演示
继承Image类,重写OnPopulateMesh方法
由于内部点的坐标会根据pivot变化,所以需要进行坐标变换
/// <summary>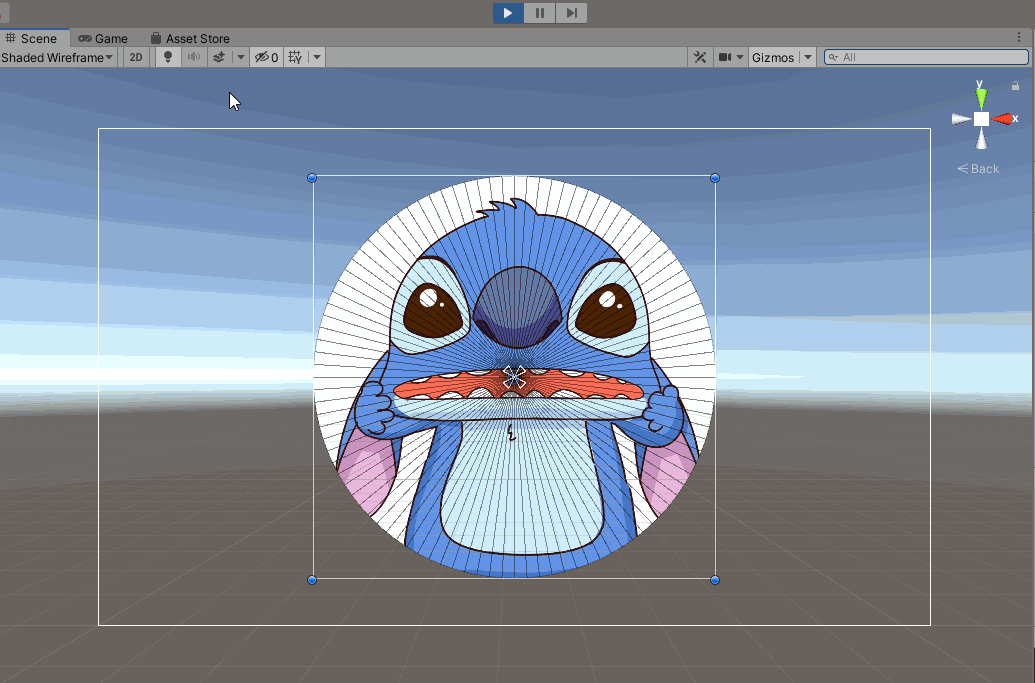
/// 该方法用于生成圆形Mesh
/// </summary>
/// <param name="vh"></param>
protected override void OnPopulateMesh(VertexHelper vh)
{
vh.Clear();
if (sprite == null) return;
innerUV = DataUtility.GetInnerUV(sprite);//在九宫格模式下内部UV,(x,y)为左下角坐标,(z,w)为右上角坐标
outerUV = DataUtility.GetOuterUV(sprite);//整个Sprite的UV,(x,y)为左下角坐标,(z,w)为右上角坐标
float width = rectTransform.rect.width;
float height = rectTransform.rect.height;
float uvWidth = outerUV.z - outerUV.x;
float uvHeight = outerUV.w - outerUV.y;
Vector2 uvCenter = new Vector2(uvWidth / 2, uvHeight / 2);
Vector2 convertToUVPos = new Vector2(uvWidth / width, uvHeight / height);
float radian = 2 * Mathf.PI / segments;
UIVertex center = new UIVertex();//添加中心点
center.color = color;
center.position = new Vector2((.5f - rectTransform.pivot.x) * rectTransform.rect.width, (.5f - rectTransform.pivot.y) * rectTransform.rect.height);//需要根据pivot进行变换,默认圆心为(0,0)
//center.position = rectTransform.rect.size / 2 + rectTransform.rect.position;//方法二:根据Rect进行变换
center.uv0 = uvCenter;
vh.AddVert(center);
float radius = width <= height ? width / 2 : height / 2;
float currentRadian = 0;
for(int i = 0; i < segments + 1; i++)//添加圆弧上的点
{
UIVertex point = new UIVertex();
point.color = color;
Vector2 tempPoint = new Vector2(radius * Mathf.Cos(currentRadian), radius * Mathf.Sin(currentRadian));
point.uv0 = new Vector2(tempPoint.x * convertToUVPos.x + uvCenter.x, tempPoint.y * convertToUVPos.y + uvCenter.y);
point.position = (Vector2)center.position + tempPoint;// 需要根据圆心位置进行变换
vh.AddVert(point);
currentRadian += radian;
}
for (int i = 0; i < segments; i++)//根据所添加点添加三角面,顺时针旋转顺序为正向
{
vh.AddTriangle(0, i+2, i+1);
}
}
重写IsRaycastLocationValid方法
/// <summary>
/// 重写该方法用于定义可点击区域
/// </summary>
/// <param name="screenPoint"></param>
/// <param name="eventCamera"></param>
/// <returns></returns>
public override bool IsRaycastLocationValid(Vector2 screenPoint, Camera eventCamera)
{
Vector2 localPoint;
RectTransformUtility.ScreenPointToLocalPointInRectangle(rectTransform, screenPoint, eventCamera, out localPoint);
localPoint =localPoint - new Vector2( (.5f -rectTransform.pivot.x )* rectTransform.rect.width, (.5f-rectTransform.pivot.y ) * rectTransform.rect.height);//根据pivot变换坐标使圆心为(0,0),以方便判断是否点击在圆内
return localPoint.magnitude < rectTransform.rect.width/2 && localPoint.magnitude < rectTransform.rect.height/2;
}