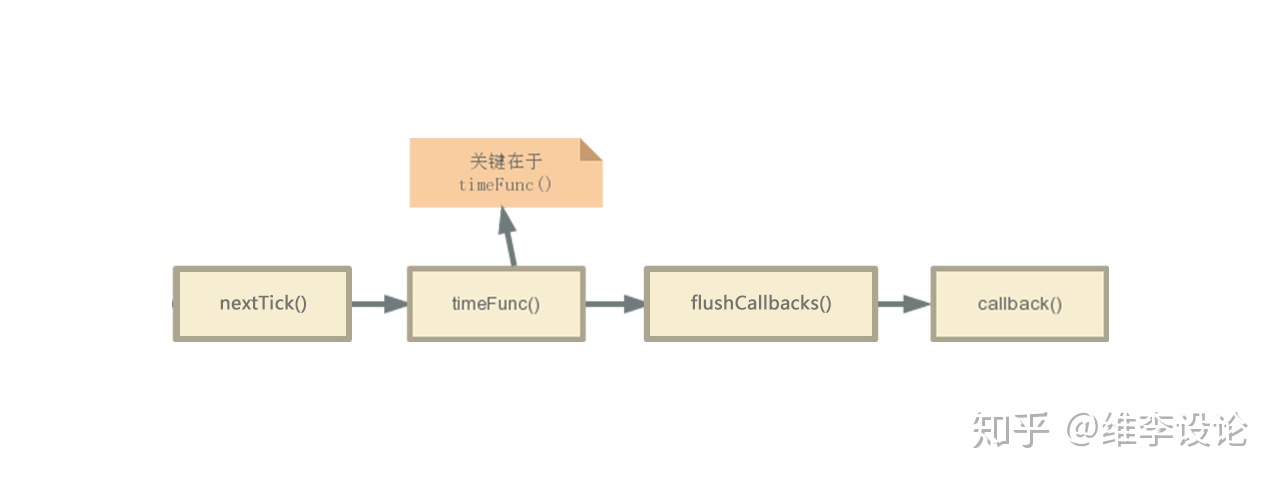
2.5之前的版本:
/** * Defer a task to execute it asynchronously. */ export const nextTick = (function () { const callbacks = [] let pending = false let timerFunc function nextTickHandler () { pending = false const copies = callbacks.slice(0) callbacks.length = 0 for (let i = 0; i < copies.length; i++) { copies[i]() } } // the nextTick behavior leverages the microtask queue, which can be accessed // via either native Promise.then or MutationObserver. // MutationObserver has wider support, however it is seriously bugged in // UIWebView in iOS >= 9.3.3 when triggered in touch event handlers. It // completely stops working after triggering a few times... so, if native // Promise is available, we will use it: /* istanbul ignore if */ if (typeof Promise !== 'undefined' && isNative(Promise)) { var p = Promise.resolve() var logError = err => { console.error(err) } timerFunc = () => { p.then(nextTickHandler).catch(logError) // in problematic UIWebViews, Promise.then doesn't completely break, but // it can get stuck in a weird state where callbacks are pushed into the // microtask queue but the queue isn't being flushed, until the browser // needs to do some other work, e.g. handle a timer. Therefore we can // "force" the microtask queue to be flushed by adding an empty timer. if (isIOS) setTimeout(noop) } } else if (!isIE && typeof MutationObserver !== 'undefined' && ( isNative(MutationObserver) || // PhantomJS and iOS 7.x MutationObserver.toString() === '[object MutationObserverConstructor]' )) { // use MutationObserver where native Promise is not available, // e.g. PhantomJS, iOS7, Android 4.4 var counter = 1 var observer = new MutationObserver(nextTickHandler) var textNode = document.createTextNode(String(counter)) observer.observe(textNode, { characterData: true }) timerFunc = () => { counter = (counter + 1) % 2 textNode.data = String(counter) } } else { // fallback to setTimeout /* istanbul ignore next */ timerFunc = () => { setTimeout(nextTickHandler, 0) } } return function queueNextTick (cb?: Function, ctx?: Object) { let _resolve callbacks.push(() => { if (cb) { try { cb.call(ctx) } catch (e) { handleError(e, ctx, 'nextTick') } } else if (_resolve) { _resolve(ctx) } }) if (!pending) { pending = true timerFunc() } if (!cb && typeof Promise !== 'undefined') { return new Promise((resolve, reject) => { _resolve = resolve }) } } })()
