import React, { useState, useEffect, useRef } from 'react';
import { Tooltip, Spin } from 'antd';
import noDataLogo from '@/assets/noData.png';
import './index.less';
import cx from 'classnames';
type TProps = {
dataMap: { [key: string]: [] };
loading: boolean;
intoView: boolean;
};
export const KanbanBar = (props: TProps) => {
const [versionDataMap, setVersionDataMap] = useState<{ [key: string]: [] }>(props.dataMap);
const messagesEndRef = useRef<HTMLDivElement>(null);
const wrapperRef = useRef<HTMLDivElement>(null);
const [wrapperWidth, setWrapperWidth] = useState<number>();
useEffect(() => {
setVersionDataMap(props.dataMap);
}, [props.dataMap]);
useEffect(() => {
if (messagesEndRef && messagesEndRef.current && props.intoView) {
messagesEndRef.current.scrollIntoView({ behavior: 'auto', block: 'end' });
}
}, [messagesEndRef, versionDataMap, props.intoView]);
useEffect(() => {
const handleScroll = () => {
if (wrapperRef.current?.clientWidth && wrapperRef.current?.scrollWidth) {
setWrapperWidth(
Object.keys(versionDataMap).length &&
Object.keys(versionDataMap).length * 170 < wrapperRef.current?.clientWidth
? wrapperRef.current?.clientWidth
: wrapperRef.current?.scrollWidth,
);
}
};
window.addEventListener('resize', handleScroll);
handleScroll();
return () => {
window.removeEventListener('resize', handleScroll);
};
}, [versionDataMap]);
return (
<div>
{!props.loading ? (
<div>
{Object.keys(versionDataMap).length > 0 ? (
<div className="wrapper" ref={wrapperRef}>
<div className="version-data-wrap">
<div className={'coordinate'} style={{ width: `${wrapperWidth}px` }}>
<div className="title">时间</div>
</div>
<div className={cx('wrap')}>
{versionDataMap &&
Object.keys(versionDataMap).map((key, i) => (
<div key={i}>
<div className={'version-data'}>
{versionDataMap[key].length > 0 &&
versionDataMap[key].map((item: any, index: number) => (
<Tooltip
key={index}
placement="top"
title={
<div>
<div>版本状态:{item.verState || '-'}</div>
<div>系统级别:{item.sysLevel || '-'}</div>
<div>测试负责人:{item.testLeaderName || '-'}</div>
<div>项目空间:{item.projectName || '-'}</div>
<div>版本依赖:{item.verDepend || '-'}</div>
</div>
}
>
<div
className={
/已超过/g.test(key) ? 'emerge-version-item' : 'version-item'
}
>
<div className="version-name">【{item.sysName || '-'}】</div>
<div className="version-code">{item.verName || '-'}</div>
</div>
</Tooltip>
))}
</div>
{versionDataMap[key].length > 0 && <div className="date">{key}</div>}
</div>
))}
</div>
</div>
<div
style={{ clear: 'both', height: '1px', width: '100%' }}
ref={messagesEndRef}
></div>
</div>
) : (
<div
style={{
width: '100%',
height: '300px',
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
}}
>
<img src={noDataLogo} alt="" />
</div>
)}
</div>
) : (
<div className="example">
<Spin />
</div>
)}
</div>
);
};
.wrapper{
height: 600px;
overflow-y: auto;
}
.coordinate{
position: absolute;
height: calc(100% - 45px);
border-left: 1px solid #999;
border-bottom: 1px solid #999;
.title{
display: inline-block;
position: absolute;
bottom: -33px;
right: 0;
}
}
.version-data-wrap{
position: relative;
min-height: 100%;
display: flex;
.wrap{
position: relative;
width: 100%;
min-width: 500px;
height: auto;
display: flex;
flex-direction: row;
align-items:flex-end;
.title{
display: inline-block;
position: absolute;
bottom: -45px;
right: 0;
}
}
}
.date{
text-align: center;
margin: 12px 20px 12px 20px;
width: 130px;
}
.version-data{
display: flex;
flex-direction: column;
.version-item{
background-color: #F5FFED;
color: #5FAD35;
border: 1px solid #5FAD35;
margin: 12px 20px 12px 20px;
z-index: 3;
cursor: pointer;
width: 130px;
min-width: 100px;
padding: 0 4px;
.version-name{
text-align: center;
}
.version-code{
text-align: center;
}
}
.emerge-version-item{
background-color: #FFF0F1;
color: #DE5257;
border: 1px solid #DE5257;
margin: 12px 20px 12px 20px;
z-index: 3;
width: 130px;
padding: 0 4px;
cursor: pointer;
.version-name{
text-align: center;
}
.version-code{
text-align: center;
}
}
}
.example {
margin: 20px 0;
margin-bottom: 20px;
padding: 30px 50px;
text-align: center;
border-radius: 4px;
}
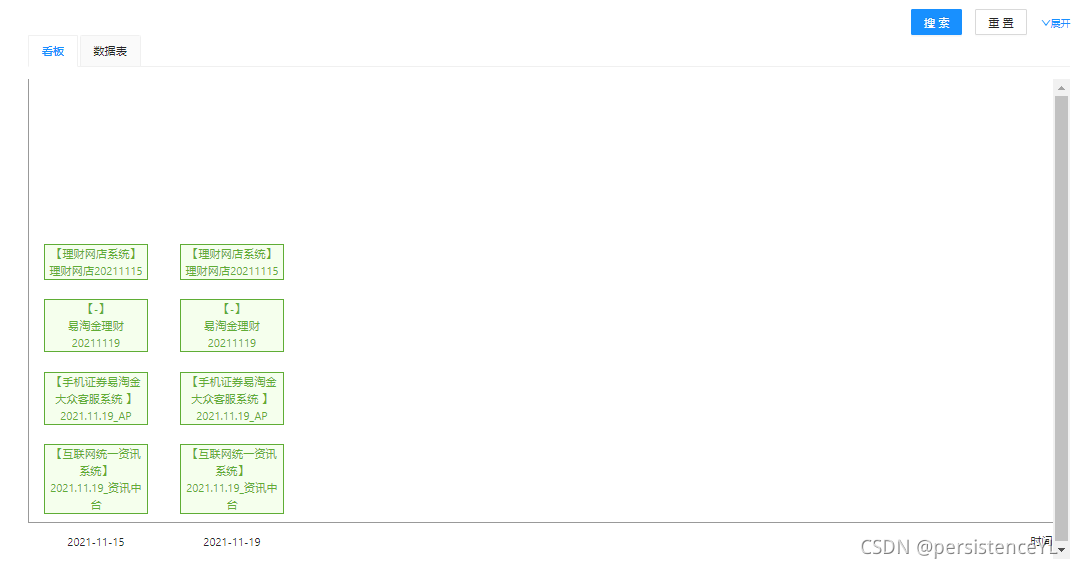