仅修改标题栏的背景色和字体颜色
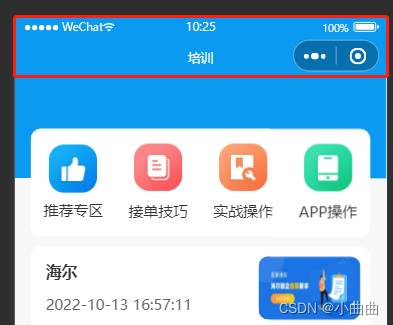
"navigationBarTitleText": "培训",
"navigationBarBackgroundColor": "#0a9af1",
"navigationBarTextStyle": "white"
- 方法二:可动态设置 ,直接在onLoad中写就ok,(基础库1.4.0才支持 ):
wx.setNavigationBarColor({
frontColor: '#ffffff',
backgroundColor: '#000000',
})
单个页面自定义头部——控制返回按钮
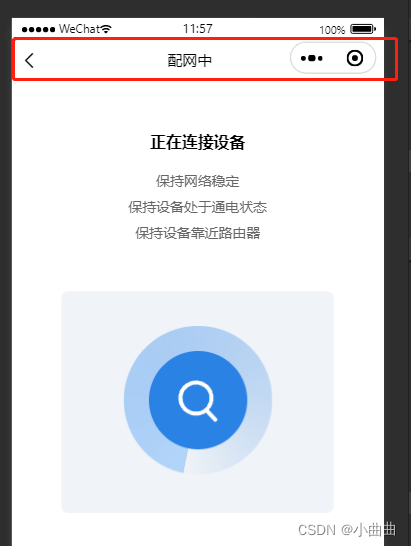
{
"navigationStyle": "custom"
}
<!-- 状态栏高度 -->
<view style="height: {{statusBarHeight}}px"></view>
<!-- 标题栏高度 -->
<view class='nav' style="height: {{toBarHeight}}px;">
<van-icon class="image" name="arrow-left" bindtap="backClick"/>
<text style="height: {{toBarHeight}}px;line-height: {{toBarHeight}}px">配网中
</text>
</view>
.box {
background: #FFF;
font-weight: 500;
width: 100%;
position: fixed;
top: 0;
right: 0;
z-index: 999;
}
.nav {
width: 100%;
font-size: 34rpx;
}
.nav .image {
font-size: 40rpx;
font-weight: bold;
margin-top: 26rpx;
float: left;
margin-left: 15rpx;
}
.nav text {
float: left;
margin-left: 30rpx;
}
onLoad(options) {
let sysinfo = wx.getSystemInfoSync();
this.setData({
statusBarHeight: sysinfo.statusBarHeight
})
},
backClick(){
wx.showModal({
title: '设备正在添加,是否退出?',
content: '设备可能正在添加,确认退出?若确认退出,请在下次添加设备时按照流程重置设备。',
confirmColor: '#217EF7',
confirmText:'继续添加',
cancelText:'仍然退出',
success(res) {
if (res.confirm) {} else if (res.cancel) {
wx.navigateBack()
}
}
})
},
完全自定义头部
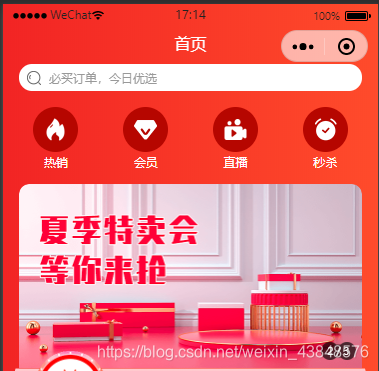
在app.js中动态获取顶部高度
import { wxp, http } from './utils/index'
import { store } from './store/index'
import log from './utils/log'
App({
onLaunch(options) {
let systemInfo = wx.getSystemInfoSync()
let rect = wx.getMenuButtonBoundingClientRect()
let gap
let barHeight
if (rect) {
gap = rect.top - systemInfo.statusBarHeight
barHeight = 2 * gap + rect.height
} else {
if (systemInfo.platform === 'android') {
barHeight = 48
} else {
barHeight = 40
}
}
store.setNavHeight({
statusHeight: systemInfo.statusBarHeight,
barHeight: barHeight,
})
http.get('/common/mini_set').then(({ data }) => {
store.setConfig(data)
})
if (!options.path.includes('login')) {
wxp.login().then(async ({ code }) => {
try {
const { data } = await http.post('/auth/login', {
code,
source: 2,
})
store.setUserInfo(data.userInfo)
store.setMemberInfo(data.memberInfo)
wx.setStorageSync('token', data.userInfo.token)
} catch (error) {}
})
}
},
onError(error) {
log.error(error)
},
})
全局组件navbar
<config>
{
"component":true,
"usingComponents": {
"van-sticky": "@vant/weapp/sticky/index"
}
}
</config>
<template lang="wxml">
<van-sticky>
