1 反转链表
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-SvtGH5UO-1675838755088)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675781896532.png)]](https://img-blog.csdnimg.cn/97de9a3c42e64df2ae3f75bfae7019e1.png)
/*
struct ListNode {
int val;
struct ListNode *next;
ListNode(int x) :
val(x), next(NULL) {
}
};*/
class Solution {
public:
ListNode* ReverseList(ListNode* pHead) {
ListNode* ans = new ListNode(0);
ListNode* p = pHead;
while(p!=NULL){
ListNode* next = p->next;
p->next = ans->next;
ans->next = p;
p = next;
}
return ans->next;
}
};
2 链表内指定区间反转
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-iEynpLUV-1675838755092)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675782037484.png)]](https://img-blog.csdnimg.cn/2615401ce67e427d9820057649dcd035.png)
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
class Solution {
public:
/**
*
* @param head ListNode类
* @param m int整型
* @param n int整型
* @return ListNode类
*/
ListNode* reverseBetween(ListNode* head, int m, int n) {
// write code here
ListNode* ans = new ListNode(0);
ans->next=head;
ListNode* pre = ans;
ListNode* cur = head;
for(int i=1;i<m;i++){
pre = cur;
cur = cur->next;
}
for(int i=m;i<n;i++){
ListNode* tem = cur->next;
cur->next = tem->next;
tem->next = pre->next;
pre->next = tem;
}
return ans->next;
}
};
3 链表中的节点每k个一组翻转
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-q13EkG7W-1675838755097)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675822009081.png)]](https://img-blog.csdnimg.cn/f7e2698908224825b8628ba2bda3fa67.png)
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
class Solution {
public:
/**
*
* @param head ListNode类
* @param k int整型
* @return ListNode类
*/
ListNode* reverseKGroup(ListNode* head, int k) {
// write code here
ListNode* tail = head;
for(int i=0;i<k;i++){
if(tail == NULL)
return head;
tail = tail->next;
}
ListNode* pre = NULL;
ListNode* cur = head;
while (cur != tail) {
ListNode* temp = cur->next;
cur->next = pre;
pre = cur;
cur = temp;
}
head->next = reverseKGroup(cur, k);
return pre;
}
};
4 合并两个排序的链表
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-0nqI2gF6-1675838755098)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675822512364.png)]](https://img-blog.csdnimg.cn/05b0f282fa8c4d60b004be4a5a2b0a68.png)
/*
