异常01:Error和Exception
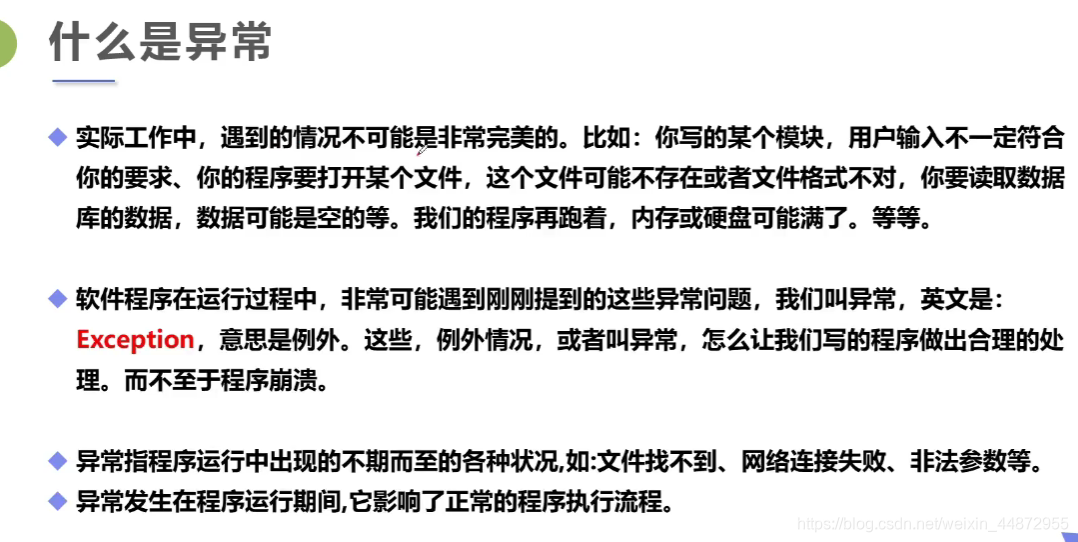
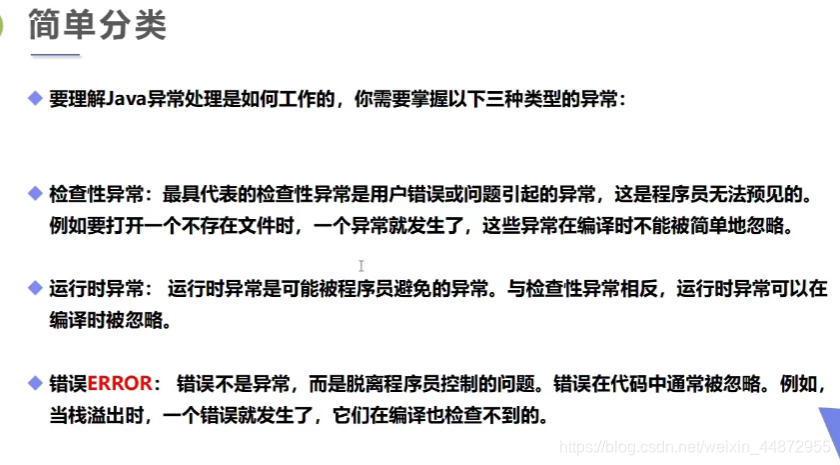
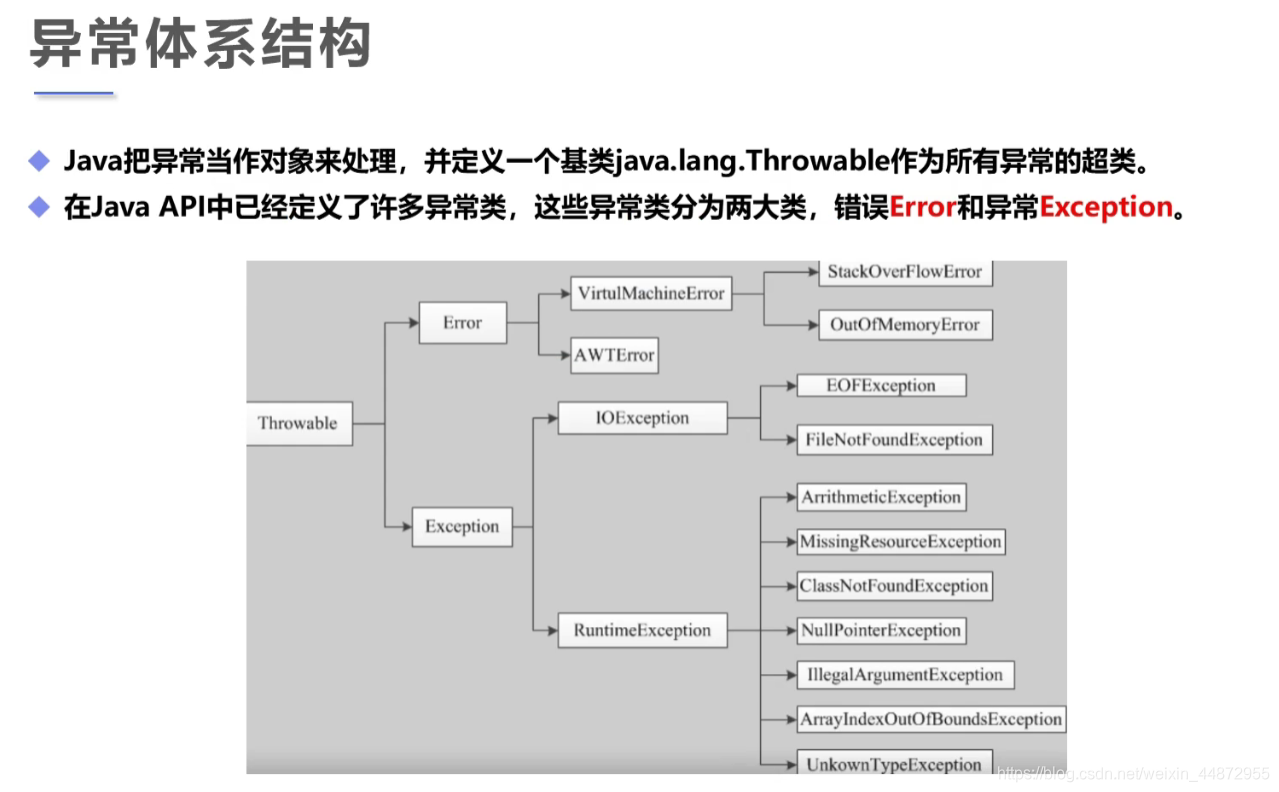
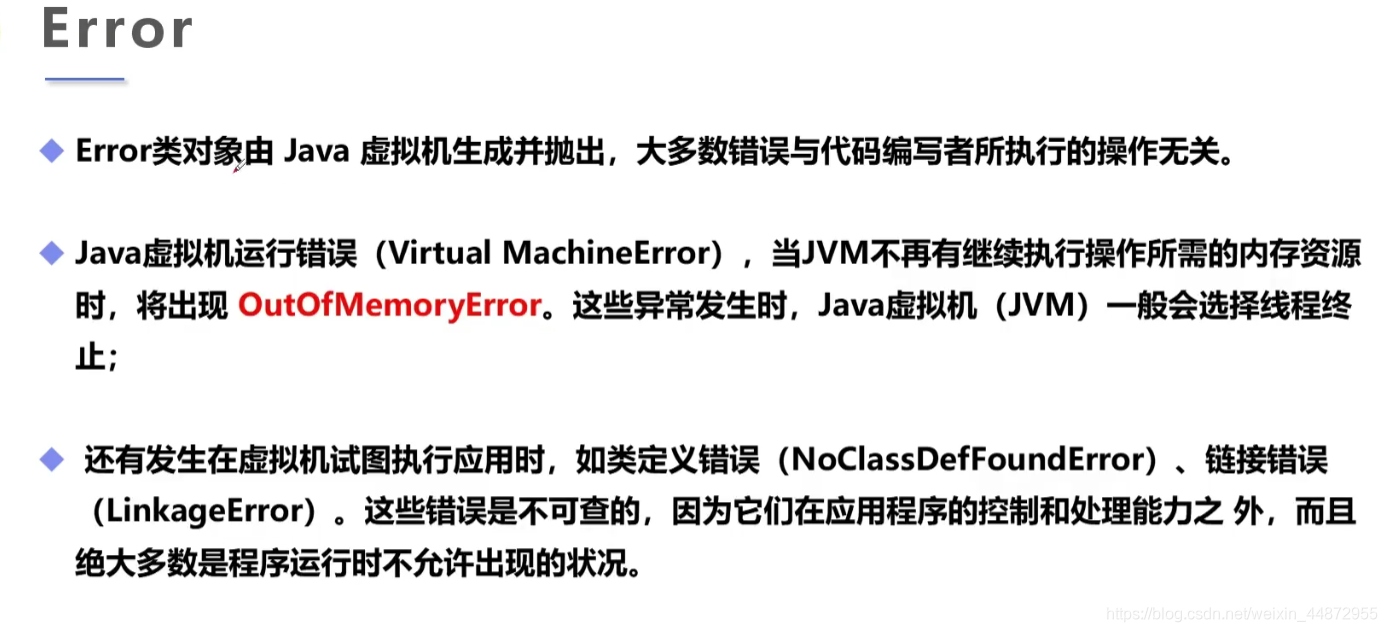
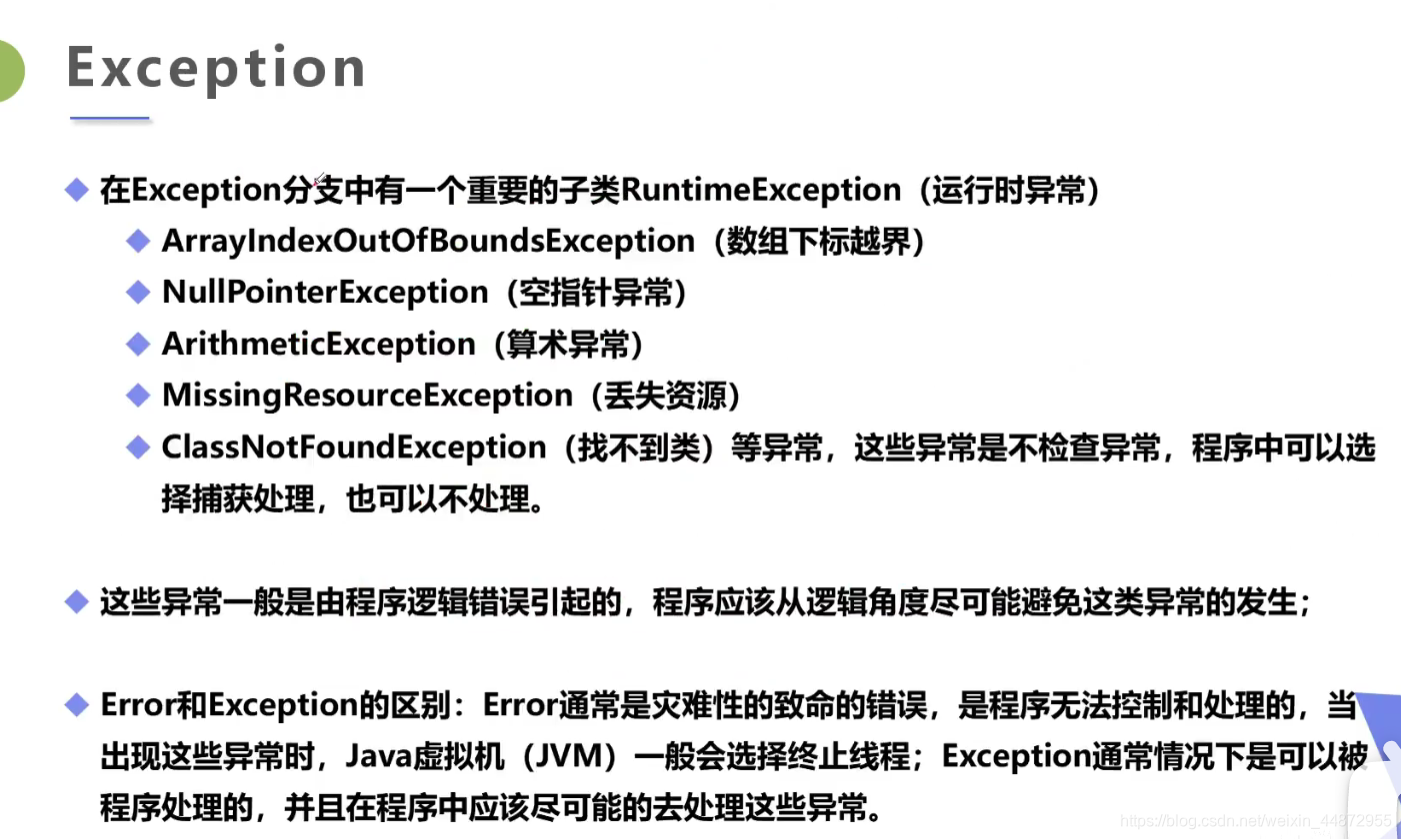
异常02:捕获和抛出异常
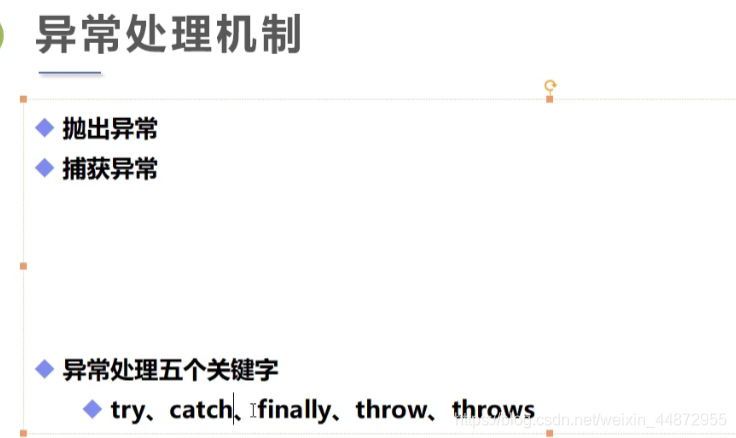
try、catch用法
package com.exception.demo01;
public class Demo01 {
public static void main(String[] args) {
int a = 1;
int b = 0;
try {
System.out.println(a / b);
}catch (ArithmeticException e){
System.out.println("程序出现异常,变量b不能为0");
}finally {
