1.菜单
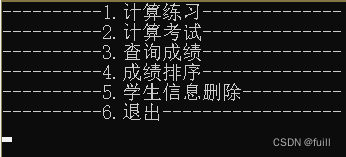
2.功能
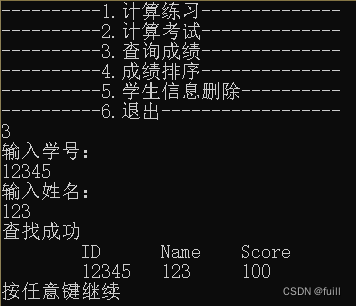
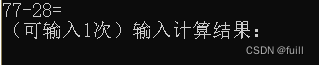
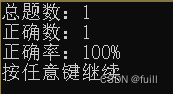
#include <iostream>
#include <ctime>
#include <cstdlib>
#include <windows.h>
#include <stdio.h>
#define MAX 255
using namespace std;
typedef struct
{
char ID[25];
char Name[25];
double Score;
int Correct;
int Wrong;
double Accuracy;
} STU;
STU stu[MAX];
int count_stu=0;
void title(int Number_of_answers,int Number_of_questions,int Is_practice)
{
double Single_question_score=100.0/Number_of_questions;
double score=0;
int correct=0;
int wrong=0;
double accuracy=1;
for(int j=0; j<Number_of_questions; j++)
{
system("cls");
srand(time(0));
int a=rand()%100;
int b=rand()%100;
int op=rand()%4;//0:+ 1:- 2:* 3:/
int c=0;
if(op==0) c=a+b;
else if(op==1)
{
if(b>a) swap(a,b);
c=a-b;
}
else if(op==2) c=a*b;
else if(op==3)
{
if(b>a) swap(a,b);
int tem[100];
int count=0;
for(int i=1; i<=a; i++)
{
if(a%i==0)
tem[count++]=i;
}
int index=rand()%count;
b=tem[index];
c=a/b;
}
char OP[5]="+-*/";
cout<<a<<OP[op]<<b<<"="<<endl;
int temp=Number_of_answers;
while(temp--)
{
cout<<"(可输入"<<temp+1<<"次)"<<"输入计算结果:"<<endl;
int ans;
cin>>ans;
if(ans==c)
{
cout<<"正确\n";
score+=Single_question_score;
correct++;
Sleep(1000);
break;
}
else
{
cout<<"错误\n";
if(temp==0&&Is_practice)
{
cout<<"正确答案:"<<c<<endl;
wrong++;
}
Sleep(1500);
}
}
}
accuracy=correct/(double)Number_of_questions;
if(Is_practice==0)
{
stu[count_stu].Score=score;
stu[count_stu].Correct=correct;
stu[count_stu].Wrong=wrong;
stu[count_stu].Accuracy=accuracy;
}
system("cls");
cout<<"总题数:"<<Number_of_questions<<endl;
cout<<"正确数:"<<correct<<endl;
cout<<"正确率:"<<accuracy*100<<"%"<<endl;
fflush(stdin);
cout<<"按任意键继续\n";
getchar();
}
void Print_Header()
{
cout<<"\t"<<"ID"<<"\t"<<"Name"<<"\t"<<"Score"<<endl;
}
void prin_(int index)
{
cout<<"\t"<<stu[index].ID<<"\t"<<stu[index].Name<<"\t"<<stu[index].Score<<endl;
}
void prin_all_stu()
{
Print_Header();
for(int i=0; i<count_stu; i++)
{
prin_(i);
}
fflush(stdin);
cout<<"按任意键继续\n";
getchar();
}
int Query_grades(char Id[],char name[])
{
for(int i=0; i<count_stu; i++)
{
if(strcmp(stu[i].ID,Id)==0)
{
if(strcmp(stu[i].Name,name)==0)
{
return i;
}
}
}
return -1;
}
void sort_score()
{
for(int i=0; i<count_stu-1; i++)
{
for(int j=0; j<count_stu-i-1; j++)
{
if(stu[j].Score<stu[j+1].Score)
{
STU tem=stu[j];
stu[j]=stu[j+1];
stu[j+1]=tem;
}
}
}
}
void del(char Id[],char name[])
{
int index=Query_grades(Id,name);
if(index!=-1)
{
cout<<"查找成功\n";
Print_Header();
prin_(index);
for(int i=index; i<count_stu-1; i++)
{
stu[i]=stu[i+1];
}
count_stu--;
cout<<"删除成功\n";
fflush(stdin);
getchar();
}
else
{
cout<<"未查找到\n";
fflush(stdin);
getchar();
}
}
void save()
{
FILE* fp;
fp=fopen("stu.txt","w");
if(fp==NULL)
{
printf("文件打开失败!\n");
return;
}
else
{
for(int i=0; i<count_stu; i++)
{
fprintf(fp,"%s %s %lf %d %d %lf\n",stu[i].ID,stu[i].Name,stu[i].Score,stu[i].Correct,stu[i].Wrong,stu[i].Accuracy);
}
}
fclose(fp);
}
void read()
{
FILE* fp;
fp=fopen("stu.txt","r");
if(fp==NULL)
{
printf("文件打开失败!\n");
return;
}
else
{
int i=0;
int fla=6;
do
{
fla=fscanf(fp,"%s %s %lf %d %d %lf\n",stu[i].ID,stu[i].Name,&stu[i].Score,&stu[i].Correct,&stu[i].Wrong,&stu[i].Accuracy);
i++;
}
while(fla==6);
i--;
count_stu+=i;
}
fclose(fp);
}
void menu()
{
read();
int option=0;
while(option!=6)
{
system("cls");
cout<<"----------1.计算练习--------------" << endl;
cout<<"----------2.计算考试--------------" << endl;
cout<<"----------3.查询成绩--------------" << endl;
cout<<"----------4.成绩排序--------------" << endl;
cout<<"----------5.学生信息删除----------" << endl;
cout<<"----------6.退出------------------" << endl;
cin>>option;
if(option==1)
{
int Number_of_questions=0;
cout<<"输入要练习的题数:\n";
fflush(stdin);
cin>> Number_of_questions;
title(3,Number_of_questions,1);
}
else if(option==2)
{
cout<<"输入学号:\n";
fflush(stdin);
cin>>stu[count_stu].ID;
cout<<"输入姓名:\n";
fflush(stdin);
cin>>stu[count_stu].Name;
int Number_of_questions=0;
cout<<"输入要练习的题数:\n";
fflush(stdin);
cin>> Number_of_questions;
title(1,Number_of_questions,0);
count_stu++;
save();
}
else if(option==3)
{
char Id[25];
char name[25];
cout<<"输入学号:\n";
fflush(stdin);
cin>>Id;
cout<<"输入姓名:\n";
fflush(stdin);
cin>>name;
int index=Query_grades(Id,name);
if(index!=-1)
{
cout<<"查找成功\n";
Print_Header();
prin_(index);
cout<<"按任意键继续\n";
fflush(stdin);
getchar();
for(int i=index; i<count_stu-1; i++)
{
stu[i]=stu[i+1];
}
count_stu--;
}
else
{
cout<<"未查找到\n";
cout<<"按任意键继续\n";
fflush(stdin);
getchar();
}
}
else if(option==4)
{
sort_score();
prin_all_stu();
}
else if(option==5)
{
char Id[25];
char name[25];
cout<<"输入学号:\n";
fflush(stdin);
cin>>Id;
cout<<"输入姓名:\n";
fflush(stdin);
cin>>name;
del(Id,name);
save();
}
}
}
int main()
{
menu();
return 0;
}