struct ListNode {
int val;
struct ListNode *next;
ListNode(int x) :
val(x), next(NULL) {
}
};*/
class Solution {
public:
ListNode* Merge(ListNode* pHead1, ListNode* pHead2) {
ListNode* ans = new ListNode(0);
ListNode* p = ans;
ListNode* p1 = pHead1;
ListNode* p2 = pHead2;
while (p1 != NULL && p2 != NULL) {
ListNode* temp = NULL;
if(p1->val <= p2->val){
temp = p1;
p1 = p1->next;
}else{
temp = p2;
p2 = p2->next;
}
p->next = temp;
p = temp;
}
if(p1 != NULL) p->next = p1;
if(p2 != NULL)p->next = p2;
return ans->next;
}
};
5 合并k个已排序的链表
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-11GwdvYn-1675838755099)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675823703640.png)]](https://img-blog.csdnimg.cn/f670fbfd833e40c89b434b961a5c7098.png)
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode *mergeKLists(vector<ListNode *> &lists) {
ListNode* ans = new ListNode(0);
ListNode* tail = ans;
while(1){
int p = 0, i = 0, t = -1;
for(i=0;i<lists.size();i++){
if(lists[i] != NULL){
p++;
if(t == -1 || lists[t]->val > lists[i]->val){
t = i;
}
}
}
if(p == 0) break;
tail->next = lists[t];
tail = tail->next;
lists[t] = lists[t]->next;
}
return ans->next;
}
};
6 判断链表中是否有环
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-xQtgn5Uk-1675838755102)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675825015884.png)]](https://img-blog.csdnimg.cn/efc721234ced42188cfebd2d6baa660d.png)
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
bool hasCycle(ListNode *head) {
ListNode* p1 = head;
ListNode* p2 = head;
while (p2 != NULL && p2->next != NULL) {
p2 = p2->next->next;
p1 = p1 ->next;
if(p1 == p2)
return true;
}
return false;
}
};
7 链表中环的入口结点
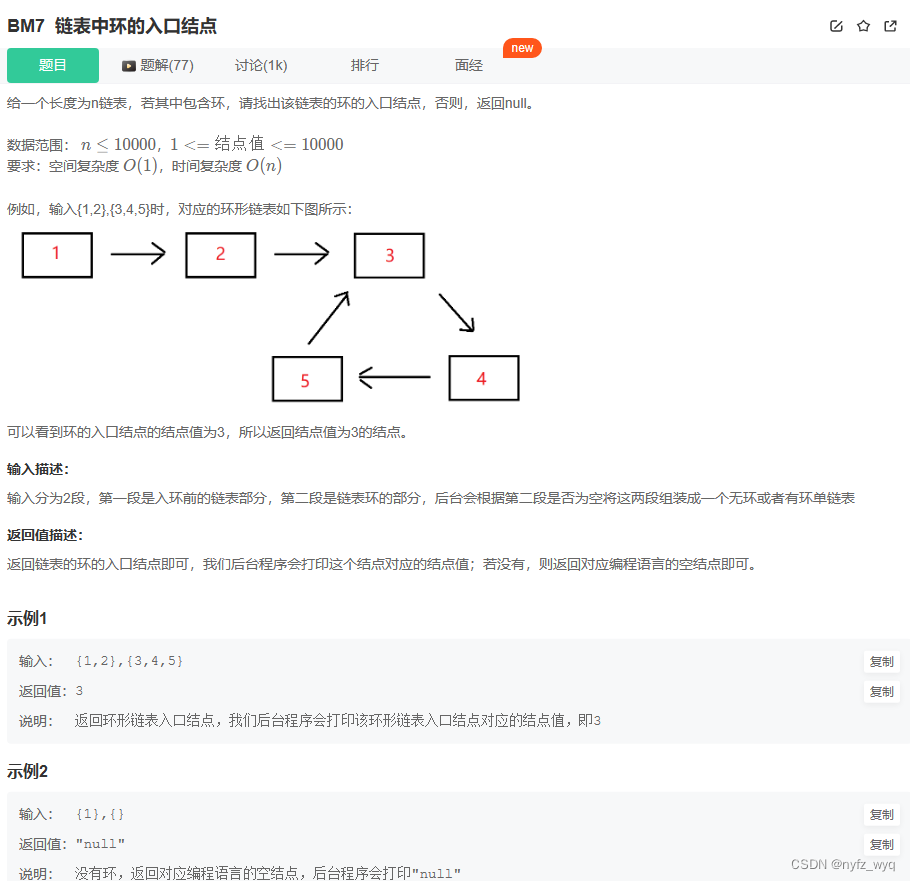
/*
struct ListNode {
int val;
struct ListNode *next;
ListNode(int x) :
val(x), next(NULL) {
}
};
*/
class Solution {
public:
ListNode* EntryNodeOfLoop(ListNode* pHead) {
ListNode* p1 = pHead;
ListNode* p2 = pHead;
while (p2 != NULL && p2->next != NULL) {
p1 = p1->next;
p2 = p2->next->next;
if(p1 == p2)
break;
}
if(p2==NULL || p2->next==NULL) return NULL;
p1 = pHead;
while(p1 != p2){
p1 = p1->next;
p2 = p2->next;
}
return p1;
}
};
8 链表中倒数最后k个结点
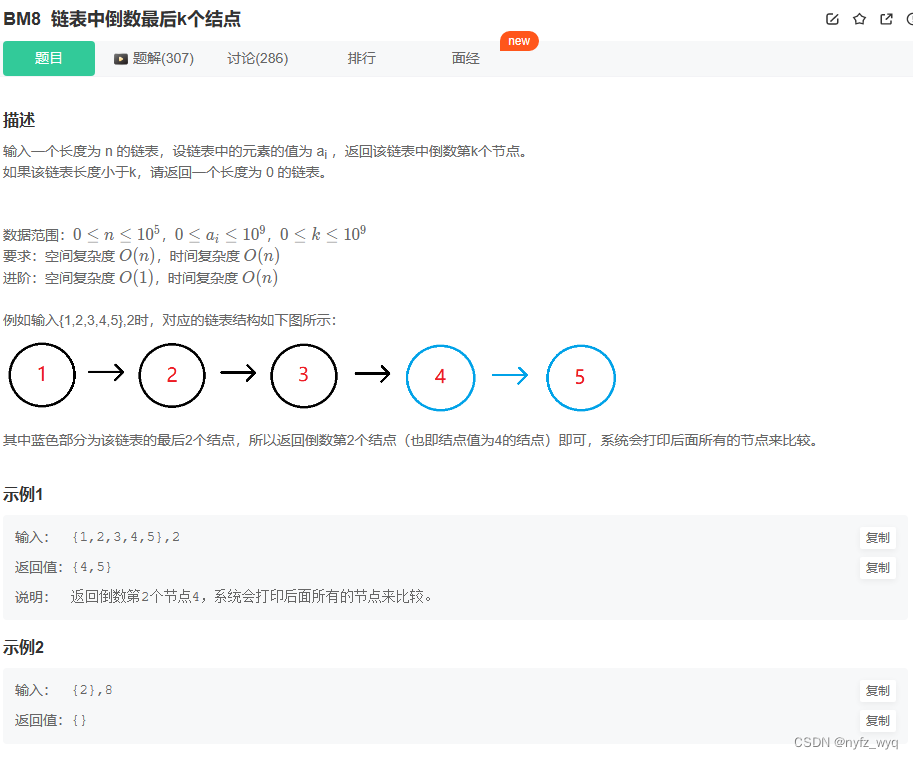
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* ListNode(int x) : val(x), next(nullptr) {}
