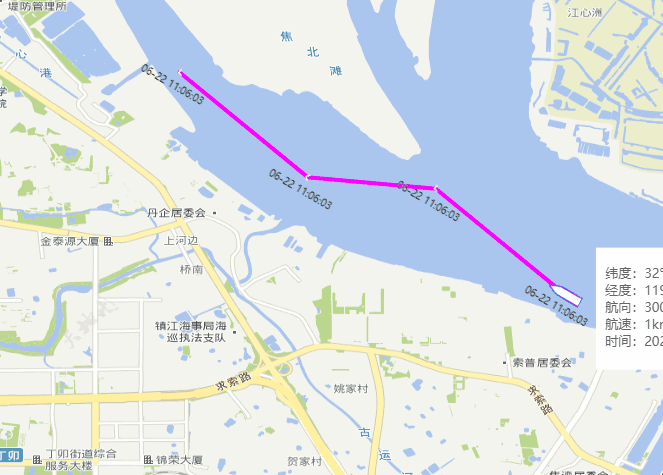
ol官方文档
ol官方文档
安装
npm install ol --save
安装后
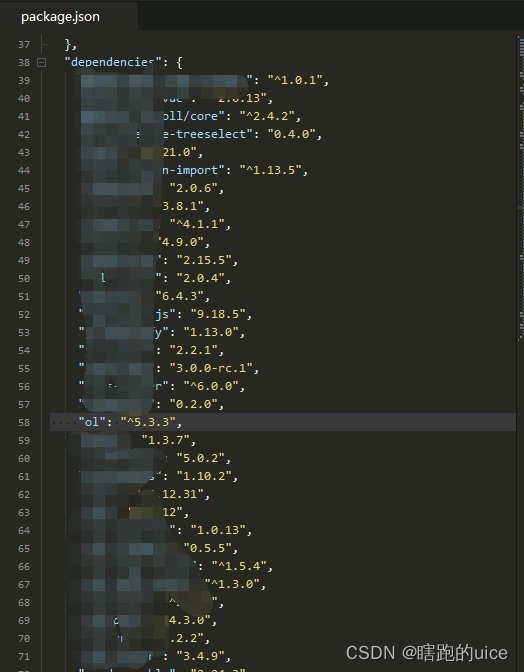
实例组件
<template>
<div style="width: 100%; height: 100%"></div>
</template>
<script>
import markerIcon from "@/assets/images/ship.png";
import Image from "ol/layer/Image";
import ImageWMS from "ol/source/ImageWMS";
import VectorLayer from "ol/layer/Vector";
import VectorSource from "ol/source/Vector";
import Feature from "ol/Feature";
import {
Draw
} from "ol/interaction";
import {
Style,
Fill,
Stroke,
Circle,
Icon,
Text,
} from "ol/style";
import {
transform,
fromLonLat,
toLonLat
} from "ol/proj";
import {
Point,
LineString
} from "ol/geom";
import {
Map,
View,
interaction,
events
} from "ol";
import TileLayer from "ol/layer/Tile";
import {
defaults
} from "ol/control";
import XYZ from "ol/source/XYZ";
var all_draw_feature = [];
const zoom = 14;
import {
duToGpsDM
} from '@/utils/gpsToDu';
export default {
props: {
coordinates: {
type: Array,
default: () =>[{
lat: 119.55648,
lng: 32.190185,
time: "2022/06/22 11:06:03",
x0a9: 1,
x0aA: 300,
},
{
lat: 119.54548,
lng: 32.199185,
time: "2022/06/22 11:06:03",
x0a9: 1,
x0aA: 270,
},
{
lat: 119.53448,
lng: 32.200185,
time: "2022/06/22 11:06:03",
x0a9: 1,
x0aA: 300,
},
{
lat: 119.52348,
lng: 32.209185,
time: "2022/06/22 11:06:03",
x0a9: 1,
x0aA: 270,
}]
},
jumpRouteIndex: {
type: Number,
default: 0,
},
timerDuration: {
type: Number,
default: 500,
},
},
watch: {
coordinates(newValue) {
this.$nextTick(() => {
if (this.map != null) {
let lat =
(this.coordinates[0].lat +
this.coordinates[this.coordinates.length - 1].lat) /
2;
let lng =
(this.coordinates[0].lng +
this.coordinates[this.coordinates.length - 1].lng) /
2;
this.map.getView().setCenter([lat, lng]);
this.map.getView().setZoom(zoom);
this.deleteFeatures();
this.iniDrawLine();
this.drawLine();
this.moveStart();
}
});
},
routeIndex(newValue) {
this.$emit("onRouterIndexChange", newValue)
let rotation = Number(this.carPoints[newValue].x0aA) * (Math.PI / 180);
rotation = isNaN(rotation) ? 0 : rotation;
this.featureMove
.getGeometry()
.setCoordinates([this.carPoints[newValue].lat, this.carPoints[newValue].lng]);
this.featureMove.getStyle()[0]
.getImage()
.setRotation(rotation);
this.featureMove.getStyle()[0]
.getText()
.setText(
`\n 纬度:${duToGpsDM(this.carPoints[newValue].lng,'N')} \n 经度:${duToGpsDM(this.carPoints[newValue].lat,'E')} \n 航向:${this.carPoints[newValue].x0aA}度 \n 航速:${this.carPoints[newValue].x0a9}kn(节) \n 时间:${this.carPoints[newValue].time} \n`
);
},
jumpRouteIndex(newValue) {
if (this.routeIndex != newValue) {
this.routeIndex = newValue
}
},
timerDuration(newValue) {
this.$nextTick(() => {
this.timePause()
this.timeStart()
})
}
},
data() {
return {
map: null,
featureMove: {},
carPoints: [],
routeIndex: 0,
timer: null,
tiandituUrl: "http://t0.tianditu.gov.cn/",
hangdaotuUrl: "http://36.156.155.131:8090/",
routeLayer: {},
};
},
mounted() {
this.initMap();
this.iniDrawLine();
this.drawLine();
this.open();
},
methods: {
initMap() {
let lat =
(this.coordinates[0].lat +
this.coordinates[this.coordinates.length - 1].lat) /
2;
let lng =
(this.coordinates[0].lng +
this.coordinates[this.coordinates.length - 1].lng) /
2;
this.map = new Map({
target: this.$el,
controls: defaults({
zoom: false,
}),
layers: [
new TileLayer({
source: new XYZ({
url: `${this.tiandituUrl}DataServer?T=vec_w&x={x}&y={y}&l={z}&tk=317e52a409b6b382957e09003ee7e235`,