<view class="navbar-box w100" style="height:{{navHeight.statusHeight + navHeight.barHeight }}px;background:{{bg}};">
<view class="navbar w100 fixed" style="height:{{navHeight.statusHeight + navHeight.barHeight}}px;padding-top:{{navHeight.statusHeight}}px">
<view class="title white size-32" style="line-height: {{navHeight.barHeight}}px;">{{title}}</view>
</view>
</view>
</van-sticky>
</template>
<script>
import { store } from '../store/index'
Component({
options: {
addGlobalClass: true,
},
properties: {
title: String,
bg: String,
},
data: {
navHeight: {},
},
lifetimes: {
attached() {
this.setData({
navHeight: store.navHeight,
})
},
},
methods: {},
})
</script>
<style>
.navbar {
top: 0rpx;
left: 0rpx;
right: 0rpx;
}
.title {
position: absolute;
left: 50%;
transform: translateX(-50%);
}
</style>
在首页中使用
"usingComponents": {
"navbar":"../components/navbar"
},
"navigationStyle":"custom"
<!-- navbar -->
<navbar title="首页" bg="{{home_diy[theme-1].bg}}"></navbar>
import { observable, action } from 'mobx-miniprogram'
import { http } from '../utils/index'
export const store = observable({
userInfo: wx.getStorageSync('userInfo') || {},
memberInfo: wx.getStorageSync('memberInfo') || {},
addressList: wx.getStorageSync('addressList') || [],
config: {},
navHeight: {},
get isvip() {
return Boolean(this.memberInfo.status && this.memberInfo.status == 1)
},
get isagent() {
return Boolean(this.userInfo.agent == 1)
},
get iszhubo() {
return this.userInfo.anchor_status
},
updateIszhubo: action(function (status) {
this.userInfo.anchor_status = status
}),
fetchUserInfo: action(function () {
return http.get('/common/userInfo').then(({ data }) => {
this.setUserInfo(data.userInfo)
this.setMemberInfo(data.memberInfo)
})
}),
setUserInfo: action(function (userInfo) {
let obj = Object.assign({}, this.userInfo, userInfo)
this.userInfo = obj
wx.setStorage({
key: 'userInfo',
data: obj,
})
}),
setMemberInfo: action(function (memberInfo) {
let obj = Object.assign({}, this.memberInfo, memberInfo)
this.memberInfo = obj
wx.setStorage({
key: 'memberInfo',
data: obj,
})
}),
fetchAddressList: action(function () {
return http.get('/common/address_index').then(({ data }) => {
this.addressList = data
wx.setStorage({
key: 'addressList',
data: data,
})
})
}),
setConfig: action(function (config) {
this.config = config
wx.setStorage({
key: 'config',
data: config,
})
}),
setNavHeight: action(function (height) {
this.navHeight = height
wx.setStorage({
key: 'navHeight',
data: height,
})
}),
})