1. 函数使用示例
package cn.cerish.container.map.linkedHashmap;
import java.util.HashMap;
import java.util.LinkedHashMap;
public class LinkedHashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> hashMap = new HashMap<>();
LinkedHashMap<String, Integer> linkedHashMap = new LinkedHashMap<>();
LinkedHashMap<String, Integer> linkedHashMap01 = new LinkedHashMap<>(16, 0.75f, true);
for(int i = 1; i <= 10; i++) {
hashMap.put("我是" + i, i);
linkedHashMap.put("我是" + i, i);
linkedHashMap01.put("我是" + i, i);
}
System.out.println("now hashmap: " + hashMap);
System.out.println("now linkedHashmap: " + linkedHashMap);
System.out.println("now linkedHashmap01: " + linkedHashMap01);
System.out.println("===== linkedHashMap accessOrder 对 get() 的影响 =====");
System.out.println("hashMap.get('我是1'): " + hashMap.get("我是1"));
System.out.println("linkedHashMap.get('我是1'): " + linkedHashMap.get("我是1"));
System.out.println("linkedHashMap01.get('我是1'): " + linkedHashMap01.get("我是1"));
System.out.println("now hashmap: " + hashMap);
System.out.println("now linkedHashmap: " + linkedHashMap);
System.out.println("now linkedHashmap01: " + linkedHashMap01);
System.out.println("======= 迭代器也是按照维护的链条顺序进行遍历 =====");
System.out.print("linkedHashMap keySet(): \t");
linkedHashMap.keySet().forEach((key) -> System.out.print(key + "\t"));
System.out.println();
System.out.print("linkedHashMap values(): \t");
linkedHashMap.values().forEach((value) -> System.out.print(value + "\t"));
System.out.println();
System.out.print("linkedHashMap entrySet(): \t");
linkedHashMap.entrySet().forEach((e) -> System.out.print(e.getKey() + " = " + e.getValue() + "\t"));
System.out.println();
System.out.println("==================================================");
System.out.println("linkedHashMap.getOrDefault('我是1', 11): " + linkedHashMap.getOrDefault("我是1", 11));
System.out.println("linkedHashMap.getOrDefault('我是1', 11): " + linkedHashMap.getOrDefault("我是11", 11));
System.out.println("now linkedHashMap: " + linkedHashMap);
System.out.println("----------------------------------");
linkedHashMap.clear();
System.out.println("clear()后, now linkedHashMap: " + linkedHashMap);
}
}
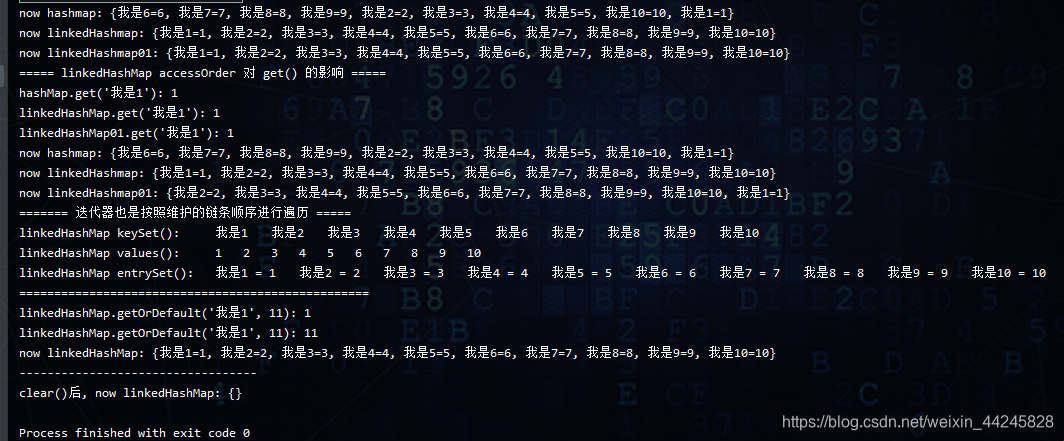
2. 线程安全问题
- LinkedHashMap 线程安全吗?HashMap 线程不安全,依赖于它的LinkedHashMap肯定不安全。
package cn.cerish.container.map.linkedHashmap;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.LinkedBlockingDeque;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
