注意:
- 微信小程序不存在跨域问题。
- 在微信web开发者工具中,使用wx.request发出请求时,需要取消验证域名。
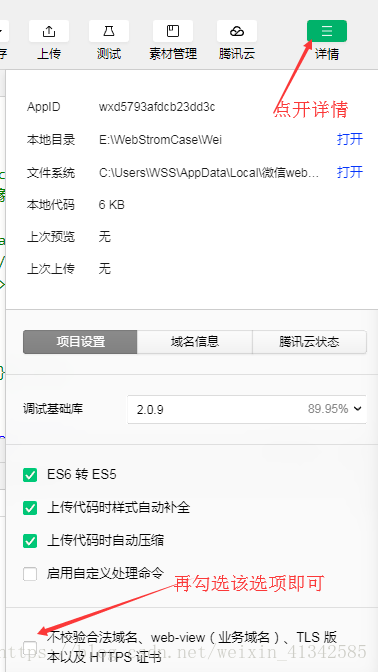
wx.request( object )
发起请求
常用的参数:
参数名 | 类型 | 说明 |
---|
url | String | 开发者服务器接口 |
data | Object、String、Array | 请求的参数 |
method | String | (必须大写)OPTIONS, GET, HEAD, POST, PUT, DELETE, TRACE, CONNECT |
dataType | String | json、text、html、xml、script |
responseType | String | 设置响应的数据类型。合法值:text、arraybuffer |
success | Function | 收到开发者服务成功返回的回调函数 |
fail | Function | 接口调用失败的回调函数 |
complete | Function | 接口调用结束的回调函数(调用成功、失败都会执行) |
wx.request({
url: 'http://219.229.222.45:6589/tag/songs/1/1',
method: "get",
dataType: "json",
success : function(data){
console.log(data.data.data.songItems)
}
});
wx.setStorage(OBJECT)
数据缓存
存储方式: 以键值对的形式存储(key – value)
将数据存储在本地缓存中指定的 key 中,会覆盖掉原来该 key 对应的内容,这是一个异步接口。
参数 | 类型 | 说明 |
---|
key | String | 本地缓存中的指定的 key |
data | Object/String | 需要存储的内容 |
success | Function | 接口调用成功的回调函数 |
fail | Function | 接口调用失败的回调函数 |
complete | Function | 接口调用结束的回调函数(调用成功、失败都会执行) |
wx.setStorage({
name : "Rose",
age : "25"
})
wx.getStorageInfo(OBJECT)
获取当前storage的相关信息
object 中的参数:
参数 | 类型 | 说明 |
---|
success | Function | 接口调用的回调函数,详见返回参数说明 |
fail | Function | 接口调用失败的回调函数 |
complete | Function | 接口调用结束的回调函数(调用成功、失败都会执行) |
success方法返回参数:
参数 | 类型 | 说明 |
---|
keys | String Array | 当前storage中所有的key |
currentSize | Number | 当前占用的空间大小, 单位kb |
limitSize | Number | 限制的空间大小,单位kb |
wx.getStorageInfo({
success: function(res) {
console.log(res.keys)
console.log(res.currentSize)
console.log(res.limitSize)
}
})
wx.removeStorage(OBJECT)
删除缓存
OBJECT参数说明:
参数 | 类型 | 说明 |
---|
key | String | 本地缓存中的指定的 key |
success | Function | 接口调用的回调函数 |
fail | Function | 接口调用失败的回调函数 |
complete | Function | 接口调用结束的回调函数(调用成功、失败都会执行) |
wx.removeStorage({
key: 'key',
success: function(res) {
console.log(res.data);
}
})
wx.clearStorage()
清理本地数据缓存。
wx.clearStorage()
wx.uploadFile
上传文件
wx.chooseImage({
success: function (res) {
var tempFilePaths = res.tempFilePaths;
console.log(tempFilePaths[0]);
wx.uploadFile({
url: 'http://127.0.0.1:80/request.php',
filePath: tempFilePaths[0],
name: 'file',
formData: {
'user': 'test'
},
success: function (res) {
var data = res.data
console.log(data);
console.log("成功了");
},
fail: function(){
console.log("失败了")
}
})
}
})
wx.downloadFile
下载文件
wx.downloadFile({
url: 'http://127.0.0.1/CSS3/images/1.jpg',
success: function (res) {
wx.saveImageToPhotosAlbum({
filePath: res.tempFilePath,
success: function (data) {
console.log(data);
},
fail: function (err) {
console.log(err);
console.log("下载文件失败");
}
})
}
});
wx.chooseImage
从本地相册选择图片或使用相机拍照
成员 | 作用 |
---|
count | 最多可选择的图片的张数 |
sizeType | original原图、compressed压缩图、默认二者都有 |
sourceType | album为从相册中选图,camera使用相机,默认二者都有 |
success | 成功的回调函数 |
fail | 失败的回调函数 |
complete | 完成的回调函数,无论失败还是成功 |
wx.chooseImage({
count: 1,
success: function(res) {
console.log(res.tempFilePaths);
console.log(res.tempFiles);
},
fail: function(){
console.log("失败了");
},
complete: function(){
console.log("选择照片完成");
}
})