目录结构
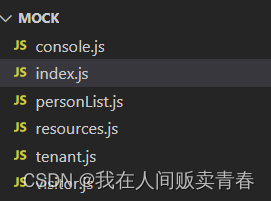
import Mock from 'mockjs'
import visitor from './visitor'
import tenant from './tenant'
import personList from './personList'
import resources from './resources'
import consoles from './console'
const mocks = [
...visitor,
...tenant,
...personList,
...resources,
...consoles,
]
function XHR2ExpressReqWrap(response) {
return function(options) {
let result = null
if (response instanceof Function) {
const { body, type, url } = options
result = response({
method: type,
body: JSON.parse(body),
query: param2Obj(url)
})
} else {
result = response
}
return Mock.mock(result)
}
}
function param2Obj(url) {
const search = url.split('?')[1]
if (!search) {
return {}
}
return JSON.parse(
'{"' +
decodeURIComponent(search)
.replace(/"/g, '\\"')
.replace(/&/g, '","')
.replace(/=/g, '":"')
.replace(/\+/g, ' ') +
'"}'
)
}
mocks.forEach(item => {
Mock.mock(new RegExp(item.url), item.type, XHR2ExpressReqWrap(item.response))
})
import Mock from 'mockjs'
var Random = Mock.Random
var getConsoleList = {
url: '/mock/tmesh/console/_all\.*',
type: 'get',
response: config => {
let response = {
entity : {
records: [],
total: 500,
page: 1,
size: 10
}
}, count = 10;
while(count > 0) {
response.entity.records.push({
'id|+1': Random.string('lower', 7, 10),
'startTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'endTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'owner': Random.first(),
'count': Random.integer(1, 20),
})
count--
}
return response
}
}
var getActionList = {
url: '/mock/tmesh/console/action\.*',
type: 'get',
response: config => {
let response = {
entity : {
records: [],
total: 501,
page: 1,
size: 10
}
}, count = 10;
while(count > 0) {
response.entity.records.push({
'id|+1': Random.string('lower', 7, 10),
'avatar': Random.image('250x250', Random.color()),
'name': Random.first(),
'card': Random.string('lower', 5, 10),
'phone': /^1[358][1-9]\d{8}/,
'startTime': Random.date('yyyy-MM-dd HH:mm:ss'),
})
count--
}
return response
}
}
export default [
getConsoleList,
getActionList
]
import Mock from 'mockjs'
var Random = Mock.Random
var getPersonnelList = {
url: '/mock/tmesh/personList/_all\.*',
type: 'get',
response: config => {
let response = {entity : []}, count = 6;
while(count > 0) {
response.entity.push({
'id|+1': Random.integer('lower', 7, 10),
'personList|5':[{
'avatar': Random.image('250x250', Random.color()),
'name': Random.first(),
'card': Random.string('lower', 5, 10),
'phone': /^1[358][1-9]\d{8}/
}],
'no': Random.integer('lower', 5, 10),
'name': `${Random.first()} ${Random.last()}`,
'groups': Random.integer(),
'groupNames': `${Random.first()} ${Random.last()}`,
'idCard': Random.integer(),
'icCard': Random.integer(),
'qrCode': Random.image('720x300', Random.color(), 'bg-img'),
'wiegand': Random.cparagraph(),
'mail': Random.integer(),
'password': Random.integer(),
'rentCount': Random.cparagraph(),
'temp': Random.cparagraph(),
'birthday': Random.date('yyyy-MM-dd HH:mm:ss'),
'getFiedList': Random.integer(),
'type': Random.cparagraph(),
'newField': Random.cparagraph(),
'entryTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'gender': Random.integer(),
'position': Random.cparagraph(),
})
count--
}
return response
}
}
var creatPersonnelList = {
url: '/mock/tmesh/personList',
type: 'post',
response: _ => {
return {
code: 200,
message: 'post success'
}
}
}
var removePersonnelList = {
url: '/mock/tmesh/personList',
type: 'post',
response: _ => {
return {
code: 200,
message: 'post success'
}
}
}
export default [
getPersonnelList,
creatPersonnelList,
removePersonnelList
]
import Mock from 'mockjs'
var Random = Mock.Random
var getResourcesList = {
url: '/mock/tmesh/resources/_all\.*',
type: 'get',
response: config => {
let response = {
entity :[]
}, count = 6;
while(count > 0) {
response.entity.push({
'id|+1': Random.string('lower', 7, 10),
'name': Random.first(),
'preview':Random.ctitle(3, 5),
'type': Random.ctitle(3, 5),
'create_time': Random.date('yyyy-MM-dd HH:mm:ss'),
'updata_time': Random.date('yyyy-MM-dd HH:mm:ss'),
'status': Random.ctitle(3, 5),
'create_name': Random.first(),
})
count--
}
return response
}
}
export default [
getResourcesList
]
import Mock from 'mockjs'
var Random = Mock.Random
var getTenantList = {
url: '/mock/tmesh/tenant/_all\.*',
type: 'get',
response: config => {
let response = { entity: [] }, count = 6;
while (count > 0) {
response.entity.push({
'id|+1': Random.integer(),
'avatar': Random.image('250x250', Random.color(), Random.first()),
'bgImage': Random.image('720x300', Random.color(), 'bg-img'),
'createTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'name': `${Random.first()} ${Random.last()}`,
'address': Random.city(true),
'title': Random.ctitle(),
'description': Random.cparagraph()
})
count--
}
return response
}
}
var creatTenant = {
url: '/mock/tmesh/tenant',
type: 'post',
response: _ => {
return {
code: 200,
message: 'post success'
}
}
}
export default [
getTenantList,
creatTenant
]
import Mock from 'mockjs'
var Random = Mock.Random
var getVisitorRecordList = {
url: '/mock/tmesh/visitor/records\.*',
type: 'get',
response: config => {
let response = {
entity: {
records: [],
total: 500,
page: 1,
size: 10
}
}, count = 10;
while (count > 0) {
response.entity.records.push({
'id|+1': Random.string('lower', 7, 10),
'visitorList|5': [
{
'avatar': Random.image('250x250', Random.color()),
'name': Random.first(),
'card': Random.string('lower', 5, 10),
'phone': /^1[358][1-9]\d{8}/
}
],
'startTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'endTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'owner': Random.first(),
'count': Random.integer(1, 20),
'state': Random.integer(0, 3),
'reason': Random.ctitle(),
'passMode': Random.string(),
'remark': Random.cparagraph()
})
count--
}
return response
}
}
var getVisitorList = {
url: '/mock/tmesh/visitor/visitors\.*',
type: 'get',
response: config => {
let response = {
entity: {
records: [],
total: 501,
page: 1,
size: 10
}
}, count = 10;
while (count > 0) {
response.entity.records.push({
'id|+1': Random.string('lower', 7, 10),
'avatar': Random.image('250x250', Random.color()),
'name': Random.first(),
'card': Random.string('lower', 5, 10),
'phone': /^1[358][1-9]\d{8}/,
'startTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'endTime': Random.date('yyyy-MM-dd HH:mm:ss'),
'owner': Random.first(),
'state': Random.integer(0, 3),
'reason': Random.ctitle(),
'passMode': Random.string(),
'remark': Random.cparagraph()
})
count--
}
return response
}
}
export default [
getVisitorList,
getVisitorRecordList
]