在Spring Boot中实现文件上传和下载通常涉及到使用Spring MVC的MultipartFile
类来处理文件上传,同时配置一个Controller
来处理上传和下载请求。
详细操作步骤
文件上传步骤:
1.创建上传目录:
在服务器上创建一个目录,用于存储上传的文件。确保这个目录具有足够的权限,以便应用程序可以向其中写入文件。
2.配置文件上传属性:
在application.yml
文件中配置文件上传的属性,包括上传目录的路径、文件大小限制等。
spring:
mvc:
servlet:
multipart:
max-file-size: 20MB # 设置单个文件最大大小为10MB
max-request-size: 200MB # 设置多个文件大小为100MB
也可以在application.properties文件中配置文件上传的属性,包括上传目录的路径、文件大小限制等。
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
3.创建
@Controller
public interface pdfToWord extends ParpentInterface {
@PostMapping(value = "/getpdffile", name = "上传pdf", produces = MediaType.APPLICATION_JSON_UTF8_VALUE)
public PdfToWordRsp getpdffile(HttpServletRequest request,
@RequestParam("file") MultipartFile files) throws InterruptedException, IOException;
@GetMapping(value = "/downloadPaper", name = "下载", produces = MediaType.APPLICATION_JSON_UTF8_VALUE)
public ResponseEntity<byte[]> download(HttpServletRequest request)throws InterruptedException, IOException;
}
4.创建service
@Service
public class PdfToWordImp implements pdfToWord {
//上传文件
@Override
public JSONObject getpdffile(HttpServletRequest request, MultipartFile files) throws InterruptedException, IOException {
PdfToWordRsp pdfToWordRsp = new PdfToWordRsp();
String id= "";
String fileName= "";
String openid= "";
try {
id= request.getParameter("id");
// 构建文件名称
fileName= request.getParameter("name");
openid= request.getParameter("openid");
}catch (Exception e){
e.printStackTrace();
pdfToWordRsp.setCode("2");
pdfToWordRsp.setMessage("文件名称获取失败");
return pdfToWordRsp;
}
// 构建上传目录路径
// request.getServletContext().getRealPath("/upload");
String uploadPath ="/usr/app/appdata/openai";
// 如果目录不存在就创建
File uploadDir = new File(uploadPath);
if (!uploadDir.exists()) {
uploadDir.mkdir();
}
// 获取文件的 名称.扩展名
String oldName = files.getOriginalFilename();
String extensionName = "";
// 获取原来的扩展名
if ((oldName != null) && (oldName.length() > 0)) {
int dot = oldName.lastIndexOf('.');
if ((dot > -1) && (dot < (oldName.length() - 1))) {
extensionName = oldName.substring(dot);
}
}
// 构建文件路径
String filePath = uploadPath +File.separator +openid+ File.separator + fileName;
// 保存文件
try {
FileUtils.writeByteArrayToFile(new File(filePath),
files.getBytes());
return ReturnMessage.success();
} catch (Exception e) {
e.printStackTrace();
throw new GlobalException("上传到服务器失败:" + e.toString());
}
//下载文件
@Override
public ResponseEntity<byte[]> download(HttpServletRequest request) throws InterruptedException, IOException {
//设置响应头
HttpHeaders headers=new HttpHeaders();
String id= "";
String fileName= "";
String openid= "";
try {
id= request.getParameter("id");
// 构建文件名称
fileName= request.getParameter("filename");
openid= request.getParameter("openid");
System.out.println("id="+id);
}catch (Exception e){
e.printStackTrace();
}
QueryWrapper queryWrapper =new QueryWrapper();
queryWrapper.eq("openid",openid);
queryWrapper.eq("id",id);
queryWrapper.eq("isuse","1");
List<PdfPath> list = pdfPathMapper.selectList(queryWrapper);
if (list.size()==0){
headers.setContentDispositionFormData("attachment","文件不存在");
return new ResponseEntity<byte[]>( headers,HttpStatus.OK);
}
PdfPath pdfPath = list.get(0);
String path = pdfPath.getWordpath();
//构建将要下载的文件对象
File file = new File(path);
//下载显示的文件名,解决中文名称乱码问题
String downloadFileName=new String(fileName.getBytes("UTF-8"),"ISO-8859-1");
