提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档
目录
前言
1.打开目录
#include <dirent.h>
DIR *opendir(const char *name);
DIR *fdopendir(int fd); 使用文件描述符,要配合open函数使用
DIR是用来描述一个打开的目录文件的结构体类型
成功时返回目录流指针;出错时返回NULL
2.读取目录
#include <dirent.h>
struct dirent *readdir(DIR *dirp);
struct dirent是用来描述目录流中一个目录项的结构体类型
包含成员char d_name[256] 参考帮助文档
成功时返回目录流dirp中下一个目录项;
出错或到末尾时时返回NULL
#include<dirent.h>
#include<stdio.h>
int main(int argc, const char *argv[])
{
DIR* dp;
struct dirent *dt;
dp = opendir("//home/linux/level_4");
if(dp == NULL){
perror("readdir: ");
return 0;
}
while((dt = readdir(dp))!= NULL){
printf("%s\n",dt->d_name);
}
return 0;
}
3.关闭目录
closedir函数用来关闭一个目录文件:
#include <dirent.h>
int closedir(DIR *dirp);
成功时返回0;出错时返回EOF
4.修改文件权限
chmod/fchmod函数用来修改文件的访问权限:
#include <sys/stat.h>
int chmod(const char *path, mode_t mode);
int fchmod(int fd, mode_t mode);
成功时返回0;出错时返回EOF
注意:在vmware和windows共享的文件夹下,有些权限不能改变。
注意:r = 4, w = 2, x = 1;下面代码中0444是用八进制表示的,实际也可以直接窗口命令行输入
chmod 444 2.txt
#include<stdio.h>
int main(int argc, const char *argv[])
{
int ret;
ret = chmod("2.txt",0444);
if(ret < 0){
perror("chmod_t: ");
}
return 0;
}
5.获取文件属性
#include <sys/stat.h>
int stat(const char *path, struct stat *buf);
int lstat(const char *path, struct stat *buf);
int fstat(int fd, struct stat *buf);
成功时返回0;出错时返回EOF
如果path是符号链接stat获取的是目标文件的属性;而lstat获取的是链接文件的属性
重要的几个成员变量
通过系统宏来判断文件类型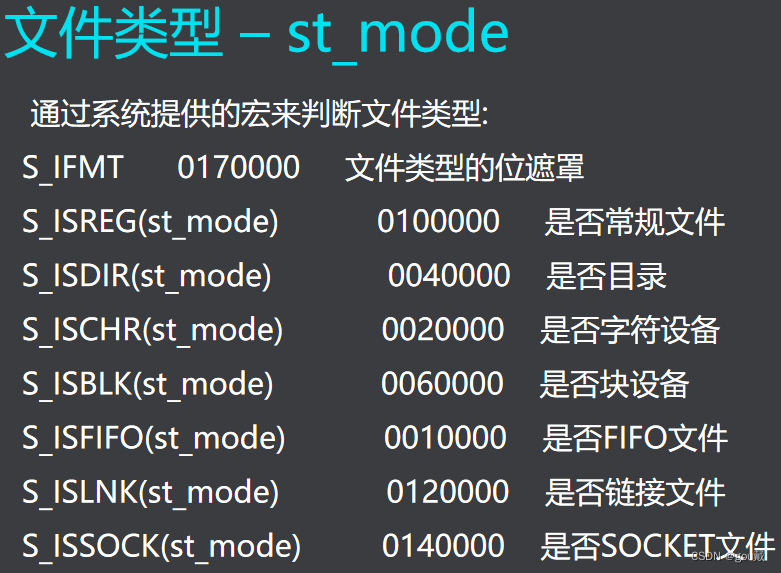
