高德地图上显示车辆及车辆信息,车辆需要旋转角度
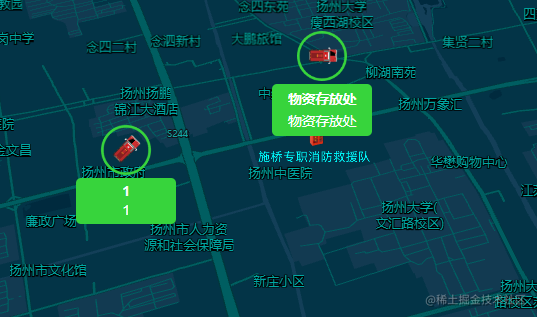
- 错误思路1:添加点标记,加上旋转角度属性,结果图标和字体一起旋转
- 错误思路2:添加一个点标记,在添加一个纯文本标记,结果图标和字体位置无法对齐,尤其是图标旋转之后位置会变化,无法以中心点旋转
解决方案—— 高德的自定义点标记内容
getHydraulicCarListApi({}).then((res) => {
res.data.forEach((item, i) => {
var markerContent =
'<div class="custom-content-marker">' +
'<img style="transform:rotate(' +
i * 45 +
'deg);width:50px;height:50px" src=" ' +
carIcon +
'">' +
'<div class="car" style="color:#fff;text-align:center;background:#37D43C;padding:6px 0;border-radius:5px;width:100px;margin-left:-25px"><div style="font-weight:700;margin-bottom:4px">' +
item.resourcesName +
"</div><div>" +
item.resourcesName +
"</div></div>" +
"</div>";
var marker = new AMap.Marker({
position: new AMap.LngLat(item.longitude, item.latitude),
content: markerContent,
offset: new AMap.Pixel(-13, -30),
});
this.map.add(marker);
});
});
路径规划
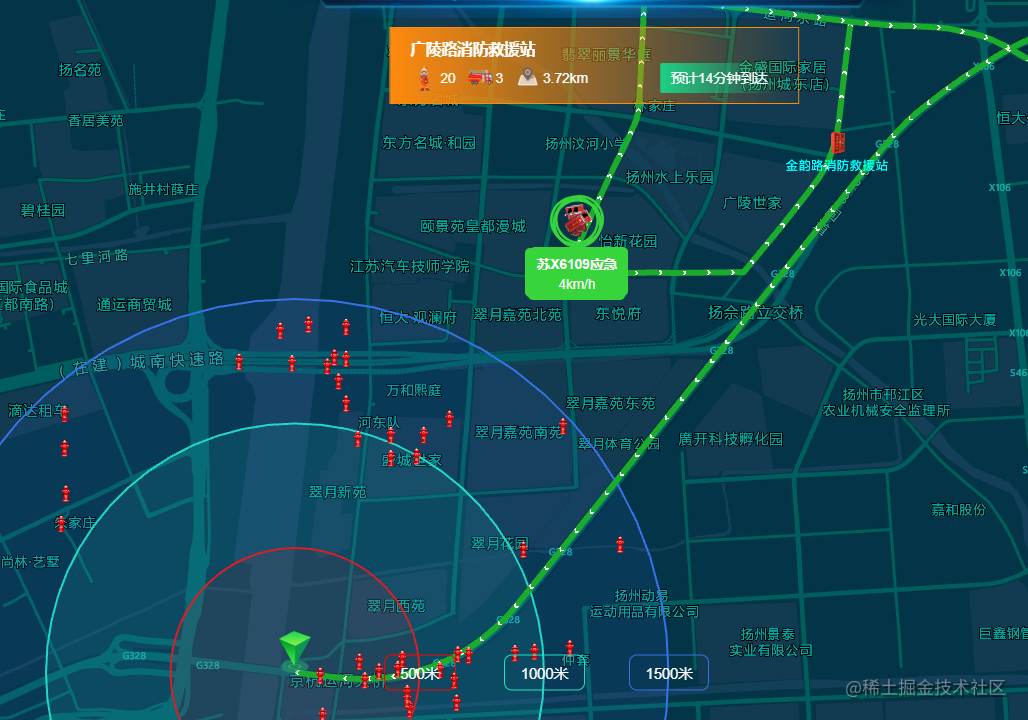
AMap.plugin("AMap.Driving", () => {
this.carLine[i] = new AMap.Driving({
policy: 0,
map: this.map,
hideMarkers: true,
isOutline: false,
autoFitView: false,
});
this.carLine[i].search(
new AMap.LngLat(
item.carLocation.longitude,
item.carLocation.latitude
),
new AMap.LngLat(...this.position),
(status, result) => {
if (status === "complete") {
} else {
console.log("获取驾车数据失败:" + result);
}
}
);
});
鼠标移入车辆显示弹窗
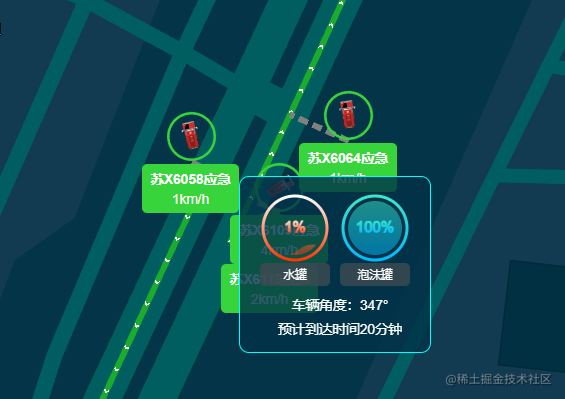
<!-- 警情车辆信息弹窗 -->
<div
class="carInfo white"
:style="`left:${left}px;top:${top}px`"
v-if="carInfoVisible"
>
<!-- <div @click="carInfoVisible = false" class="flex_r">
<i class="el-icon-circle-close sizeRem-24"></i>
</div> -->
<div class="center mb-10">
<div
v-for="(item, index) in carInfo.carLiquidLevelList"
:key="index"
class="mr-10"
>
<dv-water-level-pond
:config="item.config"
style="width: 70px; height: 70px"
/>
<div
class="ptb-4 text-center radius-5 sizeRem-12"
style="background: rgba(62, 75, 81, 0.8)"
>
{{ item.type == 0 ? "水罐" : "泡沫罐" }}
</div>
</div>
</div>
<div class="text-center">
车辆角度:{{ carInfo.carLocation.direction }}°
</div>
<div class="text-center mt-6">
预计到达时间{{ parseInt(carInfo.carLocation.estimatedTime / 60) }}分钟
</div>
</div>
this.carMarker[i].on("mouseover", (e) => {
this.left = e.pixel.x;
this.top = e.pixel.y;
this.carInfoVisible = true;
this.goodsInfoVisible = false;
this.carInfo = item;
if (this.carInfo.carLiquidLevelList) {
this.carInfo.carLiquidLevelList.forEach((el, index) => {
this.$set(el, "config", {
data: [
parseInt((el.currentLevel / el.maxLevel) * 100) > 100
? 100
