threejs项目篇 之 实现酷炫机器人展示
安装
App.vue
<template>
<div class="canvas-container" ref="canvasDom"></div>
</template>
<script setup>
import { ref, onMounted } from 'vue'
import * as THREE from "three"
import { OrbitControls } from "three/examples/jsm/controls/OrbitControls"
import { RGBELoader } from "three/examples/jsm/loaders/RGBELoader";
import { GLTFLoader } from "three/examples/jsm/loaders/GLTFLoader";
import { DRACOLoader } from "three/examples/jsm/loaders/DRACOLoader";
import { Reflector } from "three/examples/jsm/objects/Reflector";
import gsap from "gsap";
let canvasDom = ref(null)
onMounted(()=>{
const scene = new THREE.Scene()
const camera = new THREE.PerspectiveCamera(75,window.innerWidth / window.innerHeight,0.1,1000)
camera.position.set(0, 1.5, 6)
camera.updateProjectionMatrix()
scene.add( camera );
const renderer = new THREE.WebGL1Renderer({
antialias:true,
})
renderer.setSize(canvasDom.value.clientWidth, canvasDom.value.clientHeight)
canvasDom.value.appendChild(renderer.domElement)
renderer.outputEncoding = THREE.sRGBEncoding;
const gridHelper = new THREE.GridHelper(5)
scene.add(gridHelper)
let controls = new OrbitControls(camera,renderer.domElement)
controls.enableDamping = true
controls.update()
let hdrLoader = new RGBELoader();
hdrLoader.load("./assets/sky12.hdr", (texture) => {
texture.mapping = THREE.EquirectangularReflectionMapping;
scene.background = texture;
scene.environment = texture;
});
let dracoLoader = new DRACOLoader();
dracoLoader.setDecoderPath("./draco/gltf/");
dracoLoader.setDecoderConfig({ type: "js" });
let gltfLoader = new GLTFLoader();
gltfLoader.setDRACOLoader(dracoLoader);
gltfLoader.load("./assets/robot.glb", (gltf) => {
scene.add(gltf.scene);
});
let light1 = new THREE.DirectionalLight(0xffffff, 0.3);
light1.position.set(0, 10, 10);
let light2 = new THREE.DirectionalLight(0xffffff, 0.3);
light1.position.set(0, 10, -10);
let light3 = new THREE.DirectionalLight(0xffffff, 0.8);
light1.position.set(10, 10, 10);
scene.add(light1, light2, light3);
let video = document.createElement("video");
video.src = "./assets/zp2.mp4";
video.loop = true;
video.muted = true;
video.play();
let videoTexture = new THREE.VideoTexture(video);
const videoGeoPlane = new THREE.PlaneBufferGeometry(8, 4.5);
const videoMaterial = new THREE.MeshBasicMaterial({
map: videoTexture,
transparent: true,
side: THREE.DoubleSide,
alphaMap: videoTexture,
});
const videoMesh = new THREE.Mesh(videoGeoPlane, videoMaterial);
videoMesh.position.set(0, 0.2, 0);
videoMesh.rotation.set(-Math.PI / 2, 0, 0);
scene.add(videoMesh);
let reflectorGeometry = new THREE.PlaneBufferGeometry(100, 100);
let reflectorPlane = new Reflector(reflectorGeometry, {
textureWidth: window.innerWidth,
textureHeight: window.innerHeight,
color: 0x332222,
});
reflectorPlane.rotation.x = -Math.PI / 2;
scene.add(reflectorPlane);
function render() {
requestAnimationFrame( render );
renderer.render( scene, camera );
controls && controls.update()
}
render()
window.addEventListener("resize", () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.setPixelRatio(window.devicePixelRatio);
});
})
</script>
<style lang="scss" scoped>
* {
padding:0;
margin: 0;
}
.canvas-container {
width: 100vh;
height: 100vh;
}
</style>
效果
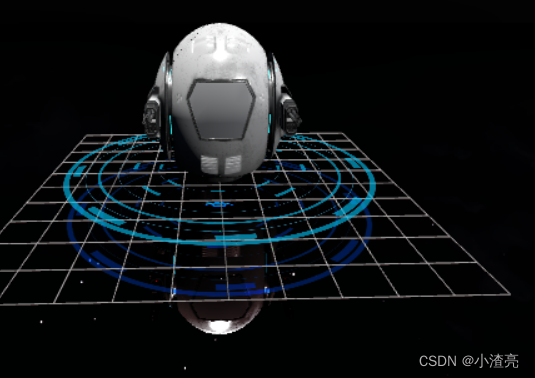