使用创建数据库
use '数据库名称'
插入,增加数据
db.user.insert({"name":"xjt"})
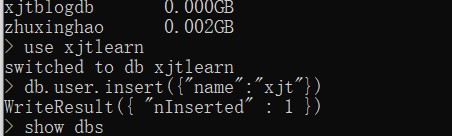
展示数据库的表
show collections
展示表的数据
> db.user.find()
{ "_id" : ObjectId("6121d6122a72a5ac17821a1d"), "name" : "xjt" }
查找具体的数据
{age:{$gte:22,$lte:25}}
> db.user.find()
{ "_id" : ObjectId("6121d9702a72a5ac17821a1f"), "age" : 22 }
{ "_id" : ObjectId("6121d9b92a72a5ac17821a20"), "age" : 25 }
> db.user.find({"age":22})
{ "_id" : ObjectId("6121d9702a72a5ac17821a1f"), "age" : 22 }
> db.user.find({age:{$gt:22}})
{ "_id" : ObjectId("6121d9b92a72a5ac17821a20"), "age" : 25 }
> db.user.find({age:{$lt:24}})
{ "_id" : ObjectId("6121d9702a72a5ac17821a1f"), "age" : 22 }
> db.user.find({"name":/zhangsan/})
>db.user.find({"name":/^zhang/})
>db.user.find({"name":/zhang$/})
>db.user.find({},{name:1})
>db.user.find({},{age:1})
>db.user.find({"age":{$gte:20}},{age:1})
>db.user.find({"name":"wangzu","age":25})
>db.user.find().limt(2)
>db.user.find().skip(10)
排序
>db.user.find().sort({"age":1})
>db.user.find().sort({"age":1})
删除数据库
> db.dropDatabase()
{ "ok" : 1 }
删除某一个表(集合)
> db.tokens.drop()
true
连贯操作
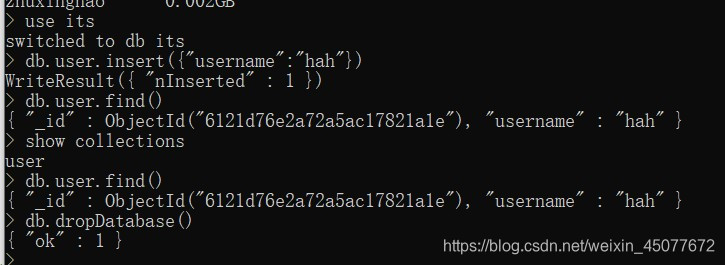
查询统计数据库数据条数
> use xjts
switched to db xjts
> for(var i=0;i<99;i++){
... db.user.insert({"name":"zhangsan"+i,"age":i})
... };
> db.user.find().count()
99
> db.user.find({age:{$gte:66}}).count()
33
> db.user.find().skip(0).limt(10)
> db.user.find().skip(10).limt(10)
>
(3-1)*10
> db.user.find().skip(20).limt(10)
算法:skip的page-1然后乘以pageSzie,然后limt(pageSize)
or 与查询
> db.user.find({$or:[{age:22},{age:25}]})
{ "_id" : ObjectId("6121f464e9b431717ff1f417"), "name" : "zhangsan22", "age" : 22 }
{ "_id" : ObjectId("6121f464e9b431717ff1f41a"), "name" : "zhangsan25", "age" : 25 }
修改数据
> db.user.update({age:25},{$set:{age:66666}})
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.user.find({age:66666})
{ "_id" : ObjectId("6121f464e9b431717ff1f41a"), "name" : "zhangsan25", "age" : 66666 }
> db.user.update({age:66666},{$set:{age:655,sex:"男人"}})))
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 }) })
> db.user.find({age:655})
{ "_id" : ObjectId("6121f464e9b431717ff1f41a"), "name" : "zhangsan25", "age" : 655, "sex" : "男人" }
> db.user.update({age:655},{age:888})
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.user.find({age:888})
{ "_id" : ObjectId("6121f464e9b431717ff1f41a"), "age" : 888 }
> db.user.update({age:{$lte:5}},{$set:{"sex":"男"}})
WriteResult({ "nMatched" : 1, "nUpserted" : 0, "nModified" : 1 })
> db.user.find().limit(5) )
{ "_id" : ObjectId("6121f464e9b431717ff1f401"), "name" : "zhangsan0", "age" : 0, "sex" : "男" }
{ "_id" : ObjectId("6121f464e9b431717ff1f402"), "name" : "zhangsan1", "age" : 1 }
{ "_id" : ObjectId("6121f464e9b431717ff1f403"), "name" : "zhangsan2", "age" : 2 }
{ "_id" : ObjectId("6121f464e9b431717ff1f404"), "name" : "zhangsan3", "age" : 3 }
{ "_id" : ObjectId("6121f464e9b431717ff1f405"), "name" : "zhangsan4", "age" : 4 }
> db.user.update({age:{$lte:5}},{$set:{"sex":"男"}},{multi:true})
WriteResult({ "nMatched" : 6, "nUpserted" : 0, "nModified" : 5 })
> db.user.find().limit(5) )
{ "_id" : ObjectId("6121f464e9b431717ff1f401"), "name" : "zhangsan0", "age" : 0, "sex" : "男" }
{ "_id" : ObjectId("6121f464e9b431717ff1f402"), "name" : "zhangsan1", "age" : 1, "sex" : "男" }
{ "_id" : ObjectId("6121f464e9b431717ff1f403"), "name" : "zhangsan2", "age" : 2, "sex" : "男" }
{ "_id" : ObjectId("6121f464e9b431717ff1f404"), "name" : "zhangsan3", "age" : 3, "sex" : "男" }
{ "_id" : ObjectId("6121f464e9b431717ff1f405"), "name" : "zhangsan4", "age" : 4, "sex" : "男" }
>
删除数据
>db.user.remove({age:22})
>db.user.remove({age:{$lte:10},{justOne:true}})
WriteResult({ "nRemoved" : 11 })