* };
*/
class Solution {
public:
/**
* 代码中的类名、方法名、参数名已经指定,请勿修改,直接返回方法规定的值即可
*
*
* @param pHead ListNode类
* @param k int整型
* @return ListNode类
*/
ListNode* FindKthToTail(ListNode* pHead, int k) {
// write code here
if(pHead == NULL || k == 0) return NULL;
ListNode* p1 = pHead;
ListNode* p2 = pHead;
for(int i=1;i<k && p2!=NULL;i++)
p2 = p2->next;
if(p2 == NULL) return NULL;
while (p2->next!=NULL) {
p1 = p1->next;
p2 = p2->next;
}
return p1;
}
};
9 删除链表的倒数第n个节点
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-erDYguSp-1675838755104)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675827354265.png)]](https://img-blog.csdnimg.cn/9fa5ba3ffae044c69c4193b0d8f2a81e.png)
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
class Solution {
public:
/**
*
* @param head ListNode类
* @param n int整型
* @return ListNode类
*/
ListNode* removeNthFromEnd(ListNode* head, int n) {
if(head==NULL || n == 0) return head;
ListNode* my = new ListNode(0);
my->next = head;
ListNode* p1 = my;
ListNode* p2 = my;
for(int i=0;i<n+1;i++){
p2 = p2->next;
}
while (p2!=NULL) {
p2 = p2->next;
p1 = p1->next;
}
p1->next = p1->next->next;
return my->next;
}
};
10 两个链表的第一个公共结点
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-U2MzX9I3-1675838755105)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675827933194.png)]](https://img-blog.csdnimg.cn/b95943d80a6f4ef88ef6667738ffb820.png)
/*
struct ListNode {
int val;
struct ListNode *next;
ListNode(int x) :
val(x), next(NULL) {
}
};*/
class Solution {
public:
ListNode* FindFirstCommonNode( ListNode* pHead1, ListNode* pHead2) {
if(pHead1==NULL || pHead2==NULL) return NULL;
ListNode* p1 = pHead1;
ListNode* p2 = pHead2;
while(p1 != p2){
p1 = p1->next;
p2 = p2->next;
if(p1==NULL && p2 == NULL)
return NULL;
if(p1 == NULL) p1 = pHead2;
if(p2 == NULL) p2 = pHead1;
}
return p1;
}
};
11 链表相加(二)
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-7m4u4BvJ-1675838755106)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675835318352.png)]](https://img-blog.csdnimg.cn/6917835cf8844eb794533ddc6d72dbe8.png)
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode* reviseList(ListNode* head) {
// write code here
ListNode* ans = new ListNode(0);
ListNode* p = head;
while (p!=NULL) {
ListNode* temp = p->next;
p->next = ans->next;
