加密
字符串
public static String encode(String encodeKey,String content){
try {
KeyGenerator keygen= KeyGenerator.getInstance("AES");
SecureRandom random = SecureRandom.getInstance("SHA1PRNG");
random.setSeed(encodeKey.getBytes());
keygen.init(128, random);
SecretKey originalKey=keygen.generateKey();
byte [] raw=originalKey.getEncoded();
SecretKey key=new SecretKeySpec(raw, "AES");
Security.addProvider(new BouncyCastleProvider());
Cipher cipher=Cipher.getInstance("AES","BC");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] data = content.getBytes("utf-8");
int inputLen = data.length;
ByteArrayOutputStream out = new ByteArrayOutputStream();
int offSet = 0;
byte[] cache;
int i = 0;
while (inputLen - offSet > 0) {
if (inputLen - offSet > MAX_ENCRYPT_BLOCK) {
cache = cipher.doFinal(data, offSet, MAX_ENCRYPT_BLOCK);
} else {
cache = cipher.doFinal(data, offSet, inputLen - offSet);
}
out.write(cache, 0, cache.length);
i++;
offSet = i * MAX_ENCRYPT_BLOCK;
}
byte[] encryptedData = out.toByteArray();
out.close();
return MyBase64Utils.encodeStringForString(encryptedData);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
map
public static String encode(String encodeKey, Map<String,Object> content){
try {
KeyGenerator keygen= KeyGenerator.getInstance("AES");
SecureRandom random = SecureRandom.getInstance("SHA1PRNG", "SUN");
random.setSeed(encodeKey.getBytes());
keygen.init(128, random);
SecretKey originalKey=keygen.generateKey();
byte [] raw=originalKey.getEncoded();
SecretKey key=new SecretKeySpec(raw, "AES");
Security.addProvider(new BouncyCastleProvider());
Cipher cipher=Cipher.getInstance("AES","BC");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] data = JsonUtils.toJson(content).getBytes();
int inputLen = data.length;
ByteArrayOutputStream out = new ByteArrayOutputStream();
int offSet = 0;
byte[] cache;
int i = 0;
while (inputLen - offSet > 0) {
if (inputLen - offSet > MAX_ENCRYPT_BLOCK) {
cache = cipher.doFinal(data, offSet, MAX_ENCRYPT_BLOCK);
} else {
cache = cipher.doFinal(data, offSet, inputLen - offSet);
}
out.write(cache, 0, cache.length);
i++;
offSet = i * MAX_ENCRYPT_BLOCK;
}
byte[] encryptedData = out.toByteArray();
out.close();
return MyBase64Utils.encodeStringForString(encryptedData);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
解密
public static String decode(String encodeKey,String decodeContent){
try {
KeyGenerator keygen=KeyGenerator.getInstance("AES");
SecureRandom random = SecureRandom.getInstance("SHA1PRNG", "SUN");
random.setSeed(encodeKey.getBytes());
keygen.init(128, random);
SecretKey originalKey=keygen.generateKey();
byte [] raw=originalKey.getEncoded();
SecretKey key=new SecretKeySpec(raw, "AES");
Security.addProvider(new BouncyCastleProvider());
Cipher cipher=Cipher.getInstance("AES","BC");
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] encryptedData = MyBase64Utils.decodeStringForByte(decodeContent);
int inputLen = encryptedData.length;
ByteArrayOutputStream out = new ByteArrayOutputStream();
int offSet = 0;
byte[] cache;
int i = 0;
while (inputLen - offSet > 0) {
if (inputLen - offSet > MAX_DECRYPT_BLOCK) {
cache = cipher.doFinal(encryptedData, offSet, MAX_DECRYPT_BLOCK);
} else {
cache = cipher.doFinal(encryptedData, offSet, inputLen - offSet);
}
out.write(cache, 0, cache.length);
i++;
offSet = i * MAX_DECRYPT_BLOCK;
}
byte[] decryptedData = out.toByteArray();
out.close();
return new String(decryptedData);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
测试
public static void main(String[] args) {
String encode = encode("128742634", "I'm encode content");
String decode = decode("128742634", encode);
System.out.println("Decode result is :"+decode);
}
测试结果
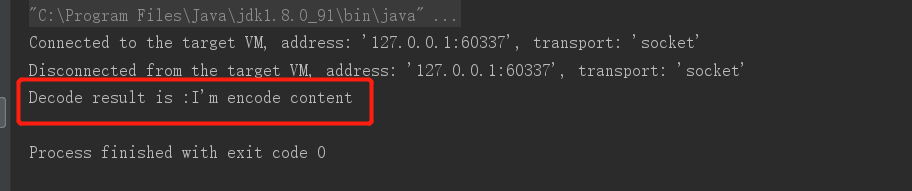
全代码
public class AESUtils {