System.out.println("finally");
}
}
}
catch参数
package com.exception.demo01;
public class Demo02 {
public void a(){
b();
}
public void b(){
a();
}
public static void main(String[] args) {
try {
new Demo02().a();
}catch (Throwable e){
System.out.println("程序发生了异常!");
}finally {
System.out.println("finally");
}
}
}
捕获多个异常
package com.exception.demo01;
public class Demo03 {
public void a(){
b();
}
public void b(){
a();
}
public static void main(String[] args) {
try {
new Demo03().a();
}catch (Error e){
System.out.println("Error异常");
}catch (Exception e){
System.out.println("Exception异常");
}catch (Throwable t){
System.out.println("Throwable异常");
}finally {
System.out.println("finally");
}
System.out.println("====================================");
int a = 1;
int b = 0;
try {
System.out.println(a / b);
} catch (Exception e) {
System.out.println("Exception异常");
e.printStackTrace();
} finally {
System.out.println("finally");
}
}
}
抛出异常
package com.exception.demo01;
public class Demo04 {
public void test(int a, int b){
if (b == 0){
throw new ArithmeticException();
}
}
public static void main(String[] args) {
new Demo04().test(1, 0);
}
}
throws向上抛出异常
package com.exception.demo01;
public class Demo05 {
public void test(int a, int b) throws ArithmeticException{
if (b == 0){
throw new ArithmeticException();
}
}
public static void main(String[] args) {
try {
new Demo05().test(1, 0);
} catch (ArithmeticException e) {
e.printStackTrace();
}
}
}
异常03:自定义异常及经验小结
自定义异常
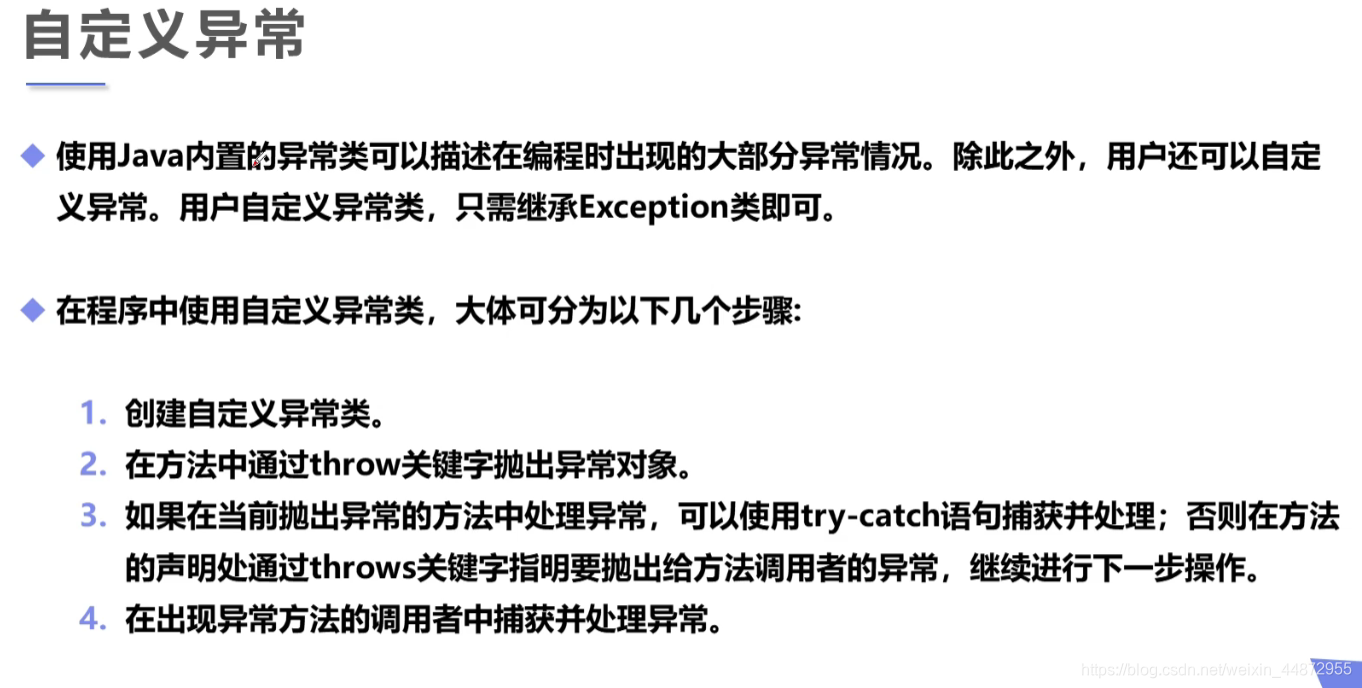
package com.exception.demo02;
public class MyException extends Exception {
private int detail;
