unzip_save.py
import os
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2' # 不显示等级2以下的提示信息
import zipfile
# import random
# import tensorflow as tf
# from tensorflow.keras.optimizers import RMSprop
# from tensorflow.keras.preprocessing.image import ImageDataGenerator
# from shutil import copyfile
local_zip = 'E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human.zip' # 数据集压缩包路径
zip_ref = zipfile.ZipFile(local_zip, 'r') # 打开压缩包,以读取方式
zip_ref.extractall('E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human') # 解压到以下路径
zip_ref.close()
print(len(os.listdir('E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human/horses/'))) # 读取出解压文件夹“horses”文件夹下面文件数量
print(len(os.listdir('E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human/humans/'))) # 读取出解压文件夹“humans”文件夹下面文件数量
train_horse_dir = os.path.join('E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human/horses')
train_human_dir = os.path.join('E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human/humans')
train_horse_names = os.listdir(train_horse_dir)
train_human_names = os.listdir(train_human_dir)
model_training_fit.py
import os
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2' # 不显示等级2以下的提示信息
# import shutil
# import random
import tensorflow as tf
# from tensorflow import keras
from tensorflow.keras.optimizers import RMSprop
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# from shutil import copyfile
# import matplotlib.image as mpimg
import matplotlib.pyplot as plt
#======== 数据预处理 =========
TRAINING_DIR = "E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human/"
train_datagen = ImageDataGenerator(rescale = 1/255) # 归一化,减少计算量
train_generator = train_datagen.flow_from_directory(TRAINING_DIR, batch_size = 128, class_mode = 'binary', target_size = (300,300))
# VALIDATION_DIR = "tmp/cats-and-dogs/testing/"
# validation_datagen = ImageDataGenerator(rescale = 1/255)
# validation_generator = validation_datagen.flow_from_directory(VALIDATION_DIR, batch_size = 100, class_mode = 'binary', target_size = (150,150))
# Expected Output :
# Found 22498 images belonging to 2 classes.
# Found 2500 images belonging to 2 classes.
#======== 模型构建 =========
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(16, (3, 3), activation = 'relu', input_shape = (300, 300, 3)), # 输入参数:过滤器数量,过滤器尺寸,激活函数:relu, 输入图像尺寸
tf.keras.layers.MaxPooling2D(2, 2), # 池化:增强特征
tf.keras.layers.Conv2D(32, (3, 3), activation = 'relu'), # 输入参数:过滤器数量、过滤器尺寸、激活函数:relu
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(64, (3, 3), activation = 'relu'),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(64, (3, 3), activation = 'relu'),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(64, (3, 3), activation = 'relu'),
tf.keras.layers.MaxPooling2D(2, 2),
# Flatten the results to feed into a DNN
tf.keras.layers.Flatten(), # 输入层
# 512 neuron hidden layer
tf.keras.layers.Dense(512, activation = 'relu'), # 神经元数量:512 ,激活函数:relu
# Only 1 output neuron. It will contain a value from 0-1 where 0 for 1 class ('horse') and 1 for the other ('human')
tf.keras.layers.Dense(1, activation = 'sigmoid')
])
#======== 模型参数编译 =========
model.compile(optimizer = RMSprop(lr = 0.001), loss = 'binary_crossentropy', metrics = ['accuracy'])
# 输入参数:lr:学习步长 RMSprop算法:修正学习步长 loss:损失函数 metrics:网络性能度量
#======== 模型训练 =========
# Note that this may take some time.
history = model.fit_generator(train_generator, steps_per_epoch = 8, epochs = 2, verbose = 1) # verbose=1 表示记录网络拟合过程
model.save('E:/Python/pythonProject_1/horse-or-human/model.h5') # model 保存
#-----------------------------------------------------------
# Retrieve a list of list result on training and test data
# set for each training epoch
#-----------------------------------------------------------
acc = history.history['accuracy']
loss = history.history['loss']
epochs = range(len(acc)) # Get number of epochs