ans->next = p;
p = temp;
}
return ans->next;
}
/**
*
* @param head1 ListNode类
* @param head2 ListNode类
* @return ListNode类
*/
ListNode* addInList(ListNode* head1, ListNode* head2) {
// write code here
head1 = reviseList(head1);
head2 = reviseList(head2);
ListNode* ans = new ListNode(0);
int t =0;
while (!(head1==NULL && head2==NULL)) {
int a = head1==NULL ? 0:head1->val;
int b = head2==NULL ? 0:head2->val;
int c = a + b + t;
t = c / 10;
ListNode* temp = new ListNode(c%10);
temp->next = ans->next;
ans->next = temp;
if(head1) head1=head1->next;
if(head2) head2=head2->next;
}
if(t != 0){
ListNode* temp = new ListNode(t);
temp->next = ans->next;
ans->next = temp;
}
return ans->next;
}
};
12 单链表的排序
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-Ah9SQRUB-1675838755106)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675836277375.png)]](https://img-blog.csdnimg.cn/0bdcfd708a09460aa847daa38fc95acf.png)
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
class Solution {
public:
ListNode* merList(ListNode* h1, ListNode* h2) {
if (h1 == NULL) return h2;
if (h2 == NULL) return h1;
ListNode* ans = new ListNode(0);
ListNode* p = ans;
while (h1 != NULL && h2 != NULL) {
if (h1->val < h2->val) {
p->next = h1;
p = h1;
h1 = h1->next;
} else {
p->next = h2;
p = h2;
h2 = h2->next;
}
}
if (h1 != NULL) p->next = h1;
if (h2 != NULL) p->next = h2;
return ans->next;
}
/**
*
* @param head ListNode类 the head node
* @return ListNode类
*/
ListNode* sortInList(ListNode* head) {
// write code here
if (head == NULL || head->next == NULL)
return head;
ListNode* p1 = head;
ListNode* p2 = head->next;
ListNode* p3 = head->next->next;
while (p3 != NULL && p3->next != NULL) {
p1 = p1->next;
p2 = p2->next;
p3 = p3->next->next;
}
p1->next = NULL;
return merList(sortInList(head),sortInList(p2));
}
};
13 判断一个链表是否为回文结构
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-MqkihkuE-1675838755107)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675837007167.png)]](https://img-blog.csdnimg.cn/05523cdc203242ee88f14f03de167ea0.png)
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
#include <vector>
class Solution {
public:
/**
*
* @param head ListNode类 the head
* @return bool布尔型
*/
bool isPail(ListNode* head) {
// write code here
vector<int> nums;
ListNode* p = head;
