Note: Regarding the code implementation of the algorithm, you can either write the pseudo-code by hand or use computer programming. If you are ready to paste the code into a document, you can take a screenshot of the whole code or submit the source file of the code. Because many students choose to paste the code directly into a word document, but the word layout is really too difficult to read.
Traversal of a binary tree:
- A binary tree is known to have a preorder traversal sequence and a inorder traversal sequence of abdgcefh and dgbaechf, respectively, find the binary tree and the postorder traversal sequence.
- Please implement four ways of traversing a binary tree. Preorder traversal.
Inorder traversal. Postorder traversal. Levelorder traversal
binary search tree:
Please implement an algorithm to find the specified value from a binary search tree. Return true if it exists and false if it does not.
AVL tree:
Select a set of 10 nodes with data of 2, 1, 0, 3, 4, 5, 6, 9, 8, 7 respectively. Construct a AVL tree from these 10 nodes and draw the construction process.
(i)
#include <iostream>
using namespace std;
// A utility function to search x in arr[] of size n
int search(int arr[], int x, int n)
{
for (int i = 0; i < n; i++)
if (arr[i] == x)
return i;
return -1;
}
// Prints postorder traversal from given inorder and preorder traversals
void printPostOrder(int in[], int pre[], int n)
{
// The first element in pre[] is always root, search it
// in in[] to find left and right subtrees
int root = search(in, pre[0], n);
// If left subtree is not empty, print left subtree
if (root != 0)
printPostOrder(in, pre + 1, root);
// If right subtree is not empty, print right subtree
if (root != n - 1)
printPostOrder(in + root + 1, pre + root + 1, n - root - 1);
// Print root
cout << pre[0] << " ";
}
// Driver program to test above functions
int main()
{
int in[] = { 4, 2, 5, 1, 3, 6 };
int pre[] = { 1, 2, 4, 5, 3, 6 };
int n = sizeof(in) / sizeof(in[0]);
cout << "Postorder traversal " << endl;
printPostOrder(in, pre, n);
return 0;
(i)
#include <iostream>
using namespace std;
// A utility function to search x in arr[] of size n
int search(int arr[], int x, int n)
{
for (int i = 0; i < n; i++)
if (arr[i] == x)
return i;
return -1;
}
// Prints postorder traversal from given inorder and preorder traversals
void printPostOrder(int in[], int pre[], int n)
{
// The first element in pre[] is always root, search it
// in in[] to find left and right subtrees