: parseInt((el.currentLevel / el.maxLevel) * 100),
],
waveNum: 1,
waveHeight: 10,
waveOpacity: 0.5,
shape: "round",
colors:
parseInt((el.currentLevel / el.maxLevel) * 100) < 50
? ["#fff", "#ff3300"]
: [],
});
});
}
});
this.carMarker[i].on("mouseout", (e) => {
this.left = 0;
this.top = 0;
this.carInfoVisible = false;
this.goodsInfoVisible = false;
this.carInfo = {};
});
完整代码
- 拿到socket推的车辆数据
- 调取创建车辆的方法
- 先清除车辆的点和路径规划的线
- 显示车辆位置以及角度
- 显示路径规划
- 定义鼠标移入移出方法
createCar(catArr) {
this.carMarker.map((item, i) => {
this.map.remove(item);
});
this.carLine.map((item, i) => {
item.clear();
});
this.carMarker = [];
this.carLine = [];
catArr.forEach((item, i) => {
var markerContent =
'<div class="custom-content-marker" >' +
'<img style="transform:rotate(' +
item.carLocation.direction +
'deg);width:.625rem;height:.625rem" src=" ' +
carIcon +
'">' +
'<div class="car" style="color:#fff;text-align:center;background:#37D43C;padding:6px 0;border-radius:5px;width:1.25rem;margin-left:-25px"><div style="font-weight:700;margin-bottom:4px">' +
item.carLocation.carNum +
"</div><div>" +
item.carLocation.speed +
"km/h" +
"</div></div>" +
"</div>";
this.carMarker[i] = new AMap.Marker({
position: new AMap.LngLat(
item.carLocation.longitude,
item.carLocation.latitude
),
content: markerContent,
offset: new AMap.Pixel(-25, -50),
});
this.map.add(this.carMarker[i]);
AMap.plugin("AMap.Driving", () => {
this.carLine[i] = new AMap.Driving({
policy: 0,
map: this.map,
hideMarkers: true,
isOutline: false,
autoFitView: false,
});
this.carLine[i].search(
new AMap.LngLat(
item.carLocation.longitude,
item.carLocation.latitude
),
new AMap.LngLat(...this.position),
(status, result) => {
if (status === "complete") {
} else {
console.log("获取驾车数据失败:" + result);
}
}
);
});
this.carMarker[i].on("mouseover", (e) => {
this.left = e.pixel.x;
this.top = e.pixel.y;
this.carInfoVisible = true;
this.goodsInfoVisible = false;
this.carInfo = item;
if (this.carInfo.carLiquidLevelList) {
this.carInfo.carLiquidLevelList.forEach((el, index) => {
this.$set(el, "config", {
data: [
parseInt((el.currentLevel / el.maxLevel) * 100) > 100
? 100
: parseInt((el.currentLevel / el.maxLevel) * 100),
],
waveNum: 1,
waveHeight: 10,
waveOpacity: 0.5,
shape: "round",
colors:
parseInt((el.currentLevel / el.maxLevel) * 100) < 50
? ["#fff", "#ff3300"]
: [],
});
});
}
});
this.carMarker[i].on("mouseout", (e) => {
this.left = 0;
this.top = 0;
this.carInfoVisible = false;
this.goodsInfoVisible = false;
this.carInfo = {};
});
});
},