while (p!=NULL) {
nums.push_back(p->val);
p = p->next;
}
for(int i=0;i<nums.size();i++){
if(nums[i] != nums[nums.size()-i-1])
return false;
}
return true;
}
};
14 链表的奇偶重排
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-2kM1gOgy-1675838755108)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675837505301.png)]](https://img-blog.csdnimg.cn/7d555b36a4e540d4a64e48ad9da3f9ab.png)
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* ListNode(int x) : val(x), next(nullptr) {}
* };
*/
class Solution {
public:
/**
* 代码中的类名、方法名、参数名已经指定,请勿修改,直接返回方法规定的值即可
*
*
* @param head ListNode类
* @return ListNode类
*/
ListNode* oddEvenList(ListNode* head) {
// write code here
if(!head) return NULL;
ListNode* L1 = new ListNode(0);
ListNode* p1 = L1;
ListNode* L2 = new ListNode(0);
ListNode* p2 = L2;
for(int i=1;head!=NULL;i++){
if(i%2 == 1){
p1->next = head;
p1 = head;
head = head->next;
p1->next = NULL;
}else {
p2->next = head;
p2 = head;
head = head->next;
p2->next = NULL;
}
}
p1->next = L2->next;
return L1->next;
}
};
15 删除有序链表中重复的元素-I
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-8Ku9rj2J-1675838755109)(C:\Users\Dell\AppData\Roaming\Typora\typora-user-images\1675838015987.png)]](https://img-blog.csdnimg.cn/9df52d01cb124d4198324619c6eef472.png)
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
class Solution {
public:
/**
*
* @param head ListNode类
* @return ListNode类
*/
ListNode* deleteDuplicates(ListNode* head) {
// write code here
if(head==NULL) return NULL;
ListNode* pre = head;
ListNode* cur = head->next;
int x = pre->val;
while (cur) {
if(cur->val == x){
pre->next = cur->next;
}else {
x = cur->val;
pre = cur;
}
cur = cur->next;
}
return head;
}
};
16 删除有序链表中重复的元素-II
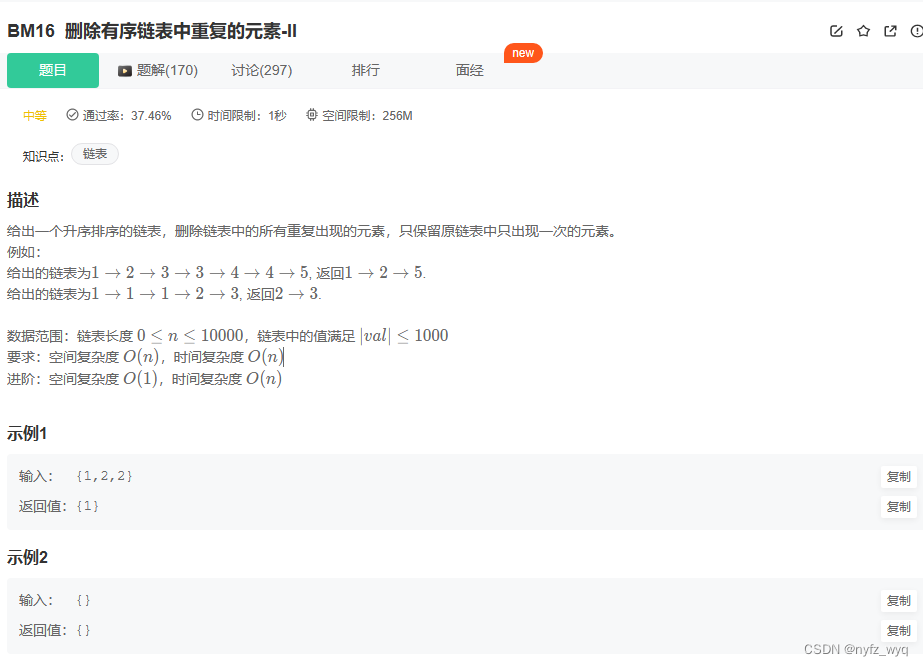
/**
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
class Solution {
public:
/**
*
* @param head ListNode类
* @return ListNode类
*/
ListNode* deleteDuplicates(ListNode* head) {
// write code here
if (!head) return head;
ListNode* ans = new ListNode(0);
ans->next = head;
ListNode* pre = ans;
ListNode* cur = head;
while (cur) {
int x = cur->val;
int n = 0;
ListNode* p = cur;
while (p != NULL && p->val == x) {
n++;
p = p->next;
}
if (n != 1) {
pre->next = p;
cur = p;
}else {
pre = cur;
cur = cur->next;
}
}
return ans->next;
}
};