int root = search(in, pre[0], n);
// If left subtree is not empty, print left subtree
if (root != 0)
printPostOrder(in, pre + 1, root);
// If right subtree is not empty, print right subtree
if (root != n - 1)
printPostOrder(in + root + 1, pre + root + 1, n - root - 1);
// Print root
cout << pre[0] << " ";
}
// Driver program to test above functions
int main()
{
int in[] = { 4, 2, 5, 1, 3, 6 };
int pre[] = { 1, 2, 4, 5, 3, 6 };
int n = sizeof(in) / sizeof(in[0]);
cout << "Postorder traversal " << endl;
printPostOrder(in, pre, n);
return 0;
(ii)
#include <iostream>
using namespace std;
struct Node {
int data;
struct Node *left, *right;
};
Node* newNode(int data)
{
Node* temp = new Node;
temp->data = data;
temp->left = temp->right = NULL;
return temp;
}
//print postorder
void printPostorder(struct Node* node)
{
if (node == NULL)
return;
printPostorder(node->left);
printPostorder(node->right);
cout << node->data << " ";
}
// print inorder
void printInorder(struct Node* node)
{
if (node == NULL)
return;
printInorder(node->left);
cout << node->data << " ";
printInorder(node->right);
}
//print preorder
void printPreorder(struct Node* node)
{
if (node == NULL)
return;
//print root data
cout << node->data << " ";
//call left side
printPreorder(node->left);
//call right side
printPreorder(node->right);
}
int main()
{
struct Node* root = newNode(1);
root->left = newNode(2);
root->right = newNode(3);
root->left->left = newNode(4);
root->left->right = newNode(5);
cout << "\nPreorder traversal of binary tree is \n";
printPreorder(root);
cout << "\nInorder traversal of binary tree is \n";
printInorder(root);
cout << "\nPostorder traversal of binary tree is \n";
printPostorder(root);
return 0;
OUTPUT:
(iii)
(iv)
bool search(struct node* root, int key)
{
// Base Cases: root is null or key is present at root
if (root == NULL || root->key == key)
return 1;
// Key is greater than root's key
if (root->key < key)
return search(root->right, key);
// Key is smaller than root's key
return search(root->left, key);
}
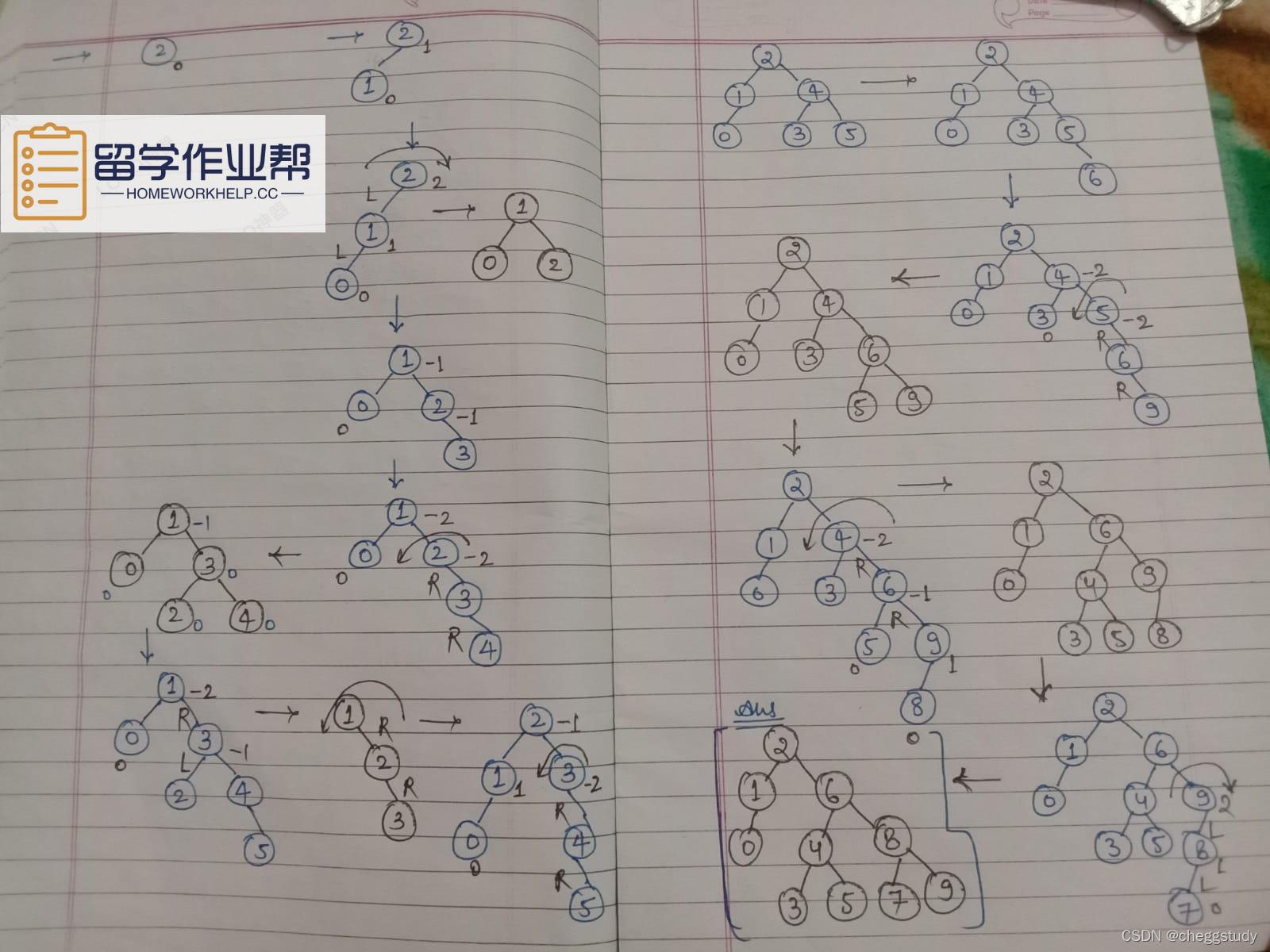
OTE: If you find it helpful then plz do give an upvote , also if you have any query then do let me know.
```cpp
(由留学作业帮www.homeworkhelp.cc整理编辑)
Bartleby|Bookrags|brainly.com|Coursehero|Chegg|eNotes|Ebook|gradebuddy|Grammerlly|Numerade |QuillBot|Oneclass|Studypool|SaveMyEaxms|Studymode|ScholarOne|SlideShare|SkillShare|Scribd|SolutionInn|Study.com|Studyblue|Termpaperwarehouse|and more…