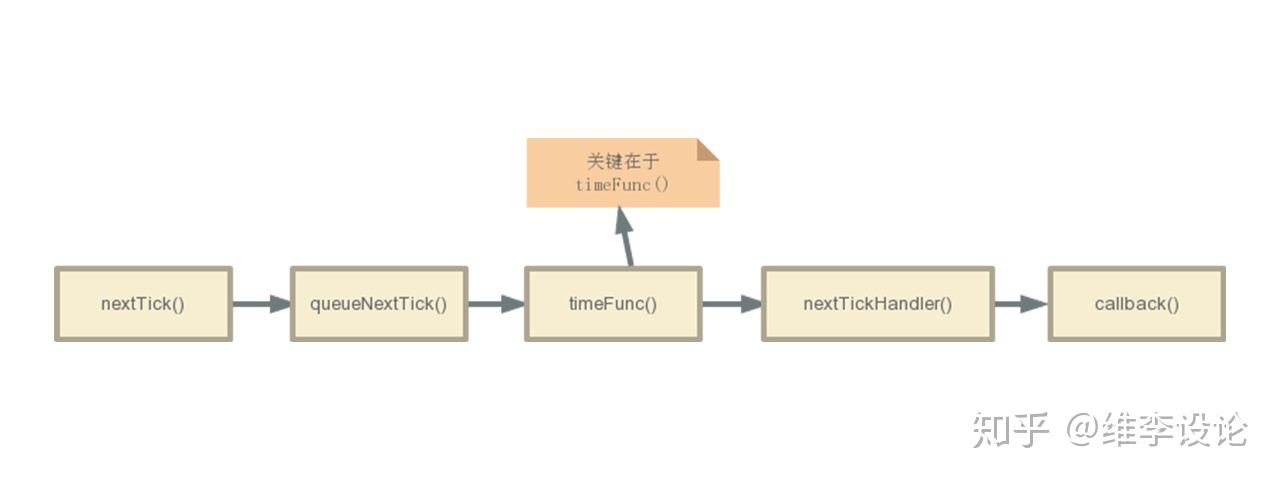
2.5之后的版本
/* @flow */ /* globals MutationObserver */ import { noop } from 'shared/util' import { handleError } from './error' import { isIE, isIOS, isNative } from './env' export let isUsingMicroTask = false const callbacks = [] let pending = false function flushCallbacks () { pending = false const copies = callbacks.slice(0) callbacks.length = 0 for (let i = 0; i < copies.length; i++) { copies[i]() } } // Here we have async deferring wrappers using microtasks. // In 2.5 we used (macro) tasks (in combination with microtasks). // However, it has subtle problems when state is changed right before repaint // (e.g. #6813, out-in transitions). // Also, using (macro) tasks in event handler would cause some weird behaviors // that cannot be circumvented (e.g. #7109, #7153, #7546, #7834, #8109). // So we now use microtasks everywhere, again. // A major drawback of this tradeoff is that there are some scenarios // where microtasks have too high a priority and fire in between supposedly // sequential events (e.g. #4521, #6690, which have workarounds) // or even between bubbling of the same event (#6566). let timerFunc // The nextTick behavior leverages the microtask queue, which can be accessed // via either native Promise.then or MutationObserver. // MutationObserver has wider support, however it is seriously bugged in // UIWebView in iOS >= 9.3.3 when triggered in touch event handlers. It // completely stops working after triggering a few times... so, if native // Promise is available, we will use it: /* istanbul ignore next, $flow-disable-line */ if (typeof Promise !== 'undefined' && isNative(Promise)) { const p = Promise.resolve() timerFunc = () => { p.then(flushCallbacks) // In problematic UIWebViews, Promise.then doesn't completely break, but // it can get stuck in a weird state where callbacks are pushed into the // microtask queue but the queue isn't being flushed, until the browser // needs to do some other work, e.g. handle a timer. Therefore we can // "force" the microtask queue to be flushed by adding an empty timer. if (isIOS) setTimeout(noop) } isUsingMicroTask = true } else if (!isIE && typeof MutationObserver !