OpenGL绘制一个简易的电子时钟:
效果图:
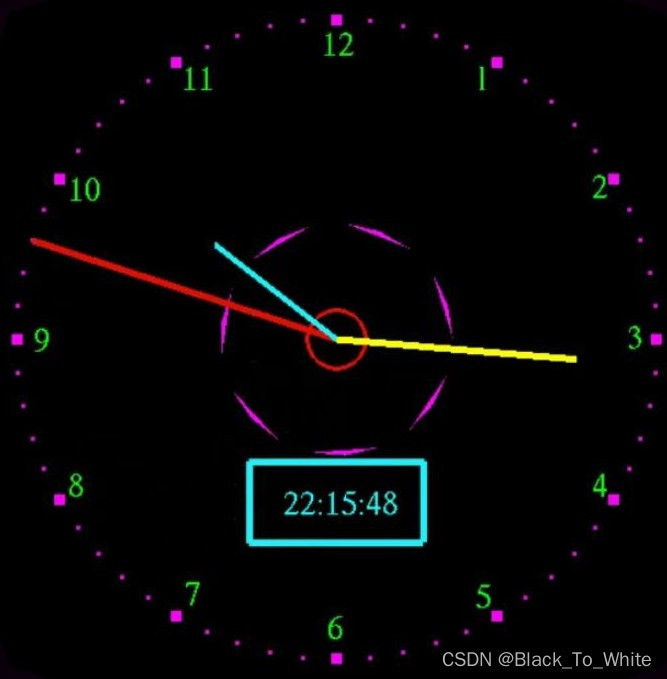
#include "framework.h"
#include "clock.h"
#include <windows.h>
#include <glut.h>
#include <math.h>
#define PI 3.1415926 //设置圆周率
int R = 11; //外接圆半径
SYSTEMTIME timeNow;
float hh, mm, ss, angle;
float xc, yc, xs, ys, xm, ym, xh, yh;
int h, s, m;
void init();
float theta = 0.0; //旋转初始角度值
void Keyboard(unsigned char key, int x, int y);
void Display(void);
void Display_2(void);
void Reshape(int w, int h);
//void myidle();
void mytime(int t);
int APIENTRY _tWinMain(HINSTANCE hInstance,
HINSTANCE hPrevInstance,
LPTSTR lpCmdLine,
int nCmdShow)
{
UNREFERENCED_PARAMETER(hPrevInstance);
UNREFERENCED_PARAMETER(lpCmdLine);
char *argv[] = { "My_Clock", " " };
int argc = 2; // must/should match the number of strings in argv
glutInit(&argc, argv); //初始化GLUT库;
glutInitWindowSize(700, 700); //设置显示窗口大小
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB); //设置显示模式;(注意双缓冲)
glutCreateWindow("Clock_2"); // 创建显示窗口
init();
glutDisplayFunc(Display); //注册显示回调函数
glutReshapeFunc(Reshape); //注册窗口改变回调函数
// glutIdleFunc(myidle); //注册闲置回调函数
glutTimerFunc(0, mytime, 10); //1000毫秒后调用时间函数 mytime
glutMainLoop(); //进入事件处理循环
return 0;
}
void init()//获取系统时间
{
GetLocalTime(&timeNow);//获取系统时间
hh = timeNow.wHour;//获取小时时间
mm = timeNow.wMinute;//获取分钟时间
ss = timeNow.wSecond;//获取秒时间
}
void Display(void)
{
glClear(GL_COLOR_BUFFER_BIT);//清屏
//绘制表盘
glMatrixMode(GL_MODELVIEW);//模型视图矩阵
glLoadIdentity();//用一个4×4的单位矩阵来替换当前矩阵,对当前矩阵进行初始化
glPointSize(3);//循环绘制多个三角形,数值需要不断更改、模拟,以达到拉长扁平效果
glBegin(GL_TRIANGLES);
for (int i = 0; i < 21; i++) {
angle = 2 * PI * i / 21;
glVertex2f(4 * cos(angle), 4 * sin(angle));
}
glEnd();
glLineWidth(3);
glColor3f(1, 0, 0);
glBegin(GL_LINE_LOOP);
for (int i = 0; i < 100; i++)
{
glVertex2f(1 * cos(2 * PI / 100 * i), 1 * sin(2 * PI / 100 * i));
}
glEnd();
//时间刻度
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glPointSize(3);
glColor3f(1, 0, 1);
glBegin(GL_POINTS);
for (int i = 0; i < 60; i++) {
angle = 2 * PI * i / 60;
glVertex2f(11 * cos(angle), 11 * sin(angle));
}
glEnd();
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glPointSize(8);
glColor3f(1, 0, 1);
glBegin(GL_POINTS);
for (int i = 0; i < 12; i++) {
angle = 2 * PI * i / 12;
glVertex2f(11 * cos(angle), 11 * sin(angle));
}
glEnd();
glColor3f(0, 1, 0);
glRasterPos2f(-0.5, 9.8);//定位当前光标,起始字符位置
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '1');
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '2');
glRasterPos2f(4.9, 8.6);//定位当前光标,起始字符位置
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, 'l');
glRasterPos2f(8.8, 4.9);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '2');
glRasterPos2f(10, -0.3);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '3');
glRasterPos2f(8.8, -5.4);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '4');
glRasterPos2f(4.8, -9.2);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '5');
glRasterPos2f(-0.3, -10.3);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '6');
glRasterPos2f(-5.2, -9.1);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '7');