private static final int MAX_ENCRYPT_BLOCK = 117;
private static final int MAX_DECRYPT_BLOCK = 128;
public static String encode(String encodeKey,String content){
try {
KeyGenerator keygen= KeyGenerator.getInstance("AES");
SecureRandom random = SecureRandom.getInstance("SHA1PRNG");
random.setSeed(encodeKey.getBytes());
keygen.init(128, random);
SecretKey originalKey=keygen.generateKey();
byte [] raw=originalKey.getEncoded();
SecretKey key=new SecretKeySpec(raw, "AES");
Security.addProvider(new BouncyCastleProvider());
Cipher cipher=Cipher.getInstance("AES","BC");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] data = content.getBytes("utf-8");
int inputLen = data.length;
ByteArrayOutputStream out = new ByteArrayOutputStream();
int offSet = 0;
byte[] cache;
int i = 0;
while (inputLen - offSet > 0) {
if (inputLen - offSet > MAX_ENCRYPT_BLOCK) {
cache = cipher.doFinal(data, offSet, MAX_ENCRYPT_BLOCK);
} else {
cache = cipher.doFinal(data, offSet, inputLen - offSet);
}
out.write(cache, 0, cache.length);
i++;
offSet = i * MAX_ENCRYPT_BLOCK;
}
byte[] encryptedData = out.toByteArray();
out.close();
return MyBase64Utils.encodeStringForString(encryptedData);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static String encode(String encodeKey, Map<String,Object> content){
try {
KeyGenerator keygen= KeyGenerator.getInstance("AES");
SecureRandom random = SecureRandom.getInstance("SHA1PRNG", "SUN");
random.setSeed(encodeKey.getBytes());
keygen.init(128, random);
SecretKey originalKey=keygen.generateKey();
byte [] raw=originalKey.getEncoded();
SecretKey key=new SecretKeySpec(raw, "AES");
Security.addProvider(new BouncyCastleProvider());
Cipher cipher=Cipher.getInstance("AES","BC");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] data = JsonUtils.toJson(content).getBytes();
int inputLen = data.length;
ByteArrayOutputStream out = new ByteArrayOutputStream();
int offSet = 0;
byte[] cache;
int i = 0;
while (inputLen - offSet > 0) {
if (inputLen - offSet > MAX_ENCRYPT_BLOCK) {
cache = cipher.doFinal(data, offSet, MAX_ENCRYPT_BLOCK);
} else {
cache = cipher.doFinal(data, offSet, inputLen - offSet);
}
out.write(cache, 0, cache.length);
i++;
offSet = i * MAX_ENCRYPT_BLOCK;
}
byte[] encryptedData = out.toByteArray();
out.close();
return MyBase64Utils.encodeStringForString(encryptedData);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static String decode(String encodeKey,String decodeContent){
try {
KeyGenerator keygen=KeyGenerator.getInstance("AES");
SecureRandom random = SecureRandom.getInstance("SHA1PRNG", "SUN");
random.setSeed(encodeKey.getBytes());
keygen.init(128, random);
SecretKey originalKey=keygen.generateKey();
byte [] raw=originalKey.getEncoded();
SecretKey key=new SecretKeySpec(raw, "AES");
Security.addProvider(new BouncyCastleProvider());
Cipher cipher=Cipher.getInstance("AES","BC");
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] encryptedData = MyBase64Utils.decodeStringForByte(decodeContent);
int inputLen = encryptedData.length;
ByteArrayOutputStream out = new ByteArrayOutputStream();
int offSet = 0;
byte[] cache;
int i = 0;
while (inputLen - offSet > 0) {
if (inputLen - offSet > MAX_DECRYPT_BLOCK) {
cache = cipher.doFinal(encryptedData, offSet, MAX_DECRYPT_BLOCK);
} else {
cache = cipher.doFinal(encryptedData, offSet, inputLen - offSet);
}
out.write(cache, 0, cache.length);
i++;
offSet = i * MAX_DECRYPT_BLOCK;
}
byte[] decryptedData = out.toByteArray();
out.close();
return new String(decryptedData);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static void main(String[] args) {
String encode = encode("128742634", "I'm encode content");
String decode = decode("128742634", encode);
System.out.println("Decode result is :"+decode);
}
}
>>>源码下载链接>>>