·····························实现FactoryBean接口配置bean························
Spring 中有两种类型的 Bean, 一种是普通Bean, 另一种是工厂Bean, 即FactoryBean.
工厂 Bean 跟普通Bean不同, 其返回的对象不是指定类的一个实例, 其返回的是该工厂 Bean 的 getObject 方法所返回的对象
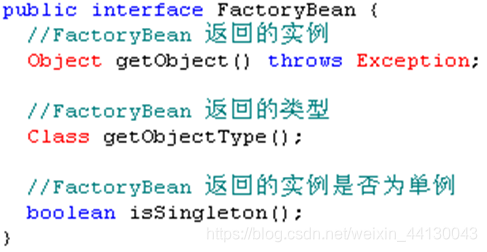
············································code·············································
(carfactorybean.java)
package factorybean;
import org.springframework.beans.factory.FactoryBean;
//自定义factorybean 需要实现factorybean 接口
public class carfactorybean implements FactoryBean<car> {
//仅供示例,使用不方便
private String brand;
public void setBrand(String brand) {
this.brand = brand;
}
//返回bean的对象
@Override
public car getObject() throws Exception {
// TODO Auto-generated method stub
return new car(brand, "123");
}
//返回bean的类型
@Override
public Class<?> getObjectType() {
// TODO Auto-generated method stub
return car.class;
}
@Override
public boolean isSingleton() {
// TODO Auto-generated method stub
return false;
}
}
(bean-factorybean.xml)
<!--
通过factorybean 来配置 bean的实例
class:指向factorybean的全类名
property: 配置factorybean的属性
但实际返回的是factorybean 的getobject()方法返回的实例
-->
<bean id = "car" class = "factorybean.carfactorybean">
<property name = "brand" value = "BMW"></property>
</bean>
·····························class path组件扫描·······································
组件扫描(component scanning): Spring 能够从 classpath 下自动扫描, 侦测和实例化具有特定注解的组件.
特定组件包括:
@Component: 基本注解, 标识了一个受 Spring 管理的组件
@Respository: 标识持久层组件
@Service: 标识服务层(业务层)组件
@Controller: 标识表现层组件
对于扫描到的组件, Spring 有默认的命名策略: 使用非限定类名, 第一个字母小写. 也可以在注解中通过 value 属性值标识组件的名称
当在组件类上使用了特定的注解之后, 还需要在 Spring 的配置文件中声明 <context:component-scan> :
base-package 属性指定一个需要扫描的基类包,Spring 容器将会扫描这个基类包里及其子包中的所有类.
当需要扫描多个包时, 可以使用逗号分隔.
如果仅希望扫描特定的类而非基包下的所有类,可使用 resource-pattern 属性过滤特定的类,示例:

<context:include-filter> 子节点表示要包含的目标类
<context:exclude-filter> 子节点表示要排除在外的目标类
<context:component-scan> 下可以拥有若干个 <context:include-filter> 和 <context:exclude-filter> 子节点
<context:include-filter> 和 <context:exclude-filter> 子节点支持多种类型的过滤表达式:
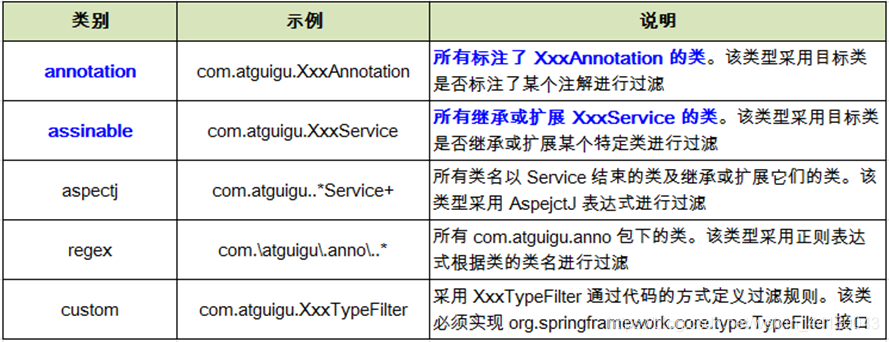
·············································code·······································
(annotaion.testObject.java)···································@Component
package annotation;
import org.springframework.stereotype.Component;
@Component
public class testObject {}
(annotation.controller.UserController.java)····················@Controller
package annotation.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import annotation.Service.UserService;
@Controller
public class UserController {
//required = false即使没有匹配的bean也不会报错,引用其他组件时要加上@autowire
@Autowired(required = false)
private UserService userservice;
public void execute() {
System.out.println("UserController execute....");
userservice.add();
}
}}
(annotation.Service.UserService.java)·······························@Service
package annotation.Service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Repository;
import org.springframework.stereotype.Service;
