1.Math
1.1 Math类概述
Math包含执行基本数字运算的方法
/*
Math类的常用方法
*/
public class MathDemo {
public static void main(String[] args) {
//public static int abs (int,a):返回参数的绝对值
System.out.println(Math.abs(80));
System.out.println(Math.abs(-80));
System.out.println("------------");
// public static double ceil (double a):返回大于或等于参数的最小double值,等于一个整数
System.out.println(Math.ceil(12.34));
System.out.println(Math.ceil(12.56));
System.out.println("------------");
// public static double floor (double a):返回小于或等于参数的最小double值,等于一个整数
System.out.println(Math.floor(12.34));
System.out.println(Math.floor(12.56));
System.out.println("------------");
// public static int round (floot a):按照四舍五入返回最接近的int
System.out.println(Math.round(12.34F));
System.out.println(Math.round(12.56F));
System.out.println("------------");
// public static int max (int a,int b):返回两个int中的较大值
System.out.println(Math.max(66,88));
System.out.println("------------");
// public static int min (int a,int b):返回两个int中的较小值
System.out.println(Math.min(66,88));
System.out.println("------------");
// public static double pow (double a,double b):返回a的b次幂的值
System.out.println(Math.pow(2.0,3.0));
System.out.println("------------");
// public static double random ():返回值为random的正值,[0.0,1.0)
// System.out.println(Math.random());
System.out.println((int)(Math.random() *100) + 1);
System.out.println("------------");
}
}
2. System
2.1 System 类概述
System包含了几个有用的类字段和方法,它不能被实例化
/*
System类的常用方法
*/
public class SystemDemo {
public static void main(String[] args) {
/*System.out.println("开始");
//public static void exit(int status):终止当前运行的Java虚拟机,非零表示异常终止
System.exit(0);
System.out.println("结束");*/
// public static long currentTimeMillis():返回当前(以毫秒为单位)
// System.out.println(System.currentTimeMillis());
// System.out.println(System.currentTimeMillis() *1.0 / 1000 / 60 /60 / 24 /365 +"年" );
long start = System.currentTimeMillis();
for (int i = 0; i < 10000; i++) {
System.out.println(i);
}
long end = System.currentTimeMillis();
System.out.println("共耗时"+ (end - start) +"毫秒");
}
}
3. Object
3.1 Object 类的概述
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
/*
Object 类是基层结构的根,每个类都可以将 Object类作为超类,所有类都直接或间接继承自该类
建议所有子类重写该方法
*/
public class ObjectDemo {
public static void main(String[] args) {
Student s = new Student();
s.setName("成龙");
s.setAge(55);
System.out.println(s); //com.it_01.Student@1b6d3586
System.out.println(s.toString()); //com.it_01.Student@1b6d3586
}
}
3.2 Object 类的常用方法
/*
测试类
public boolean equals (Object obj) :指示一些其它对象是否等于此
*/
public class ObjectDemo {
public static void main(String[] args) {
Student s1 = new Student();
s1.setName("成龙");
s1.setAge(55);
Student s2 = new Student();
s2.setName("成龙");
s2.setAge(55);
//需求 比较两个内容是否相同
// System.out.println(s1 == s2);
System.out.println(s1.equals(s2));
}
}
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Student student = (Student) o;
if (age != student.age) return false;
return name != null ? name.equals(student.name) : student.name == null;
}
}
对equals 方法解释
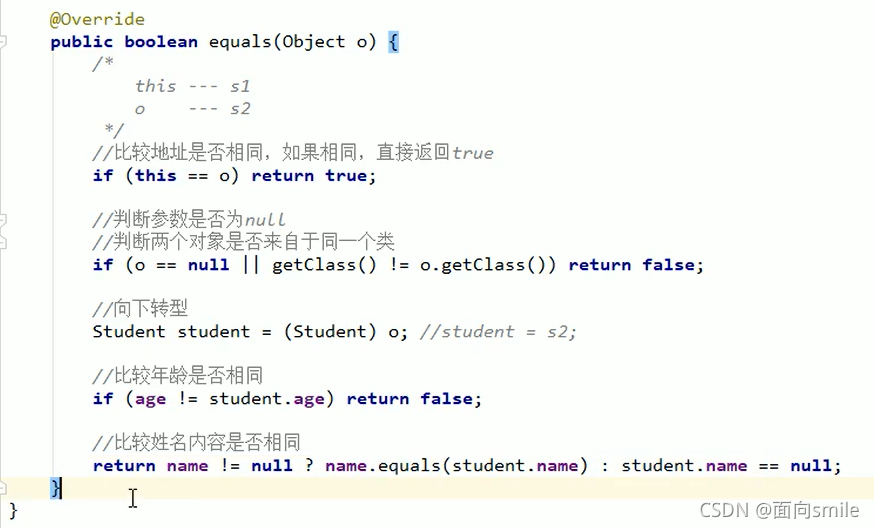
4. Arrays
4.1 冒泡排序
/*
冒泡排序
一种排序的方式,对要进行排序的数据中相邻的数据进行两两比较,将较大的数据放在后面
依次对所有的数据进行操作,直至所有数据按要求完成排序
*/
public class ArrayDemo {
public static void main(String[] args) {
//定义一个数组
int[] arr = {24,69,80,57,13};
System.out.println("排序前:"+arrayToString(arr) );
/*
//第一比较
for (int i = 0; i < arr.length - 1 - 0; i++){
if (arr[i] > arr[i+1]){
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
}
System.out.println("第一次比较后:"+arrayToString(arr) );
//第二比较
for (int i = 0; i < arr.length - 1 - 1; i++){
if (arr[i] > arr[i+1]){
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
}
System.out.println("第二次比较后:"+arrayToString(arr) );
//第三比较
