原始视频C:/video.mp4

现在视频C:/temp/1/10位uuid/Tom.mp4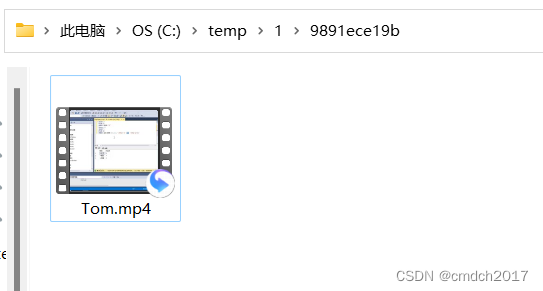
package org.example.newStudy;
import org.example.util.UUIDUtils;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
@Controller
@RequestMapping("/test")
public class Test {
private static String parentPath = "C:/temp/1/";
private static byte[] nowbytes;
@RequestMapping("/test")
public void getVideoStream(HttpServletResponse response) {
FileUtils.getVideoOutStream(response,nowbytes);
}
public static void main(String[] args) throws Exception {
FileUtils.buildDirectory(parentPath);
int count = 6;
while (count-- > 0) {
String fileName = UUIDUtils.generateUUID(10);
String dirPath = FileUtils.buildDirectory(parentPath + fileName);
byte[] bytes = FileUtils.fileToBytes(new File("C:/video.mp4"));
nowbytes=bytes;
FileUtils.fileToBytes(bytes, dirPath, "Tom.mp4");
}
}
}
package org.example.newStudy;
import lombok.extern.slf4j.Slf4j;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
@Slf4j
public class FileUtils {
private static final int CACHE_SIZE = 1024;
public static String buildFile(String path, String suffix) {
if (suffix == null || suffix.equals("")) {
} else {
path = path + suffix;
}
File file = new File(path);
if (!file.exists()) {
try {
file.createNewFile();
} catch (Exception e) {
e.printStackTrace();
}
}
return path;
}
public static String buildDirectory(String path) {
File file = new File(path);
if (!file.exists()) {
try {
file.mkdirs();
} catch (Exception e) {
e.printStackTrace();
}
}
return path;
}
public static void getVideoOutStream(HttpServletResponse response, byte[] bytes) {
OutputStream outputStream = null;
try {
outputStream = response.getOutputStream();
outputStream.write(bytes);
outputStream.flush();
} catch (Exception e) {
log.error("error", e);
throw new RuntimeException(e);
} finally {
try {
outputStream.close();
} catch (IOException e) {
log.error("error", e);
}
}
}
public static void fileToBytes(byte[] bytes, String filePath, String fileName) {
BufferedOutputStream bos = null;
FileOutputStream fos = null;
File file = null;
try {
if (!filePath.endsWith("/")) {
filePath += "/";
}
file = new File(filePath + fileName);
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
fos = new FileOutputStream(file);
bos = new BufferedOutputStream(fos);
bos.write(bytes);
} catch (Exception e) {
e.printStackTrace();
} finally {
if (bos != null) {
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public static byte[] fileToBytes(File file) throws Exception {
byte[] data = new byte[0];
if (file.exists()) {
FileInputStream in = new FileInputStream(file);
ByteArrayOutputStream out = new ByteArrayOutputStream(2048);
byte[] cache = new byte[CACHE_SIZE];
int nRead = 0;
while ((nRead = in.read(cache)) != -1) {
out.write(cache, 0, nRead);
out.flush();
}
out.close();
in.close();
data = out.toByteArray();
}
return data;
}
}