import annotation.repository.UserRepository;
@Service
public class UserService {
//当一个接口有两个实现类时,会报错,可以指定唯一id解决(@Repository("userRepository"))
//或者指定类的名字 @Qualifier("UserRepositoryimple") 前提是该类中没有指定id
@Autowired
@Qualifier("userRepositoryimple")
private UserRepository userRepository;
public void add() {
System.out.println("UserService add...");
userRepository.save();
}
(annotaion.repository.UserRepository.java)·····················@Repository
package annotation.repository;
public interface UserRepository {
void save();
}
(annotaion.repository.UserJdbcRepository.java)·····················@Repository
package annotation.repository;
import org.springframework.stereotype.Repository;
@Repository
public class UserJdbcRepository implements UserRepository {
@Override
public void save() {
// TODO Auto-generated method stub
System.out.println("UserJdbcRepository save...");
}
}
(annotaion.repository.UserRepositoryImple.java)·····················@Repository
package annotation.repository;
import org.springframework.stereotype.Repository;
//指定id
@Repository("userRepository")
public class UserRepositoryimple implements UserRepository {
@Override
public void save() {
// TODO Auto-generated method stub
System.out.println("UserRepositoryimple...");
}
}
(main)·························································(Main)
public class Main {
public static void main(String[] args) {
ApplicationContext app = new ClassPathXmlApplicationContext("bean-annotation.xml");
// testObject to = (testObject)app.getBean("testObject");
// System.out.println(to);
UserController usercontroller = (UserController)app.getBean("userController");
System.out.println(usercontroller);
usercontroller.execute();
// UserService us = (UserService)app.getBean("userService");
// System.out.println(us);
//
// UserRepository user = (UserRepository)app.getBean("userRepository");
// System.out.println(user);
(bean-annotation.xml)···········································(XML)
<!-- 指定 spring IOC容器扫描的包 -->
<!-- 可以通过 resource-pattern 指定扫描的资源
<context:component-scan
base-package="annotation"
resource-pattern = "repository/*.class"
></context:component-scan>
-->
<!-- context:exclude-filter "annotation"子节点指定排除哪些指定表达式的组件 -->
<!-- context:include-filter "annotation"子节点指定包含哪些表达式的组件 该节点需要在component-scan中把use-default-filters属性设为false-->
<!-- context:exclude-filter "assignable"包含指定实现类或接口的组件 -->
<!-- context:include-filter "assignable" 排除指定实现类或接口的组件 该节点需要在component-scan中把use-default-filters属性设为false-->
<context:component-scan
base-package="annotation" ><!-- use-default-filters = "false" -->
<!--
<context:exclude-filter type="annotation"
expression="org.springframework.stereotype.Repository"/>
<context:include-filter type="annotation"
expression="org.springframework.stereotype.Repository"/>
<context:exclude-filter type="assignable"
expression="annotation.repository.UserRepository"/>
<context:include-filter type="assignable"
expression="annotation.repository.UserRepository"/>
-->
</context:component-scan>
}