== 'undefined' && ( isNative(MutationObserver) || // PhantomJS and iOS 7.x MutationObserver.toString() === '[object MutationObserverConstructor]' )) { // Use MutationObserver where native Promise is not available, // e.g. PhantomJS, iOS7, Android 4.4 // (#6466 MutationObserver is unreliable in IE11) let counter = 1 const observer = new MutationObserver(flushCallbacks) const textNode = document.createTextNode(String(counter)) observer.observe(textNode, { characterData: true }) timerFunc = () => { counter = (counter + 1) % 2 textNode.data = String(counter) } isUsingMicroTask = true } else if (typeof setImmediate !== 'undefined' && isNative(setImmediate)) { // Fallback to setImmediate. // Technically it leverages the (macro) task queue, // but it is still a better choice than setTimeout. timerFunc = () => { setImmediate(flushCallbacks) } } else { // Fallback to setTimeout. timerFunc = () => { setTimeout(flushCallbacks, 0) } } export function nextTick (cb?: Function, ctx?: Object) { let _resolve callbacks.push(() => { if (cb) { try { cb.call(ctx) } catch (e) { handleError(e, ctx, 'nextTick') } } else if (_resolve) { _resolve(ctx) } }) if (!pending) { pending = true timerFunc() } // $flow-disable-line if (!cb && typeof Promise !== 'undefined') { return new Promise(resolve => { _resolve = resolve }) } }
不同版本主要在于timeFunc的异步函数使用优先顺序不同,2.5之后也有些许不同,但主要在于要不要暴露微任务函数和宏任务函数的问题(ps:上边的2.5之后的版本是2.6.11)
2.5之前版本: Promise => MutationObserver => setTimeout
2.5之后版本: setImmediate => MessageChannel => Promise => setTimeout
总结
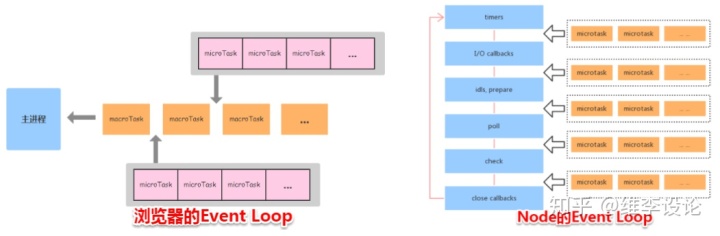
js的异步执行机制是前端同学必须掌握的知识,其中nextTick就是其中一个很典型的代表,node中也有nextTick相关的方法,面试中也常常问到相关方法的实现,深刻理解js的基础方法和特性,对前端开发中避坑还是很有用处的,每每出现问题几乎在所有的面试题中都有相关知识的展现,打好基础永远是一个工程师上升的坚实的基础!
let callbacks = [] let pending = false function nextTick (cb) { callbacks.push(cb) if (!pending) { pending = true setTimeout(flushCallback, 0) } } function flushCallback () { pending = false let copies = callbacks.slice() callbacks.length = 0 copies.forEach(copy => { copy() }) }
参考
- Vue.nextTick 的原理和用途
- 简单理解Vue中的nextTick
- nextTick源码解析
- Vue nextTick 机制
- Vue源码解析之nextTick
- 浅析Node的nextTick
- Nodejs的nextTick和setTimeout
- Vue.js中this.$nextTick()的使用
- vue发布订阅者模式$emit、$on
- 详解vue父组件传递props异步数据到子组件的问题
- 一次弄懂Event Loop(彻底解决此类面试问题)