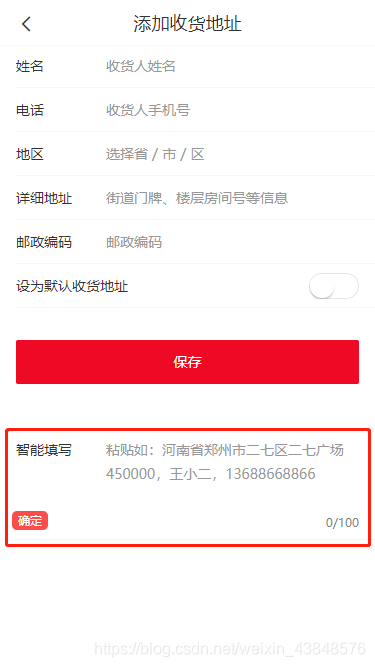
安装
npm install vue-smart-parse -d -s
import smartParse from 'vue-smart-parse';
Vue.use(smartParse)
使用
methods: {
text() {
console.log(this.smartParse(this.message));
this.textAdd = this.smartParse(this.message);
this.$set(this.info, "name", this.textAdd.name);
this.$set(this.info, "tel", this.textAdd.phone);
this.$set(this.info, "addressDetail", this.textAdd.address);
this.$set(this.info, "postalCode", this.textAdd.zipCode);
this.$set(this.info, "city", this.textAdd.city);
this.$set(this.info, "county", this.textAdd.county);
this.$set(this.info, "isDefault", true);
this.$set(this.info, "areaCode", this.textAdd.countyCode);
this.$set(this.info, "province", this.textAdd.province);
console.log(this.info);
},
}
{
zipCode:710061
province:陕西省
provinceCode:61
city:西安市
cityCode:6101
county:雁塔区
countyCode:610113
street:丈八沟街道
streetCode:610113007
address:高新四路高新大都荟
name:刘国良
phone:13593464918
idCard:211381198512096810
}
整个页面代码
<template>
<div class="add">
<tabbar title="添加收货地址"></tabbar>
<van-address-edit
:area-list="areaList"
:address-info="info"
show-postal
show-set-default
show-search-result
:search-result="searchResult"
@save="onSave"
style="margin-top:44px;"
/>
<van-cell-group>
<van-field
v-model="message"
rows="3"
autosize
label="智能填写"
type="textarea"
maxlength="100"
placeholder="粘贴如:河南省郑州市二七区二七广场450000,王小二,13688668866"
show-word-limit
/>
<button @click="text" class="btn">
确定
</button>
</van-cell-group>
</div>
</template>
<script>
import tabbar from "../../components/navbar";
import areaList from "../../common/js/newArea";
export default {
components: {
tabbar
},
data() {
return {
areaList,
searchResult: [],
message: "",
textAdd: {},
info: {}
};
},
mounted() {},
methods: {
text() {
console.log(this.smartParse(this.message));
this.textAdd = this.smartParse(this.message);
this.$set(this.info, "name", this.textAdd.name);
this.$set(this.info, "tel", this.textAdd.phone);
this.$set(this.info, "addressDetail", this.textAdd.address);
this.$set(this.info, "postalCode", this.textAdd.zipCode);
this.$set(this.info, "city", this.textAdd.city);
this.$set(this.info, "county", this.textAdd.county);
this.$set(this.info, "isDefault", true);
this.$set(this.info, "areaCode", this.textAdd.countyCode);
this.$set(this.info, "province", this.textAdd.province);
console.log(this.info);
},
onSave(con) {
console.log(con);
this.$toast("save");
this.axios
.post("/api/Address/add", {
name: con.name,
phone: con.tel,
mail: con.postalCode,
areas_id: con.areaCode,
details: con.addressDetail,
type: 1,
status: con.isDefault ? 1 : 0
})
.then(data => {
this.$toast("添加成功");
setTimeout(() => {
this.$router.push("/address");
}, 1000);
});
}
}
};
</script>
<style lang="less" scoped>
.add {
.van-button::before {
background: #f0f;
}
.van-cell-group {
position: relative;
}
.btn {
color: #fff;
padding: 0 6px 1px;
background: #fc4c4c;
border-radius: 5px;
position: absolute;
bottom: 10px;
left: 12px;
}
}
</style>