通过系统宏来获取文件访问权限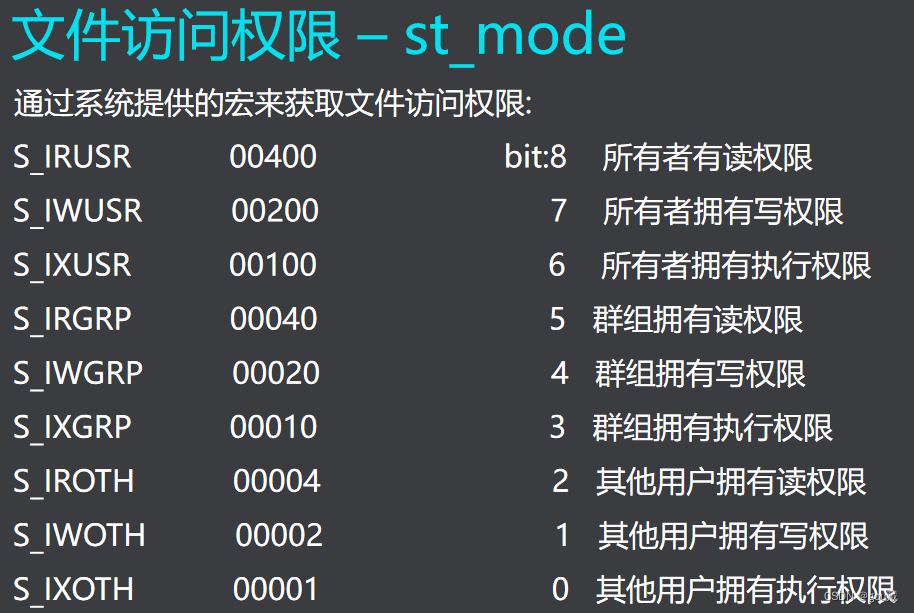
练习:
获取文件并显示文件属性
#include<stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <time.h>
int main(int argc, const char *argv[])
{
struct stat buf;
int ret;
//获取文件属性
ret = stat("1.txt",&buf);
if(ret < 0){
perror("stst_t.c: ");
return 0;
}
//判断文件类型
if(S_ISREG(buf.st_mode)){
printf("-");
}
if(S_ISDIR(buf.st_mode)){
printf("d");
}
if(S_ISCHR(buf.st_mode)){
printf("c");
}
if(S_ISBLK(buf.st_mode)){
printf("b");
}
if(S_ISFIFO(buf.st_mode)){
printf("p");
}
if(S_ISSOCK(buf.st_mode)){
printf("s");
}
//文件权限
int i;
for(i = 8; i >= 0;i--){
if(buf.st_mode & (1<<i)){
switch(i%3){
case 2:
printf("r");
break;
case 1:
printf("w");
break;
case 0:
printf("x");
break;
}
}else{
printf("-");
}
}
//打印文件大小
printf(" %d",(int)buf.st_size);
//打印时间信息
struct tm *loct;
loct = localtime(&buf.st_ctime);
printf(" %04d-%02d-%02d %02d:%02d:%02d",loct->tm_year+1900,loct->tm_mon+1,loct->tm_mday,
loct->tm_hour,loct->tm_min,loct->tm_sec);
printf(" stat_t.c\n");
return 0;
}
6.库的概念:
参考文章:
库文件是在链接阶段使用的,分为静态库libxxx.a和动态库*.so
6.1静态库创建静态库步骤:
1. 编写库文件代码,编译为.o 目标文件。
2. ar 命令 创建 libxxxx.a 文件
ar -rsv libxxxx.a xxxx.o
注意:1 静态库名字要以lib开头,后缀名为.a
2 没有main函数的.c 文件不能生成可执行文件。
3.链接静态库:
gcc -o 目标文件 源码.c -L 路径 -l xxxx
-L 表示库所在的路径
-l 后面跟库的名称
注:-L. .表示当前目录
链接错误:
test.c:(.text+0x15):对‘hello’未定义的引用
collect2: error: ld returned 1 exit status
含义:表示hello函数在编译的源码内没有找到实现
解决:实现代码或者找到对应函数的库并且链接它。
6.2动态库的生成步骤:
1.生成位置无关代码的目标文件
gcc -c -fPIC xxx.c xxxx.c ....