for (int i = 0; i < arr.length - 1 - 2 ; i++){
if (arr[i] > arr[i+1]){
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
}
System.out.println("第三次比较后:"+arrayToString(arr) );
//第四比较
for (int i = 0; i < arr.length - 1 - 3; i++){
if (arr[i] > arr[i+1]){
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
}
System.out.println("第四次比较后:"+arrayToString(arr) );
*/
for (int x = 0; x < arr.length - 1; x++) {
for (int i = 0; i < arr.length - 1 - x; i++){
if (arr[i] > arr[i+1]){
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
}
}
System.out.println("排序后:"+arrayToString(arr) );
}
//把数组中的元素按照指定的规则组成一个字符串:[元素1,元素2,,,,]
public static String arrayToString(int[] arr){
StringBuilder sb = new StringBuilder();
sb.append("[");
for (int i = 0; i < arr.length; i++){
if (i == arr.length - 1){
sb.append(arr[i]);
}else {
sb.append(arr[i]).append(",");
}
}
sb.append("]");
String s = sb.toString();
return s;
}
}
4.2 Arrays 类的概述和常用方法
import java.util.Arrays;
/*
Arrays 类包含用于操作数组的各种方法
public static String toString (int[] a):返回指定数组内容的字符串表现形式
public static void sort (int[] a) :按照数字顺序排列指定的数组
*/
public class ArraysDemo {
public static void main(String[] args) {
//定义一个数组
int[] arr = {24,69,80,57,13};
System.out.println("排序前:"+ Arrays.toString(arr));
Arrays.sort(arr);
System.out.println("排序后:"+ Arrays.toString(arr));
}
}
5. 基本类型包装类
5.1 基本类型包装类概述
/*
基本类型包装类
*/
public class IntegerDemo {
public static void main(String[] args) {
//需求:获取int的范围
System.out.println(Integer.MAX_VALUE);
System.out.println(Integer.MIN_VALUE);
}
}
5.2 Integer 类的概述和使用
/*
构造方法:
public Integer (int value) :根据 int 值创建Integer对象(过时)
public Integer (String s) :根据 String 值创建Integer对象(过时)
静态方法获取对象
public static Integer valueOf(int i) :表示返回指定int 值的Integer实例
public static Integer valueOf(String s) :表示返回指定的Integer 对象String
*/
public class IntegerDemo {
public static void main(String[] args) {
/* //public Integer (int value) :根据 int 值创建Integer对象(过时)
Integer i1 = new Integer(100);
System.out.println(i1);
//public Integer (String s) :根据 String 值创建Integer对象(过时)
Integer i2 = new Integer("123");
System.out.println(i2);*/
//public static Integer valueOf(int i) :表示返回指定int 值的Integer实例
Integer i1 = Integer.valueOf(100);
System.out.println(i1);
// public static Integer valueOf(String s) :表示返回指定的Integer 对象String
Integer i2 = Integer.valueOf("123");
System.out.println(i2);
}
}
5.3 Int 和 String 的相互转换
/*
int 和 String 相互转换
*/
public class IntegerDemo {
public static void main(String[] args) {
//int --> String
int num = 100;
//方式1
String s1 = "" + num;
System.out.println(s1);
//方式2
String s2 = String.valueOf(num);
System.out.println(s2);
System.out.println("----------");
//String --> int
String s = "100";
//方式1
// String --> Integer --> int
Integer i1 = Integer.valueOf(s);
//public intValue();
int x = i1.intValue();
System.out.println(x);
//方式2
int t = Integer.parseInt(s);
System.out.println(s);
}
}
案例 字符串中数据排序
import java.util.Arrays;
public class IntegerTest {
public static void main(String[] args) {
//定义一个字符串
String s = "91 27 46 38 50";
//把字符串中的数据存储到int数组中
String[] strArray = s.split(" ");
/*for (int i = 0; i< strArray.length;i++){
System.out.println(strArray[i]);
}*/
//定义一个int数组,把String[] 数组中的每一个元素存储到int数组中
int[] arr = new int[strArray.length];
for (int i = 0; i < arr.length; i++){
arr[i] = Integer.parseInt(strArray[i]);
}
/*for (int i = 0; i < arr.length; i++){
System.out.println(arr[i]);
}*/
//对int数组排序
Arrays.sort(arr);
//把排序后的int数组中的元素进行拼接到一个字符串,采用StringBuilder
StringBuilder sb = new StringBuilder();
for (int i = 0; i < arr.length; i++){
if (i == arr.length -1){
sb.append(arr[i]);
}else {
sb.append(arr[i]).append(" ");
}
}
String result = sb.toString();
//输出结果
System.out.println(result);
}
}