}),
}),
new TileLayer({
source: new XYZ({
url: `${this.tiandituUrl}DataServer?T=cva_w&x={x}&y={y}&l={z}&tk=317e52a409b6b382957e09003ee7e235`,
}),
}),
new Image({
source: new ImageWMS({
url: `${this.hangdaotuUrl}geoserver/CJ/wms`,
params: {
SERVICE: "WMS",
VERSION: "1.1.1",
REQUEST: "GetMap",
FORMAT: "image/png",
TRANSPARENT: true,
tiled: true,
STYLES: "",
LAYERS: "CJ:G_CJ_NJ",
CJAUTH: "CJUSER",
WIDTH: 256,
HEIGHT: 256,
SRS: "EPSG:3857",
},
}),
}),
],
view: new View({
projection: "EPSG:4326",
center: [lat, lng],
zoom: zoom,
}),
});
},
async open() {
this.moveStart();
},
iniDrawLine() {
this.routeLayer = new VectorLayer({
source: new VectorSource({
features: [],
}),
});
this.map.addLayer(this.routeLayer);
},
drawLine() {
let comDots = [];
let wireFeature = {};
this.coordinates.map((item) => {
comDots.push([item.lat, item.lng]);
wireFeature = new Feature({
geometry: new LineString(comDots),
});
wireFeature.setStyle(
new Style({
stroke: new Stroke({
color: "#FF00FF",
width: 4,
}),
})
);
all_draw_feature.push(wireFeature);
let iconFeature = new Feature({
geometry: new Point(
[item.lat, item.lng]
),
});
let rotation = (Number(item.x0aA) - 90) * (Math.PI / 180);
rotation = isNaN(rotation) ? 0 : rotation;
iconFeature.setStyle(
new Style({
image: new Icon({
scale: 0.5,
rotation: rotation,
snapToPixel: true,
src: 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAABAAAAAQCAYAAAAf8/9hAAAAnElEQVQ4T63TsQ0CMQyF4f/NgMQQ0CBR0FIx190cFIiWhhFoKdgEiRUeSoF0gO8cjkub+Evs2OLPpc9422dgBRwlNZn/BtjeApdOUJsh0QuuwKYWiYAFcAKWHaSR1EbpfAHlkO0ICdMJgV+QXmAAmUu6v9IZA8wkPVKgtg7TF7H25t4UbN+A9ahGmqqVD8AO2GdzUF45+I3ZJJb9JxbwRhEhB66xAAAAAElFTkSuQmCC'
}),
text: new Text({
font: '10px Microsoft YaHei',
fill: new Fill({
color: '#000'
}),
textAlign: 'center',
text: ` ${this.parseTime(item.time, "{m}-{d} {h}:{i}:{s}")} `,
offsetX: 0,
offsetY: 15,
rotation: 30 * (Math.PI / 180),
})
})
);
this.routeLayer.getSource().addFeatures([iconFeature]);
});
this.routeLayer.getSource().addFeatures([wireFeature]);
},
moveStart() {
this.dotsData = JSON.parse(JSON.stringify(this.coordinates));
this.carPoints = JSON.parse(JSON.stringify(this.dotsData));
this.featureMove = new Feature({
geometry: new Point([this.carPoints[0].lat, this.carPoints[0].lng]),
});
let rotation = Number(this.carPoints[0].x0aA) * (Math.PI / 180);
rotation = isNaN(rotation) ? 0 : rotation;
this.featureMove.setStyle(
[
new Style({
image: new Icon({
src: markerIcon,
scale: 0.85,
anchor: [0.5, 0.5],
rotation: rotation,
}),
text: new Text({
font: '13px Microsoft YaHei',
fill: new Fill({
color: '#666'
}),
backgroundFill: new Fill({
color: '#fff'
}),
stroke: new Stroke({
color: '#fff',
width: 3
}),
textAlign: 'left',
text: ` 纬度:${duToGpsDM(this.carPoints[0].lng,'N')} \n 经度:${duToGpsDM(this.carPoints[0].lng,'E')} \n 航向:${this.carPoints[0].x0aA}度 \n 航速:${this.carPoints[0].x0a9}kn(节) \n 时间:${this.carPoints[0].time} `,
offsetX: 35,
offsetY: 15
})
}),
]
);
this.routeLayer.getSource().addFeature(this.featureMove);
this.timeStart();
},
timeStart() {
this.timePause()
this.timer = setInterval(() => {
if (this.routeIndex + 1 >= this.carPoints.length) {
this.routeIndex = 0;
return;
}
this.routeIndex++;
}, 1100 - this.timerDuration);
},
timePause() {
clearInterval(this.timer);
},
deleteFeatures() {
this.routeLayer.getSource().clear()
},
},
};
</script>
<style lang="scss" scoped>
</style>