2.生成动态库
gcc -shared -o libxxxx.so xxx.o xxx.o ....
3.编译可执行文件
gcc -o 目标文件 源码.c -L路径 -lxxxx
-- dynamic为自定义动态库文件名 --L.表示本目录
这里直接执行t1文件会出错,因为动态库文件找不到,需要用到下面的方法解决:
执行动态库的可执行文件错误
./test: error while loading shared libraries: libmyheby.so: cannot open shared object file: No such file or directory
含义:可执行文件所使用的动态库找不到(方法2只能在本窗口使用,方法3用脚本可解决)
解决办法:
1.找到动态库,添加到/usr/lib里面
2.或使用export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:你的动态库目录(终端直接输入)
3.上述命令添加在~/.bashrc 文件里面(vim ~/.bashrc),使用source ~/.bashrc 生效。
6.3查看可执行文件使用的动态库:
ldd 命令 : ldd 你的可执行文件
正常时:
root@haas-virtual-machine:/mnt/hgfs/share/newIOP# ldd test
linux-vdso.so.1 => (0x00007fff6548d000)
libmyheby.so => /mnt/hgfs/share/newIOP/day5/libmyheby.so (0x00007f5c89521000)
libc.so.6 => /lib/x86_64-linux-gnu/libc.so.6 (0x00007f5c89144000)
/lib64/ld-linux-x86-64.so.2 (0x000055fe52211000)
出错时:
root@haas-virtual-machine:/mnt/hgfs/share/newIOP/day5# ldd test
linux-vdso.so.1 => (0x00007ffcb652c000)
libmyheby.so => not found
libc.so.6 => /lib/x86_64-linux-gnu/libc.so.6 (0x00007fbeeffaf000)
/lib64/ld-linux-x86-64.so.2 (0x0000561003c3b000)
作业
遍历目录,打印所有文件的相关信息
#include<stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <time.h>
#include<dirent.h>
#include <string.h>
int main(int argc, const char *argv[])
{
DIR *dp;
struct dirent *dt;
struct stat buf;
int ret;
//遍历目标目录所有文件
//打开目录
char path[] = "/home/linux/";
dp = opendir(path);
if(dp == NULL){
perror("stat_t01: ");
return 0;
}
//读取目录
while((dt = readdir(dp)) != NULL){
//获取文件属性
char temppath[100] = {0};
sprintf(temppath,"%s%s",path,dt->d_name);
ret = stat(temppath,&buf);
if(ret < 0){
perror("stat_t.c02: ");
return 0;
}
//判断文件类型
if(S_ISREG(buf.st_mode)){
printf("-");
}
if(S_ISDIR(buf.st_mode)){
printf("d");
}
if(S_ISCHR(buf.st_mode)){
printf("c");
}
if(S_ISBLK(buf.st_mode)){
printf("b");
}
if(S_ISFIFO(buf.st_mode)){
printf("p");
}
if(S_ISSOCK(buf.st_mode)){
printf("s");
}
//文件权限
int i;
for(i = 8; i >= 0;i--){
if(buf.st_mode & (1<<i)){
switch(i%3){
case 2:
printf("r");
break;
case 1:
printf("w");
break;
case 0:
printf("x");
break;
}
}else{
printf("-");
}
}
//打印文件大小
printf(" %d",(int)buf.st_size);
//打印时间信息
struct tm *loct;
loct = localtime(&buf.st_ctime);
printf(" %04d-%02d-%02d %02d:%02d:%02d",loct->tm_year+1900,loct->tm_mon+1,loct->tm_mday,
loct->tm_hour,loct->tm_min,loct->tm_sec);
printf("\n");
}
//关闭目录
closedir(dp);
return 0;
}