//通知浏览器以下载方式(attachment)打开文件
headers.setContentDispositionFormData("attachment",downloadFileName);
//定义以二进制流数据(最常见的文件下载)的形式下载返回文件数据
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
//使用spring mvc框架的ResponseEntity对象封装返回下载数据
return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file),headers, HttpStatus.OK);
}
}
5.前端上传代码
var that = this
wx.chooseMessageFile({
type: "file",
count: 1,
// ['.zip', '.docx', '.doc', '.pdf', 'txt', '.csv', '.xlsx', '.ppt', '.pptx']
success: function (res) {
wx.showToast({
title: '等待中...',
icon: 'loading',
duration: 99999999 //持续的时间
})
// console.log("选择的文件是", res);
let temp_path = res.tempFiles[0].path
let name = res.tempFiles[0].name
if (name.split(".")[1] == "pdf") { //我这里只上传pdf文件
wx.uploadFile({
accept: "all",
url: that.data.url + '/getpdffile', // 指定服务器接口URL
filePath: temp_path, // 本地文件路径,即选择文件返回的路径
header: {
//'token': wx.getStorageSync("token"),// 请求需要token就带,不需要就删除
},
//参数绑定
formData: {
name: name,
id: '10000',
openid: openid,
},
name: 'file', // 上传文件的key,后台要用到
success: function (res) { //成功后的回调函数
// console.log(res);
let datarsq = JSON.parse(res.data)
if (datarsq.code == 1) {
wx.showToast({
title: '转换成功!',
icon: 'success',
duration: 2000 //持续的时间
})
let fileTable = {
id: datarsq.fileid,
title: datarsq.fileName,
url: './pdftoworld/pdftoword'
}
that.data.tabitem.push(fileTable);
that.setData({
tabitem: that.data.tabitem,
// message: "",
// toView: `item${this.data.msgList.length+1}`
});
} else {
wx.showToast({
icon: 'none',
title: '上传失败!',
duration: 2000
})
}
}
})
} else {
wx.showToast({
title: '请选择PDF文件',
icon: 'none',
duration: 2000 //持续的时间
})
}
}
})
}
6.前端下载代码
downloadFile: function (e) {
wx.showLoading({
title: '加载中'
})
setTimeout(function () {
wx.hideLoading()
}, 2000)
let id = e.currentTarget.id;
let filename = e.currentTarget.title;
// console.log(id)
const openid = wx.getStorageSync('openid')
if (!openid) {
wx.showModal({
title: '提示',
content: '请先登录',
success: function (res) {
if (res.confirm) { //这里是点击了确定以后
wx.switchTab({
url: "../../my1/my1", //要跳转到的页面路径
})
} else { //这里是点击了取消以后
// console.log('用户点击取消')
}
}
})
}else{
var that = this;
wx.downloadFile({
url: that.data.url + "/downloadPaper?id=" + id + "&openid=" + openid + "&filename=" + filename, //参数可以自己设置
success: function (res) {
// console.log(res)
var wxfilePath = res.tempFilePath;
const fs = wx.getFileSystemManager();
fs.saveFile({
tempFilePath: wxfilePath, //Path我这里是wx.downloadFile()下载下来的文件临时地址
//wx.env.USER_DATA_PATH这个是微信文件的路径 没试过别的 别的路径一般没有权限
filePath: wx.env.USER_DATA_PATH + '/' + Date.parse(new Date()) + '.docx',
success: function (res) {
// console.log(res)
// console.log(wxfilePath)
wx.openDocument({
filePath: res.savedFilePath,
success: function (res) {
//现在再分享给别人的话 就有后缀了
//迷之bug
// console.log('打开成功');
}
})
}
// console.log(filePath);
// wx.openDocument({
// filePath: filePath,
// fileType: 'doc',
// success: function(res) {
// console.log('打开文档成功')
// },
// fail: function(res) {
// console.log(res);
// },
// complete: function(res) {
// console.log(res);
// }
})
},
fail: function (res) {
// console.log('文件下载失败');
},
complete: function (res) {},
})
}
},
最后成品
// console.log(res);
// },
// complete: function(res) {
// console.log(res);
// }
})
},
fail: function (res) {
// console.log(‘文件下载失败’);
},
complete: function (res) {},
})
}
},
## 最后成品
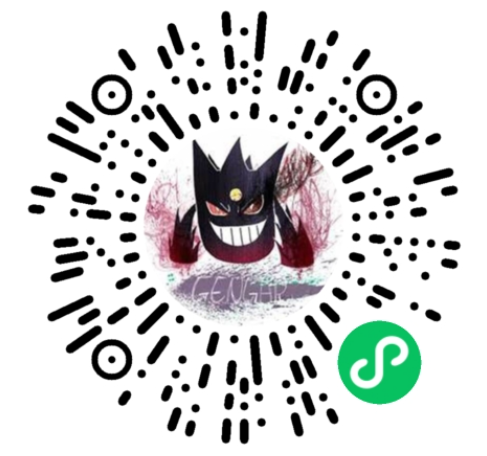