二叉树的前序/中序/后序遍历以及删除实现
概念
1.每个节点最多只有两个子节点的树称之为二叉树
2.二叉树的子节点分为左节点/右节点
3.如果二叉树所有的叶子节点都在最后一层,并且节点总数=2*n-1(n为层数),则称之为满二叉树
4.如果该二叉树的所有叶子节点最后一层或者倒数第二层,而且最后一层叶子节点在左面连续,倒数第二层节点在右面连续,我们称之为完全二叉树
分析
前/中/后序遍历都是针对于父节点来说的
前序遍历:
先输出父节点,再遍历左子树,最后遍历右子树
中序遍历:
先遍历左子树,再输出父节点,最后遍历右子树
后续遍历:
先遍历左子树,再遍历右子树,最后输出父节点
代码实现
/**
*链表结构定义一个树
*/
public class Node {
private int value;
//左数
private Node left;
//右数
private Node right;
@Override
public String toString() {
return "Node{" +
"value=" + value +
'}';
}
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
public Node getLeft() {
return left;
}
public void setLeft(Node left) {
this.left = left;
}
public Node getRight() {
return right;
}
public void setRight(Node right) {
this.right = right;
}
//前序遍历
public void preTree(){
//先输出父节点
System.out.println(this.toString());
//再递归输出左节点
if(this.getLeft()!=null){
this.getLeft().preTree();
}
//再递归输出右节点
if(this.getRight()!=null){
this.getRight().preTree();
}
}
//中序遍历
public void inTree(){
//先递归左节点
if(this.getLeft()!=null){
this.getLeft().inTree();;
}
//再查询父节点
System.out.println(this.toString());
//在查询右节点
if(this.getRight()!=null){
this.getRight().preTree();
}
}
//后序遍历
public void afterTree(){
//先递归右节点
if(this.getRight()!=null){
this.getRight().afterTree();
}
//再递归左节点
if(this.getLeft()!=null){
this.getLeft().afterTree();
}
//最后输出父节点
System.out.println(this.toString());
}
/**
* 删除一个节点(如果所删除节点不是叶子节点,则当前节点下所有节点都会删除)
* */
public void deleteNode(int val){
//查看当前节点左右节点是否是需要删除的数据
if(this.getLeft()!=null&&this.getLeft().getValue()==val){
this.setLeft(null);
return;
}
if(this.getRight()!=null&&this.getRight().getValue()==val){
this.setRight(null);
return;
}
//递归左节点删除
if(this.getLeft()!=null){
this.getLeft().deleteNode(val);
}
//递归右节点删除
if(this.getRight()!=null){
this.getRight().deleteNode(val);
}
}
}
/**
*定义一个二叉树接收类用于遍历
* */
public class BinarTree {
private Node node;
public BinarTree(Node node){
this.node=node;
}
//前序遍历
public void preTree(){
node.preTree();
}
//中序遍历
public void inTree(){
node.inTree();
}
//后序遍历
public void afterTree(){
node.afterTree();
}
//删除节点
public void deleteNode(int val){
//判断是否是父节点左右值,不是的话再进行传递给下一个方法
if(node.getLeft().getValue()==val){
node=null;
return;
}
else if(node.getRight().getValue()==val){
node=null;
}
else{
node.deleteNode(val);
}
}
}
测试遍历
public static void main(String[] args) {
Node node = new Node();
Node node1 = new Node();
Node node2 = new Node();
Node node3 = new Node();
Node node4 = new Node();
node.setValue(0);
node1.setValue(1);
node2.setValue(2);
node3.setValue(3);
node4.setValue(4);
node.setLeft(node1);
node.setRight(node2);
node1.setLeft(node3);
node1.setRight(node4);
BinarTree binarTree = new BinarTree(node);
System.out.println("前序遍历-------------------");
binarTree.preTree();
System.out.println("中序遍历-------------------");
//binarTree.inTree();
System.out.println("后序遍历-------------------");
//binarTree.afterTree();
}```
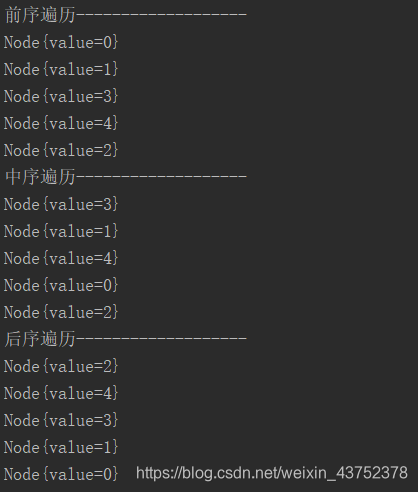
测试删除节点
```java
public static void main(String[] args) {
Node node = new Node();
Node node1 = new Node();
Node node2 = new Node();
Node node3 = new Node();
Node node4 = new Node();
node.setValue(0);
node1.setValue(1);
node2.setValue(2);
node3.setValue(3);
node4.setValue(4);
node.setLeft(node1);
node.setRight(node2);
node1.setLeft(node3);
node1.setRight(node4);
BinarTree binarTree = new BinarTree(node);
System.out.println("前序遍历-------------------");
binarTree.preTree();
/*System.out.println("中序遍历-------------------");
binarTree.inTree();
System.out.println("后序遍历-------------------");
binarTree.afterTree();*/
//删除叶子节点3
binarTree.deleteNode(3);
System.out.println("删除后进行前序遍历-------------------");
binarTree.preTree();
}