public class ThreadSafeTest {
public static void main(String[] args) throws InterruptedException {
LinkedHashMap<String , Integer> linkedHashMap = new LinkedHashMap<>();
CountDownLatch countDownLatch = new CountDownLatch(10);
ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(50, 100, 0L, TimeUnit.SECONDS,
new LinkedBlockingDeque<>());
for(int i = 1; i <= 10; i++) {
int finalI = i;
threadPoolExecutor.execute(() -> {
for(int j = 1; j <= 10; j++) {
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
linkedHashMap.put(String.valueOf(finalI * 100 + j), j);
};
countDownLatch.countDown();
});
}
countDownLatch.await();
System.out.println("hashMap.size(): " + linkedHashMap.size());
AtomicInteger size = new AtomicInteger();
linkedHashMap.forEach((key, value) -> {
System.out.print(key + " = " + value + "; \t");
if(size.incrementAndGet() >= 10) {
size.set(0);
System.out.println();
}
});
}
}
- size 变小,元素个数变少,具体原因回看HashMap篇即可
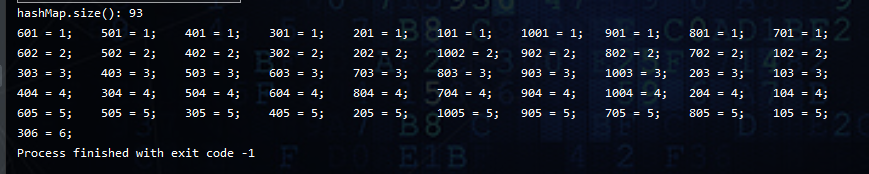
2.1 线程安全问题解决
- 使用
Map<String, Integer> linkedHashMap = Collections.synchronizedMap(new LinkedHashMap<String, Integer>());
即可。
package cn.cerish.container.map.linkedHashmap;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.LinkedBlockingDeque;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
public class ThreadSafeTest {
public static void main(String[] args) throws InterruptedException {
Map<String, Integer> linkedHashMap = Collections.synchronizedMap(new LinkedHashMap<String, Integer>());
CountDownLatch countDownLatch = new CountDownLatch(10);
ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(50, 100, 0L, TimeUnit.SECONDS,
new LinkedBlockingDeque<>());
for(int i = 1; i <= 10; i++) {
int finalI = i;
threadPoolExecutor.execute(() -> {
for(int j = 1; j <= 10; j++) {
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
linkedHashMap.put(String.valueOf(finalI * 100 + j), j);
};
countDownLatch.countDown();
});
}
countDownLatch.await();
System.out.println("hashMap.size(): " + linkedHashMap.size());
AtomicInteger size = new AtomicInteger();
linkedHashMap.forEach((key, value) -> {
System.out.print(key + " = " + value + "; \t");
if(size.incrementAndGet() >= 10) {
size.set(0);
System.out.println();
}
});
}
}
- 一家人齐齐整整,任务完成。
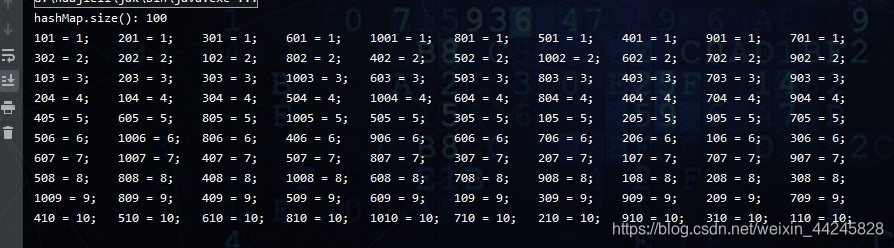