public MyException(int a) {
this.detail = a;
}
@Override
public String toString() {
return "MyException{" +
"detail=" + detail +
'}';
}
}
package com.exception.demo02;
public class Test {
public static void test(int a) throws MyException {
System.out.println("传递的参数为:" + a);
if (a > 10){
throw new MyException(a);
}
System.out.println("ok");
}
public static void main(String[] args) {
try {
new Test().test(15);
} catch (MyException e) {
System.out.println(e);
e.printStackTrace();
}
}
}
小结
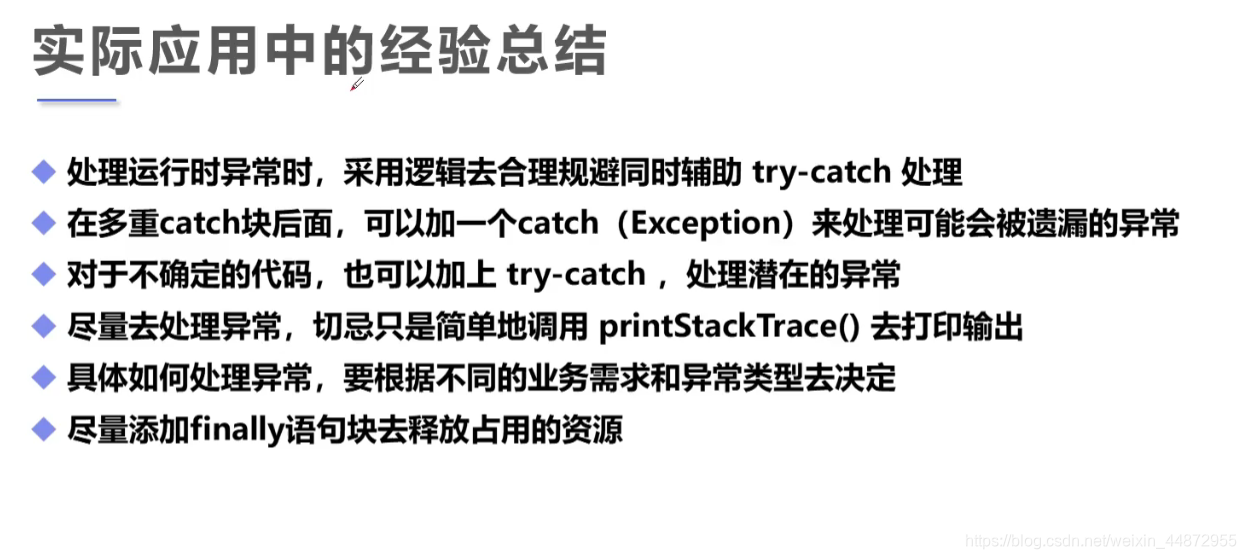