glRasterPos2f(-9.2, -5.4);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '8');
glRasterPos2f(-10.4, -0.4);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '9');
glRasterPos2f(-9.2, 4.8);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '1');
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '0');
glRasterPos2f(-5.3, 8.6);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '1');
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, '1');
//xc,yc为时针中心点坐标
//xs,ys, 为秒针终止点坐标
xs = xc + R * cos(PI / 2.0 - ss / 60 * 2 * PI);
ys = yc + R * sin(PI / 2.0 - ss / 60 * 2 * PI);
xm = xc + (R - 2.7) * cos(PI / 2.0 - (mm + ss / 60.0) / 60.0 * 2.0 * PI);
ym = yc + (R - 2.7) * sin(PI / 2.0 - (mm + ss / 60.0) / 60.0 * 2.0 * PI);
xh = xc + (R - 5.7) * cos(PI / 2.0 - (hh + (mm + ss / 60.0) / 60.0) / 12.0 * 2.0 * PI);
yh = yc + (R - 5.7) * sin(PI / 2.0 - (hh + (mm + ss / 60.0) / 60.0) / 12.0 * 2.0 * PI);
//时针
glColor3f(1, 0, 0);
glLineWidth(5);
glBegin(GL_LINES);
glVertex2f(xc, yc);
glVertex2f(xs, ys);
glEnd();
glColor3f(1, 1, 0);
glBegin(GL_LINES);
glVertex2f(xc, yc);
glVertex2f(xm, ym);
glEnd();
glColor3f(0, 1, 1);
glBegin(GL_LINES);
glVertex2f(xc, yc);
glVertex2f(xh, yh);
glEnd();
//电子时钟
//时钟外框
glBegin(GL_LINES);
glVertex2f(-3, -7);
glVertex2f(3, -7);
glEnd();
glBegin(GL_LINES);
glVertex2f(-3, -7);
glVertex2f(-3, -4.2);
glEnd();
glBegin(GL_LINES);
glVertex2f(-3, -4.2);
glVertex2f(3, -4.2);
glEnd();
glBegin(GL_LINES);
glVertex2f(3, -4.2);
glVertex2f(3, -7);
glEnd();
//时间显示
glRasterPos2f(-1.8, -6);
if (hh <= 9) {
h = (int)hh;
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, h + '0');
}
else {
h = (int)hh;
int k, q;
k = h % 10;
q = int(h / 10);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, q + '0');
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, k + '0');
}
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, ':');
if (mm <= 9) {
m = (int)mm;
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, m + '0');
}
else {
m = (int)mm;
int k, q;
k = m % 10;
q = int(m / 10);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, q + '0');
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, k + '0');
}
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, ':');
if (ss <= 9) {
s = (int)ss;
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, s + '0');
}
else {
s = (int)ss;
int k, q;
k = s % 10;
q = int(s / 10);
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, q + '0');
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, k + '0');
}
glutSwapBuffers(); //双缓冲的刷新模式
}
void mytime(int t)
{
theta += 0.1;
if (theta >= 2 * PI) theta -= 2 * PI;
GetLocalTime(&timeNow); //获取系统时间
hh = timeNow.wHour; //获取小时时间
mm = timeNow.wMinute; //获取分钟时间
ss = timeNow.wSecond; //获取秒时间
glutPostRedisplay(); //重画,相当于重新调用Display(),改编后的变量得以传给绘制函数
glutTimerFunc(100, mytime, 10); //1000毫秒后调用时间函数 mytime
}
void Reshape(GLsizei w, GLsizei h)
{
glMatrixMode(GL_PROJECTION); //投影矩阵模式
glLoadIdentity(); //矩阵堆栈清空
gluOrtho2D(-1.5*R*w / h, 1.5*R*w / h, -1.5*R, 1.5*R); //设置裁剪窗口大小
glViewport(0, 0, w, h); //设置视区大小
glMatrixMode(GL_MODELVIEW); //模型矩阵模式
glLoadIdentity(); //矩阵堆栈清空
}