hook 本质是一个函数,把setup函数中使用的 Composition API 进行了封装
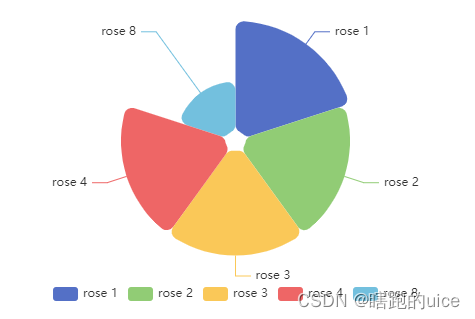
封装echarts组件基底
<template>
<div :style="{ height, width }"></div>
</template>
<script setup>
import * as echarts from 'echarts';
import useResize from '@/hooks/useResize';
const { proxy } = getCurrentInstance();
let echartInstance;
const props = defineProps({
option: {
type: Object,
default: () => ({
xAxis: {
type: 'category',
data: ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']
},
yAxis: {
type: 'value'
},
series: [
{
data: [150, 230, 224, 218, 135, 147, 260],
type: 'line'
}
]
})
},
height: {
type: [Number, String],
default: '300px'
},
width: {
type: [Number, String],
default: '100%'
}
});
const { option, height, width } = toRefs(props);
watch(
option,
(newValue) => {
nextTick(() => {
echartInstance.setOption(
{
...newValue,
backgroundColor: 'rgba(0,0,0,0)'
},
true
);
resize();
});
},
{
deep: true
}
);
watch(height, (newValue) => {
resize();
});
watch(
() => proxy.$store.state.settings.sideTheme.split('-')[1],
(newValue) => {
echartInstance.dispose();
echartInstance = echarts.init(proxy.$el, newValue, { renderer: 'svg' });
echartInstance.setOption(
{
...option.value,
backgroundColor: 'rgba(0,0,0,0)'
},
true
);
}
);
onMounted(() => {
nextTick(() => {
echartInstance = echarts.init(proxy.$el, proxy.$store.state.settings.sideTheme.split('-')[1], { renderer: 'svg' });
echartInstance.setOption(option.value, true);
echartInstance.on('click', (params) => {
console.log(params)
proxy.$emit('clicked', params);
});
});
});
onUnmounted(() => {
echartInstance && echartInstance.dispose();
});
function resize() {
echartInstance.resize();
}
defineExpose({
resize
});
useResize();
</script>
hook (useResize)
export default function () {
let proxy
onMounted(() => {
proxy = getCurrentInstance();
window.addEventListener('resize', resize)
})
onBeforeUnmount(() => {
window.removeEventListener('resize', resize)
})
function resize() {
proxy.exposed.resize()
}
}
二次封装调用
<template>
<Echart :option="option" :height="props.height" @clicked="clicked" />
</template>
<script setup>
import Echart from "@/components/Echarts/index"
const {
proxy
} = getCurrentInstance();
const props = defineProps({
data: {
type: Object,
default: () => ({
max: 3000,
value: 0,
name: "name",
show: false,
unit: "",
radius: "90%"
})
},
title: {
type: Object,
default: () => ({
text: null,
left: 'center'
})
},
legend: {
type: Object,
default: () => ({
left: 'center',
bottom: '10',
})
},
height: {
type: [Number, String],
default: "300px",
},
});
const option = computed(() => ({
title: props.title,
tooltip: {
trigger: 'item',
formatter: '{b} : {c} ({d}%)'
},
legend: props.legend,
series: [{
type: 'pie',
radius: '50%',
data: props.data,
emphasis: {
itemStyle: {
shadowBlur: 10,
shadowOffsetX: 0,
shadowColor: 'rgba(0, 0, 0, 0.5)'
}
}
}]
}));
const handleGetColorByTheme = () => proxy.$store.state.settings.sideTheme.indexOf("dark") != -1 ? "#fff" : "#000"
const clicked = (event)=>{
proxy.$emit('clicked', event);
}
</script>
```
# 最外层调用
```html
<PieChart :data="data" height="100%" @clicked="handlePieChartClicked" />
<script setup>
import PieChart from '@/components/Echarts/pieChart/index';
</script>
```