#-----------------------------------------------------------
# Plot training and validation accuracy per epoch
#-----------------------------------------------------------
plt.plot(epochs, acc, 'r', "train_acc")
plt.title("training accuracy")
plt.figure()
plt.show()
# 曲线呈直线是因为epochs/轮次太少
#-----------------------------------------------------------
# Plot training and validation loss per epoch
#-----------------------------------------------------------
plt.plot(epochs, loss, 'r', "train_loss")
plt.title("training loss")
plt.figure()
plt.show()
# 曲线呈直线是因为epochs/轮次太少
visualizing_intermediate_representation.py
import os
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2' # 不显示等级2以下的提示信息
import numpy as np
np.seterr(divide='ignore',invalid='ignore')
import random
import tensorflow as tf
from tensorflow.keras.preprocessing.image import img_to_array, load_img
import matplotlib.pyplot as plt
from tensorflow import keras
model = keras.models.load_model('model.h5')
successive_outputs = [layer.output for layer in model.layers[1:]]
visualization_model = tf.keras.models.Model(inputs = model.input, outputs = successive_outputs)
train_horse_dir = os.path.join('E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human/horses')
train_human_dir = os.path.join('E:/Python/pythonProject_1/horse-or-human/tmp/horse-or-human/humans')
train_horse_names = os.listdir(train_horse_dir)
train_human_names = os.listdir(train_human_dir)
horse_img_files = [os.path.join(train_horse_dir, f) for f in train_horse_names]
human_img_files = [os.path.join(train_human_dir, f) for f in train_human_names]
img_path = random.choice(horse_img_files + human_img_files)
img = load_img(img_path, target_size=(300, 300))
x = img_to_array(img)
x = x.reshape((1,) + x.shape)
x /= 255
successive_feature_maps = visualization_model.predict(x)
layer_names = [layer.name for layer in model.layers]
for layer_name, feature_map in zip(layer_names, successive_feature_maps):
if len(feature_map.shape) == 4:
n_features = feature_map.shape[-1]
size = feature_map.shape[1]
display_grid = np.zeros((size, size * n_features))
for i in range(n_features):
x = feature_map[0, :, :, i]
x -= x.mean()
x /= x.std()
x *= 64
x += 128
x = np.clip(x, 0, 255).astype('uint8')
display_grid[:, i * size : (i + 1) * size] = x
scale = 20. / n_features
plt.figure(figsize = (scale * n_features, scale))
plt.title(layer_name)
plt.grid(False)
plt.imshow(display_grid, aspect = 'auto', cmap = 'viridis')
plt.show()
main.py
# Here's a codeblock just for fun,You should be able to upload an image here
# and have it claassified without crashing
import numpy as np
# from google.colab import files
from tensorflow.keras.preprocessing import image
from tensorflow import keras
model = keras.models.load_model('model.h5')
# upload = files.upload()
#
# for fn in upload.key():
# # predicting images
# path = '/content/' + fn
# img = image.load_img(path, target_size=(150, 150))
# x = image.img_to_array(img)
# x = np.expand_dims(x, axis=0)
#
# images = np.vstack([x])
# classes = model.predict(images, batch_size=10)
# print(classes[0])
# if classes[0]>0.5:
# print(fn + " is a dog")
# else:
# print(fn + " is a cat")
# predicting images
path = 'E:\Python\pythonProject_1\horse-or-human\human.png'
img = image.load_img(path, target_size=(300, 300))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
images = np.vstack([x])
classes = model.predict(images, batch_size=10)
print(classes[0])
if classes[0]>0.5:
print(" is a human")
else:
print(" is a horse")
评估结果:
Epoch 1/2
8/8 [==============================] - 41s 5s/step - loss: 1.0350 - accuracy: 0.5284
Epoch 2/2
8/8 [==============================] - 30s 4s/step - loss: 0.6679 - accuracy: 0.5784
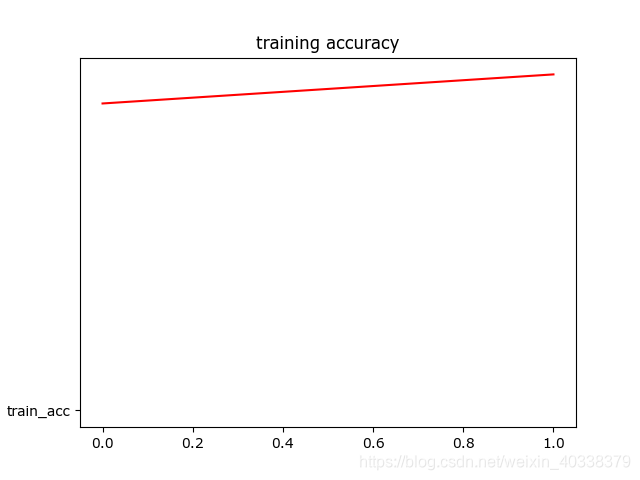
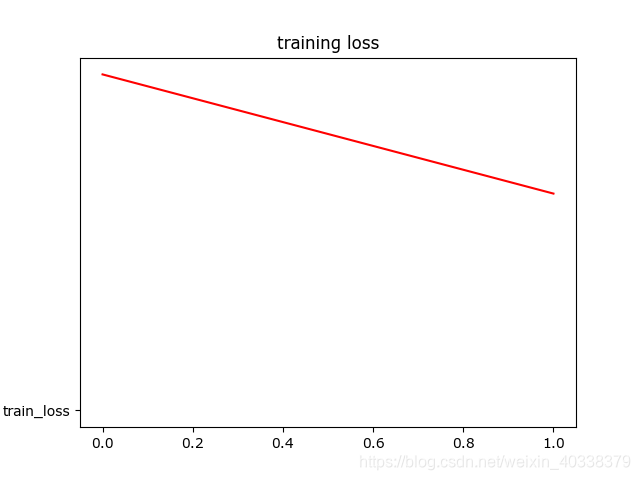
预测结果:
runfile('E:/Python/pythonProject_1/horse-or-human/main.py', wdir='E:/Python/pythonProject_1/horse-or-human')
[1.]
is a human